更改 Vuex 的 store 中的状态的唯一方法是提交 mutation:
组件中commit提交,传统写法
<template>
<div>
<h2>当前计数: {{ $store.state.counter }}</h2>
<hr>
<button @click="$store.commit('increment')">+1</button>
<button @click="$store.commit('decrement')">-1</button>
<button @click="addTen">+10</button>
<hr>
</div>
</template>
<script>
import { INCREMENT_N } from '../store/mutation-types'
export default {
methods: {
addTen() {
//带参数的操作
this.$store.commit('incrementN', 10)
this.$store.commit('incrementN', {n: 10, name: "why", age: 18})
// 另外一种提交风格
this.$store.commit({
type: incrementN,
n: 10,
name: "why",
age: 18
})
//将名字设置一个常量抽取出来
this.$store.commit({
type: INCREMENT_N,
n: 10,
name: "why",
age: 18
})
}
}
}
</script>
mutation的辅助函数
<template>
<div>
<h2>当前计数: {{ $store.state.counter }}</h2>
<hr>
<button @click="increment">+1</button>
<button @click="add">+1</button>
<button @click="decrement">-1</button>
<button @click="increment_n({n: 10})">+10</button>
<hr>
</div>
</template>
<script>
import { mapMutations, mapState } from 'vuex'
import { INCREMENT_N } from '../store/mutation-types'
export default {
methods: {
...mapMutations(["increment", "decrement", INCREMENT_N]),
...mapMutations({
add: "increment"
})
},
setup() {
const storeMutations = mapMutations(["increment", "decrement", INCREMENT_N])
return {
...storeMutations
}
}
}
</script>
store下的index.js中
很多时候我们在提交mutation的时候,会携带一些数据,这个时候我们可以使用参数:payload
import { INCREMENT_N } from './mutation-types'
mutations: {
increment(state) {
state.counter++;
},
decrement(state) {
state.counter--;
},
// 10 -> payload
// {n: 10, name: "why", age: 18} -> payload
//[INCREMENT_N]为抽取的常量,不抽取为incrementN
[INCREMENT_N](state, payload) {
console.log(payload);
state.counter += payload.n
},
addBannerData(state, payload) {
state.banners = payload
}
},
payload为对象类型
若是抽取常量,还需在index.js中再建mutation-types.js
export const INCREMENT_N = "increment_n"
总结来说,Mutation常量类型时为下面三步
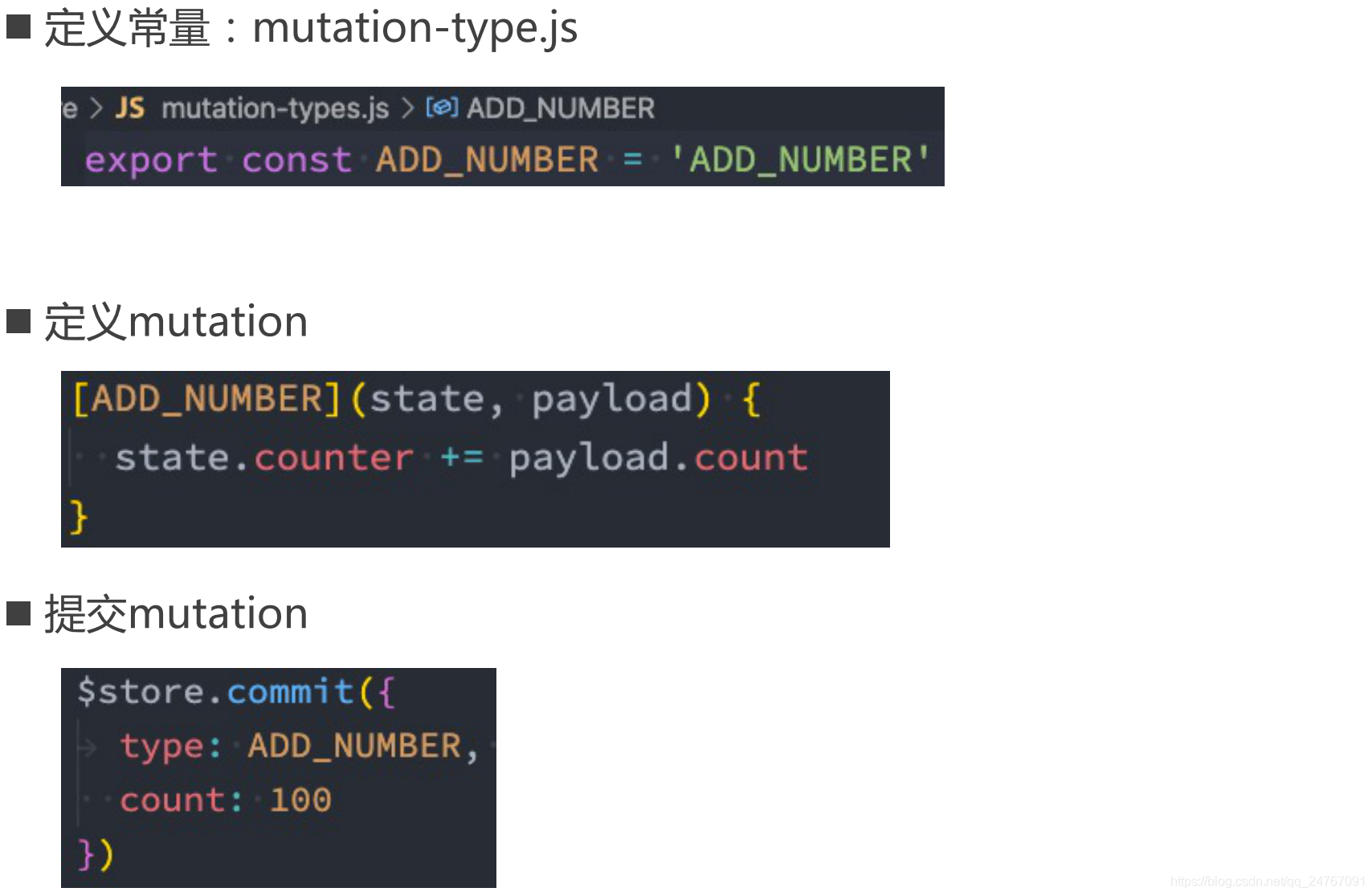
mutation重要原则
一条重要的原则就是要记住 mutation 必须是同步函数
这是因为devtool工具会记录mutation的日记;
每一条mutation被记录,devtools都需要捕捉到前一状态和后一状态的快照;
但是在mutation中执行异步操作,就无法追踪到数据的变化;
所以Vuex的重要原则中要求 mutation必须是同步函数;