from collections import deque
grid = [
[0, 0, 1, 0, 0, 0, 0],
[0, 0, 1, 1, 0, 0, 0],
[0, 0, 0, 0, 1, 0, 0],
[0, 0, 0, 1, 1, 0, 0],
[1, 0, 0, 0, 1, 0, 0],
[1, 1, 1, 0, 0, 0, 0],
[1, 1, 1, 0, 0, 0, 0],
]
def findPath(start, finish, grid):
m, n, newx, newy, l = len(grid), len(grid[0]), 0, 0, 0
# 起始点 == 终止点
if start == finish:
return len
# 四周加墙
for i in range(m):
grid[i][0] = grid[i][-1] = 1
for i in range(n):
grid[0][i] = grid[-1][i] = 1
grid[start[0]][start[1]] = 2
dct = [(0, 1), (1, 0), (0, -1), (-1, 0)]
here = start
Q = deque()
p(grid)
# 查找路径
while True:
for d in dct:
newx = here[0] + d[0]
newy = here[1] + d[1]
if grid[newx][newy] == 0: # 未被选中
grid[newx][newy] = grid[here[0]][here[1]] + 1
p(grid)
if (newx, newy) == finish:
break
Q.append((newx, newy))
if (newx, newy) == finish:
break
if len(Q) == 0:
break
here = Q.pop()
# p(grid)
# 计算路径
pl = grid[finish[0]][finish[1]] - 2
path = []
here = finish
for j in range(pl - 1, -2, -1):
path.append(here)
for d in dct:
newx = here[0] + d[0]
newy = here[1] + d[1]
if grid[newx][newy] == j + 2:
break
here = (newx, newy)
print(path[::-1])
return len
def p(grid):
for i in range(len(grid)):
print(grid[i])
print('\n')
findPath((2, 1), (3, 5), grid)
运行过程
[1, 1, 1, 1, 1, 1, 1]
[1, 0, 1, 1, 0, 0, 1]
[1, 2, 0, 0, 1, 0, 1]
[1, 0, 0, 1, 1, 0, 1]
[1, 0, 0, 0, 1, 0, 1]
[1, 1, 1, 0, 0, 0, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 0, 1, 1, 0, 0, 1]
[1, 2, 3, 0, 1, 0, 1]
[1, 0, 0, 1, 1, 0, 1]
[1, 0, 0, 0, 1, 0, 1]
[1, 1, 1, 0, 0, 0, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 0, 1, 1, 0, 0, 1]
[1, 2, 3, 0, 1, 0, 1]
[1, 3, 0, 1, 1, 0, 1]
[1, 0, 0, 0, 1, 0, 1]
[1, 1, 1, 0, 0, 0, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 3, 1, 1, 0, 0, 1]
[1, 2, 3, 0, 1, 0, 1]
[1, 3, 0, 1, 1, 0, 1]
[1, 0, 0, 0, 1, 0, 1]
[1, 1, 1, 0, 0, 0, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 3, 1, 1, 0, 0, 1]
[1, 2, 3, 0, 1, 0, 1]
[1, 3, 4, 1, 1, 0, 1]
[1, 0, 0, 0, 1, 0, 1]
[1, 1, 1, 0, 0, 0, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 3, 1, 1, 0, 0, 1]
[1, 2, 3, 0, 1, 0, 1]
[1, 3, 4, 1, 1, 0, 1]
[1, 4, 0, 0, 1, 0, 1]
[1, 1, 1, 0, 0, 0, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 3, 1, 1, 0, 0, 1]
[1, 2, 3, 0, 1, 0, 1]
[1, 3, 4, 1, 1, 0, 1]
[1, 4, 5, 0, 1, 0, 1]
[1, 1, 1, 0, 0, 0, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 3, 1, 1, 0, 0, 1]
[1, 2, 3, 0, 1, 0, 1]
[1, 3, 4, 1, 1, 0, 1]
[1, 4, 5, 6, 1, 0, 1]
[1, 1, 1, 0, 0, 0, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 3, 1, 1, 0, 0, 1]
[1, 2, 3, 0, 1, 0, 1]
[1, 3, 4, 1, 1, 0, 1]
[1, 4, 5, 6, 1, 0, 1]
[1, 1, 1, 7, 0, 0, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 3, 1, 1, 0, 0, 1]
[1, 2, 3, 0, 1, 0, 1]
[1, 3, 4, 1, 1, 0, 1]
[1, 4, 5, 6, 1, 0, 1]
[1, 1, 1, 7, 8, 0, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 3, 1, 1, 0, 0, 1]
[1, 2, 3, 0, 1, 0, 1]
[1, 3, 4, 1, 1, 0, 1]
[1, 4, 5, 6, 1, 0, 1]
[1, 1, 1, 7, 8, 9, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 3, 1, 1, 0, 0, 1]
[1, 2, 3, 0, 1, 0, 1]
[1, 3, 4, 1, 1, 0, 1]
[1, 4, 5, 6, 1, 10, 1]
[1, 1, 1, 7, 8, 9, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 3, 1, 1, 0, 0, 1]
[1, 2, 3, 0, 1, 0, 1]
[1, 3, 4, 1, 1, 11, 1]
[1, 4, 5, 6, 1, 10, 1]
[1, 1, 1, 7, 8, 9, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 3, 1, 1, 0, 0, 1]
[1, 2, 3, 0, 1, 12, 1]
[1, 3, 4, 1, 1, 11, 1]
[1, 4, 5, 6, 1, 10, 1]
[1, 1, 1, 7, 8, 9, 1]
[1, 1, 1, 1, 1, 1, 1]
[(2, 1), (3, 1), (4, 1), (4, 2), (4, 3), (5, 3), (5, 4), (5, 5), (4, 5), (3, 5), (2, 5)]
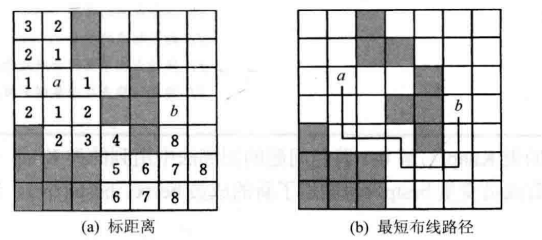
布线问题-分支限界法
最新推荐文章于 2022-11-21 13:42:42 发布