- 不知不觉java 8已经是个很多年前的版本了,但是他真的很强大,有其是Stream相关类,用好了用习惯了真的很难再去使用for 循环配合其他逻辑去写一些繁琐的方法。我用了很久的Stream类,其中有很多因为使用而发现的API,今天做了个整理,分享给大家。
- Stream类主要是对集合进行各种快捷的处理,他是流式,或者说是管道式的处理,与我们平常的直接循环调用各种API而言他的API速度要更快。
- Stream可以认为是一步一步的,链式调用的,有步骤的API,从流程上可以分为:创建流、数据处理、数据收集三种类型。
- 其中数据处理的API最多,大致可以分为以下几种操作类型:筛选与切片、映射与排序、查找与匹配、规约与收集。
- Stream主要是对集合类,即Collection类的处理为主。接下来开始结合代码讲解,本文只讲解常用的,过于生僻的或者差不多的笔者在此不多赘述。
- 首先是第一部分,创建流。
String[] str = {"5", "2", "3", "4", "1"};
List<String> strList = Arrays.asList(str);
Stream<String> stream1 = strList.stream();
Stream<String> stream2 = strList.parallelStream();
Stream<List<String>> stream3 = Stream.of(strList);
- 通过以上方法就可以获取到一个新的stream对象了,接下来则是数据处理部分的API。
private static List<StudentModel> list= Arrays.asList(
new StudentModel("1","张三",11,"男"),
new StudentModel("2","李四",22,"女"),
new StudentModel("3","王五",21,"男"),
new StudentModel("4","赵六",17,"女"),
new StudentModel("4","赵六",17,"女"),
new StudentModel("4","赵六",17,"女")
);
private static void test3(){
Stream<StudentModel> stream = list.stream().filter(a -> a.getAge() > 15);
list.stream().filter(a->a.getAge()>15).forEach(param-> System.out.println(param));
System.out.println("over-filter");
list.stream().filter(a->a.getAge()>15).limit(2).forEach(param-> System.out.println(param));
System.out.println("over-limit");
list.stream().skip(2).forEach(param-> System.out.println(param));
System.out.println("over-skip");
list.stream().distinct().forEach(param-> System.out.println(param));
}
private static List<StudentModel> list= Arrays.asList(
new StudentModel("1","张三",11,"男"),
new StudentModel("2","李四",22,"女"),
new StudentModel("3","王五",21,"男"),
new StudentModel("4","赵六",17,"女"),
new StudentModel("4","赵其",17,"女"),
new StudentModel("4","赵芭",17,"女")
);
public static void test1(){
List<String> stringList = Arrays.asList("aa", "bb", "cc", "dd");
stringList.stream().map(str->str.toUpperCase()).forEach(str-> System.out.println(str));
Stream<Stream<Character>> streamStream = stringList.stream().map(ThirdTest::filterCharacter);
Stream<Character> characterStream = stringList.stream().flatMap(ThirdTest::filterCharacter);
list.stream().map(model->model.getName()).forEach(name-> System.out.println(name));
Stream<String> newStrList = stringList.stream().flatMap(str -> Stream.of(str.toUpperCase()));
newStrList.forEach(str-> System.out.println(str));
}
private static List<StudentModel> list= Arrays.asList(
new StudentModel("1","张三",11,"男", StudentModel.Status.FREE),
new StudentModel("2","李四",22,"女", StudentModel.Status.BUSY),
new StudentModel("3","王五",21,"男", StudentModel.Status.MOYU),
new StudentModel("4","赵六",17,"女",StudentModel.Status.FREE),
new StudentModel("4","赵其",17,"女",StudentModel.Status.BUSY),
new StudentModel("4","赵其",17,"女",StudentModel.Status.BUSY),
new StudentModel("4","赵芭",17,"女",StudentModel.Status.FREE)
);
private static void test1(){
boolean a = list.stream().allMatch(student -> student.getStatus().equals(StudentModel.Status.BUSY));
System.out.println(a+"--a");
boolean b =list.stream().anyMatch(student -> student.getStatus().equals(StudentModel.Status.BUSY));
System.out.println(b+"--b");
boolean c =list.stream().noneMatch(student -> student.getStatus().equals(StudentModel.Status.BUSY));
System.out.println(c+"--c");
Optional<StudentModel> first = list.stream().findFirst();
System.out.println(first+"---first");
Optional<StudentModel> any = list.stream().findAny();
System.out.println(any+"---any");
Optional<StudentModel> any1 = list.parallelStream().findAny();
System.out.println(any1.get()+"---any1");
long count = list.stream().count();
System.out.println(count+"--count");
Optional<StudentModel> max = list.stream().max((e1,e2) -> Integer.compare(e1.getAge(),e2.getAge()));
System.out.println(max.get()+"--max");
Optional<StudentModel> min = list.stream().min((e1, e2) -> Integer.compare(e1.getAge(),e2.getAge()));
System.out.println(min.get()+"--min");
Optional<Integer> minSimple = list.stream().map(student -> student.getAge()).min(Integer::compareTo);
System.out.println(minSimple.get()+"--minSimple");
}
- 接下来则是数据收集部分的API了,数据收集可以概括为将处理完的数据存为一个新的对象或者新的集合。
private static List<StudentModel> list= Arrays.asList(
new StudentModel("1","张三",11,"男", StudentModel.Status.FREE),
new StudentModel("2","李四",22,"女", StudentModel.Status.BUSY),
new StudentModel("3","王五",21,"男", StudentModel.Status.MOYU),
new StudentModel("4","赵六",17,"女",StudentModel.Status.FREE),
new StudentModel("4","赵其",17,"女",StudentModel.Status.BUSY),
new StudentModel("4","赵其",17,"女",StudentModel.Status.BUSY),
new StudentModel("4","赵芭",17,"女",StudentModel.Status.FREE)
);
private static void test2(){
List<Integer> integerList = Arrays.asList(1, 2, 3, 4, 5, 6);
Integer reduceInteger = integerList.stream().reduce(0, (x, y) -> x + y);
System.out.println(reduceInteger+"--reduceInteger");
Integer reduceModel = list.stream().map(studentModel -> studentModel.getAge()).reduce(0, (x, y) -> x + y);
System.out.println(reduceModel+"--reduceModel");
Optional<Integer> reduceModelInOptional = list.stream().map(StudentModel::getAge).reduce(Integer::sum);
System.out.println(reduceModelInOptional.get()+"--reduceModelInOptional");
}
private static void test3(){
Stream<String> nameStream = list.stream().map(studentModel -> studentModel.getName());
List<String> nameList = nameStream.collect(Collectors.toList());
nameList.forEach(name-> System.out.println(name));
System.out.println("nameList-------------------------");
Stream<String> nameStreamSecond = list.stream().map(studentModel -> studentModel.getName());
Set<String> nameSet = nameStreamSecond.collect(Collectors.toSet());
nameSet.forEach(name-> System.out.println(name));
System.out.println("nameSet-------------------------");
}
- 以上是我在学习 stream时学到的API,接下来放上一些我自己在实际开发中发现的一些小技巧及特殊的API吧。
AtomicReference<Integer> inventory= new AtomicReference<>(0);
list.forEach(obj->
inventory.updateAndGet(v -> v + obj.getInventory()));
vo.setInventory(inventory.get());
int inventory = list.stream().
mapToInt(GoodsDetailSkuRel::getInventory).sum();
vo.setInventory(inventory);
List<Object> collect= list.stream().
filter(item-> item.getIsSeckill()!=null && item.getIsSeckill().equals(0))
.collect(Collectors.toList());
map.get(user.getId()).stream().
mapToLong(param->param.getRipeBeansNum()).sum();
stream().peek(item->{
});
Map<String, List<T>> collect = list.stream().collect(Collectors.groupingBy(
item -> String.join(StrUtil.DASHED, this.getPropertiesValueStrs(item, properties))
, Collectors.toList())
);
Map<String, List<String>> businessLineMap = channelInfoDtos.stream()
.filter(item -> channelInfoIds.contains(item.getChannelId()))
.collect(Collectors.groupingBy(ChannelInfoDto::getBusinessLine,
Collectors.mapping(ChannelInfoDto::getChannelName, Collectors.toList())));
- 以上记载了笔者认为最为常用的API,希望能帮到大家。
留言:老婆做了一个小红书账号,全是瓷砖方面的干货,大佬们如果有需求或者有兴趣可以移步了解一下,嘻嘻~
小红书地址,GO GO GO!!!
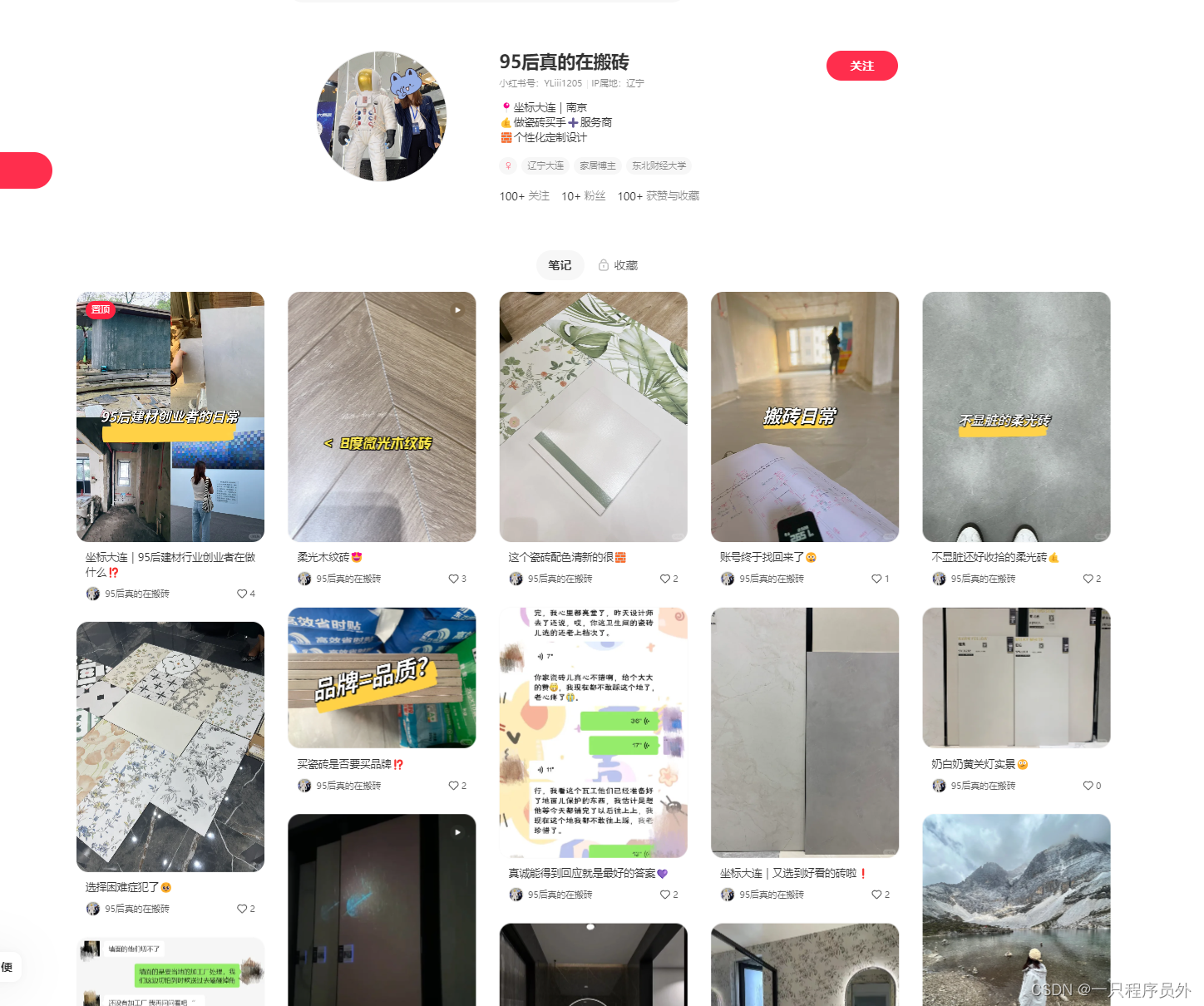