众所周知,递归思想在许多算法里尤为重要,尤其是遍历和搜索里,所以总结下:
递归算法的实质是:
把求解问题转化为规模缩小了的同类问题的子问题,然后递归调用函数(或子过程)来表示问题的解,通过多次递归调用,最终可求出最小问题的解,然后通过这个最小问题的解返回上层调用,再求出次小问题的解,
再返回上层调用,不断重复,最终得到整个问题的解,完成递归操作。
注意点:
1、使用递归时,必须有一个明确的递归结束条件,称为递归出口
2、递归效率较低,容易堆栈溢出
我们通过几个例题来详细了解下递归的思想。
例题一、求阶乘(子函数递归)
#include <cstdio>
int print(int x)
{
if(x<=1)
return 1;
else
return x*print(x-1);
}
int main()
{
int i,n;
printf("请输入要求阶乘的一个整除:");
scanf("%d",&n);
printf("结果为:%d\n",print(n));
return 0;
}
例题二、进制转换
从运行窗口很明显能发现递归返回的过程,每次调用子过程。
#include <cstdio>
#include <cstring>
char bit[]={"0123456789ABCDEF"};
void convert(char *s,int n,int b)
{
if(n==0)
{
strcpy(s,"");
return;
}
convert(s,n/b,b);
int len;
len=strlen(s);
s[len]=bit[n%b];
printf("********* %d %c\n",len,s[len]);
s[len+1]='\0';
}
int main()
{
int base,n,i;
char a[50];
printf("请输入十进制数:");
scanf("%d",&n);
printf("请输入转换的进制:");
scanf("%d",&base);
convert(a,n,base);
printf("%s\n",a);
return 0;
}
例题三、DFS题
经典:http://acm.hdu.edu.cn/showproblem.php?pid=1016
A ring is compose of n circles as shown in diagram. Put natural number 1, 2, ..., n into each circle separately, and the sum of numbers in two adjacent circles should be a prime.
Note: the number of first circle should always be 1.
Note: the number of first circle should always be 1.
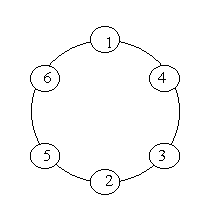
Input
n (0 < n < 20).
Output
The output format is shown as sample below. Each row represents a series of circle numbers in the ring beginning from 1 clockwisely and anticlockwisely. The order of numbers must satisfy the above requirements. Print solutions in lexicographical order.
You are to write a program that completes above process.
Print a blank line after each case.
You are to write a program that completes above process.
Print a blank line after each case.
Sample Input
6 8
Sample Output
Case 1: 1 4 3 2 5 6 1 6 5 2 3 4 Case 2: 1 2 3 8 5 6 7 4 1 2 5 8 3 4 7 6 1 4 7 6 5 8 3 2 1 6 7 4 3 8 5 2
n个座位编号为1~n,要求求出两两相邻和为素数的方案。
典型的DFS,搜索每个状态,不符合则递归
#include<stdio.h>
#include<string.h>
int prime[40]={0,1,1,1,0,1,0,1,0,0,0,1,0,1,0,0,0,1,0,1,0,0,0,1,0,0,0,0,0,1,0,1,0,0,0,0,0,1,0,0};
//素数打表,因为n最大是20,所以只要打到40
int visited[21],a[21],n;
void dfs(int num)//深搜,num为个数
{
int i;
if(num==n && prime[a[num-1]+a[0]]) //满足条件了,就输出来
{
for(i=0;i<num-1;i++)
printf("%d ",a[i]);
printf("%d\n",a[num-1]);
}
else
{
for(i=2;i<=n;i++)
{
if(visited[i]==0)//是否用过了
{
if(prime[i+a[num-1]]) //是否和相邻的加起来是素数
{
visited[i]=1;//标记了
a[num++]=i;//放进数组
dfs(num); //递归调用
visited[i]=0; //退去标记
num--;
}
}
}
}
}
int main()
{
int num=0;
while(~scanf("%d",&n))
{
num++;
printf("Case %d:\n",num);
memset(visited,0,sizeof(visited));
a[0]=1;
dfs(1);
printf("\n");
}
return 0;
}