导入依赖:
<!--easypoi 导入导出 -->
<dependency>
<groupId>cn.afterturn</groupId>
<artifactId>easypoi-base</artifactId>
<version>4.2.0</version>
</dependency>
<dependency>
<groupId>cn.afterturn</groupId>
<artifactId>easypoi-web</artifactId>
<version>4.2.0</version>
</dependency>
<dependency>
<groupId>cn.afterturn</groupId>
<artifactId>easypoi-annotation</artifactId>
<version>4.2.0</version>
</dependency>
主表
package com.diye.cloud.project.modular.competition.vo;
import cn.afterturn.easypoi.excel.annotation.Excel;
import cn.afterturn.easypoi.excel.annotation.ExcelCollection;
import lombok.Data;
import java.math.BigDecimal;
import java.util.Date;
import java.util.List;
/**
* 导出送出培训信息接收类(主表)
* 2022年7月26日09:16:43
* 张小辉
*/
@Data
public class CmpInfoExportVo {
private String id;
@Excel(name="培训性质",needMerge = true,replace = {"计划内_0","计划外_1"},width = 20)
private String planProperties;
@Excel(name="类别",needMerge = true,replace = {"国网_0","省专业部门_1","系统外_2"},width = 20)
private String projectType;
@Excel(name="培训班名称",needMerge = true,width = 30)
private String className;
@Excel(name="开始时间" , format = "yyyy-MM-dd",needMerge = true,width = 20)
private Date planStartTime;
@Excel(name="结束时间" , format = "yyyy-MM-dd",needMerge = true,width = 20)
private Date planEndTime;
@Excel(name="培训地点",needMerge = true,width = 20)
private String address;
@Excel(name="培训天数",needMerge = true,width = 20)
private Integer planDays;
@Excel(name="费用标准",needMerge = true,width = 20)
private BigDecimal bidPrice;
@Excel(name="总人数",needMerge = true,width = 20)
private Integer peopleNum;
@ExcelCollection(name = "")
private List<StudentInfoExportVo> students;
}
子表
package com.diye.cloud.project.modular.competition.vo;
import cn.afterturn.easypoi.excel.annotation.Excel;
import lombok.Data;
/**
* 导出送出培训-学生信息接收类(子表)
* 2022年7月26日09:24:20
* 张小辉
*/
@Data
public class StudentInfoExportVo {
@Excel(name="参培单位",width = 20)
private String companyName;
@Excel(name="参培部门",width = 20)
private String deptName;
@Excel(name="姓名",width = 20)
private String name;
@Excel(name="学历",width = 20)
private String education;
@Excel(name="职务",width = 20)
private String post;
@Excel(name="岗位",width = 20)
private String station;
@Excel(name="技术等级",width = 20)
private String technicalLevel;
@Excel(name="技能等级",width = 20)
private String skillLevel;
}
controller
```java
@SneakyThrows
@PostResource(name = "根据条件查询导出", path = "/exportExcel", requiredPermission = false)
@ApiOperation(value = "根据条件查询导出")
public void exportExcel(@RequestBody CmpParam param,
HttpServletResponse response) {
param = buildCmpParam(param);
List<CmpInfoExportVo> exportList = cmpService.getExportList(param);
List<StudentInfoExportVo> studentList = new ArrayList<>();
for (CmpInfoExportVo cmpInfoExportVo : exportList) {
//根据id去查询学员
studentList = cmpStudentMaterialsService.getExportList(cmpInfoExportVo.getId());
cmpInfoExportVo.setStudents(studentList);
Integer peopleNum = cmpService.getPeopleNumber(cmpInfoExportVo.getId());
if(ToolUtil.isNotEmpty(peopleNum)){
cmpInfoExportVo.setPeopleNum(peopleNum);
}else{
cmpInfoExportVo.setPeopleNum(0);
}
}
// 简单模板导出方法
ExportParams params = new ExportParams();
params.setSheetName("送出培训");//设置sheet名
Workbook workbook = ExcelExportUtil.exportExcel(params, CmpInfoExportVo.class, exportList);
try {
response.setCharacterEncoding("UTF-8");
response.setHeader("content-Type", "application/vnd.ms-excel");
response.setHeader("Content-Disposition", "attachment;filename=" +
URLEncoder.encode("送出培训导出", "UTF-8"));
OutputStream ouputStream = response.getOutputStream();
workbook.write(ouputStream);
ouputStream.flush();
ouputStream.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
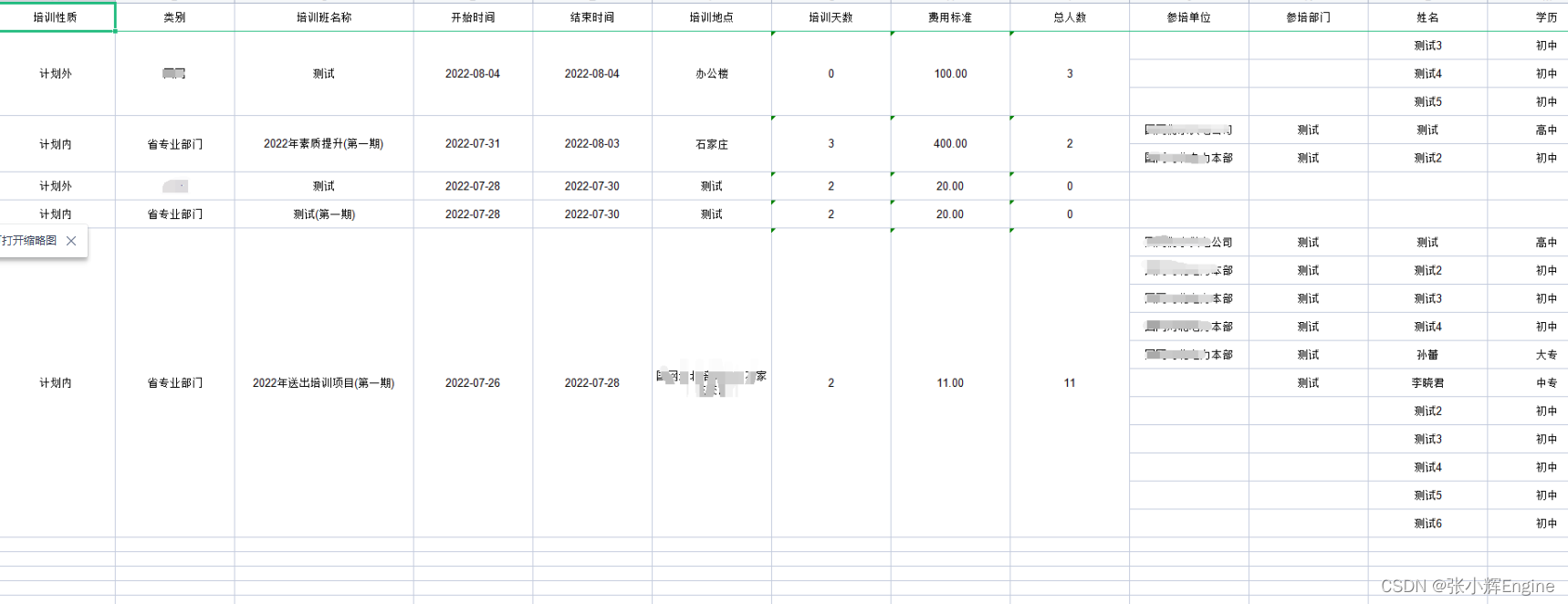
同时可参考easypoi一对多复杂导入:https://blog.csdn.net/qq_26144365/article/details/126947292