pom文件坐标:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>net.sourceforge.nekohtml</groupId>
<artifactId>nekohtml</artifactId>
<version>1.9.22</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
application.properties文件配置:
spring.datasource.url=jdbc:mysql://127.0.0.1:3306/basedb?characterEncoding=utf-8
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
spring.datasource.username=root
spring.datasource.password=root
spring.jpa.show-sql=true
spring.jpa.hibernate.ddl-auto=update
spring.thymleaf.cache = false
spring.thymeleaf.mode=HTML
spring.thymeleaf.suffix=.html
cbs.imagesPath=file:/E:/images/
实体类就不说了,主要是上传图片文件部分,上传之前,因为上传的图片不是放在项目下,而是其他路径,这里是E:/images/路径下,为了能在浏览器中能访问到E:/images/下的图片,需要构建一个虚拟地址:
@Configuration
public class MyWebAppConfigurer extends WebMvcConfigurerAdapter {
// 获取配置文件中图片的路径
@Value("${cbs.imagesPath}")
private String mImagesPath;
// 访问图片方法
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
if (mImagesPath.equals("") || mImagesPath.equals("${cbs.imagesPath}")) {
String imagesPath = MyWebAppConfigurer.class.getClassLoader().getResource("").getPath();
if (imagesPath.indexOf(".jar") > 0) {
imagesPath = imagesPath.substring(0, imagesPath.indexOf(".jar"));
} else if (imagesPath.indexOf("classes") > 0) {
imagesPath = "file:" + imagesPath.substring(0, imagesPath.indexOf("classes"));
}
imagesPath = imagesPath.substring(0, imagesPath.lastIndexOf("/")) + "/images/";
mImagesPath = imagesPath;
System.out.println(mImagesPath);
}
LoggerFactory.getLogger(MyWebAppConfigurer.class).info("imagesPath=" + mImagesPath);
registry.addResourceHandler("/images/**").addResourceLocations(mImagesPath);
super.addResourceHandlers(registry);
}
}
接下来就是上传文件的处理:
//处理文件上传
@RequestMapping(value="/uploadimg")
public @ResponseBody Map<String,Object> demo(@RequestParam(value = "editormd-image-file", required = false) MultipartFile file, HttpServletRequest request) {
Map<String,Object> resultMap = new HashMap<String,Object>();
String fileName = file.getOriginalFilename();
//保存
try {
byte[] bytes = file.getBytes();
Path path = Paths.get(UPLOADED_FOLDER + file.getOriginalFilename());
Files.write(path, bytes);
resultMap.put("success", 1);
resultMap.put("message", "上传成功!");
resultMap.put("url","../images/"+fileName);
} catch (Exception e) {
resultMap.put("success", 0);
resultMap.put("message", "上传失败!");
e.printStackTrace();
}
System.out.println(resultMap.get("success"));
return resultMap;
}
后端部分已经写好,现在来处理前端部分
网上有很多Markdown编辑器,可以自行百度,这是用的是editormd这款富文本编辑器,把下载好的editormd文件夹加到static文件夹中:
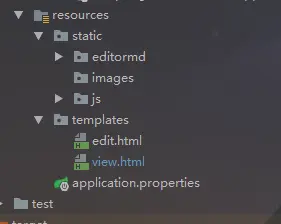
这里写图片描述
edit.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="utf-8"/>
<meta http-equiv="X-UA-Compatible" content="IE=edge"/>
<meta name="viewport" content="width=device-width, initial-scale=1"/>
<title>Edit</title>
<link rel="stylesheet" th:href="@{/editormd/css/editormd.min.css}"
href="/editormd/css/editormd.min.css"/>
</head>
<body>
<div>
<input type="text" name="title" style="width: 500px" id="title"/>
<input type="submit" title="发布" id="submitBtn" />
</div>
<!--class="editormd"-->
<div id="test-editormd">
<textarea style="display:none;" name="test-editormd-markdown-doc" id="content"></textarea>
<!-- 第二个隐藏文本域,用来构造生成的HTML代码,方便表单POST提交,这里的name可以任意取,后台接受时以这个name键为准 -->
<textarea class="editormd-html-textarea" name="text" id="htmlContent"></textarea>
</div>
<script th:src="@{/js/jquery.min.js}" src="/js/jquery.min.js"></script>
<script th:src="@{/editormd/js/editormd.min.js}" src="/editormd/js/editormd.min.js"></script>
<script type="text/javascript">
$(function() {
editormd("test-editormd", {
width: "1000px",
height: 640,
syncScrolling : "single",
//你的lib目录的路径,我这边用JSP做测试的
tocm : true, // Using [TOCM]
tex : true, // 开启科学公式TeX语言支持,默认关闭
flowChart : true, // 开启流程图支持,默认关闭
path : "/editormd/lib/",
//这个配置在simple.html中并没有,但是为了能够提交表单,使用这个配置可以让构造出来的HTML代码直接在第二个隐藏的textarea域中,方便post提交表单。
saveHTMLToTextarea : true,
imageUpload : true,
imageFormats : [ "jpg", "jpeg", "gif", "png", "bmp", "webp" ],
imageUploadURL : "/uploadimg",
onload: function () {
this.width("100%");
this.height(480);
}
});
$("#submitBtn").click(
function () {
submitblog();
}
)
function submitblog() {
var title = $("#title").val();
var content = $("#content").val();
var htmlContent = $("#htmlContent").val();
$.ajax({
url: "submit",
data: {title: title, content:content,htmlContent:htmlContent},
success:function () {
alert("发布成功");
},
error:function () {
alert("发布失败");
}
})
}
});
</script>
</body>
</html>
启动项目:
- 编辑文章
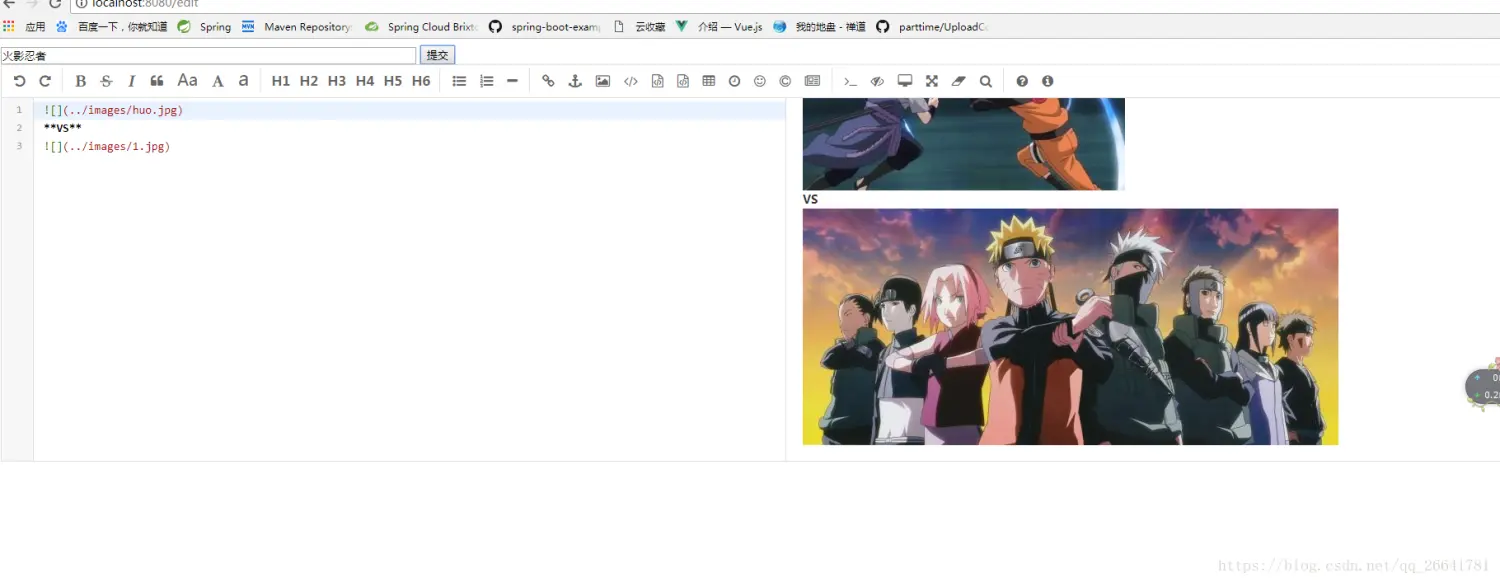
这里写图片描述
- 查看文章
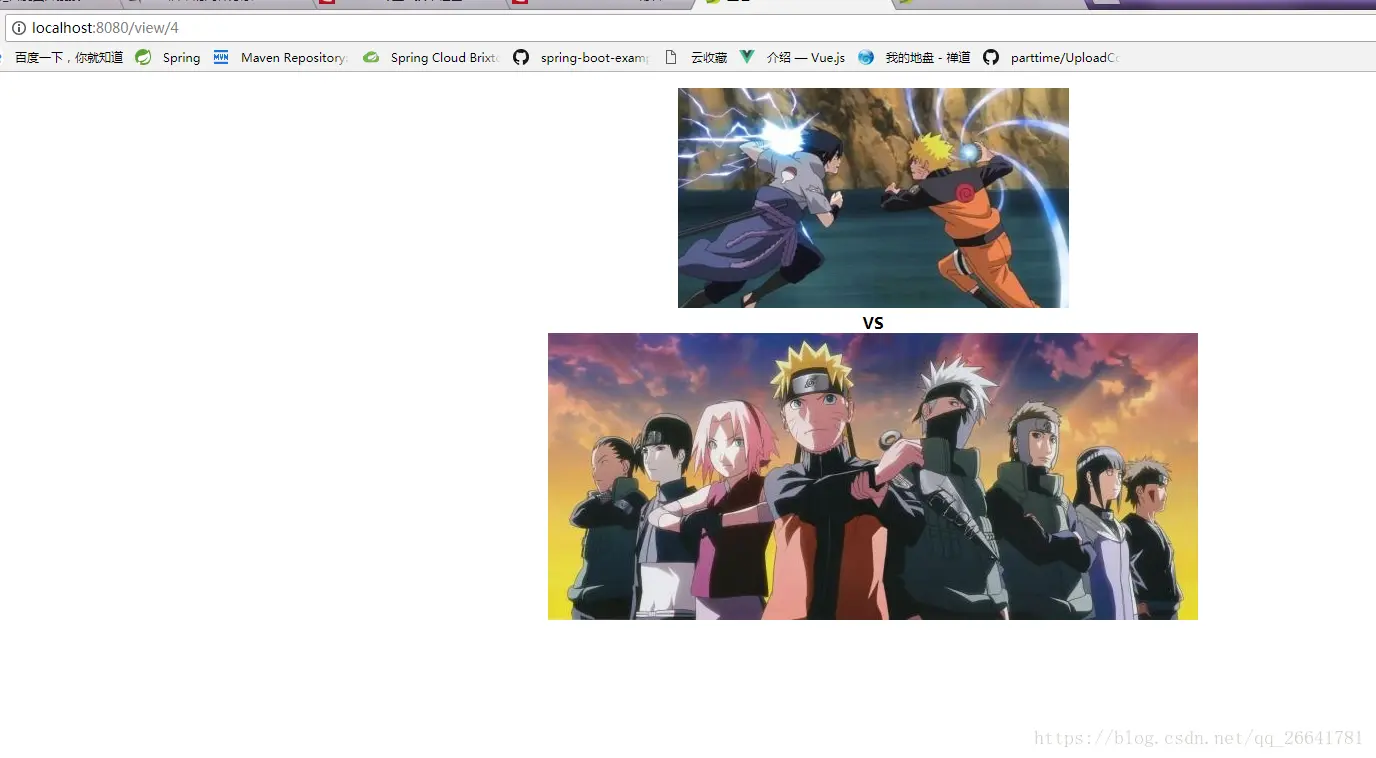
这里写图片描述