一、自定义登录页面
我们不能每次都去使用SpringSecurity默认的很简单的登录页面样式去进行登录,需要我们去自定义自己的登录页面的话,我们怎么实现呢?
在BrowserSecurityConfig类中的configure方法中,我在http.formLogin()的后面去添加一行代码“.loginPage(“url”)”,那么在用户访问页面的时候就会去根据配置的这个url去访问相对应的自定义登录页面。
1.写页面
位置:在src->main->resources中我新建了一个文件夹resources,然后在此文件夹下创建文件“tinner-signIn.html”
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>登录</title>
</head>
<body>
<h1>标准登录页面</h1>
<h3>表单登录</h3>
<form action="/authentication/form" method="post">
<table>
<tr>
<td>用户名:</td>
<td><input type="text" name="username"/></td>
</tr>
<tr>
<td>密码:</td>
<td><input type="password" name="password"/></td>
</tr>
<tr>
<td colspan="2"><button type="submit">登录</button></td>
</tr>
</table>
</form>
</body>
</html>
2.自定义配置
@Configuration
public class BrowserSecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private SecurityProperties securityProperties;
@Bean
public PasswordEncoder passwordEncoder(){
return new BCryptPasswordEncoder();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
// super.configure(http);
//实现的效果:让它去表单登录,而不是alert框
http.formLogin()
.loginPage("/tinner-signIn.html")
.loginProcessingUrl("/authentication/form")
.and()
.authorizeRequests()//对请求进行授权
.antMatchers("/tinner-signIn.html").permitAll()
.anyRequest()//任何请求
.authenticated()//都需要身份认证
.and().csrf().disable();
}
}
可以看到我额外地添加了两行代码,一个是指定了登录的页面,另一个是对于"/tinner-signIn.html"这个页面,我们必须将它放行,不能让它去进行资源权限的校验,否则会发生“重定向次数过多”(死循环)。csrf().disable(); 是跨站请求伪造的安全机制。SpringSecurity默认地会有csrf跨站请求伪造防护的机制。因为是从一个html,进行post去提交给服务器的,涉及到跨站请求。
二、自定义登录改进
虽然可以用一个自定义的html页面来完成表单登录,但是现在出现了两个问题:
- 在起始的时候发送的是一个rest服务的请求,但是在跳转控制上返回去的是一个html,这种方式是不合理的。rest服务应该返回的是状态码和Json的信息。我们需要做的是如果是html请求就跳到登录页上,如果不是就返回Json数据
- 我写了一个标准的登录页面,但是我希望能提供一个可重用的安全模块(即有多个项目都会使用这个安全木块,但是这些项目不可能只用这一个简单样式的登录页面)。如何能让这些项目使用自己自定义的登录页面?如果不使用自己定义的登录页面,才会使用我自己写的这个简单样式的登录页面。
基于以上问题,我来对这个安全模块进行改进:
1、处理不同类型的请求
先来看看整体的流程图
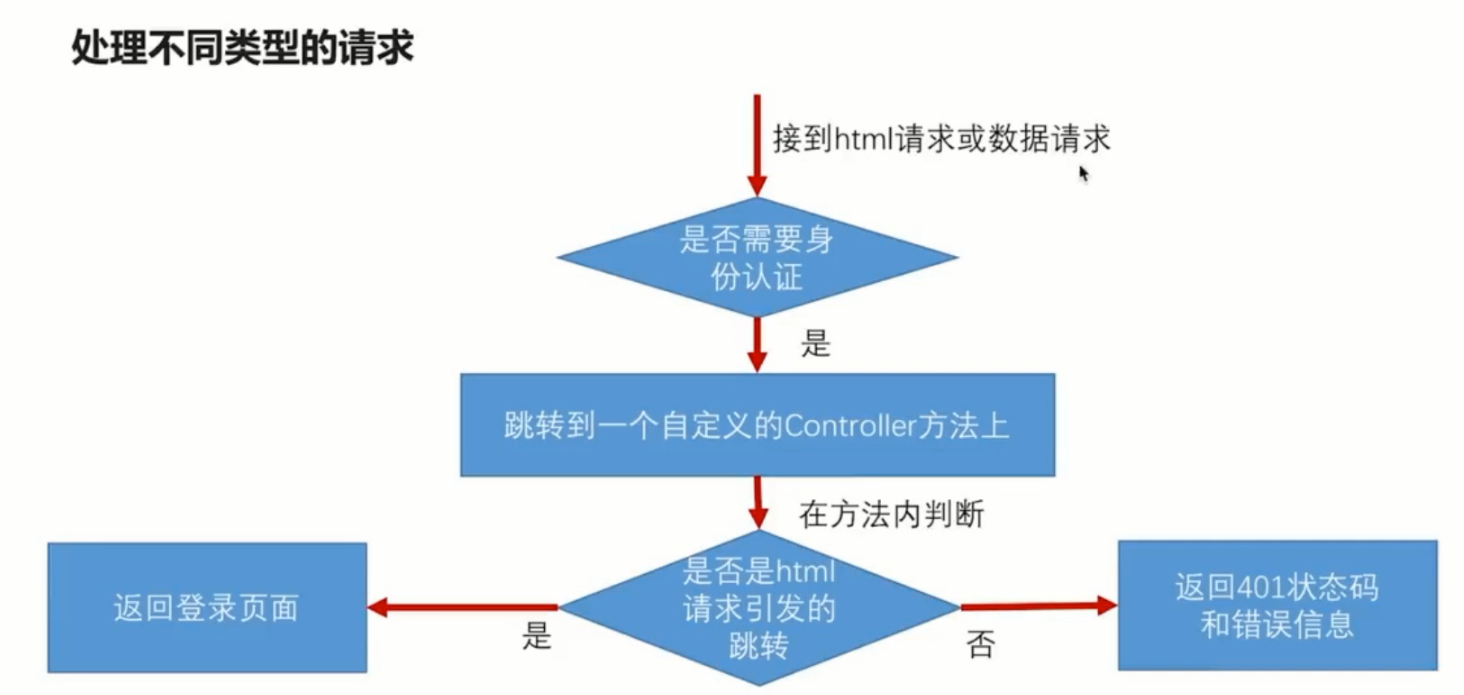
<1>定义返回数据类型
在写这个Controller时,我们需要知道,对于html请求,我们不可能永远跳转到一个死的登录页面上去,我需要提供一个能力,去读取配置文件实现活的返回页面。
在这个方法上,我返回的是一个http的状态码。
这个方法应该返回的是一个自定义的类,里面去返回各种各样的属性,因此我来创建一个返回类SimpleResponse ,里面只有一个Object类型的变量
public class SimpleResponse {
public SimpleResponse(Object content) {
this.content = content;
}
private Object content;
public Object getContent() {
return content;
}
public void setContent(Object content) {
this.content = content;
}
}
<2>将页面配置写活
返回的数据类型定义完了,就需要去将配置的自定义页面去写活,在这里我们使用demo项目去引用Browser项目,在demo的配置文件中我定义了一个html
tinner.security.browser.loginPage=/login.html
我希望如果demo项目做了这个页面配置就跳转到demo配置的自定义页面,如果没有进行配置则跳转到browser项目定义的登录页面。
为了实现这个逻辑,我需要去将这些配置进行封装,将它们封装到一个类里面中去。
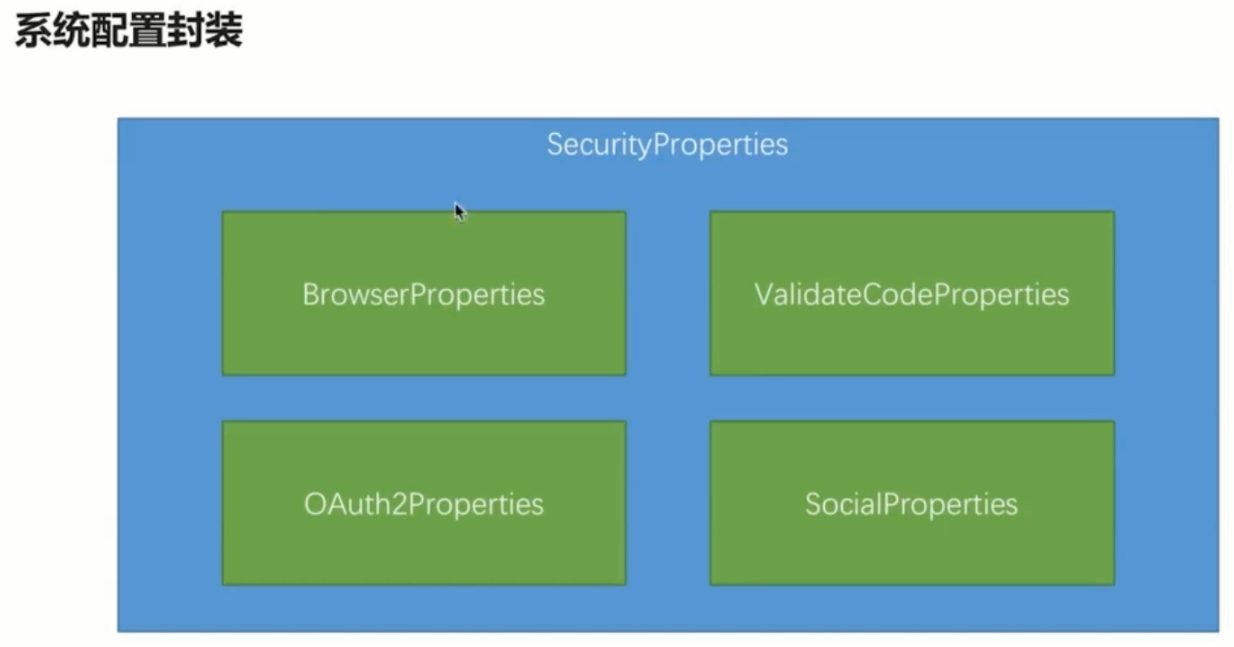
这个图就是对整个项目配置文件的封装,我会去定义一个SecurityProperties类,然后这个类中又分为几个子类(根据配置项的不同去划分)。
代码我写在了Tinner-security-core项目中
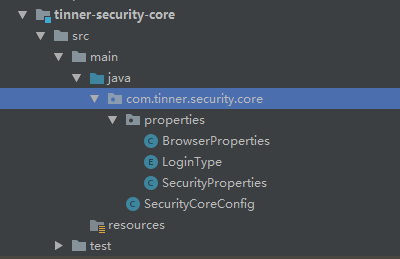
因为系统的配置无论是浏览器模块还是app模块中都会用到。
先去定义BrowserProperties类
public class BrowserProperties {
private String loginPage = "/tinner-signIn.html";
private LoginType loginType = LoginType.JSON;
public LoginType getLoginType() {
return loginType;
}
public void setLoginType(LoginType loginType) {
this.loginType = loginType;
}
public String getLoginPage() {
return loginPage;
}
public void setLoginPage(String loginPage) {
this.loginPage = loginPage;
}
}
然后去定义SecurityProperties类
import org.springframework.boot.context.properties.ConfigurationProperties;
@ConfigurationProperties(prefix = "tinner.security")
public class SecurityProperties {
private BrowserProperties browser = new BrowserProperties();
public BrowserProperties getBrowser() {
return browser;
}
public void setBrowser(BrowserProperties browser) {
this.browser = browser;
}
}
可以看到在SecurityProperties类中包含了BrowserProperties,BrowserProperties类中有一个属性loginPage,默认值是browser模块中的自定义标准登录页面,LoginType定义了登录的方式,是基于html还是json的,LoginType是一个枚举,其定义如下:
public enum LoginType {
REDIRECT,
JSON;
}
<3>写自定义的Controller
@RestController
public class BrowserSecurityController {
private Logger LOGGER = LoggerFactory.getLogger(this.getClass());
private RequestCache requestCache = new HttpSessionRequestCache();
private RedirectStrategy redirectStrategy = new DefaultRedirectStrategy();
@Autowired
private SecurityProperties securityProperties;
/**
* 当需要身份认证时,跳转到这里
* @param request
* @param response
* @return
*/
@RequestMapping("/authentication/require")
@ResponseStatus(code = HttpStatus.UNAUTHORIZED)
public SimpleResponse requireAuthentication(HttpServletRequest request, HttpServletResponse response) throws IOException {
//判断引发跳转的是html还是不是html
SavedRequest savedRequest = requestCache.getRequest(request,response);
if (savedRequest != null ){
String target = savedRequest.getRedirectUrl();
LOGGER.info("引发跳转的请求是:"+target);
if (StringUtils.endsWithIgnoreCase(target,"html")){
redirectStrategy.sendRedirect(request,response,securityProperties.getBrowser().getLoginPage());
}
}
return new SimpleResponse("访问的服务需要身份认证,请引导用户到登录页");
}
}
然后在BrowserSecurityConfig去进行配置
@Configuration
public class BrowserSecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private SecurityProperties securityProperties;
@Bean
public PasswordEncoder passwordEncoder(){
return new BCryptPasswordEncoder();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
// super.configure(http);
//实现的效果:让它去表单登录,而不是alert框
http.formLogin()
.loginPage("/authentication/require")
.loginProcessingUrl("/authentication/form")
.and()
.authorizeRequests()//对请求进行授权
.antMatchers("/authentication/require",securityProperties.getBrowser().getLoginPage()).permitAll()
.anyRequest()//任何请求
.authenticated()
.and().csrf().disable();//都需要身份认证
}
}
最后这个自定义的controller的逻辑就是跟我流程图里面的逻辑一样,如果请求是一个html请求,去根据配置文件去进行跳转(如果用户配了自定义登录页面就走其对应的页面,如果用户没配置,则走browser默认的标准登录页面去进行登录。)
三、自定义登录成功处理
在默认的处理中,登录成功之后会重定向到在地址栏中输入的url上面,但是往往不满足我们真实的开发场景:比如登录成功后进行签到处理、进行积分的累积等。
如果要实现登录成功处理其实非常简单,只需要实现一个接口(AuthenticationSuccessHandler)即可。
代码写在了browser项目中
@Component("tinnerAuthentivationSuccessHandler")
public class TinnerAuthentivationSuccessHandler implements AuthenticationSuccessHandler {
private Logger LOGGER = LoggerFactory.getLogger(this.getClass());
@Autowired
private ObjectMapper objectMapper;
@Autowired
private SecurityProperties securityProperties;
@Override
public void onAuthenticationSuccess(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse, Authentication authentication) throws IOException, ServletException {
LOGGER.info("登录成功");
httpServletResponse.setContentType("application/json;charset=UTF-8");
httpServletResponse.getWriter().write(objectMapper.writeValueAsString(authentication));
}
}
实现了AuthenticationSuccessHandler接口后,只需要重写onAuthenticationSuccess方法即可,这个方法会在登录成功之后调用,里面除了HttpServletRequest 和HttpServletResponse 对象之外,还有一个Authentication 接口对象,这个接口的作用是封装认证信息,比如认证通过之后的session信息。我在用户登录成功之后将authentication对象中的信息返回给了前台。
然后在BrowserSecurityConfig去进行配置
/**
* WebSecurityConfigurerAdapter是springSecurity提供的一个适配器类
*/
@Configuration
public class BrowserSecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private SecurityProperties securityProperties;
@Autowired
private AuthenticationSuccessHandler tinnerAuthentivationSuccessHandler;
@Bean
public PasswordEncoder passwordEncoder(){
return new BCryptPasswordEncoder();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
// super.configure(http);
//实现的效果:让它去表单登录,而不是alert框
http.formLogin()
.loginPage("/authentication/require")
.loginProcessingUrl("/authentication/form")
.successHandler(tinnerAuthentivationSuccessHandler)
.and()
.authorizeRequests()//对请求进行授权
.antMatchers("/authentication/require",securityProperties.getBrowser().getLoginPage()).permitAll()
.anyRequest()//任何请求
.authenticated()
.and().csrf().disable();//都需要身份认证
}
}
然后我们去访问系统的资源,会先跳转到登录页面,登录成功之后,我会看到一系列的用户相关信息
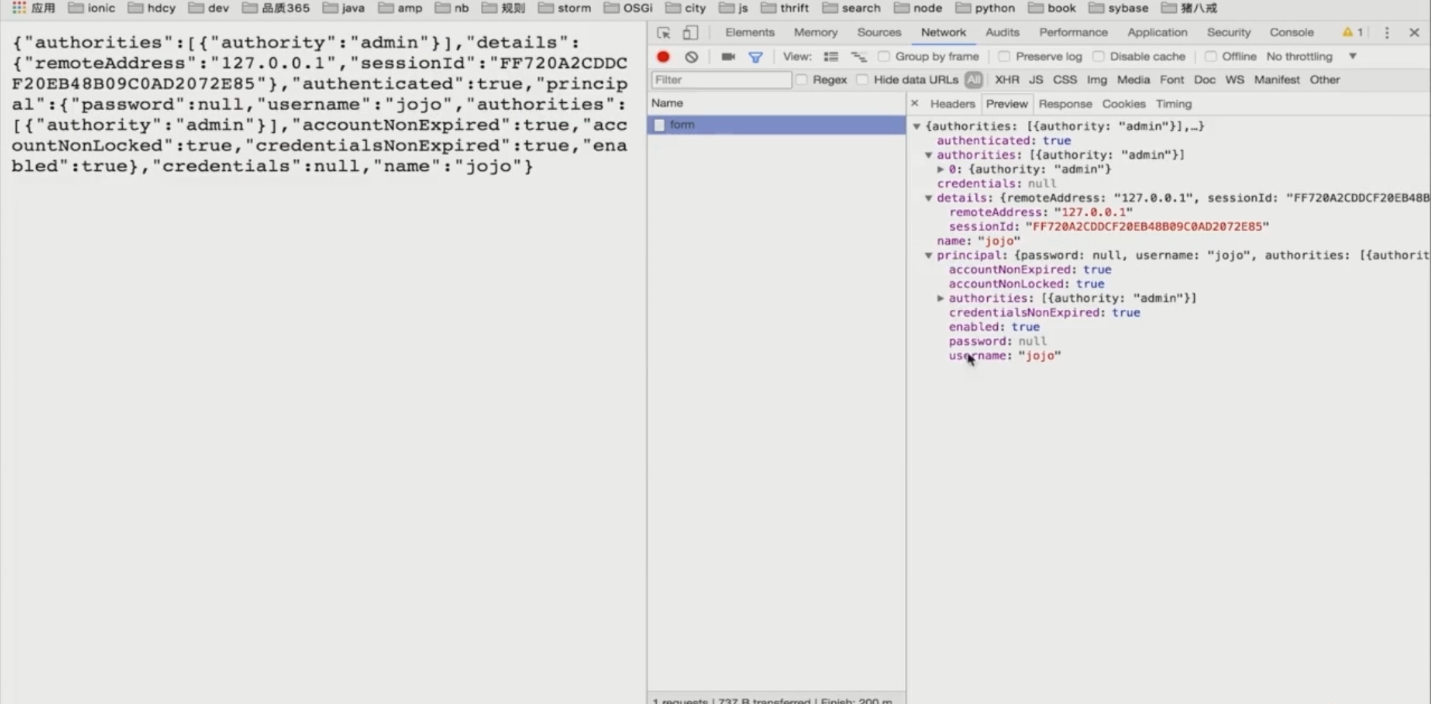
解释:
第一个是authenticated为true,表示用户通过了身份认证
authorities中封装了用户的身份权限信息
credentials是用户输入的用户密码,但是默认的SpringSecurity对其进行了处理返回的是null
details包含了认证请求的一些信息:访问地址以及sessionId
name是用户写的用户名
principal是userdetails里面的内容
在未来如果用到QQ微信授权登录,其authentication信息是不一样的。
四、自定义登录失败处理
SpringSecurity默认的是返回错误信息,比如我们的业务场景需要“连续三次输入密码不允许登录、记录错误日志”等的逻辑。
失败处理也应该是返回一个json对象,只需要实现AuthenticationFailureHandler接口即可
@Component("tinnerAuthentivationFailureHandler")
public class TinnerAuthentivationFailureHandler implements AuthenticationFailureHandler {
private Logger LOGGER = LoggerFactory.getLogger(this.getClass());
@Autowired
private ObjectMapper objectMapper;
@Autowired
private SecurityProperties securityProperties;
@Override
public void onAuthenticationFailure(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse, AuthenticationException e) throws IOException, ServletException {
LOGGER.info("登录失败");
httpServletResponse.setStatus(HttpStatus.INTERNAL_SERVER_ERROR.value());
httpServletResponse.setContentType("application/json;charset=UTF-8");
httpServletResponse.getWriter().write(objectMapper.writeValueAsString(e));
}
}
可以看到,在实现了这个接口之后实现了onAuthenticationFailure方法,其中的第三个参数不再是验证信息的那个对象,而是一个异常,这是因为登录失败的时候会抛出一系列异常(用户名未找到、密码错误等),这个时候登录信息是不完整的,我们就拿不到那个登录验证信息,取而代之的是一个认证过程中发生的错误所包含的异常。
同样,去config中进行配置
/**
* WebSecurityConfigurerAdapter是springSecurity提供的一个适配器类
*/
@Configuration
public class BrowserSecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private SecurityProperties securityProperties;
@Autowired
private AuthenticationSuccessHandler tinnerAuthentivationSuccessHandler;
@Autowired
private AuthenticationFailureHandler tinnerAuthentivationFailureHandler;
@Bean
public PasswordEncoder passwordEncoder(){
return new BCryptPasswordEncoder();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
// super.configure(http);
//实现的效果:让它去表单登录,而不是alert框
http.formLogin()
.loginPage("/authentication/require")
.loginProcessingUrl("/authentication/form")
.successHandler(tinnerAuthentivationSuccessHandler)
.failureHandler(tinnerAuthentivationFailureHandler)
// http.httpBasic()
.and()
.authorizeRequests()//对请求进行授权
.antMatchers("/authentication/require",securityProperties.getBrowser().getLoginPage()).permitAll()
.anyRequest()//任何请求
.authenticated()
.and().csrf().disable();//都需要身份认证
}
}
然后访问系统中的rest服务资源,输入错误的用户密码,然后会受到一个500的请求,里面包含了错误消息以及其堆栈信息。
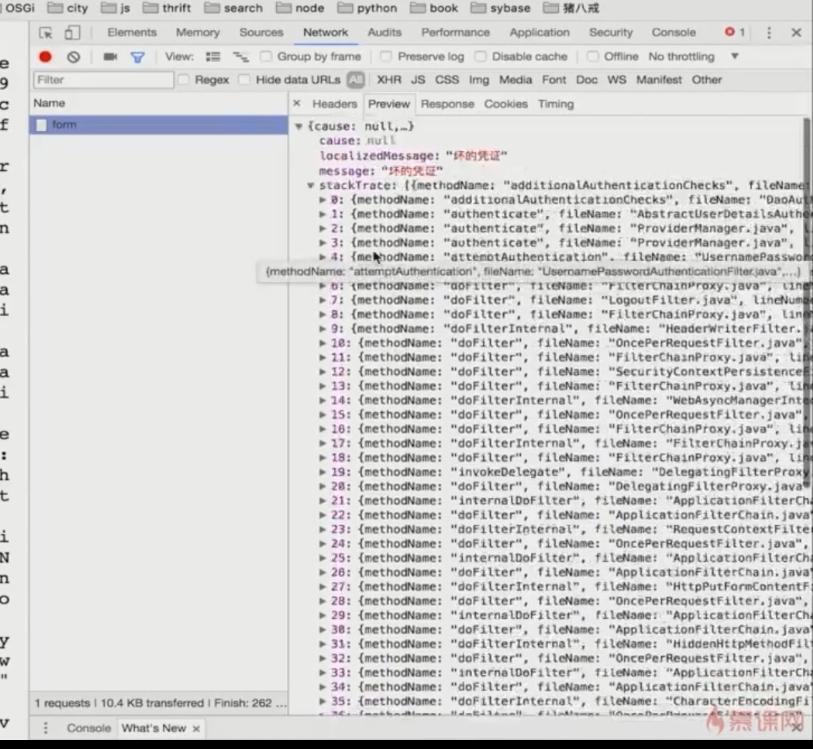
五、改进
如果我把登录和失败的处理方式写死,写成只返回json的方式也是不合适的,因为在某些项目中,我们可能会用到相关模板去进行架构(jsp、freemarker模板等)。它的登录不是异步进行登录的而是同步进行的。我们需要让用户可以进行自己的配置去进行动态地选择同步登录还是异步登录(跳转、返回JSON)。
在LoginType中我定义了两个枚举类型:JSON和redirect。通过判断这个枚举类型来动态地实现登录方式。
然后重新构造登录成功处理器和登录失败处理器:
登录成功处理器:
@Component("tinnerAuthentivationSuccessHandler")
//public class TinnerAuthentivationSuccessHandler implements AuthenticationSuccessHandler {
public class TinnerAuthentivationSuccessHandler extends SavedRequestAwareAuthenticationSuccessHandler {
private Logger LOGGER = LoggerFactory.getLogger(this.getClass());
@Autowired
private ObjectMapper objectMapper;
@Autowired
private SecurityProperties securityProperties;
@Override
public void onAuthenticationSuccess(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse, Authentication authentication) throws IOException, ServletException {
LOGGER.info("登录成功");
if (LoginType.JSON.equals(securityProperties.getBrowser().getLoginType())){
httpServletResponse.setContentType("application/json;charset=UTF-8");
httpServletResponse.getWriter().write(objectMapper.writeValueAsString(authentication));
}else{
super.onAuthenticationSuccess(httpServletRequest,httpServletResponse,authentication);
}
}
}
我们现在没有去实现AuthenticationSuccessHandler 接口,而是去继承了SavedRequestAwareAuthenticationSuccessHandler 类,这个类是SpringSecurity提供的默认的登录成功处理器,然后重写父类的方法,去进行了if判断。
同样的在登录失败处理器中:
@Component("tinnerAuthentivationFailureHandler")
//public class TinnerAuthentivationFailureHandler implements AuthenticationFailureHandler {
public class TinnerAuthentivationFailureHandler extends SimpleUrlAuthenticationFailureHandler {
private Logger LOGGER = LoggerFactory.getLogger(this.getClass());
@Autowired
private ObjectMapper objectMapper;
@Autowired
private SecurityProperties securityProperties;
@Override
public void onAuthenticationFailure(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse, AuthenticationException e) throws IOException, ServletException {
LOGGER.info("登录失败");
if (LoginType.JSON.equals(securityProperties.getBrowser().getLoginType())){
httpServletResponse.setStatus(HttpStatus.INTERNAL_SERVER_ERROR.value());
httpServletResponse.setContentType("application/json;charset=UTF-8");
httpServletResponse.getWriter().write(objectMapper.writeValueAsString(e));
}else{
super.onAuthenticationFailure(httpServletRequest,httpServletResponse,e);
}
}
}
然后如果我在demo项目中指定了登录类型为重定向
tinner.security.browser.loginType=REDIRECT
然后在src->main->resources目录下我定义了一个index.hmtl,然后在浏览器中访问这个html页面,会先重定向到登录页面,输入错误的用户名密码,则跳转到了spring默认的登录失败的页面中(报401错误),然后重新输入一次正确的用户名密码,则自动跳转到了index.hmtl页面中去。