最近比较闲,提前为下个项目中需要用得到功能造个轮子.alertView在项目中应该经常用的到,然而用自带的系统控件多少缺乏点儿激情.于是打算给alertView加上一点点儿动画,(这里的动画用到的是faceBook的POP)提高用户体验.话不多说.看效果图先:
第一个是弹出提示信息后自动消失的alertView
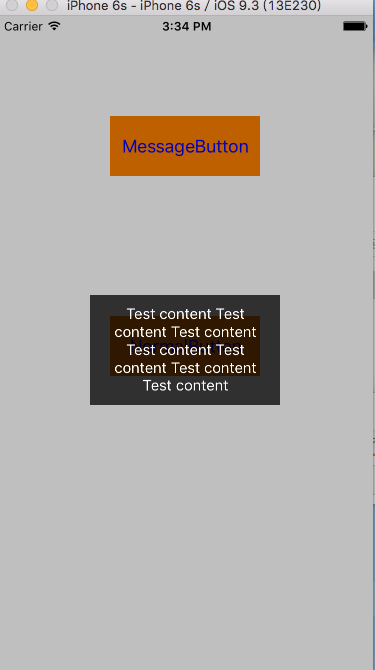
第二个是弹出带有button的alertView
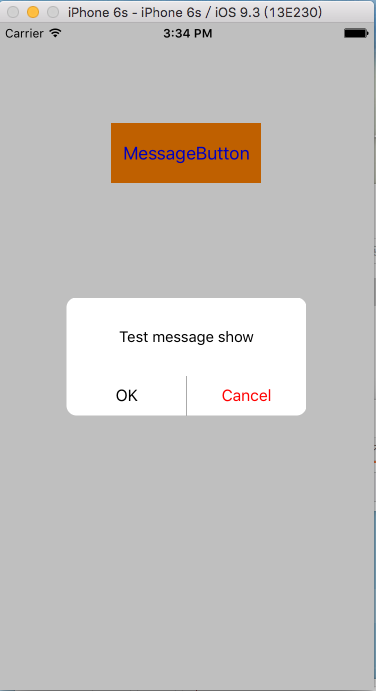
接下来大体说一下思路:
首先创建这两个不同形式alert的基
类
在.h中:AbstractBasicAlertView
基类里面定义了所有需要用到的控件的属性title
subTitle
message
buttonsTitle
contentView
autoHiden
delayAutoHidenDuration
以及方法:-(void)show;
-(void)hide
-(void)setView:(UIView *)view withKey:(NSString *)key;
和-(UIView *)viewWithKey:(NSString *)key;
以及协议方法:协议方法会在viewController中使用到的时候说明他们的意思
- (void)alertView:(AbstractBasicAlertView *)alertView data:(id)data atIndex:(NSInteger)index;
- (void)alertViewWillHide:(AbstractBasicAlertView *)alertView;
- (void)alertViewDidHide:(AbstractBasicAlertView *)alertView;```
在.m中:
声明一个字典:```NSMapTable```这是一个弱引用的字典,也可以替换成NSMutableDictionary.
-
(instancetype)init {
if (self = [super init]) {
self.delayAutoHidenDuration = 2.f; self.autoHiden = NO; self.mapTable = [NSMapTable strongToWeakObjectsMapTable];
}
return self;
}
在初始化方法中,设置自动隐藏延迟2秒,自动隐藏为NO,初始化mapTable
这两个方法是将view根据key放入字典中存起来,在viewController中会有用到
-
(void)setView:(UIView *)view withKey:(NSString *)key {
[self.mapTable setObject:view forKey:key];
} -
(UIView *)viewWithKey:(NSString *)key {
return [self.mapTable objectForKey:key];
}
#####MessageAlertView
基于```AbstractBasicAlertView```创建提示信息的alertView.
复写父类方法:
-
(void)setContentView:(UIView *)contentView {
self.frame = contentView.bounds;
[super setContentView:contentView];
} -
(void)show {
if (self.contentView) {
[self.contentView addSubview:self];
//当show的时候创建背景view和MessageView
[self createBlackView];
[self createMessageView];//如果设置了自动隐藏,执行定时器的方法 if (self.autoHiden) { [self performSelector:@selector(hide) withObject:nil afterDelay:self.delayAutoHidenDuration]; }
}
} -
(void)hide {
if (self.contentView) {
[self removeViews];
}
}
-
(void)createMessageView {
//创建信息label
NSString *text = self.message;
UILabel *textLabel = [[UILabel alloc] initWithFrame:CGRectMake(0, 0, 170, 0)];
textLabel.text = text;
textLabel.font = [UIFont systemFontOfSize:15.f];
textLabel.textColor = [UIColor whiteColor];
textLabel.textAlignment = NSTextAlignmentCenter;
textLabel.numberOfLines = 0;
[textLabel sizeToFit];//创建信息窗体view
self.messageView = [[UIView alloc] initWithFrame:CGRectMake(0, 0, 190, textLabel.height + 20)];
self.messageView.backgroundColor = [[UIColor blackColor] colorWithAlphaComponent:0.75f];
self.messageView.center = self.contentView.middlePoint;
textLabel.center = self.messageView.middlePoint;
self.messageView.alpha = 0.f;
[self.messageView addSubview:textLabel];
[self addSubview:self.messageView];//执行动画
POPBasicAnimation *alpha = [POPBasicAnimation animationWithPropertyNamed:kPOPViewAlpha];
alpha.toValue = @(1.f);
alpha.duration = 0.3f;
[self.messageView pop_addAnimation:alpha forKey:nil];POPSpringAnimation *scale = [POPSpringAnimation animationWithPropertyNamed:kPOPLayerScaleXY];
scale.fromValue = [NSValue valueWithCGSize:CGSizeMake(1.75f, 1.75f)];
scale.toValue = [NSValue valueWithCGSize:CGSizeMake(1.f, 1.f)];
scale.dynamicsTension = 1000;
scale.dynamicsMass = 1.3;
scale.dynamicsFriction = 10.3;
scale.springSpeed = 20;
scale.springBounciness = 15.64;
[self.messageView.layer pop_addAnimation:scale forKey:nil];
}
-
(void)removeViews {
[UIView animateWithDuration:0.2f animations:^{
self.blackView.alpha = 0.f; self.messageView.alpha = 0.f; self.messageView.transform = CGAffineTransformMakeScale(0.75f, 0.75f);
} completion:^(BOOL finished) {
[self removeFromSuperview];
}];
}
#####ButtonsAlertView
其实大部分是模仿alertView构建相似的一个视图
初始化:
-
(instancetype)init {
if (self) {
self = [super init];self.firstButton = [[UIButton alloc] initWithFrame:CGRectZero]; self.secondButton = [[UIButton alloc] initWithFrame:CGRectZero]; self.firstButton.userInteractionEnabled = NO; self.firstButton.tag = 0; self.firstButton.titleLabel.font = [UIFont systemFontOfSize:16]; [self.firstButton setTitleColor:[UIColor blackColor] forState:UIControlStateNormal]; [self.firstButton setTitleColor:[UIColor grayColor] forState:UIControlStateHighlighted]; [self.firstButton addTarget:self action:@selector(messageButtonsEvent:) forControlEvents:UIControlEventTouchUpInside]; self.secondButton.userInteractionEnabled = NO; self.secondButton.tag = 0; self.secondButton.titleLabel.font = [UIFont systemFontOfSize:16]; [self.secondButton setTitleColor:[UIColor blackColor] forState:UIControlStateNormal]; [self.secondButton setTitleColor:[UIColor grayColor] forState:UIControlStateHighlighted]; [self.secondButton addTarget:self action:@selector(messageButtonsEvent:) forControlEvents:UIControlEventTouchUpInside]; //Store View [self setView:self.firstButton withKey:@"firstButton"]; [self setView:self.secondButton withKey:@"secondButton"];
}
return self;
}
重写父类方法:
-
(void)setContentView:(UIView *)contentView {
[super setContentView: contentView];
self.frame = contentView.bounds;
}
-
(void)show {
if (self.contentView) {
[self.contentView addSubview:self]; [self createBlackView]; [self createMessageView];
}
} -
(void)hide {
if (self.contentView) {
[self removeViews];
}
}
创建视图:
-
(void)createBlackView {
self.blackView = [[UIView alloc] initWithFrame:self.contentView.bounds];
self.blackView.backgroundColor = [UIColor blackColor];
self.blackView.alpha = 0;
[self addSubview:self.blackView];[UIView animateWithDuration:0.3f animations:^{
self.blackView.alpha = 0.25f;
}];
} -
(void)createMessageView {
//创建信息label
NSString *text = self.message;
UILabel *textLabel = [[UILabel alloc] initWithFrame:CGRectMake(0, 0, 210, 0)];
textLabel.text = text;
textLabel.font = [UIFont systemFontOfSize:15];
textLabel.textColor = [UIColor blackColor];
textLabel.textAlignment = NSTextAlignmentCenter;
textLabel.numberOfLines = 0;
[textLabel sizeToFit];//创建信息窗体view
self.messageView = [[UIView alloc] initWithFrame:CGRectMake(0, 0, 240, textLabel.height + 100)];
self.messageView.backgroundColor = [UIColor whiteColor];
self.messageView.layer.cornerRadius = 10.f;
self.messageView.center = self.contentView.middlePoint;
textLabel.center = CGPointMake(self.messageView.middleX, 0);
textLabel.top = 30;
self.messageView.alpha = 0.f;
[self.messageView addSubview:textLabel];
[self addSubview:self.messageView];//处理按钮
NSArray *buttonsInfo = self.buttonsTitle;
//如果有一个按钮
if (buttonsInfo.count == 1) {
[self.messageView addSubview:[[self class] lineViewWithFrame:CGRectMake(0, self.messageView.height - 40, self.messageView.width, 0.5f) color:[UIColor lightGrayColor]]];
self.firstButton.frame = CGRectMake(0, self.messageView.height - 40, self.messageView.width, 40);
self.firstButton.userInteractionEnabled = NO;
self.firstButton.tag = 0;
self.firstButton.titleLabel.font = [UIFont systemFontOfSize:16.f];
[self.firstButton setTitle:self.buttonsTitle[0] forState:UIControlStateNormal];
[self.firstButton setTitleColor:[UIColor blackColor] forState:UIControlStateNormal];
[self.firstButton setTitleColor:[UIColor grayColor] forState:UIControlStateHighlighted];
[self.firstButton addTarget:self action:@selector(messageButtonsEvent:) forControlEvents:UIControlEventTouchUpInside];
[self.messageView addSubview:self.firstButton];
}//如果有2个按钮
if (buttonsInfo.count == 2) {
[self.messageView addSubview:[[self class] lineViewWithFrame:CGRectMake(0, self.messageView.height - 40, self.messageView.width, 0.5f) color:[UIColor lightGrayColor]]]; [self.messageView addSubview:[[self class] lineViewWithFrame:CGRectMake(self.messageView.width / 2.f, self.messageView.height - 40, 0.5f, 40.f) color:[UIColor lightGrayColor]]]; self.firstButton.frame = CGRectMake(0, self.messageView.height - 40, self.messageView.width / 2.f, 40); [self.firstButton setTitle:self.buttonsTitle[0] forState:UIControlStateNormal]; [self.messageView addSubview:self.firstButton]; self.secondButton.frame = CGRectMake(self.messageView.width / 2.f, self.messageView.height - 40, self.messageView.width / 2.f, 40); [self.secondButton setTitle:self.buttonsTitle[1] forState:UIControlStateNormal]; [self.messageView addSubview:self.secondButton];
}
//执行动画
POPBasicAnimation *alpha = [POPBasicAnimation animationWithPropertyNamed:kPOPViewAlpha];
alpha.toValue = @(1.f);
alpha.duration = 0.3f;
[self.messageView pop_addAnimation:alpha forKey:nil];POPSpringAnimation *scale = [POPSpringAnimation animationWithPropertyNamed:kPOPLayerScaleXY];
scale.fromValue = [NSValue valueWithCGSize:CGSizeMake(1.75f, 1.75f)];
scale.toValue = [NSValue valueWithCGSize:CGSizeMake(1.f, 1.f)];
scale.dynamicsTension = 1000;
scale.dynamicsMass = 1.3;
scale.dynamicsFriction = 10.3;
scale.springSpeed = 20;
scale.springBounciness = 15.64;
scale.delegate = self;
[self.messageView.layer pop_addAnimation:scale forKey:nil];
} -
(void)removeViews {
[UIView animateWithDuration:0.2f animations:^{
self.blackView.alpha = 0.f; self.messageView.alpha = 0.f; self.messageView.transform = CGAffineTransformMakeScale(0.75f, 0.75f);
} completion:^(BOOL finished) {
[self removeFromSuperview];
}];
}
按钮点击后将代理方法传递出去
- (void)messageButtonsEvent:(UIButton *)button {
if (self.delegate && [self.delegate respondsToSelector:@selector(alertView:data:atIndex:)]) {
[self.delegate alertView:self data:nil atIndex:button.tag];
}
}
#####ViewController中,定义两种alertView的样式
-
(void)btnClick:(UIButton *)btn {
if (btn.tag == 100) {
//MessageButtonAbstractBasicAlertView *alertView = [[MessageAlertView alloc] init]; alertView.message = @"Test content Test content Test content Test content Test content Test content Test content "; alertView.contentView = self.view; alertView.autoHiden = YES; alertView.delayAutoHidenDuration = 5.f; [alertView show];
} else {
//NormalButton
AbstractBasicAlertView *showView = [[ButtonsAlertView alloc] init];
showView.delegate = self;
showView.contentView = self.view;
showView.buttonsTitle = @[@"OK",@"Cancel"];
showView.message = @"Test message show";
UIButton *button = (UIButton *)[showView viewWithKey:@"secondButton"];
[button setTitleColor:[UIColor redColor] forState:UIControlStateNormal];
[showView show];
}
}
执行代理方法,可以在这个店里方法中做一些其他的操作,不过我们alertView的大致作用就是一个提示作用了.
-
(void)alertView:(AbstractBasicAlertView *)alertView data:(id)data atIndex:(NSInteger)index {
[alertView hide];
}
demo地址:https://github.com/irembeu/AnimationAlertView.git