1.需求场景
1.1 在我们公司的国际官网上有一处手机客户意见反馈的地方,见:http://www.alilo-world.com/contact.html 。我们售后的一个需求就是当客户有反馈的时候能够及时通知到他们,然后能准确的、实时的处理客户的反馈。
2.解决方案
2.1 使用阿里的钉钉智能机器人在群里实时的将用户的反馈通知到特定的人或者全部的人。
2.2 查阅阿里钉钉的自能机器人,见:https://ding-doc.dingtalk.com/doc#/serverapi2/qf2nxq 。
2.3 先看下最终的一个效果图:
2.4 实时步骤:
2.4.1 创建一个钉钉群,在群里创建一个钉钉自动机人,后去机器人的webHook地址和secret
2.4.2 下载阿里的sdk
下载阿里的钉钉机器人的sdk的时候会有两个文件(见下图),有一个是jar包,另一个是源码文件。我们只需要使用一个就好了,即:taobao-sdk-java-auto_1479188381469-20200409.jar 。下载地址:https://ding-doc.dingtalk.com/doc#/faquestions/vzbp02
2.4.3 编码实现以及服务部署
我这边实现的话是用一个web接口把我们的官网和刚刚创建的钉钉智能机器人链接起来的。
2.4.3.1 web接口部分:
@RestController
@RequestMapping("/feedback")
public class TbCustomerFeedbackController {
//这里因为业务逻辑简单直接用Dao层来操作数据库了
@Autowired
private TbCustomerFeedbackDao customerFeedbackDao;
@RequestMapping(value = "/add", method = RequestMethod.POST)
@ResponseBody
public Result addCustomerFeedBack(final TbCustomerFeedback customerFeedback, HttpServletRequest request) throws NoSuchAlgorithmException, InvalidKeyException, UnsupportedEncodingException {
customerFeedback.setIp(HttpUtil.getRemortIP(request));
customerFeedback.setCreateTime(new Date());
int row = customerFeedbackDao.insert(customerFeedback);
//调用阿里的钉钉机器人的sdk发送消息到自己创建的机器人
ThreadPoolUtils.runTask(()->{
try {
DingTalkAutoRobotUtils.sendMsg(customerFeedback);
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
} catch (InvalidKeyException e) {
e.printStackTrace();
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
});
return row > 0 ? Result.success() : Result.fail("0", "反馈信息失败!");
}
}
2.4.3.2 钉钉机器人的代码部分(access_token和secret替换成自己创建的机器人的即可):
public class DingTalkAutoRobotUtils {
public static void sendMsg(TbCustomerFeedback customerFeedback) throws NoSuchAlgorithmException, InvalidKeyException, UnsupportedEncodingException {
String url = "https://oapi.dingtalk.com/robot/send?access_token=5695bfecf2302c9f3fe42db78f3378b7XXXXXXXXXXX655ad27638751×tamp=" + System.currentTimeMillis() + "&sign=" + getDingTalkaAutoRobotSing();
DingTalkClient client = new DefaultDingTalkClient(url);
OapiRobotSendRequest request = new OapiRobotSendRequest();
request.setMsgtype("text");
OapiRobotSendRequest.Text text = new OapiRobotSendRequest.Text();
StringBuilder msg = new StringBuilder();
msg.append("反馈主题:").append(customerFeedback.getTheme());
msg.append("\n");
msg.append("反馈人:").append(customerFeedback.getName());
msg.append("\n");
msg.append("联方式:").append(customerFeedback.getEmail());
msg.append("\n");
msg.append("反馈时间:").append(DateUtil.dateToDateStr(customerFeedback.getCreateTime()));
msg.append("\n");
msg.append("反馈内容:").append(customerFeedback.getContent());
msg.append("\n");
text.setContent(msg.toString());
request.setText(text);
OapiRobotSendRequest.At at = new OapiRobotSendRequest.At();
at.setIsAtAll("true");
request.setAt(at);
//下面是其他类型的消息,我只是用了text类型具体支持哪些参照:https://ding-doc.dingtalk.com/doc#/serverapi2/qf2nxq
// request.setMsgtype("link");
// OapiRobotSendRequest.Link link = new OapiRobotSendRequest.Link();
// link.setMessageUrl("https://www.dingtalk.com/");
// link.setPicUrl("");
// link.setTitle("时代的火车向前开");
// link.setText("这个即将发布的新版本,创始人xx称它为“红树林”。\n" +
// "而在此之前,每当面临重大升级,产品经理们都会取一个应景的代号,这一次,为什么是“红树林");
// request.setLink(link);
//
// request.setMsgtype("markdown");
// OapiRobotSendRequest.Markdown markdown = new OapiRobotSendRequest.Markdown();
// markdown.setTitle("杭州天气");
// markdown.setText("#### 杭州天气 @156xxxx8827\n" +
// "> 9度,西北风1级,空气良89,相对温度73%\n\n" +
// "> 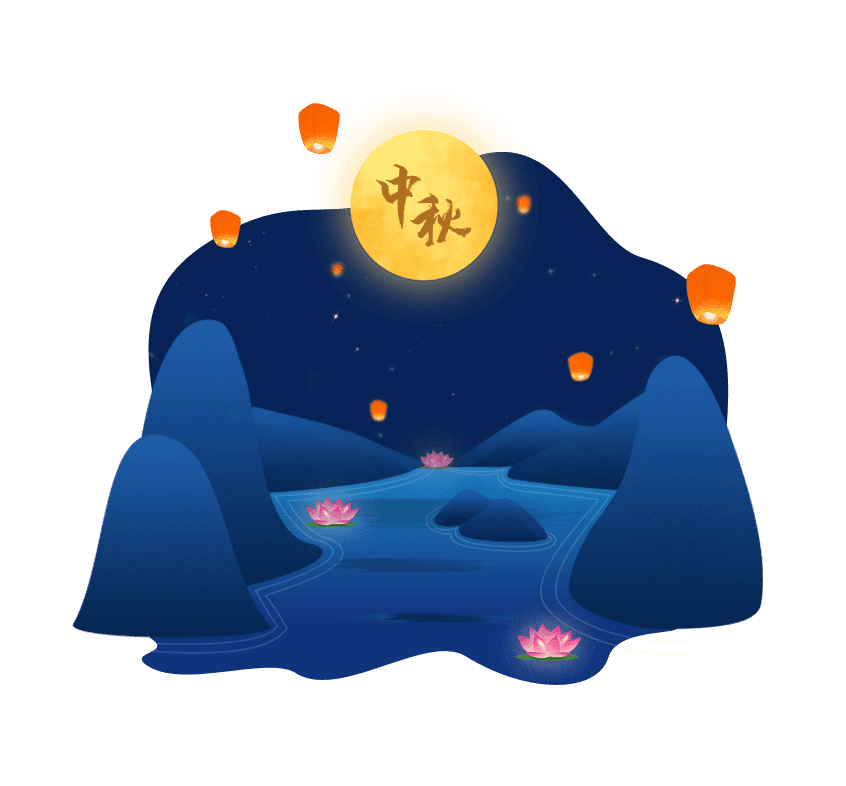\n" +
// "> ###### 10点20分发布 [天气](http://www.thinkpage.cn/) \n");
// request.setMarkdown(markdown);
try {
OapiRobotSendResponse response = client.execute(request);
} catch (ApiException e) {
e.printStackTrace();
}
}
private static String getDingTalkaAutoRobotSing() throws NoSuchAlgorithmException, UnsupportedEncodingException, InvalidKeyException {
Long timestamp = System.currentTimeMillis();
String secret = "SEC8c4bce59281ffbbc04XXXXXXXXXX43b92275477a01163f8ae";
String stringToSign = timestamp + "\n" + secret;
Mac mac = Mac.getInstance("HmacSHA256");
mac.init(new SecretKeySpec(secret.getBytes("UTF-8"), "HmacSHA256"));
byte[] signData = mac.doFinal(stringToSign.getBytes("UTF-8"));
String sign = URLEncoder.encode(new String(Base64.encodeBase64(signData)), "UTF-8");
return sign;
}
}