<?php
namespace base;
use app\service\UserService;
class Braintree
{
protected $environment;
protected $merchantId;
protected $publicKey;
protected $privateKey;
protected $gateway;
protected $merchantAccountId;
protected $merchantAccountArr = [
'AUD' => 'AUD',
'CAD' => 'CAD',
'EUR' => 'EUR',
'GBP' => 'GBP',
'USD' => 'USD',
];
public function __construct()
{
$this->environment = 'sandbox';
$this->merchantId = 'xxxx';
$this->publicKey = 'xxxx';
$this->privateKey = 'xxxxxx';
$this->gateway = new \Braintree\Gateway([
'environment' => $this->environment,
'merchantId' => $this->merchantId,
'publicKey' => $this->publicKey,
'privateKey' => $this->privateKey
]);
// $this->gateway->applePay()->registerDomain('xxx.xxxx.com');
}
public function getClientToken()
{
try {
// pass $clientToken to your front-end
$clientToken = $this->gateway->clientToken()->generate();
} catch (\Exception $e) {
return DataReturn(' Error:' . $e->getMessage(), -1);
}
return DataReturn('success', 0, $clientToken);
}
public function checkout($amount, $nonce, $deviceDataFromTheClient, $currency, $orderId, $threeDSecure = false)
{
if ($amount <= 0 || empty($nonce)) {
return DataReturn('Incorrect payment parameters', -1);
}
$this->setMerchantAccountId($currency);
try {
if ($threeDSecure) {
$result = $this->gateway->transaction()->sale([
'amount' => $amount,
'orderId' => $orderId,
'paymentMethodNonce' => $nonce,
'deviceData' => $deviceDataFromTheClient,
'merchantAccountId' => $this->merchantAccountId,
'options' => [
'submitForSettlement' => true,
'threeDSecure' => [
'required' => true
],
]
]);
} else {
$result = $this->gateway->transaction()->sale([
'amount' => $amount,
'orderId' => $orderId,
'paymentMethodNonce' => $nonce,
'deviceData' => $deviceDataFromTheClient,
'merchantAccountId' => $this->merchantAccountId,
'options' => [
'submitForSettlement' => true
]
]);
}
if ($result->success && !is_null($result->transaction)) {
// $transaction = $result->transaction;
return DataReturn('success', 0, $result);
} else {
$errorString = "";
foreach($result->errors->deepAll() as $error) {
$errorString .= 'Error: ' . $error->code . ": " . $error->message . "\n";
}
if (empty($errorString)) {
$errorString = $result->message;
}
return DataReturn($errorString, -1, $result);
}
} catch (\Exception $e) {
return DataReturn(' Error:' . $e->getMessage(), -1);
}
}
public function transaction($id)
{
$transaction = $this->gateway->transaction()->find($id);
$transactionSuccessStatuses = [
\Braintree\Transaction::AUTHORIZED,
\Braintree\Transaction::AUTHORIZING,
\Braintree\Transaction::SETTLED,
\Braintree\Transaction::SETTLING,
\Braintree\Transaction::SETTLEMENT_CONFIRMED,
\Braintree\Transaction::SETTLEMENT_PENDING,
\Braintree\Transaction::SUBMITTED_FOR_SETTLEMENT
];
if (in_array($transaction->status, $transactionSuccessStatuses)) {
$header = "Sweet Success!";
$icon = "success";
$message = "Your test transaction has been successfully processed. See the Braintree API response and try again.";
} else {
$header = "Transaction Failed";
$icon = "fail";
$message = "Your test transaction has a status of " . $transaction->status . ". See the Braintree API response and try again.";
}
return DataReturn($header, 0, $transaction);
}
public function refund($trade_no, $amount)
{
try {
$transaction = $this->gateway->transaction()->find($trade_no);
if($transaction->status == 'submitted_for_settlement'){
//如果交易尚未结算 暂不让退款
return DataReturn('braintree尚未结算,暂不能退款', -1);
}
$response = $this->gateway->transaction()->refund($trade_no, $amount);
if($response->success) {
return DataReturn('braintree_refund_success');
}else{
return DataReturn('braintree_refund_fail:'. $response->message, -1);
}
} catch (\Exception $e) {
return DataReturn(' Error:' . $e->getMessage(), -1);
}
}
public function setMerchantAccountId($currency)
{
$currency = strtoupper($currency);
if (!isset($this->merchantAccountArr[$currency])) {
throw new \Exception('Merchant account id error');
}
$this->merchantAccountId = $this->merchantAccountArr[$currency];
}
}
Braintree php支付(二)
于 2022-07-25 15:50:49 首次发布
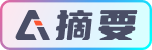