IP地址输入框
效果图
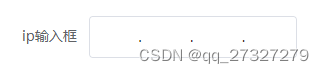
<template>
<div class="items rowCC" ref="itemRef">
<div class="item rowCC" v-for="(item,index) in ip" :key="index">
<el-input
v-model="item.value"
@input="onChange($event,index)"
@keyup="onControl($event,index)"
@keydown="onControl($event,index)"
@keypress.prevent.enter="initAddress"
:ref="(el)=>setItemRef(el, 'ipRef'+index)"
/>
<div class="point" v-if="!(index === ip.length - 1)">.</div>
</div>
</div>
</template>
<script lang="ts" setup>
let ip = ref([
{value: ''},
{value: ''},
{value: ''},
{value: ''},
])
let ipAddress = ref('')
let refs = ref({})
const setItemRef = (el, key) => {
refs.value[key] = el
}
let props = defineProps(["value"])
const emits = defineEmits(['update:value'])
const initAddress = () => {
ipAddress.value = ip.value[0].value + '.' + ip.value[1].value + '.' + ip.value[2].value + '.' + ip.value[3].value
}
const onControl = (e, index) => {
switch (e.code) {
case "Backspace":
if (ip.value[index].value === '') {
initAddress()
if (index === 0) {
return
}
nextTick(() => {
refs.value['ipRef' + (index - 1)].focus()
})
}
break
case "ArrowLeft":
if (index === 0) return
if (e.srcElement.selectionStart === 0) {
nextTick(() => {
refs.value['ipRef' + (index - 1)].focus()
})
}
break
case "ArrowRight":
if (index === 3) {
return
}
if (e.srcElement.selectionStart === e.srcElement.value.length) {
nextTick(() => {
refs.value['ipRef' + (index + 1)].focus()
})
}
break
}
}
const onChange = (e: string, index: Number) => {
if (e[e.length - 1] === '.' || e[e.length - 1] === '。') {
e = e.substring(0, e.length - 1)
if (index === 3) {
ip.value[index].value = e
return
}
refs.value['ipRef' + (index + 1)].focus()
}
if (isNaN(parseInt(Number(e)) && Number(e) < 0)) {
ip.value[index].value = ''
return
}
if (Number(e) >= 255) {
ip.value[index].value = 255
nextTick(() => {
if (index + 1 === 4) {
initAddress()
return
}
refs.value['ipRef' + (index + 1)].focus()
})
return
} else if (Number(e) >= 100) {
ip.value[index].value = e
nextTick(() => {
if (index + 1 === 4) {
initAddress()
return
}
})
return
} else {
ip.value[index].value = e
}
initAddress()
emits('update:value', ipAddress.value)
}
</script>
<style scoped lang="scss">
.items {
border: 1px solid #dcdfe6;
border-radius: var(--el-input-border-radius, var(--el-border-radius-base));
.point {
user-select: none;
}
:deep(.el-input--large .el-input__wrapper) {
border: none !important;
box-shadow: none !important;
padding: 1px 10px !important;
}
:deep(.el-input__inner) {
width: 100%;
padding: 0 !important;
}
}
</style>
组件引用
import IpAddressInput from "@/components/ipaddressinput/index.vue";
<IpAddressInput v-model:value="Form.ipAddress"/>