需要的jar包
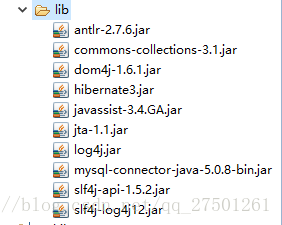
1、mysql的表employee(工具navicat for mysql)注意id是主键
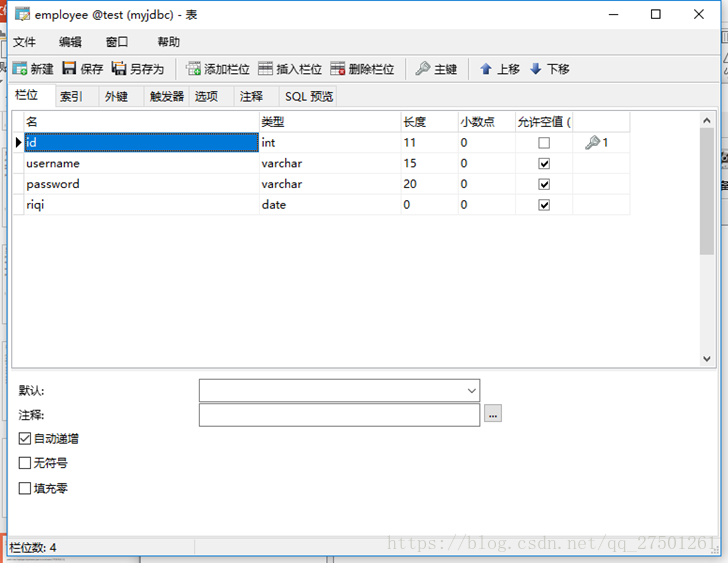
2、开发domain对象(类Employee继承接口java.io.Serializable )
package com.lw.test1;
import java.io.Serializable;
import java.util.Date;
public class Employee implements Serializable /*可序列化*/ {
private static final long serialVersionUID = 1L;
private Integer id;
private String username;
private String password;
private Date riqi;
public Date getRiqi() {
return riqi;
}
public void setRiqi(Date riqi) {
this.riqi = riqi;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
3、开发对象关系映射文件(配置文件employee.hbm.xml)employee.hbm.xml和Employee一般放在同一个文件夹下(包下)
<?xml version='1.0' encoding='utf-8'?>
<!-- 映射文件需要一个dtd来指定格式 -->
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
<!-- 该文件用户配置domain对象和表的映射关系 -->
<hibernate-mapping package="com.lw.test1">
<class name="com.lw.test1.Employee" table="employee">
<!-- id元素用于指定主键属性 -->
<id name="id" column="id" type="java.lang.Integer">
<!-- 该元素用于指定主键值生成策略hilo native increment sepuence uuid -->
<generator class="sequence">
<param name="sequence">emp_seq</param>
</generator>
</id>
<!-- 对其他属性还有配置 -->
<property name="username" type="java.lang.String">
<colum name="name" not-null="true"/>
</property>
<property name="password" type="java.lang.String">
<colum name="password" not-null="true"/>
</property>
<property name="riqi" type="java.util.Date">
<colum name="riqi" not-null="true"/>
</property>
</class>
</hibernate-mapping>
4、配置hibernate.cfg.xml文件(配置文件hibernate.cfg.xml)放在src包下即可
<?xml version="1.0" encoding="utf-8"?>
<!--标准的XML文件的起始行,version='1.0'表明XML的版本,encoding='utf-8'表明XML文件的编码方式-->
<!--表明解析本XML文件的DTD文档位置,DTD是Document Type Definition 的缩写,即文档类型的定义,XML解析器使用DTD文档来检查XML文件的合法性。
hibernate.sourceforge.net/hibernate-configuration-3.0dtd
可以在Hibernate3.1.3软件包中的src\org\hibernate目录中找到此文件-->
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<!-- hibernate 设计者,给我们提供一些常用的配置 -->
<!-- 配置使用的driver -->
<property name="connection.driver_class">com.mysql.jdbc.Driver</property>
<property name="connection.url">jdbc:mysql://127.0.0.1:3306/test</property>
<property name="connection.username">root</property>
<property name="connection.password">123456</property>
<!-- 配置dialect方言,明确告诉hibernate连接是哪种数据库 -->
<property name="dialect">org.hibernate.dialect.MySQLDialect</property>
<!-- 指定管理的对象映射文件 -->
<mapping resource="com/lw/test1/employee.hbm.xml"/>
</session-factory>
</hibernate-configuration>
5、测试类App1
package com.lw.test1;
import java.util.Date;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
import org.hibernate.cfg.Configuration;
public class App1 {
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
//我们使用hibernate完成CRUD操作!这里我们只见对象,不见表!
//现在我们不是用service,直接调试
//1、创建Configuration,该对象用于读取hibernate.cfg.xml,并完成初始化.
//configure()里面的参数默认放hibernate.cfg.xml,如果你的配置文件名不是这个,那个就写你的那个,底层是函数重载
Configuration configuration = new Configuration().configure();
//2、创建SessionFactory这是一个会话工厂,是一个重量级的对象
SessionFactory sessionFactory = configuration.buildSessionFactory();
//3、创建Session 相当于JDBC connection (不是servlet、HttpSession、jsp Session)
Session session = sessionFactory.openSession();
//4、对hibernate而言,要求程序员,在进行 增加、删除、修改的时候使用事务提交。
Transaction transaction = session.beginTransaction();
//添加一个用户
Employee employee = new Employee();
employee.setUsername("张三");
employee.setPassword("232323");
employee.setRiqi(new Date());
//保存
session.save(employee);
//提交事务
transaction.commit();
//关流
session.close();
}
}