文章目录
重温上节的路由。
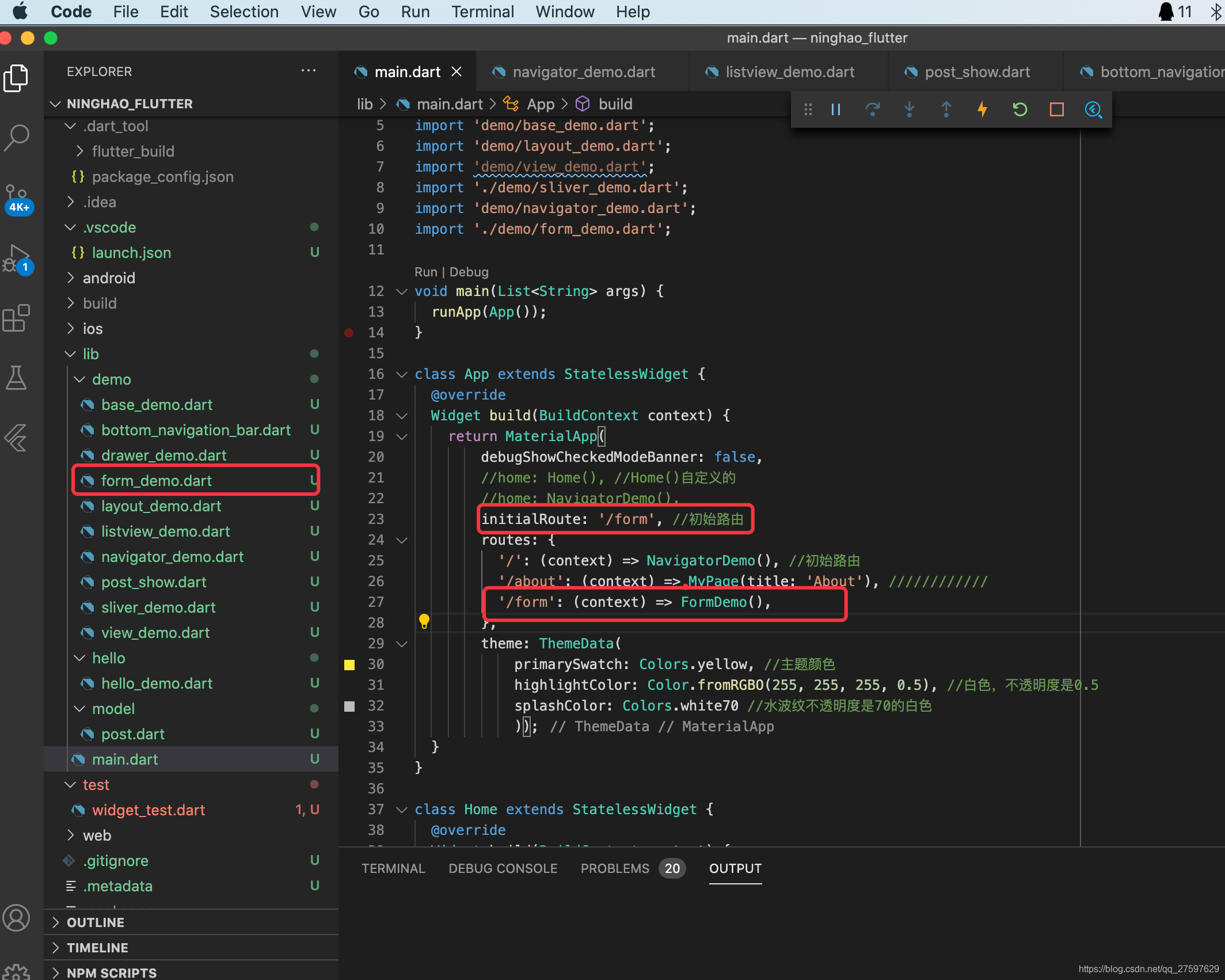
Theme:定制、使用、重置、覆盖主题
main.dart
class App extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
//home: Home(), //Home()自定义的
//home: NavigatorDemo(),
initialRoute: '/form', //初始路由
routes: {
'/': (context) => NavigatorDemo(), //初始路由
'/about': (context) => MyPage(title: 'About'),
'/form': (context) => FormDemo(),
},
theme: ThemeData(
primarySwatch: Colors.yellow, //主题颜色
highlightColor: Color.fromRGBO(255, 255, 255, 0.5), //白色,不透明度是0.5
splashColor: Colors.white70, //水波纹不透明度是70的白色
accentColor: Color.fromRGBO(3, 54, 255, 1.0),
));
}
}
form_demo.dart
import 'package:flutter/material.dart';
class FormDemo extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Theme(
data: Theme.of(context).copyWith(
//除了要替代的主题primaryColor,其他的主题会保留
//ThemeData(//专门又设置里ThemeData的颜色,会完全替代之前的主题
primaryColor: Colors.black,
),
child: ThemDemo(),
));
}
}
class ThemDemo extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Container(
color: Theme.of(context).accentColor,
/*Theme.of(context)
.primaryColor, //main.dart里设置了ThemeData的primarySwatch黄色,如果没有另外设置,这里会是黄色*/
);
}
}
TextField:文本字段
用StatefulWidget创建一个textFieldDemo类
import 'package:flutter/material.dart';
class FormDemo extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Theme(
data: Theme.of(context).copyWith(
//除了要替代的主题primaryColor,其他的主题会保留
//ThemeData(//专门又设置里ThemeData的颜色,会完全替代之前的主题
primaryColor: Colors.black,
),
child: Container(
padding: EdgeInsets.all(16.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
TextFieldDemo(),
],
),
)));
}
}
class ThemDemo extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Container(
color: Theme.of(context).accentColor,
/*Theme.of(context)
.primaryColor, //main.dart里设置了ThemeData的primarySwatch黄色,如果没有另外设置,这里会是黄色*/
);
}
}
class TextFieldDemo extends StatefulWidget {
TextFieldDemo({Key key}) : super(key: key);
@override
_TextFieldDemoState createState() => _TextFieldDemoState();
}
class _TextFieldDemoState extends State<TextFieldDemo> {
@override
Widget build(BuildContext context) {
return TextField();//默认样式的
}
}
6 TextField:文本字段样式(InputDecoration)
class _TextFieldDemoState extends State<TextFieldDemo> {
@override
Widget build(BuildContext context) {
return TextField(
decoration: InputDecoration(
icon: Icon(Icons.subject), //图标
labelText: 'Title', //题目
hintText: 'Enter the post title.', //提示文字
//border: InputBorder.none, //隐藏边框
//border: OutlineInputBorder(),//有边框
filled: true, //启用背景颜色边框,可用color属性设置颜色
fillColor: Colors.green,
),
);
//return TextField();//默认样式的
}
}
TextField:监视文本字段的值的变化与提交
class _TextFieldDemoState extends State<TextFieldDemo> {
@override
Widget build(BuildContext context) {
return TextField(
onChanged: (value) {//文本框内容改变时
debugPrint('input:$value');
},
onSubmitted: (value) {//按下确认按钮后
debugPrint('submit:$value');
},
decoration: InputDecoration(
icon: Icon(Icons.subject), //图标
labelText: 'Title', //题目
hintText: 'Enter the post title.', //提示文字
//border: InputBorder.none, //隐藏边框
//border: OutlineInputBorder(),//有边框
filled: true, //启用背景颜色边框,可用color属性设置颜色
fillColor: Colors.green,
),
);
//return TextField();//默认样式的
}
}
TextField:使用 TextEditingController 监听文本字段变化_
class TextFieldDemo extends StatefulWidget {
TextFieldDemo({Key key}) : super(key: key);
@override
_TextFieldDemoState createState() => _TextFieldDemoState();
}
class _TextFieldDemoState extends State<TextFieldDemo> {
final textEditingController = TextEditingController();//文本监听器
@override
void dispose() {
textEditingController.dispose(); //消化掉textEditingController,不然会浪费资源
super.dispose();
}
@override
void initState() {
//初始数据
// TODO: implement initState
super.initState();
textEditingController.text = 'hi';
textEditingController.addListener(() {
//监听文本框,变化时会输出
debugPrint('input:${textEditingController.text}');
});
}
@override
Widget build(BuildContext context) {
return TextField(
controller: textEditingController,
/*onChanged: (value) {
//文本框内容改变时
debugPrint('input:$value');
},*/ //用textEditingController的话需要注释掉onChanged
onSubmitted: (value) {
//按下确认按钮后
debugPrint('submit:$value');
},
decoration: InputDecoration(
icon: Icon(Icons.subject), //图标
labelText: 'Title', //题目
hintText: 'Enter the post title.', //提示文字
//border: InputBorder.none, //隐藏边框
//border: OutlineInputBorder(),//有边框
filled: true, //启用背景颜色边框,可用color属性设置颜色
fillColor: Colors.green,
),
);
//return TextField();//默认样式的
}
}
9 Form:表单
class RegisterForm extends StatefulWidget {
RegisterForm({Key key}) : super(key: key);
@override
_RegisterFormState createState() => _RegisterFormState();
}
class _RegisterFormState extends State<RegisterForm> {
@override
Widget build(BuildContext context) {
return Form(
child: Column(
children: [
TextFormField(
decoration: InputDecoration(
labelText: 'Username',
),
),
TextFormField(
obscureText: true, //密码比较敏感,启用这一项,输入的内容就不会直接显示出来
decoration: InputDecoration(
labelText: 'Password',
),
),
SizedBox(
height: 32.0,
), //用SizedBox添加点距离
Container(
width: double.infinity,
child: RaisedButton(
color: Theme.of(context).accentColor,
child: Text(
'Register',
style: TextStyle(color: Colors.white),
),
elevation: 0.0,
onPressed: () {}),
)
],
),
);
}
}
Form:保存与获取表单里的数据
save
class RegisterForm extends StatefulWidget {
RegisterForm({Key key}) : super(key: key);
@override
_RegisterFormState createState() => _RegisterFormState();
}
class _RegisterFormState extends State<RegisterForm> {
final registerFormKey = GlobalKey<FormState>();
String username, password;
void submitRegisterForm() {
if (registerFormKey.currentState.validate()) {
registerFormKey.currentState.save();
debugPrint('username: $username');
debugPrint('password: $password');
Scaffold.of(context).showSnackBar(SnackBar(
content: Text('Registering...'),
));
} else {}
}
@override
Widget build(BuildContext context) {
return Form(
key: registerFormKey,
child: Column(
children: [
TextFormField(
decoration: InputDecoration(
labelText: 'Username',
),
onSaved: (newValue) {
username = newValue;
},
),
TextFormField(
obscureText: true, //密码比较敏感,启用这一项,输入的内容就不会直接显示出来
decoration: InputDecoration(
labelText: 'Password',
),
onSaved: (newValue) {
password = newValue;
},
),
SizedBox(
height: 32.0,
), //用SizedBox添加点距离
Container(
width: double.infinity,
child: RaisedButton(
color: Theme.of(context).accentColor,
child: Text(
'Register',
style: TextStyle(color: Colors.white),
),
elevation: 0.0,
onPressed: submitRegisterForm,
))
],
),
);
}
}
11 Form:验证表单里的数据
validate
class RegisterForm extends StatefulWidget {
RegisterForm({Key key}) : super(key: key);
@override
_RegisterFormState createState() => _RegisterFormState();
}
class _RegisterFormState extends State<RegisterForm> {
final registerFormKey = GlobalKey<FormState>();
String username, password;
void submitRegisterForm() {
if (registerFormKey.currentState.validate()) {
registerFormKey.currentState.save();
debugPrint('username: $username');
debugPrint('password: $password');
Scaffold.of(context).showSnackBar(SnackBar(
content: Text('Registering...'),
));
} else {}
}
String validateUsername(value) {
if (value.isEmpty) {
return 'Username is required.';
} else
return null;
}
String validatePassword(value) {
if (value.isEmpty) {
return 'Password is required.';
} else
return null;
}
@override
Widget build(BuildContext context) {
return Form(
key: registerFormKey,
child: Column(
children: [
TextFormField(
decoration: InputDecoration(
labelText: 'Username',
//helperText: '', //表单验证的时候会自动出现helperText内容,设置成空,看起来正常点
),
onSaved: (newValue) {
username = newValue;
},
validator: validateUsername,
),
TextFormField(
obscureText: true, //密码比较敏感,启用这一项,输入的内容就不会直接显示出来
decoration: InputDecoration(
labelText: 'Password',
),
onSaved: (newValue) {
password = newValue;
},
validator: validatePassword,
),
SizedBox(
height: 32.0,
), //用SizedBox添加点距离
Container(
width: double.infinity,
child: RaisedButton(
color: Theme.of(context).accentColor,
child: Text(
'Register',
style: TextStyle(color: Colors.white),
),
elevation: 0.0,
onPressed: submitRegisterForm,
))
],
),
);
}
}
12 Form:自动验证
autovalidate: true,
可用用一个bool型属性来控制自动验证功能的开启,点击提交功能后开启
class RegisterForm extends StatefulWidget {
RegisterForm({Key key}) : super(key: key);
@override
_RegisterFormState createState() => _RegisterFormState();
}
class _RegisterFormState extends State<RegisterForm> {
final registerFormKey = GlobalKey<FormState>();
String username, password;
bool autovalidate = false;
void submitRegisterForm() {
if (registerFormKey.currentState.validate()) {
//如果验证成功,保存数据
registerFormKey.currentState.save();
debugPrint('username: $username');
debugPrint('password: $password');
Scaffold.of(context).showSnackBar(SnackBar(
content: Text('Registering...'),
));
} else {
setState(() {
autovalidate = true; //打开表单自动验证
});
}
}
String validateUsername(value) {
if (value.isEmpty) {
return 'Username is required.';
} else
return null;
}
String validatePassword(value) {
if (value.isEmpty) {
return 'Password is required.';
} else
return null;
}
@override
Widget build(BuildContext context) {
return Form(
key: registerFormKey,
child: Column(
children: [
TextFormField(
decoration: InputDecoration(
labelText: 'Username',
//helperText: '', //表单验证的时候会自动出现helperText内容,设置成空,看起来正常点
),
onSaved: (newValue) {
username = newValue;
},
validator: validateUsername,
autovalidate: autovalidate,
),
TextFormField(
obscureText: true, //密码比较敏感,启用这一项,输入的内容就不会直接显示出来
decoration: InputDecoration(
labelText: 'Password',
),
onSaved: (newValue) {
password = newValue;
},
validator: validatePassword,
autovalidate: autovalidate,
),
SizedBox(
height: 32.0,
), //用SizedBox添加点距离
Container(
width: double.infinity,
child: RaisedButton(
color: Theme.of(context).accentColor,
child: Text(
'Register',
style: TextStyle(color: Colors.white),
),
elevation: 0.0,
onPressed: submitRegisterForm,
))
],
),
);
}
}