srping底层原理
文章目录
1. 概述
spring有ioc和aop两大特性,是常用的开发框架
2. spring的组件
2.2 InitializingBean
源码 :
public interface InitializingBean {
// 初始化方法
void afterPropertiesSet() throws Exception;
}
----------------------------------------下面的bean实例化的源码-------------------------------------------------
protected void invokeInitMethods(String beanName, Object bean, @Nullable RootBeanDefinition mbd)
throws Throwable {
// 判断实例后的bean 是否实现了InitializingBean接口
boolean isInitializingBean = (bean instanceof InitializingBean);
if (isInitializingBean && (mbd == null || !mbd.isExternallyManagedInitMethod("afterPropertiesSet"))) {
if (logger.isTraceEnabled()) {
logger.trace("Invoking afterPropertiesSet() on bean with name '" + beanName + "'");
}
if (System.getSecurityManager() != null) {
try {
AccessController.doPrivileged((PrivilegedExceptionAction<Object>) () -> {
// 如果实现了 直接强转 调用afterPropertiesSet方法
((InitializingBean) bean).afterPropertiesSet();
return null;
}, getAccessControlContext());
}
catch (PrivilegedActionException pae) {
throw pae.getException();
}
}
else {
// 如果实现了 直接强转 调用afterPropertiesSet方法
((InitializingBean) bean).afterPropertiesSet();
}
}
...
}
原理 : 如果这个bean实现了InitializingBean接口,那么会在这个bean初始化的时候都会执行该afterPropertiesSet方法。
2.3 BeanPostProcessor
原理 : 这个接口里面提供了两个接口,一个初始化前一个初始化后,只要有一个bean实现了这个接口,其他所有的bean在初始化的时候调用这两个方法,并且aop就是基于这个接口实现的。
2.4 BeanDefinition
源码 :
public interface BeanDefinition extends AttributeAccessor, BeanMetadataElement {
@Nullable
String getParentName();
@Nullable
String getBeanClassName();
@Nullable
String getScope();
// 是否懒加载
boolean isLazyInit();
@Nullable
String[] getDependsOn();
boolean isAutowireCandidate();
boolean isPrimary();
@Nullable
String getFactoryBeanName();
···
-----------------------------------------------下面是合并的逻辑----------------------------------------------
for (String beanName : beanNames) {
// 这里在创建bean之前 会先把BeanDefinition合并,然后根据合并之后的RootBeanDefinition来创建bean
,
RootBeanDefinition bd = getMergedLocalBeanDefinition(beanName);
// 只有单例 和非懒加载的bean 才会在容器初始化的时候创建,然后这个bean还要是非接口的
//(这里的接口指的是bean的属性,不是java的接口)
if (!bd.isAbstract() && bd.isSingleton() && !bd.isLazyInit()) {
···
else {
// 这里就是创建 或者获取bean
getBean(beanName);
}
···
/* getMergedLocalBeanDefinition最终会进入到这两行方法 */
// 根据父类 创建 一个新的 RootBeanDefinition
mbd = new RootBeanDefinition(pbd);
// 然后覆盖父类没有 或者子类已经用的属性 (合并的逻辑 不止父子bean属性合并)
mbd.overrideFrom(bd);
原理 : BeanDefinition表示Bean定义,里面定义了很对对于bean的属性。如class属性,是否懒加载,是否单例等。然后根据这些属性来创建bean。
还有对不同种类的BeanDefinition合并,最终创建bean的是通过合并后的RootBeanDefinition来创建的。( 合并是指我这个bean某个属性,没有指定,那么我会先从父类bean去找,没有找到的情况下再使用默认值; 如是否懒加载,默认情况下为false,但如果父类bean是true的话,那这个bean创建的时候懒加载的属性也要为true,所以就会有 BeanDefinition合并的逻辑)
2.5 FactoryBean
源码 :
// 原对象(原本的bean)创建
for (String beanName : beanNames) {
RootBeanDefinition bd = getMergedLocalBeanDefinition(beanName);
if (!bd.isAbstract() && bd.isSingleton() && !bd.isLazyInit()) {
// 如果这个bean实现了FactoryBean接口
if (isFactoryBean(beanName)) {
// 创建/获取 bean (注意 这里获取的是原对象, 传参是&+beanName)
// 带‘&’符号 表示从单例池获取原对象 不带 则直接返回 getObject对象
// FACTORY_BEAN_PREFIX 这个常量其实就是字符串 ‘&’符号
Object bean = getBean(FACTORY_BEAN_PREFIX + beanName);
if (bean instanceof FactoryBean) {
FactoryBean<?> factory = (FactoryBean<?>) bean;
boolean isEagerInit;
if (System.getSecurityManager() != null && factory instanceof SmartFactoryBean) {
isEagerInit = AccessController.doPrivileged(
(PrivilegedAction<Boolean>) ((SmartFactoryBean<?>)factory)::isEagerInit,
getAccessControlContext());
}
else {
isEagerInit = (factory instanceof SmartFactoryBean &&
((SmartFactoryBean<?>) factory).isEagerInit());
}
// 如果isEagerInit == true 那么在容器初始化的时候 就实例化 getObject对象 否则就在getBean的时候创建
if (isEagerInit) {
getBean(beanName);
}
}
}
else {
getBean(beanName);
}
}
}
···
------------------------------------------下面看看getObject对象是怎么创建的-------------------------------------
/* 1.传进来的name 有没有带&符号 没有则继续往2和3步骤走 */
protected Object getObjectForBeanInstance(
Object beanInstance, String name, String beanName, @Nullable RootBeanDefinition mbd) {
// Don't let calling code try to dereference the factory if the bean isn't a factory.
// 判断getbean 传进来的name 有没有带&符号 如果有 直接返回原对象beanInstance(从单例池获取的),没有继续往下走
if (BeanFactoryUtils.isFactoryDereference(name)) {
if (beanInstance instanceof NullBean) {
return beanInstance;
}
// 不是FactoryBean不会进到这个方法 直接抛异常
if (!(beanInstance instanceof FactoryBean)) {
throw new BeanIsNotAFactoryException(beanName, beanInstance.getClass());
}
if (mbd != null) {
mbd.isFactoryBean = true;
}
// 直接返回原对象
return beanInstance;
}
···
/* 2. 如果没有返回原对象 就会进入到getObjectFromFactoryBean方法*/
protected Object getObjectFromFactoryBean(FactoryBean<?> factory, String beanName, boolean shouldPostProcess) {
if (factory.isSingleton() && containsSingleton(beanName)) {
synchronized (getSingletonMutex()) {
// 先从map缓存获取
Object object = this.factoryBeanObjectCache.get(beanName);
if (object == null) {
// 不存在就从getObject获取
object = doGetObjectFromFactoryBean(factory, beanName);
// 下面的方法最终返回object 也就是getObject里面的对象
...
/* 3. 不存在就从getObject获取(doGetObjectFromFactoryBean方法的源码) */
private Object doGetObjectFromFactoryBean(FactoryBean<?> factory, String beanName) throws BeanCreationException {
Object object;
try {
if (System.getSecurityManager() != null) {
AccessControlContext acc = getAccessControlContext();
try {
// 直接回返getObject的对象
object = AccessController.doPrivileged((PrivilegedExceptionAction<Object>) factory::getObject, acc);
}
catch (PrivilegedActionException pae) {
throw pae.getException();
}
}
else {
// 直接回返getObject的对象
object = factory.getObject();
}
}
...
原理 : FactoryBean是一个比较特殊的bean,实现里面的getObject方法,getBean返回的就不是原对象,而是getObject里面的对象
但其实原对象是存在的,而且会放在单例池中,只不过在getbean的时候,spring会判断如果实现了FactoryBean接口,就返回getObject接口里的对象,如果想要获取原对象,可以使用getBean(&+beanName)的方式
2.6 MetadataReader
采用元数据的方式,获取这个class文件的注解,方法等信息,达到跟反射一样的效果;跟反射的区别是元数据是基于文件获取注解,更适合ioc容器初始化扫描文件,而反射需要把class文件加载到jvm中。
2.7 ApplicationContext
bean工厂的子类,除了拥有getbean的功能,还有其他国际化、aop等功能。
3.原理解析
3.1 spring原理之扫描
3.1.1 源码
- spring启动容器的时候会调scan方法
public void scan(String... basePackages) {
Assert.notEmpty(basePackages, "At least one base package must be specified");
StartupStep scanPackages = this.getApplicationStartup().start("spring.context.base-packages.scan")
.tag("packages", () -> Arrays.toString(basePackages));
this.scanner.scan(basePackages);
scanPackages.end();
}
- 然后进入到doScan方法,通过元数据的方式把class类传成beanDefinition,(元数据MetadataReader和beanDefinition上面有讲)在通过元数据把注解上面的值,比如是否懒加载之类的,set到beanDefinition中,最后把这些beanDefinition注册到beanDefinitionMap中
/* scanner.scan(basePackages)的源码 */
public int scan(String... basePackages) {
int beanCountAtScanStart = this.registry.getBeanDefinitionCount();
/* 最终进入到 doScan方法 */
doScan(basePackages);
// Register annotation config processors, if necessary.
if (this.includeAnnotationConfig) {
AnnotationConfigUtils.registerAnnotationConfigProcessors(this.registry);
}
// 这里是返回了这次扫描获取BeanDefinition的数量 (这个方法不是重点)
return (this.registry.getBeanDefinitionCount() - beanCountAtScanStart);
}
------------------------------------------最终进入到 doScan方法----------------------------------
protected Set<BeanDefinitionHolder> doScan(String... basePackages) {
Assert.notEmpty(basePackages, "At least one base package must be specified");
Set<BeanDefinitionHolder> beanDefinitions = new LinkedHashSet<>();
for (String basePackage : basePackages) {
// 通过包路径 或者 配置文件的方式 拿到class文件 转成 BeanDefinition对象
Set<BeanDefinition> candidates = findCandidateComponents(basePackage);
for (BeanDefinition candidate : candidates) {
// 通过元数据的方式 获取 Scope注解
ScopeMetadata scopeMetadata = this.scopeMetadataResolver.resolveScopeMetadata(candidate);
// 把Scope注解的值 set到 BeanDefinition的属性中
candidate.setScope(scopeMetadata.getScopeName());
// 获取bean的名字 如果有指定名字 就用指定的 否则 默认class类名首字母小写
String beanName = this.beanNameGenerator.generateBeanName(candidate, this.registry);
if (candidate instanceof AbstractBeanDefinition) {
// 设置 BeanDefinition的一些默认值 如 默认是单例 默认非懒加载
postProcessBeanDefinition((AbstractBeanDefinition) candidate, beanName);
}
if (candidate instanceof AnnotatedBeanDefinition) {
// 把这个bean的注解上面的值 全部 set到 BeanDefinition中 如 @Lazy @Primary @Description 等注解
AnnotationConfigUtils.processCommonDefinitionAnnotations((AnnotatedBeanDefinition) candidate);
}
// 校验这个bean 是否已存在,存在就抛异常 || 不放在beanDefinitionMap中 也就是返回false
if (checkCandidate(beanName, candidate)) {
BeanDefinitionHolder definitionHolder = new BeanDefinitionHolder(candidate, beanName);
definitionHolder =
AnnotationConfigUtils.applyScopedProxyMode(scopeMetadata, definitionHolder, this.registry);
beanDefinitions.add(definitionHolder);
// 最后把这个beanDefinitions注册到beanDefinitionMap中
//里面最终会进入到这行代码 this.beanDefinitionMap.put(beanName, beanDefinition);
registerBeanDefinition(definitionHolder, this.registry);
}
}
}
return beanDefinitions;
}
- findCandidateComponents方法解析
public Set<BeanDefinition> findCandidateComponents(String basePackage) {
if (this.componentsIndex != null && indexSupportsIncludeFilters()) {
// 通过spring.components文件 读取需要扫描的class文件 不常用
return addCandidateComponentsFromIndex(this.componentsIndex, basePackage);
}
else {
// 直接扫描配置路径下的所有 class 文件 主要看这个
return scanCandidateComponents(basePackage);
}
}
----------------------------------scanCandidateComponents方法源码-------------------------------
private Set<BeanDefinition> scanCandidateComponents(String basePackage) {
Set<BeanDefinition> candidates = new LinkedHashSet<>();
try {
// 获取到完整的路径
String packageSearchPath = ResourcePatternResolver.CLASSPATH_ALL_URL_PREFIX +
resolveBasePackage(basePackage) + '/' + this.resourcePattern;
// 拿到这个路径下的所有 class文件
Resource[] resources = getResourcePatternResolver().getResources(packageSearchPath);
boolean traceEnabled = logger.isTraceEnabled();
boolean debugEnabled = logger.isDebugEnabled();
for (Resource resource : resources) {
if (traceEnabled) {
logger.trace("Scanning " + resource);
}
try {
// 通过元数据工厂把class文件 转成元数据
MetadataReader metadataReader = getMetadataReaderFactory().getMetadataReader(resource);
// 这个方法里面判断这个class 没有被excludeFilters包含 && 被includeFilters包含,被包含表示是bean
// includeFilters里面初始的时候就有一个默认的@Component注解 所以 被Component注释的都是bean
if (isCandidateComponent(metadataReader)) {
// 元数据转成BeanDefinition
ScannedGenericBeanDefinition sbd = new ScannedGenericBeanDefinition(metadataReader);
// 设置 setSource
sbd.setSource(resource);
// 这里面判断 (是否接口同时被Lookup注解标记 或者 不是接口和抽象类) && (不是内部类)
// 因为内部类会单独的创建一个class 文件,所以需要排除掉这种class文件
// 然后抽象类 是不能为bean的,接口如果没有被Lookup注解标记 也不能为bean
if (isCandidateComponent(sbd)) {
if (debugEnabled) {
logger.debug("Identified candidate component class: " + resource);
}
// 校验通过就放到list中
candidates.add(sbd);
}
else {
if (debugEnabled) {
logger.debug("Ignored because not a concrete top-level class: " + resource);
}
}
}
else {
if (traceEnabled) {
logger.trace("Ignored because not matching any filter: " + resource);
}
}
}
catch (FileNotFoundException ex) {
if (traceEnabled) {
logger.trace("Ignored non-readable " + resource + ": " + ex.getMessage());
}
}
catch (Throwable ex) {
throw new BeanDefinitionStoreException(
"Failed to read candidate component class: " + resource, ex);
}
}
}
catch (IOException ex) {
throw new BeanDefinitionStoreException("I/O failure during classpath scanning", ex);
}
return candidates;
}
3.1.2 流程图
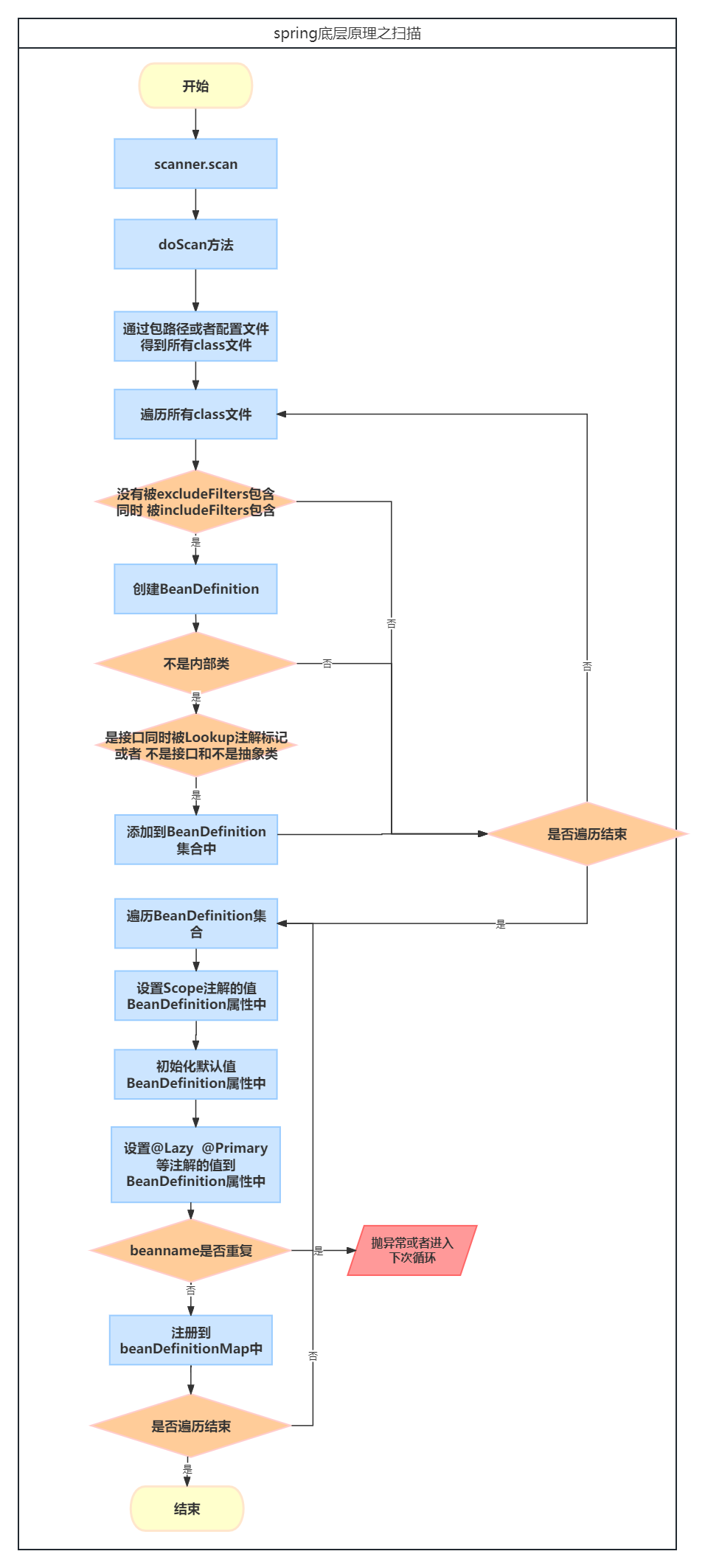
3.1.3 总结
spring启动容器的时候会调scan方法,然后通过包路径或者配置文件扫描拿到class文件,然后通过元数据的方式获取上面的注解,判断这个class是否是一个bean,有没有被Component注解标识,如果是就创建BeanDefinition对象,里面有一些对bean的定义,如单例还是多例,这个bean的class对象是谁,并通过注解对BeanDefinition属性进行赋值,初始化一些默认值,如果这个beanname不重复,就放在BeanDefinitionMap中。
3.2 spring原理之获取或创建Bean(Bean的生命周期)
3.2.1 BeanPostProcessor子类
-
InstantiationAwareBeanPostProcessor
提供实例化前和实例化后的方法,还有postProcessProperties方法,在属性注入的时候会调用。
Autowired注解和@Value注解,Resources注解都是通过这个接口实现的,在实例化后方法获取哪些属性需要注入,放在一个map中,在postProcessProperties方法中通过这个map来进行属性注入。
-
SmartInstantiationAwareBeanPostProcessor
-
DestructionAwareBeanPostProcessor
-
MergedBeanDefinitionPostProcessor
提供了postProcessMergedBeanDefinition方法,在这个bean实例化之后调用的,可以对这个bean的RootBeanDefinition做修改操作。
3.2.1 spring原理获取Bean(1)
3.2.1.1 源码
- 如果存在bean直接返回
// getBean 方法具体实现逻辑在doGetBean
protected <T> T doGetBean(
String name, @Nullable Class<T> requiredType, @Nullable Object[] args, boolean typeCheckOnly)
throws BeansException {
// 获取bean的名称
// 如果以& 就会去去掉&符号 FactoryBean中的实现
String beanName = transformedBeanName(name);
Object beanInstance;
// Eagerly check singleton cache for manually registered singletons.
// 从单例池 或者 二级 三级缓存获取
Object sharedInstance = getSingleton(beanName);
if (sharedInstance != null && args == null) {
if (logger.isTraceEnabled()) {
if (isSingletonCurrentlyInCreation(beanName)) {
logger.trace("Returning eagerly cached instance of singleton bean '" + beanName +
"' that is not fully initialized yet - a consequence of a circular reference");
}
else {
logger.trace("Returning cached instance of singleton bean '" + beanName + "'");
}
}
// 存在直接返回bean对象
// 如果这个bean继承了FactoryBean, 参数name带&符号就返回单例池的源对象,不带则返回getObject的对象 FactoryBean中的实现
beanInstance = getObjectForBeanInstance(sharedInstance, name, beanName, null);
}
else {
... 不存在的逻辑放第二点
}
- 如果不存在,调用createBean方法创建bean
// Fail if we're already creating this bean instance:
// We're assumably within a circular reference.
if (isPrototypeCurrentlyInCreation(beanName)) {
throw new BeanCurrentlyInCreationException(beanName);
}
// Check if bean definition exists in this factory.
// 拿父类的BeanFactory
// 如果父类BeanFactory存在且当前容器不包含该beanName 就用父类的bean工厂创建或获取
BeanFactory parentBeanFactory = getParentBeanFactory();
if (parentBeanFactory != null && !containsBeanDefinition(beanName)) {
// Not found -> check parent.
String nameToLookup = originalBeanName(name);
if (parentBeanFactory instanceof AbstractBeanFactory) {
return ((AbstractBeanFactory) parentBeanFactory).doGetBean(
nameToLookup, requiredType, args, typeCheckOnly);
}
else if (args != null) {
// Delegation to parent with explicit args.
return (T) parentBeanFactory.getBean(nameToLookup, args);
}
else if (requiredType != null) {
// No args -> delegate to standard getBean method.
return parentBeanFactory.getBean(nameToLookup, requiredType);
}
else {
return (T) parentBeanFactory.getBean(nameToLookup);
}
}
// 标记这个bean已经创建或者正要创建
if (!typeCheckOnly) {
markBeanAsCreated(beanName);
}
StartupStep beanCreation = this.applicationStartup.start("spring.beans.instantiate")
.tag("beanName", name);
try {
if (requiredType != null) {
beanCreation.tag("beanType", requiredType::toString);
}
// 拿合并后的BeanDefinition 来创建bean
RootBeanDefinition mbd = getMergedLocalBeanDefinition(beanName);
// 如果这个bean的定义是抽象类 直接抛异常
checkMergedBeanDefinition(mbd, beanName, args);
// Guarantee initialization of beans that the current bean depends on.
String[] dependsOn = mbd.getDependsOn();
if (dependsOn != null) {
for (String dep : dependsOn) {
if (isDependent(beanName, dep)) {
throw new BeanCreationException(mbd.getResourceDescription(), beanName,
"Circular depends-on relationship between '" + beanName + "' and '" + dep + "'");
}
registerDependentBean(dep, beanName);
try {
getBean(dep);
}
catch (NoSuchBeanDefinitionException ex) {
throw new BeanCreationException(mbd.getResourceDescription(), beanName,
"'" + beanName + "' depends on missing bean '" + dep + "'", ex);
}
}
}
// Create bean instance.
// 创建单例bean
if (mbd.isSingleton()) {
// 如果单例池没有,就执行createBean方法
sharedInstance = getSingleton(beanName, () -> {
try {
return createBean(beanName, mbd, args);
}
catch (BeansException ex) {
// Explicitly remove instance from singleton cache: It might have been put there
// eagerly by the creation process, to allow for circular reference resolution.
// Also remove any beans that received a temporary reference to the bean.
destroySingleton(beanName);
throw ex;
}
});
// 同上 一样的逻辑
beanInstance = getObjectForBeanInstance(sharedInstance, name, beanName, mbd);
}
// 创建多例bean
else if (mbd.isPrototype()) {
// It's a prototype -> create a new instance.
Object prototypeInstance = null;
try {
beforePrototypeCreation(beanName);
prototypeInstance = createBean(beanName, mbd, args);
}
finally {
afterPrototypeCreation(beanName);
}
// 同上 一样的逻辑
beanInstance = getObjectForBeanInstance(prototypeInstance, name, beanName, mbd);
}
else {
// 创建作用域是请求或者会话的bean
String scopeName = mbd.getScope();
if (!StringUtils.hasLength(scopeName)) {
throw new IllegalStateException("No scope name defined for bean '" + beanName + "'");
}
Scope scope = this.scopes.get(scopeName);
if (scope == null) {
throw new IllegalStateException("No Scope registered for scope name '" + scopeName + "'");
}
try {
Object scopedInstance = scope.get(beanName, () -> {
beforePrototypeCreation(beanName);
try {
return createBean(beanName, mbd, args);
}
finally {
afterPrototypeCreation(beanName);
}
});
// 存在直接返回bean对象
// 如果这个bean继承了FactoryBean, 参数name带&符号就返回单例池的源对象,不带则返回getObject的对象 FactoryBean中的实现
beanInstance = getObjectForBeanInstance(scopedInstance, name, beanName, mbd);
}
catch (IllegalStateException ex) {
throw new ScopeNotActiveException(beanName, scopeName, ex);
}
}
}
catch (BeansException ex) {
beanCreation.tag("exception", ex.getClass().toString());
beanCreation.tag("message", String.valueOf(ex.getMessage()));
cleanupAfterBeanCreationFailure(beanName);
throw ex;
}
finally {
beanCreation.end();
}
}
return adaptBeanInstance(name, beanInstance, requiredType);
3.2.1.2 流程图
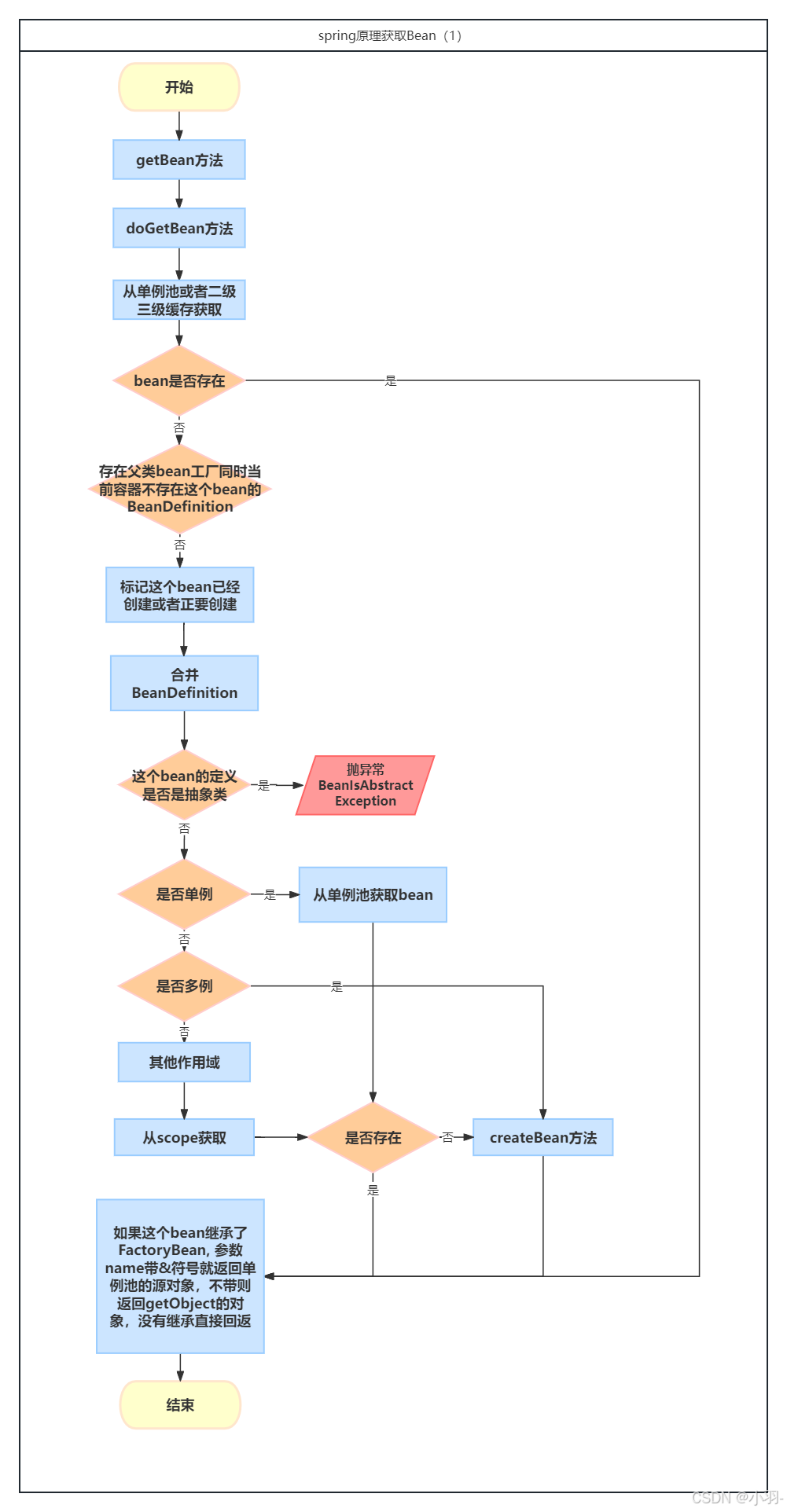
3.2.1.3 总结
先从单例池和缓存中获取,如果不存在就创建或者从父类工厂获取,创建之前打上正在创建的标记,还有合并BeanDefinition,根据不同的作用域创建,然后放到不同的缓存里面,最终调用创建bean是调用的createBean方法。
3.2.2 spring原理创建Bean(2)
3.2.2.1 源码
1.首先先调createBean方法
/************************************createBean源码*********************************************************/
@Override
protected Object createBean(String beanName, RootBeanDefinition mbd, @Nullable Object[] args)
throws BeanCreationException {
... 删掉日志代码太长
// 通过类加载器加载class(默认使用的app类加载器,可以设置指定的类加载器)
Class<?> resolvedClass = resolveBeanClass(mbd, beanName);
if (resolvedClass != null && !mbd.hasBeanClass() && mbd.getBeanClassName() != null) {
mbdToUse = new RootBeanDefinition(mbd);
// 把class对象设置倒BeanDefinition属性中
mbdToUse.setBeanClass(resolvedClass);
}
// Prepare method overrides.
try {
mbdToUse.prepareMethodOverrides();
}
catch (BeanDefinitionValidationException ex) {
throw new BeanDefinitionStoreException(mbdToUse.getResourceDescription(),
beanName, "Validation of method overrides failed", ex);
}
try {
// 调用InstantiationAwareBeanPostProcessor的实例化前方法,如果不为空就直接返回
// 具体逻辑在下面
Object bean = resolveBeforeInstantiation(beanName, mbdToUse);
// 实例化前方法有回返的对象,spring不走正常的实例化逻辑,直接返回这个对象为bean。
if (bean != null) {
return bean;
}
}
catch (Throwable ex) {
throw new BeanCreationException(mbdToUse.getResourceDescription(), beanName,
"BeanPostProcessor before instantiation of bean failed", ex);
}
try {
// 具体创建bean的方法
// 具体看这个方法
Object beanInstance = doCreateBean(beanName, mbdToUse, args);
if (logger.isTraceEnabled()) {
logger.trace("Finished creating instance of bean '" + beanName + "'");
}
return beanInstance;
}
catch (BeanCreationException | ImplicitlyAppearedSingletonException ex) {
// A previously detected exception with proper bean creation context already,
// or illegal singleton state to be communicated up to DefaultSingletonBeanRegistry.
throw ex;
}
catch (Throwable ex) {
throw new BeanCreationException(
mbdToUse.getResourceDescription(), beanName, "Unexpected exception during bean creation", ex);
}
}
/*************************resolveBeforeInstantiation源码(实例化前的方法逻辑)******************************/
@Nullable
protected Object resolveBeforeInstantiation(String beanName, RootBeanDefinition mbd) {
Object bean = null;
if (!Boolean.FALSE.equals(mbd.beforeInstantiationResolved)) {
if (!mbd.isSynthetic() && hasInstantiationAwareBeanPostProcessors()) {
Class<?> targetType = determineTargetType(beanName, mbd);
if (targetType != null) {
// 遍历InstantiationAwareBeanPostProcessor 调用实例化前方法 返回对象
bean = applyBeanPostProcessorsBeforeInstantiation(targetType, beanName);
if (bean != null) {
// 不为空调用 BeanPostProcessors的初始化前方法 (这种方式生成的bean 也是要调初始化前方法的)
bean = applyBeanPostProcessorsAfterInitialization(bean, beanName);
}
}
}
mbd.beforeInstantiationResolved = (bean != null);
}
// bean为null 就走正常的流程,实例化 依赖注入等流程,不为null,直接返回这个对象为bean
return bean;
}
// applyBeanPostProcessorsBeforeInstantiation 源码
protected Object applyBeanPostProcessorsBeforeInstantiation(Class<?> beanClass, String beanName) {
// 从getBeanPostProcessorCache缓存中拿到 遍历InstantiationAwareBeanPostProcessor的list遍历
for (InstantiationAwareBeanPostProcessor bp : getBeanPostProcessorCache().instantiationAware) {
// 调用实例化前方法,只要有一个返回的对象不为null,就中断遍历。
Object result = bp.postProcessBeforeInstantiation(beanClass, beanName);
if (result != null) {
return result;
}
}
return null;
}
- 进入到doCreateBean方法
/************************************doCreateBean源码************************************/
protected Object doCreateBean(String beanName, RootBeanDefinition mbd, @Nullable Object[] args)
throws BeanCreationException {
// Instantiate the bean.
BeanWrapper instanceWrapper = null;
if (mbd.isSingleton()) {
instanceWrapper = this.factoryBeanInstanceCache.remove(beanName);
}
if (instanceWrapper == null) {
// 对bean实例化 推断构造方法等逻辑(具体3.2.4讲)
instanceWrapper = createBeanInstance(beanName, mbd, args);
}
Object bean = instanceWrapper.getWrappedInstance();
Class<?> beanType = instanceWrapper.getWrappedClass();
if (beanType != NullBean.class) {
mbd.resolvedTargetType = beanType;
}
// Allow post-processors to modify the merged bean definition.
synchronized (mbd.postProcessingLock) {
if (!mbd.postProcessed) {
try {
// 遍历MergedBeanDefinitionPostProcessor 对BeanDefinition进行赋值操作
applyMergedBeanDefinitionPostProcessors(mbd, beanType, beanName);
}
catch (Throwable ex) {
throw new BeanCreationException(mbd.getResourceDescription(), beanName,
"Post-processing of merged bean definition failed", ex);
}
mbd.postProcessed = true;
}
}
··· 解决循环依赖代码太长先删掉
// Initialize the bean instance.
Object exposedObject = bean;
try {
// 依赖注入(具体3.2.5讲)
// 在依赖注入之前会调用实例化后的方法,因为依赖注入就是基于InstantiationAwareBeanPostProcessor实现的
populateBean(beanName, mbd, instanceWrapper);
// 具体看这个方法 初始化
exposedObject = initializeBean(beanName, exposedObject, mbd);
}
catch (Throwable ex) {
if (ex instanceof BeanCreationException && beanName.equals(((BeanCreationException) ex).getBeanName())) {
throw (BeanCreationException) ex;
}
else {
throw new BeanCreationException(
mbd.getResourceDescription(), beanName, "Initialization of bean failed", ex);
}
}
··· 解决循环依赖代码太长先删掉
// Register bean as disposable.
try {
// 注册bean
registerDisposableBeanIfNecessary(beanName, bean, mbd);
}
catch (BeanDefinitionValidationException ex) {
throw new BeanCreationException(
mbd.getResourceDescription(), beanName, "Invalid destruction signature", ex);
}
return exposedObject;
}
- initializeBean(初始化)方法具体实现
/************************************initializeBean源码************************************/
protected Object initializeBean(String beanName, Object bean, @Nullable RootBeanDefinition mbd) {
if (System.getSecurityManager() != null) {
AccessController.doPrivileged((PrivilegedAction<Object>) () -> {
invokeAwareMethods(beanName, bean);
return null;
}, getAccessControlContext());
}
else {
// 如果这个bean有实现回调接口 (beanNameAware BeanFactoryAware beanClassLoredAware )
// 就强转调用方法
invokeAwareMethods(beanName, bean);
}
Object wrappedBean = bean;
if (mbd == null || !mbd.isSynthetic()) {
// 调用所有BeanPostProcessors 的初始化前方法
wrappedBean = applyBeanPostProcessorsBeforeInitialization(wrappedBean, beanName);
}
try {
// 如果这个bean有实现接口 InitializingBean
// 就调用初始化方法
invokeInitMethods(beanName, wrappedBean, mbd);
}
catch (Throwable ex) {
throw new BeanCreationException(
(mbd != null ? mbd.getResourceDescription() : null),
beanName, "Invocation of init method failed", ex);
}
if (mbd == null || !mbd.isSynthetic()) {
// 调用所有BeanPostProcessors 的初始化后方法(aop基于初始化后实现的,所以这里有aop返回的是代理对象)
wrappedBean = applyBeanPostProcessorsAfterInitialization(wrappedBean, beanName);
}
return wrappedBean;
}
3.2.2.2 流程图
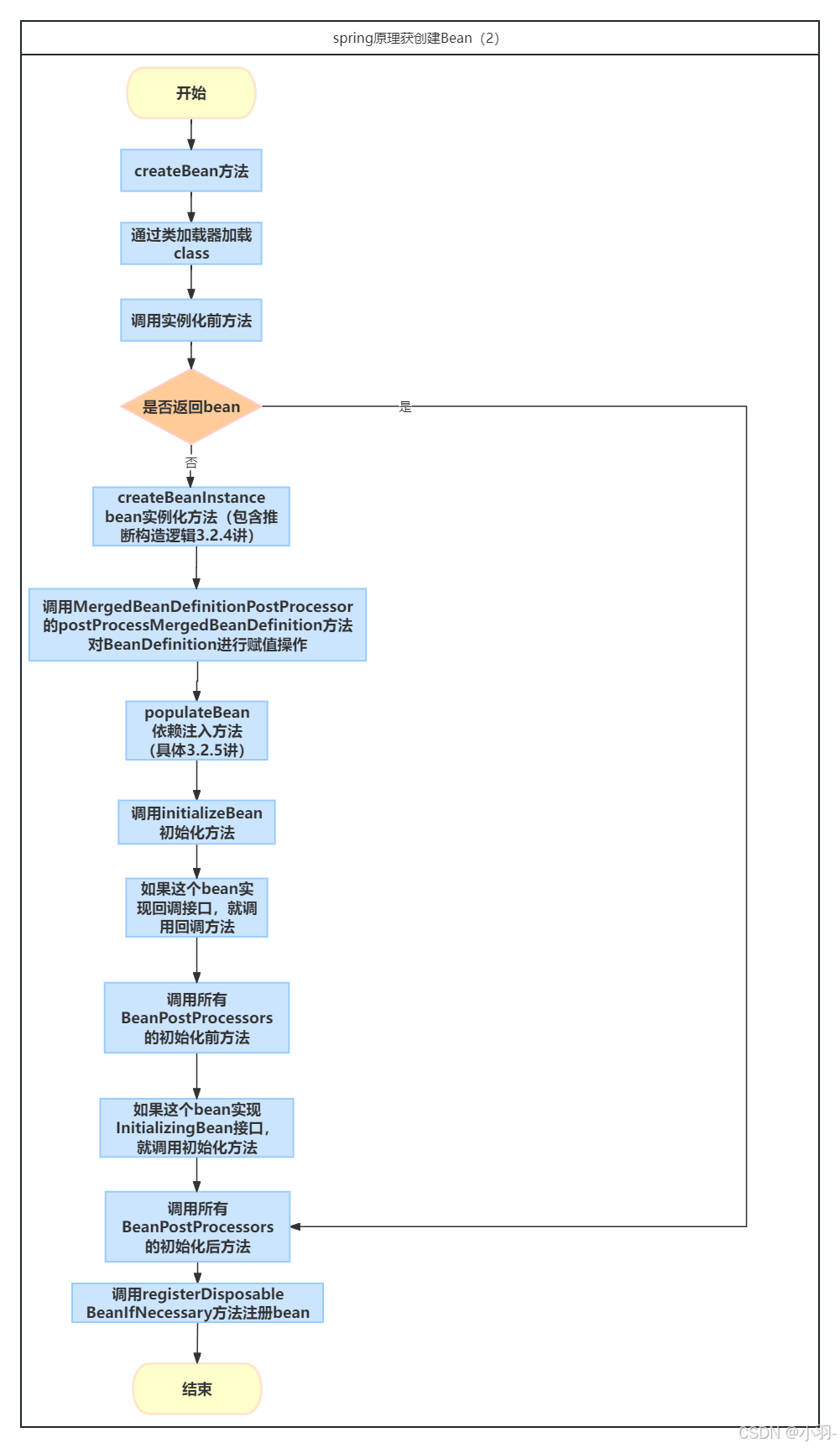
3.2.2.3 总结
先加载class文件,调用实例化前方法,然后实例化bean,在调用postProcessMergedBeanDefinition方法对BeanDefinition赋值,然后进行依赖注入(里面会调用实例化后方法),然后调用回调方法,在调用BeanPostProcessors初始化前方法,在调用初始化方法,在调用BeanPostProcessors初始化后方法,然后注册bean。
3.2.3 spring原理Bean的三级缓存(3)
3.2.3.1 源码
- getbean方法在创建bean之前会调getSingleton方法看看是否已存在,存在直接返回这个对象。
/************************************getSingleton源码*******************************************/
protected Object getSingleton(String beanName, boolean allowEarlyReference) {
// Quick check for existing instance without full singleton lock
// 先从一级缓存拿,也是就单例池
Object singletonObject = this.singletonObjects.get(beanName);
// 如果配置是支持循环依赖 同时 这个bean正在创建 (那么代表这个bean发生了循环依赖)
if (singletonObject == null && isSingletonCurrentlyInCreation(beanName)) {
// 从二级缓存获取 (二级缓存存的是未完成的bean,也就是没有进行没有初始化)
singletonObject = this.earlySingletonObjects.get(beanName);
if (singletonObject == null && allowEarlyReference) {
// 加锁
synchronized (this.singletonObjects) {
// Consistent creation of early reference within full singleton lock
// 下面的代码 再次从二级 一级缓存 拿对象,运用了双重检测机制
singletonObject = this.singletonObjects.get(beanName);
if (singletonObject == null) {
singletonObject = this.earlySingletonObjects.get(beanName);
if (singletonObject == null) {
// 从三级缓存拿到值,value是lambda表达式,也就是一个方法
ObjectFactory<?> singletonFactory = this.singletonFactories.get(beanName);
if (singletonFactory != null) {
// 调用后 得到 原对象或者代理对象,方法具体的实现后面讲
singletonObject = singletonFactory.getObject();
// 返回的结果存到二级缓存,删除三级缓存
this.earlySingletonObjects.put(beanName, singletonObject);
this.singletonFactories.remove(beanName);
}
}
}
}
}
}
return singletonObject;
}
- 在创建这个bean之前,会调用方法,标记这个bean正在创建
/************************************beforeSingletonCreation源码*******************************************/
// 创建单例bean 之前会调用这个方法,标记这个bean正在创建
protected void beforeSingletonCreation(String beanName) {
// 最终会添加到singletonsCurrentlyInCreation这个set集合中
// 判断这个对象是否在创建中,也是判断这个beanName是否在singletonsCurrentlyInCreation集合中
if (!this.inCreationCheckExclusions.contains(beanName) && !this.singletonsCurrentlyInCreation.add(beanName)) {
throw new BeanCurrentlyInCreationException(beanName);
}
}
- 实例化bean后,会放入三级缓存
/************************************doCreateBean部分源码*******************************************/
protected Object doCreateBean(String beanName, RootBeanDefinition mbd, @Nullable Object[] args)
throws BeanCreationException {
... 省略不重要的代码
if (instanceWrapper == null) {
// 实例化bean
instanceWrapper = createBeanInstance(beanName, mbd, args);
}
Object bean = instanceWrapper.getWrappedInstance();
... 省略不重要的代码
// 如果单例(多例不支持循环依赖)
// 同时 配置支持循环依赖
// 同时 这个bean正在创建 也是就在singletonsCurrentlyInCreation集合中
boolean earlySingletonExposure = (mbd.isSingleton() && this.allowCircularReferences &&
isSingletonCurrentlyInCreation(beanName));
if (earlySingletonExposure) {
if (logger.isTraceEnabled()) {
logger.trace("Eagerly caching bean '" + beanName +
"' to allow for resolving potential circular references");
}
// 就把它添加到三级缓存等其他操作
// getEarlyBeanReference方法 如果这个bean没有实现aop就返回原对象 否则返回代理对象
addSingletonFactory(beanName, () -> getEarlyBeanReference(beanName, mbd, bean));
}
... 省略不重要的代码
return exposedObject;
// addSingletonFactory方法源码
protected void addSingletonFactory(String beanName, ObjectFactory<?> singletonFactory) {
Assert.notNull(singletonFactory, "Singleton factory must not be null");
synchronized (this.singletonObjects) {
// 不存在单例池
if (!this.singletonObjects.containsKey(beanName)) {
// 把这个lambda表达式放入三级缓存
this.singletonFactories.put(beanName, singletonFactory);
// 删除 二级缓存
this.earlySingletonObjects.remove(beanName);
this.registeredSingletons.add(beanName);
}
}
}
}
/********************getEarlyBeanReference方法实现(也就是三级缓存中lambda表达式的实现)************************/
protected Object getEarlyBeanReference(String beanName, RootBeanDefinition mbd, Object bean) {
Object exposedObject = bean;
if (!mbd.isSynthetic() && hasInstantiationAwareBeanPostProcessors()) {
for (SmartInstantiationAwareBeanPostProcessor bp : getBeanPostProcessorCache().smartInstantiationAware) {
// 调用getEarlyBeanReference方法
exposedObject = bp.getEarlyBeanReference(exposedObject, beanName);
}
}
return exposedObject;
}
// AbstractAutoProxyCreator中的 getEarlyBeanReference方法实现
@Override
public Object getEarlyBeanReference(Object bean, String beanName) {
// 获取缓存key
Object cacheKey = getCacheKey(bean.getClass(), beanName);
// 添加到 earlyProxyReferences集合中,代表这个bean已经提前进行了aop
this.earlyProxyReferences.put(cacheKey, bean);
// 如果这个bean开启了aop,就提前创建代理对象并返回,否则返回原bean
return wrapIfNecessary(bean, beanName, cacheKey);
}
// AbstractAutoProxyCreator中的 postProcessAfterInitialization(初始化后)方法实现
@Override
public Object postProcessAfterInitialization(@Nullable Object bean, String beanName) {
if (bean != null) {
Object cacheKey = getCacheKey(bean.getClass(), beanName);
// 判断如果之前循环依赖 已经提前创建代理对象了,那么就直接返回bean
if (this.earlyProxyReferences.remove(cacheKey) != bean) {
return wrapIfNecessary(bean, beanName, cacheKey);
}
}
// 这里返回的是原对象
return bean;
}
- 在初始化后,会判断是否已经初始化
/************************************doCreateBean部分源码*******************************************/
protected Object doCreateBean(String beanName, RootBeanDefinition mbd, @Nullable Object[] args)
throws BeanCreationException {
... 省略不重要的代码
try {
// 依赖注入
populateBean(beanName, mbd, instanceWrapper);
// 初始化,如果已经提前进行aop 那么这里不会返回代理对象,而是原对象
exposedObject = initializeBean(beanName, exposedObject, mbd);
}
... 省略不重要的代码
// 如果出现了循环依赖
if (earlySingletonExposure) {
// 从二级缓存里面拿到 代理对象(如果有aop的话,否则是原对象)
Object earlySingletonReference = getSingleton(beanName, false);
if (earlySingletonReference != null) {
// 初始化返回的对象 跟 原对象相同
if (exposedObject == bean) {
// 就把代理对象赋值返回(如果有aop的话,否则是原对象)
exposedObject = earlySingletonReference;
}
... 省略不重要的代码
return exposedObject;
}
3.2.3.2 流程图
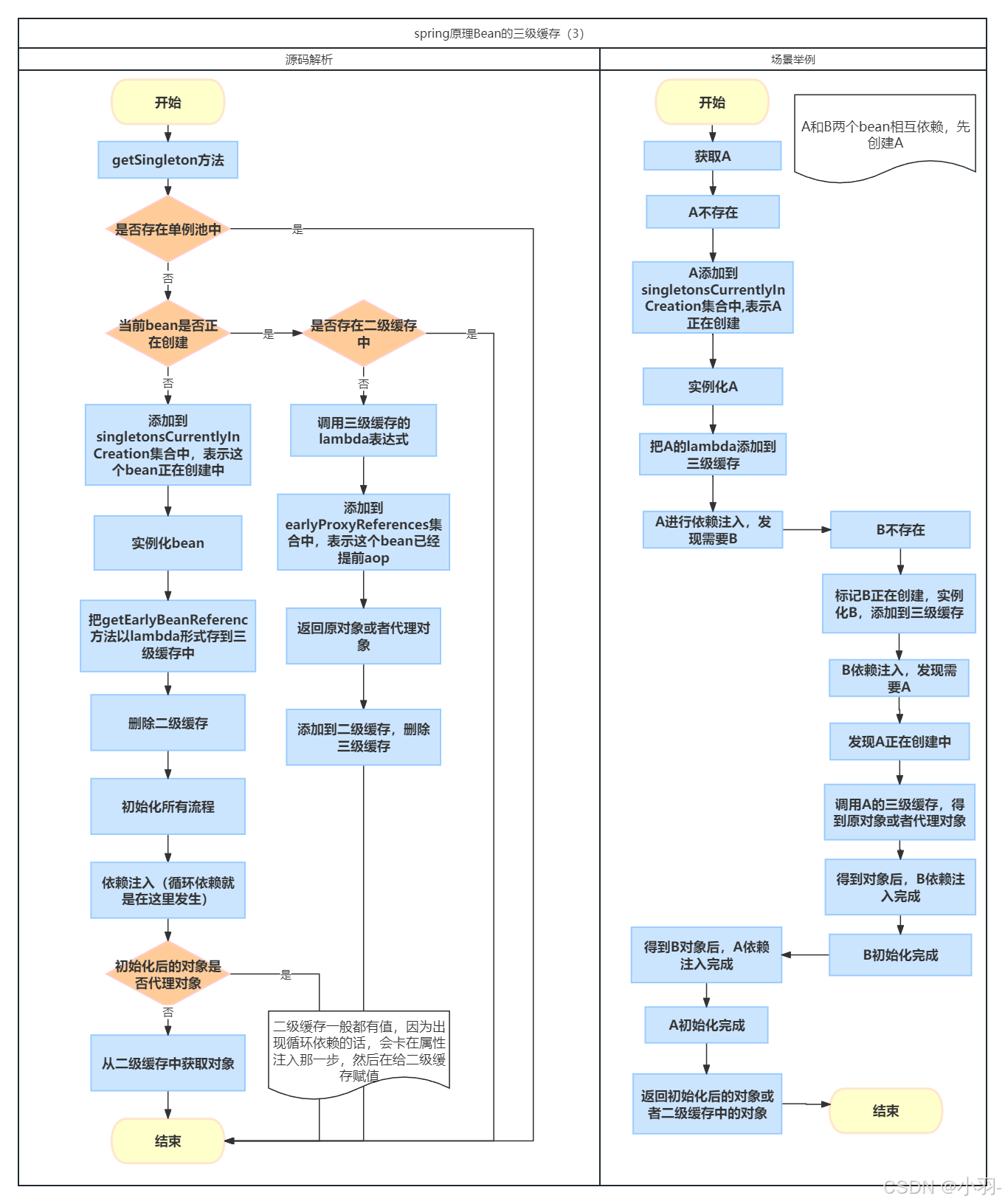
3.2.3.3 总结
spring是通过三个缓存,两个set来解决循环依赖的,创建bean的时候会把beanname放在singletonsCurrentlyInCreation集合中,表示这个bean正在创建,同时在实例化完成后会把,如果没有aop就返回实例化对象,有就提前创建代理对象这个逻辑,以lambda的方式放入三级缓存;在后续的属性注入的流程中,就可以通过这个bean是否正在创建来,判断是否出现了循环依赖,如果出现循环依赖,通过三级缓存的lambda表达式,提前aop并记录到earlyProxyReferences集合中,表示这个bean已经提前aop,得到最终的对象,然后完成属性注入和赋值,这样就解决了循环依赖。
3.2.4 spring原理Bean的依赖注入(4)
3.2.4.1 源码
- 依赖注入之前,先调用applyMergedBeanDefinitionPostProcessors方法,调用MergedBeanDefinitionPostProcessor接口中的postProcessMergedBeanDefinition方法,@vlaue@Autowired的实现类,实现了postProcessMergedBeanDefinition方法,在postProcessMergedBeanDefinition方法中提前扫码这个bean的属性和set方法,符合条件的放入集合中。
/************************************doCreateBean部分源码*******************************************/
protected Object doCreateBean(String beanName, RootBeanDefinition mbd, @Nullable Object[] args)
throws BeanCreationException {
... 省略不重要的代码
synchronized (mbd.postProcessingLock) {
if (!mbd.postProcessed) {
try {
// 里面遍历所有实现MergedBeanDefinitionPostProcessor接口的类,调用postProcessMergedBeanDefinition方法
// @vlaue@Autowired的实现类,实现了MergedBeanDefinitionPostProcessor接口
//在postProcessMergedBeanDefinition方法中提,前扫码这个bean的属性和set方法,符合条件的放入集合中
// 具体逻辑 后面单独看
applyMergedBeanDefinitionPostProcessors(mbd, beanType, beanName);
}
catch (Throwable ex) {
throw new BeanCreationException(mbd.getResourceDescription(), beanName,
"Post-processing of merged bean definition failed", ex);
}
mbd.postProcessed = true;
}
}
... 省略不重要的代码
try {
// 调用依赖注入方法
populateBean(beanName, mbd, instanceWrapper);
... 省略不重要的代码
}
... 省略不重要的代码
return exposedObject;
}
- 进入populateBean方法
/************************************populateBean部分源码*******************************************/
protected void populateBean(String beanName, RootBeanDefinition mbd, @Nullable BeanWrapper bw) {
if (bw == null) {
if (mbd.hasPropertyValues()) {
throw new BeanCreationException(
mbd.getResourceDescription(), beanName, "Cannot apply property values to null instance");
}
else {
// Skip property population phase for null instance.
return;
}
}
// 调用实例化后方法
if (!mbd.isSynthetic() && hasInstantiationAwareBeanPostProcessors()) {
for (InstantiationAwareBeanPostProcessor bp : getBeanPostProcessorCache().instantiationAware) {
if (!bp.postProcessAfterInstantiation(bw.getWrappedInstance(), beanName)) {
return;
}
}
}
PropertyValues pvs = (mbd.hasPropertyValues() ? mbd.getPropertyValues() : null);
// 拿到@Bean注解里面Autowire属性的值。
int resolvedAutowireMode = mbd.getResolvedAutowireMode();
if (resolvedAutowireMode == AUTOWIRE_BY_NAME || resolvedAutowireMode == AUTOWIRE_BY_TYPE) {
MutablePropertyValues newPvs = new MutablePropertyValues(pvs);
// 如果Autowire属性的值是byname
// 扫码set方法,通过name去找bean注入
// 具体逻辑 后面单独看
if (resolvedAutowireMode == AUTOWIRE_BY_NAME) {
autowireByName(beanName, mbd, bw, newPvs);
}
// 如果Autowire属性的值是byType
// 扫码set方法,通过入参的类型去找bean注入
if (resolvedAutowireMode == AUTOWIRE_BY_TYPE) {
autowireByType(beanName, mbd, bw, newPvs);
}
// 赋值到pvs集合中
pvs = newPvs;
}
boolean hasInstAwareBpps = hasInstantiationAwareBeanPostProcessors();
boolean needsDepCheck = (mbd.getDependencyCheck() != AbstractBeanDefinition.DEPENDENCY_CHECK_NONE);
PropertyDescriptor[] filteredPds = null;
if (hasInstAwareBpps) {
if (pvs == null) {
pvs = mbd.getPropertyValues();
}
for (InstantiationAwareBeanPostProcessor bp : getBeanPostProcessorCache().instantiationAware) {
PropertyValues pvsToUse = bp.postProcessProperties(pvs, bw.getWrappedInstance(), beanName);
if (pvsToUse == null) {
if (filteredPds == null) {
filteredPds = filterPropertyDescriptorsForDependencyCheck(bw, mbd.allowCaching);
}
// 调用注入方法。
//@vlaue@Autowired的实现类,还实现了InstantiationAwareBeanPostProcessor中的注入方法
// 具体逻辑 后面单独看
pvsToUse = bp.postProcessPropertyValues(pvs, filteredPds, bw.getWrappedInstance(), beanName);
if (pvsToUse == null) {
return;
}
}
pvs = pvsToUse;
}
}
if (needsDepCheck) {
if (filteredPds == null) {
filteredPds = filterPropertyDescriptorsForDependencyCheck(bw, mbd.allowCaching);
}
checkDependencies(beanName, mbd, filteredPds, pvs);
}
if (pvs != null) {
// 最后把pvs集合中需要注入的属性和BeanDefinition设置的属性赋值。
applyPropertyValues(beanName, mbd, bw, pvs);
}
}
- autowireByName逻辑实现,autowireBType的逻辑和autowireByName差不多。
/************************************autowireByName源码*******************************************/
protected void autowireByName(
String beanName, AbstractBeanDefinition mbd, BeanWrapper bw, MutablePropertyValues pvs) {
// 通过方法 属性 拿到这个bean需要注入的属性name,会过滤调没有set方法的属性
// 具体逻辑看下面
String[] propertyNames = unsatisfiedNonSimpleProperties(mbd, bw);
for (String propertyName : propertyNames) {
// 判断这个bean 是否存在bean工厂
if (containsBean(propertyName)) {
// 获取或者创建bean (也就是这里会触发循环依赖)
Object bean = getBean(propertyName);
// 添加到集合中记录下来,最后在方法中统一赋值注入。
pvs.add(propertyName, bean);
registerDependentBean(propertyName, beanName);
if (logger.isTraceEnabled()) {
logger.trace("Added autowiring by name from bean name '" + beanName +
"' via property '" + propertyName + "' to bean named '" + propertyName + "'");
}
}
else {
if (logger.isTraceEnabled()) {
logger.trace("Not autowiring property '" + propertyName + "' of bean '" + beanName +
"' by name: no matching bean found");
}
}
}
}
// unsatisfiedNonSimpleProperties方法源码
protected String[] unsatisfiedNonSimpleProperties(AbstractBeanDefinition mbd, BeanWrapper bw) {
Set<String> result = new TreeSet<>();
PropertyValues pvs = mbd.getPropertyValues();
// 通过这个bean的方法和属性 拿到PropertyDescriptor数组
PropertyDescriptor[] pds = bw.getPropertyDescriptors();
for (PropertyDescriptor pd : pds) {
// pd.getWriteMethod() 表示没有set方法
// !pvs.contains(pd.getName()) 表示不存在 pvs中
// !BeanUtils.isSimpleProperty(pd.getPropertyType()) 表示 类型不能是基础类型 如String、Number等
if (pd.getWriteMethod() != null && !isExcludedFromDependencyCheck(pd) && !pvs.contains(pd.getName()) &&
!BeanUtils.isSimpleProperty(pd.getPropertyType())) {
// 添加到集合中 返回
result.add(pd.getName());
}
}
return StringUtils.toStringArray(result);
}
- @vlaue@Autowired的实现原理,AutowiredAnnotationBeanPostProcessor类中才是具体实现了@Autowired等注解,AutowiredAnnotationBeanPostProcessor实现了MergedBeanDefinitionPostProcessor的postProcessMergedBeanDefinition方法,在这个方法实现了注入之前提前找到需要注入的方法和属性的逻辑。
/************AutowiredAnnotationBeanPostProcessor的postProcessMergedBeanDefinition方法部分源码******/
// postProcessMergedBeanDefinition 这个方法主要提前找到注入点缓存下来
@Override
public void postProcessMergedBeanDefinition(RootBeanDefinition beanDefinition, Class<?> beanType, String beanName) {
// 找到注入点 具体看这个方法
InjectionMetadata metadata = findAutowiringMetadata(beanName, beanType, null);
metadata.checkConfigMembers(beanDefinition);
}
// findAutowiringMetadata源码
private InjectionMetadata findAutowiringMetadata(String beanName, Class<?> clazz, @Nullable PropertyValues pvs) {
// 先找缓存
String cacheKey = (StringUtils.hasLength(beanName) ? beanName : clazz.getName());
InjectionMetadata metadata = this.injectionMetadataCache.get(cacheKey);
// 缓存没有
if (InjectionMetadata.needsRefresh(metadata, clazz)) {
// 加锁
synchronized (this.injectionMetadataCache) {
// 双重检测
metadata = this.injectionMetadataCache.get(cacheKey);
if (InjectionMetadata.needsRefresh(metadata, clazz)) {
if (metadata != null) {
metadata.clear(pvs);
}
// 找到注入点 具体看这个方法
metadata = buildAutowiringMetadata(clazz);
this.injectionMetadataCache.put(cacheKey, metadata);
}
}
}
return metadata;
}
// buildAutowiringMetadata源码
private InjectionMetadata buildAutowiringMetadata(Class<?> clazz) {
// 如果bean的类型是string 直接返回
if (!AnnotationUtils.isCandidateClass(clazz, this.autowiredAnnotationTypes)) {
return InjectionMetadata.EMPTY;
}
List<InjectionMetadata.InjectedElement> elements = new ArrayList<>();
Class<?> targetClass = clazz;
do {
final List<InjectionMetadata.InjectedElement> currElements = new ArrayList<>();
// 遍历这个bean中的属性
ReflectionUtils.doWithLocalFields(targetClass, field -> {
// 判断 这个属性上是否有 @vlaue@Autowired
MergedAnnotation<?> ann = findAutowiredAnnotation(field);
if (ann != null) {
// 过滤静态属性
if (Modifier.isStatic(field.getModifiers())) {
if (logger.isInfoEnabled()) {
logger.info("Autowired annotation is not supported on static fields: " + field);
}
return;
}
// 添加到注入点
boolean required = determineRequiredStatus(ann);
currElements.add(new AutowiredFieldElement(field, required));
}
});
// 遍历这个bean中的方法
ReflectionUtils.doWithLocalMethods(targetClass, method -> {
// 过滤泛型的桥接方法
Method bridgedMethod = BridgeMethodResolver.findBridgedMethod(method);
if (!BridgeMethodResolver.isVisibilityBridgeMethodPair(method, bridgedMethod)) {
return;
}
MergedAnnotation<?> ann = findAutowiredAnnotation(bridgedMethod);
// 判断 这个方法上是否有 @vlaue@Autowired
if (ann != null && method.equals(ClassUtils.getMostSpecificMethod(method, clazz))) {
// 过滤掉静态方法
if (Modifier.isStatic(method.getModifiers())) {
if (logger.isInfoEnabled()) {
logger.info("Autowired annotation is not supported on static methods: " + method);
}
return;
}
// 方法参数为空 打印log
if (method.getParameterCount() == 0) {
if (logger.isInfoEnabled()) {
logger.info("Autowired annotation should only be used on methods with parameters: " +
method);
}
}
// 添加到注入点
boolean required = determineRequiredStatus(ann);
PropertyDescriptor pd = BeanUtils.findPropertyForMethod(bridgedMethod, clazz);
currElements.add(new AutowiredMethodElement(method, required, pd));
}
});
elements.addAll(0, currElements);
targetClass = targetClass.getSuperclass();
}
// 循环this 和父类
while (targetClass != null && targetClass != Object.class);
// 封装成对象返回
return InjectionMetadata.forElements(elements, clazz);
}
- @vlaue@Autowired的实现原理,AutowiredAnnotationBeanPostProcessor类中才是具体实现了@Autowired等注解,AutowiredAnnotationBeanPostProcessor实现了InstantiationAwareBeanPostProcessor的postProcessPropertyValues方法,在这个方法实现了注入逻辑
/***************AutowiredAnnotationBeanPostProcessor的postProcessProperties方法部分源码****************/
// postProcessProperties 在这个方法里面注入赋值
@Override
public PropertyValues postProcessProperties(PropertyValues pvs, Object bean, String beanName) {
// 从缓存中拿到注入点
InjectionMetadata metadata = findAutowiringMetadata(beanName, bean.getClass(), pvs);
try {
// 具体看这个方法
metadata.inject(bean, beanName, pvs);
}
catch (BeanCreationException ex) {
throw ex;
}
catch (Throwable ex) {
throw new BeanCreationException(beanName, "Injection of autowired dependencies failed", ex);
}
return pvs;
}
// inject 方法源码
public void inject(Object target, @Nullable String beanName, @Nullable PropertyValues pvs) throws Throwable {
Collection<InjectedElement> checkedElements = this.checkedElements;
Collection<InjectedElement> elementsToIterate =
(checkedElements != null ? checkedElements : this.injectedElements);
if (!elementsToIterate.isEmpty()) {
for (InjectedElement element : elementsToIterate) {
// 这个方法有两个实现,一个方法层的,一个属性层的
// 具体看这个方法
element.inject(target, beanName, pvs);
}
}
}
/********************************************inject属性层实现*****************************************/
protected void inject(Object bean, @Nullable String beanName, @Nullable PropertyValues pvs) throws Throwable {
Field field = (Field) this.member;
Object value;
// 如果有缓存从缓存里面拿
if (this.cached) {
try {
value = resolvedCachedArgument(beanName, this.cachedFieldValue);
}
catch (NoSuchBeanDefinitionException ex) {
// Unexpected removal of target bean for cached argument -> re-resolve
value = resolveFieldValue(field, bean, beanName);
}
}
else {
// 主要看这个方法
value = resolveFieldValue(field, bean, beanName);
}
if (value != null) {
ReflectionUtils.makeAccessible(field);
// 拿到value后 通过反射给属性赋值
field.set(bean, value);
}
}
// resolveFieldValue方法实现
DependencyDescriptor desc = new DependencyDescriptor(field, this.required);
desc.setContainingClass(bean.getClass());
Set<String> autowiredBeanNames = new LinkedHashSet<>(1);
Assert.state(beanFactory != null, "No BeanFactory available");
TypeConverter typeConverter = beanFactory.getTypeConverter();
Object value;
try {
// 主要看这个方法 属性赋值 和方法赋值都是从这里找的bean
value = beanFactory.resolveDependency(desc, beanName, autowiredBeanNames, typeConverter);
}
catch (BeansException ex) {
throw new UnsatisfiedDependencyException(null, beanName, new InjectionPoint(field), ex);
}
... 省略不重要的代码
/********************************************inject方法层实现*****************************************/
protected void inject(Object bean, @Nullable String beanName, @Nullable PropertyValues pvs) throws Throwable {
if (checkPropertySkipping(pvs)) {
return;
}
Method method = (Method) this.member;
Object[] arguments;
if (this.cached) {
try {
arguments = resolveCachedArguments(beanName);
}
catch (NoSuchBeanDefinitionException ex) {
// Unexpected removal of target bean for cached argument -> re-resolve
arguments = resolveMethodArguments(method, bean, beanName);
}
}
else {
// 主要看这个方法
arguments = resolveMethodArguments(method, bean, beanName);
}
if (arguments != null) {
try {
ReflectionUtils.makeAccessible(method);
// 拿到方法入参数组后,通过反射调用方法赋值
method.invoke(bean, arguments);
}
catch (InvocationTargetException ex) {
throw ex.getTargetException();
}
}
}
// resolveMethodArguments 方法
private Object[] resolveMethodArguments(Method method, Object bean, @Nullable String beanName) {
// 获取方法参数的数量
int argumentCount = method.getParameterCount();
// 通过数量 创建方法入参数组
Object[] arguments = new Object[argumentCount];
DependencyDescriptor[] descriptors = new DependencyDescriptor[argumentCount];
Set<String> autowiredBeans = new LinkedHashSet<>(argumentCount);
Assert.state(beanFactory != null, "No BeanFactory available");
TypeConverter typeConverter = beanFactory.getTypeConverter();
for (int i = 0; i < arguments.length; i++) {
MethodParameter methodParam = new MethodParameter(method, i);
DependencyDescriptor currDesc = new DependencyDescriptor(methodParam, this.required);
currDesc.setContainingClass(bean.getClass());
descriptors[i] = currDesc;
try {
// 主要看这个方法 属性赋值 和方法赋值都是从这里找的bean
Object arg = beanFactory.resolveDependency(currDesc, beanName, autowiredBeans, typeConverter);
if (arg == null && !this.required) {
arguments = null;
break;
}
// 拿到后添加到数组里
arguments[i] = arg;
}
catch (BeansException ex) {
throw new UnsatisfiedDependencyException(null, beanName, new InjectionPoint(methodParam), ex);
}
}
... 省略不重要的代码
return arguments;
}
/*********************beanFactory.resolveDependency方法实现-所有依赖注入找对象都是通过这个方法*******************/
public Object resolveDependency(DependencyDescriptor descriptor, @Nullable String requestingBeanName,
@Nullable Set<String> autowiredBeanNames, @Nullable TypeConverter typeConverter) throws BeansException {
descriptor.initParameterNameDiscovery(getParameterNameDiscoverer());
// 类型是Optional的实现
if (Optional.class == descriptor.getDependencyType()) {
return createOptionalDependency(descriptor, requestingBeanName);
}
// 类型是ObjectFactory/ObjectProvider的实现
else if (ObjectFactory.class == descriptor.getDependencyType() ||
ObjectProvider.class == descriptor.getDependencyType()) {
return new DependencyObjectProvider(descriptor, requestingBeanName);
}
else if (javaxInjectProviderClass == descriptor.getDependencyType()) {
return new Jsr330Factory().createDependencyProvider(descriptor, requestingBeanName);
}
else {
// 如果是懒加载,先返回一个代理对象,在使用的时候才去找bean注入
Object result = getAutowireCandidateResolver().getLazyResolutionProxyIfNecessary(
descriptor, requestingBeanName);
if (result == null) {
// 主要看这个方法
result = doResolveDependency(descriptor, requestingBeanName, autowiredBeanNames, typeConverter);
}
return result;
}
}
// doResolveDependency
public Object doResolveDependency(DependencyDescriptor descriptor, @Nullable String beanName,
@Nullable Set<String> autowiredBeanNames, @Nullable TypeConverter typeConverter) throws BeansException {
InjectionPoint previousInjectionPoint = ConstructorResolver.setCurrentInjectionPoint(descriptor);
try {
Object shortcut = descriptor.resolveShortcut(this);
if (shortcut != null) {
return shortcut;
}
Class<?> type = descriptor.getDependencyType();
// 如果是@value,拿到@value注解里面的值
Object value = getAutowireCandidateResolver().getSuggestedValue(descriptor);
if (value != null) {
if (value instanceof String) {
// 占位符$填充
String strVal = resolveEmbeddedValue((String) value);
BeanDefinition bd = (beanName != null && containsBean(beanName) ?
getMergedBeanDefinition(beanName) : null);
// 解析spring表达式#{}
value = evaluateBeanDefinitionString(strVal, bd);
}
TypeConverter converter = (typeConverter != null ? typeConverter : getTypeConverter());
try {
// 通过属性描述器转换 然后返回
return converter.convertIfNecessary(value, type, descriptor.getTypeDescriptor());
}
catch (UnsupportedOperationException ex) {
// A custom TypeConverter which does not support TypeDescriptor resolution...
return (descriptor.getField() != null ?
converter.convertIfNecessary(value, type, descriptor.getField()) :
converter.convertIfNecessary(value, type, descriptor.getMethodParameter()));
}
}
// 如果属性的类型的map/list等集合类型,直接找到匹配类型的所有bean封装成list或者map返回
// map的key是beanname
Object multipleBeans = resolveMultipleBeans(descriptor, beanName, autowiredBeanNames, typeConverter);
// 不为空代表类型是集合类型 直接找到返回
if (multipleBeans != null) {
return multipleBeans;
}
// 通过属性或者方法入参的类型 通过BeanDefinition找到所有的对象
// 然后进行第一次过滤
//1.autowireCandidate过滤掉false的
//2.如果注入点是泛型,找到具体的class类型和注入的class类型一样
//3. 如果注入点上有@Qualifier注解,那么过滤掉没有@Qualifier注解的,或者注解上的值不一致
// 封装成map对象返回
Map<String, Object> matchingBeans = findAutowireCandidates(beanName, type, descriptor);
if (matchingBeans.isEmpty()) {
// 为空,如果开启校验配置,直接抛异常;否则返回为空
if (isRequired(descriptor)) {
raiseNoMatchingBeanFound(type, descriptor.getResolvableType(), descriptor);
}
return null;
}
String autowiredBeanName;
Object instanceCandidate;
// 找到多个bean 进行二次过滤
if (matchingBeans.size() > 1) {
// 第二次过滤
// 如果找到的bean被@primary注解修饰,代表是主bean,直接返回这个bean
// 如果找到的bean被@priority注解修饰,根据优先级选择,数字越小的直接返回
// 根据name去匹配bean
autowiredBeanName = determineAutowireCandidate(matchingBeans, descriptor);
if (autowiredBeanName == null) {
// 找不到想要的bean,同时配置开启校验,直接抛异常
if (isRequired(descriptor) || !indicatesMultipleBeans(type)) {
return descriptor.resolveNotUnique(descriptor.getResolvableType(), matchingBeans);
}
else {
// In case of an optional Collection/Map, silently ignore a non-unique case:
// possibly it was meant to be an empty collection of multiple regular beans
// (before 4.3 in particular when we didn't even look for collection beans).
return null;
}
}
instanceCandidate = matchingBeans.get(autowiredBeanName);
}
else {
// We have exactly one match.
// 如果是一个对象,直接赋值key value
Map.Entry<String, Object> entry = matchingBeans.entrySet().iterator().next();
autowiredBeanName = entry.getKey();
instanceCandidate = entry.getValue();
}
if (autowiredBeanNames != null) {
autowiredBeanNames.add(autowiredBeanName);
}
if (instanceCandidate instanceof Class) {
// 如果对象的值是class对象,代表还没有创建,直接调用getbean方法获取/创建bean
instanceCandidate = descriptor.resolveCandidate(autowiredBeanName, type, this);
}
Object result = instanceCandidate;
// 如果为空对象
if (result instanceof NullBean) {
// 抛异常或者返回空
if (isRequired(descriptor)) {
raiseNoMatchingBeanFound(type, descriptor.getResolvableType(), descriptor);
}
result = null;
}
if (!ClassUtils.isAssignableValue(type, result)) {
throw new BeanNotOfRequiredTypeException(autowiredBeanName, type, instanceCandidate.getClass());
}
return result;
}
finally {
ConstructorResolver.setCurrentInjectionPoint(previousInjectionPoint);
}
}
- CommonAnnotationBeanPostProcessor类实现了Resource注解,逻辑和Autowired差不多
3.2.4.2 流程图
-
依赖注入整体流程和@vlaue或@Autowired实现原理
-
resolveDependency方法实现逻辑(通过类型找到要注入的值)
3.2.4.3 总结
spring在注入之前会调用postProcessMergedBeanDefinition方法,然后进入到依赖注入方法,然后调用实例化后方法,在判断@Bean注解是否开启了autowireByName或者autowireByType,这个东西用的很少已经过时了;原理是找到这个bean所有的属性和set方法,过滤掉没有set方法的属性,得到一个beanname的数组,然后判断这个beanname是否存在bean工厂里,存在就通过getbean的方式获取,然后放到一个pvs集合中,区别是一个通过name获取(getbean),一个通过type获取(resolveDependency);然后在调用postProcessPropertyValues方法,最后在把pvs集合和BeanDefinition设置的属性赋值。
@value@Autowired@Resources 是基于postProcessMergedBeanDefinition和postProcessPropertyValues方法实现的,他们的实现类实现了这两个方法在,在postProcessMergedBeanDefinition方法提前找到注入点,先遍历这个bean的方法和属性,过滤掉没有@value@Autowired@Resources 注解标识的,然后过滤掉被静态方法修饰的(@Resources的实现类是发现直接抛异常),过滤掉桥接方法,然后放入一个集合中,封装成对象缓存起来;在postProcessPropertyValues方法实现属性注入,先从缓存中拿到注入点,方法是遍历入参,然后通过resolveDependency方法找到对象,最终返回一个入参数组,通过反射加入参调用方法完成依赖注入。
方法和属性都是通过resolveDependency方法找到对象,先判断这个属性或者入参是否懒加载,如果是返回一个代理对象,不是拿到@value的值,解析占位符和spring表达式,通过属性转换器返回,如果没有值,那就是@Autowired的逻辑,如果属性和入参的类型是集合,直接通过类型找到所有值,然后封装成list或者map返回,不是集合的情况,也是通过类型在BeanDefinition找到符合条件的bean,然后进行筛选,过滤掉autowireCandidatefalse的、找到具体的class类型过滤掉类型不一样的、注入点上有@Qualifier注解,那么过滤掉没有@Qualifier注解的,或者注解上的值不一致,如果筛选出来的bean没有,就抛异常;如果有同时大于两个,进行二次筛选,找到的bean有被@primary注解修饰的,直接返回这个bean,找到被@priority注解修饰的bean选优先级高的,直接返回这个bean,都没有的情况下,通过name去找,如果name没找到,就抛异常;如果找到的不是实例对象,而是一个class对象,证明这个bean还没创建,先创建获取后再返回。
3.2.5 spring原理Bean的推断构造 (5)
3.2.5.1 源码
- 进入createBeanInstance方法
protected BeanWrapper createBeanInstance(String beanName, RootBeanDefinition mbd, @Nullable Object[] args) {
// Make sure bean class is actually resolved at this point.
// 加载class到jvm
Class<?> beanClass = resolveBeanClass(mbd, beanName);
if (beanClass != null && !Modifier.isPublic(beanClass.getModifiers()) && !mbd.isNonPublicAccessAllowed()) {
throw new BeanCreationException(mbd.getResourceDescription(), beanName,
"Bean class isn't public, and non-public access not allowed: " + beanClass.getName());
}
// 如果BeanDefinition设置了Supplier接口,那么就直接返回Supplier接口get方法的值
Supplier<?> instanceSupplier = mbd.getInstanceSupplier();
if (instanceSupplier != null) {
return obtainFromSupplier(instanceSupplier, beanName);
}
// @bean的实现
if (mbd.getFactoryMethodName() != null) {
return instantiateUsingFactoryMethod(beanName, mbd, args);
}
// Shortcut when re-creating the same bean...
// 有缓存的情况 一般只有多例才会用到缓存
boolean resolved = false;
boolean autowireNecessary = false;
if (args == null) {
synchronized (mbd.constructorArgumentLock) {
if (mbd.resolvedConstructorOrFactoryMethod != null) {
resolved = true;
autowireNecessary = mbd.constructorArgumentsResolved;
}
}
}
if (resolved) {
if (autowireNecessary) {
return autowireConstructor(beanName, mbd, null, null);
}
else {
return instantiateBean(beanName, mbd);
}
}
// Candidate constructors for autowiring?
// 去找构造方法
// 具体逻辑是,1. 如果所有构造方法没有加Autowired注解,
// 1.2 有多个构造方法 返回 null 最后用无参构造方法创建对象
// 1.3 只有一个无参构造方法 返回 null 最后用无参构造方法创建对象
// 1.4 只有一个有参构造方法 返回这个方法
// 2. 有构造方法加了Autowired注解
// 2.1 有一个构造方法required参数为true,同时加了Autowired注解的构造方法大于1,抛异常
// 2.2 有一个构造方法required参数为true,同时加了Autowired注解的构造方法等于1,返回这个方法
// 2.3 所有加了Autowired注解的构造方法的required参数都为false,返回这些方法
// 具体看这个方法
Constructor<?>[] ctors = determineConstructorsFromBeanPostProcessors(beanClass, beanName);
// mbd.getResolvedAutowireMode() == AUTOWIRE_CONSTRUCTOR代表开启了spring自动找构造方法的配置
// 如果找到构造方法 或者 开启自动找构造方法配置 或者 getbean方法传了构造方法的参数
if (ctors != null || mbd.getResolvedAutowireMode() == AUTOWIRE_CONSTRUCTOR ||
mbd.hasConstructorArgumentValues() || !ObjectUtils.isEmpty(args)) {
// 1.getbean法传了构造方法的参数
// 同时没有开启AUTOWIRE_CONSTRUCTOR配置,选择入参数量和类型匹配的
// 同时开启AUTOWIRE_CONSTRUCTOR配置,选入参最多的
// 1.有多个构造方法 通过Autowired找到的
// 选择入参参数最大的,如果构造方法参数相同,选评分最低的
return autowireConstructor(beanName, mbd, ctors, args);
}
// Preferred constructors for default construction?
ctors = mbd.getPreferredConstructors();
if (ctors != null) {
return autowireConstructor(beanName, mbd, ctors, null);
}
// No special handling: simply use no-arg constructor.
// 没有找到构造方法的情况 直接调用无参构造方法实例化对象
return instantiateBean(beanName, mbd);
}
- determineConstructorsFromBeanPostProcessors方法具体实现
@Nullable
protected Constructor<?>[] determineConstructorsFromBeanPostProcessors(@Nullable Class<?> beanClass, String beanName)
throws BeansException {
if (beanClass != null && hasInstantiationAwareBeanPostProcessors()) {
for (SmartInstantiationAwareBeanPostProcessor bp : getBeanPostProcessorCache().smartInstantiationAware) {
// 具体是调用了AutowiredAnnotationBeanPostProcessor类的determineCandidateConstructors方法
Constructor<?>[] ctors = bp.determineCandidateConstructors(beanClass, beanName);
if (ctors != null) {
return ctors;
}
}
}
return null;
}
// AutowiredAnnotationBeanPostProcessor类的determineCandidateConstructors方法源码逻辑
public Constructor<?>[] determineCandidateConstructors(Class<?> beanClass, final String beanName)
throws BeanCreationException {
...省略掉Lookup注解的实现
// Quick check on the concurrent map first, with minimal locking.
// 从缓存里面拿
Constructor<?>[] candidateConstructors = this.candidateConstructorsCache.get(beanClass);
if (candidateConstructors == null) {
// Fully synchronized resolution now...
synchronized (this.candidateConstructorsCache) {
candidateConstructors = this.candidateConstructorsCache.get(beanClass);
if (candidateConstructors == null) {
Constructor<?>[] rawCandidates;
try {
// 拿到所以构造方法
rawCandidates = beanClass.getDeclaredConstructors();
}
catch (Throwable ex) {
throw new BeanCreationException(beanName,
"Resolution of declared constructors on bean Class [" + beanClass.getName() +
"] from ClassLoader [" + beanClass.getClassLoader() + "] failed", ex);
}
List<Constructor<?>> candidates = new ArrayList<>(rawCandidates.length);
Constructor<?> requiredConstructor = null;
Constructor<?> defaultConstructor = null;
Constructor<?> primaryConstructor = BeanUtils.findPrimaryConstructor(beanClass);
int nonSyntheticConstructors = 0;
for (Constructor<?> candidate : rawCandidates) {
if (!candidate.isSynthetic()) {
nonSyntheticConstructors++;
}
else if (primaryConstructor != null) {
continue;
}
// 判断这个构造码放是否有Autowired注解
MergedAnnotation<?> ann = findAutowiredAnnotation(candidate);
if (ann == null) {
Class<?> userClass = ClassUtils.getUserClass(beanClass);
if (userClass != beanClass) {
try {
Constructor<?> superCtor =
userClass.getDeclaredConstructor(candidate.getParameterTypes());
ann = findAutowiredAnnotation(superCtor);
}
catch (NoSuchMethodException ex) {
// Simply proceed, no equivalent superclass constructor found...
}
}
}
// 有Autowired注解
if (ann != null) {
// 如果还有其他Autowired注解 同时required参数为true 就抛异常
// 意思是有一个Autowired注解为true的构造方法了,其他构造方法就不能有Autowired注解
if (requiredConstructor != null) {
throw new BeanCreationException(beanName,
"Invalid autowire-marked constructor: " + candidate +
". Found constructor with 'required' Autowired annotation already: " +
requiredConstructor);
}
boolean required = determineRequiredStatus(ann);
// 如果当前构造方法 required参数为true
if (required) {
// 同时还有其他构造方法 带有Autowired注解 就抛异常
if (!candidates.isEmpty()) {
throw new BeanCreationException(beanName,
"Invalid autowire-marked constructors: " + candidates +
". Found constructor with 'required' Autowired annotation: " +
candidate);
}
requiredConstructor = candidate;
}
// 如果当前构造方法带有Autowired注解 就添加到candidates集合
candidates.add(candidate);
}
// 没有Autowired注解 同时入参为0
else if (candidate.getParameterCount() == 0) {
// 记录无参构造方法
defaultConstructor = candidate;
}
}
// 如果所以构造方法都没有加Autowired注解
if (!candidates.isEmpty()) {
// Add default constructor to list of optional constructors, as fallback.
if (requiredConstructor == null) {
if (defaultConstructor != null) {
candidates.add(defaultConstructor);
}
else if (candidates.size() == 1 && logger.isInfoEnabled()) {
logger.info("Inconsistent constructor declaration on bean with name '" + beanName +
"': single autowire-marked constructor flagged as optional - " +
"this constructor is effectively required since there is no " +
"default constructor to fall back to: " + candidates.get(0));
}
}
// 如果有构造方法加Autowired注解,直接返回这些加了注解的构造方法
candidateConstructors = candidates.toArray(new Constructor<?>[0]);
}
// 没有只有一个构造方法 并且是有参的
else if (rawCandidates.length == 1 && rawCandidates[0].getParameterCount() > 0) {
candidateConstructors = new Constructor<?>[] {rawCandidates[0]};
}
else if (nonSyntheticConstructors == 2 && primaryConstructor != null &&
defaultConstructor != null && !primaryConstructor.equals(defaultConstructor)) {
candidateConstructors = new Constructor<?>[] {primaryConstructor, defaultConstructor};
}
else if (nonSyntheticConstructors == 1 && primaryConstructor != null) {
candidateConstructors = new Constructor<?>[] {primaryConstructor};
}
else {
candidateConstructors = new Constructor<?>[0];
}
this.candidateConstructorsCache.put(beanClass, candidateConstructors);
}
}
}
// 没有过滤出构造方法就返回null
return (candidateConstructors.length > 0 ? candidateConstructors : null);
}
3.2.5.2 流程图
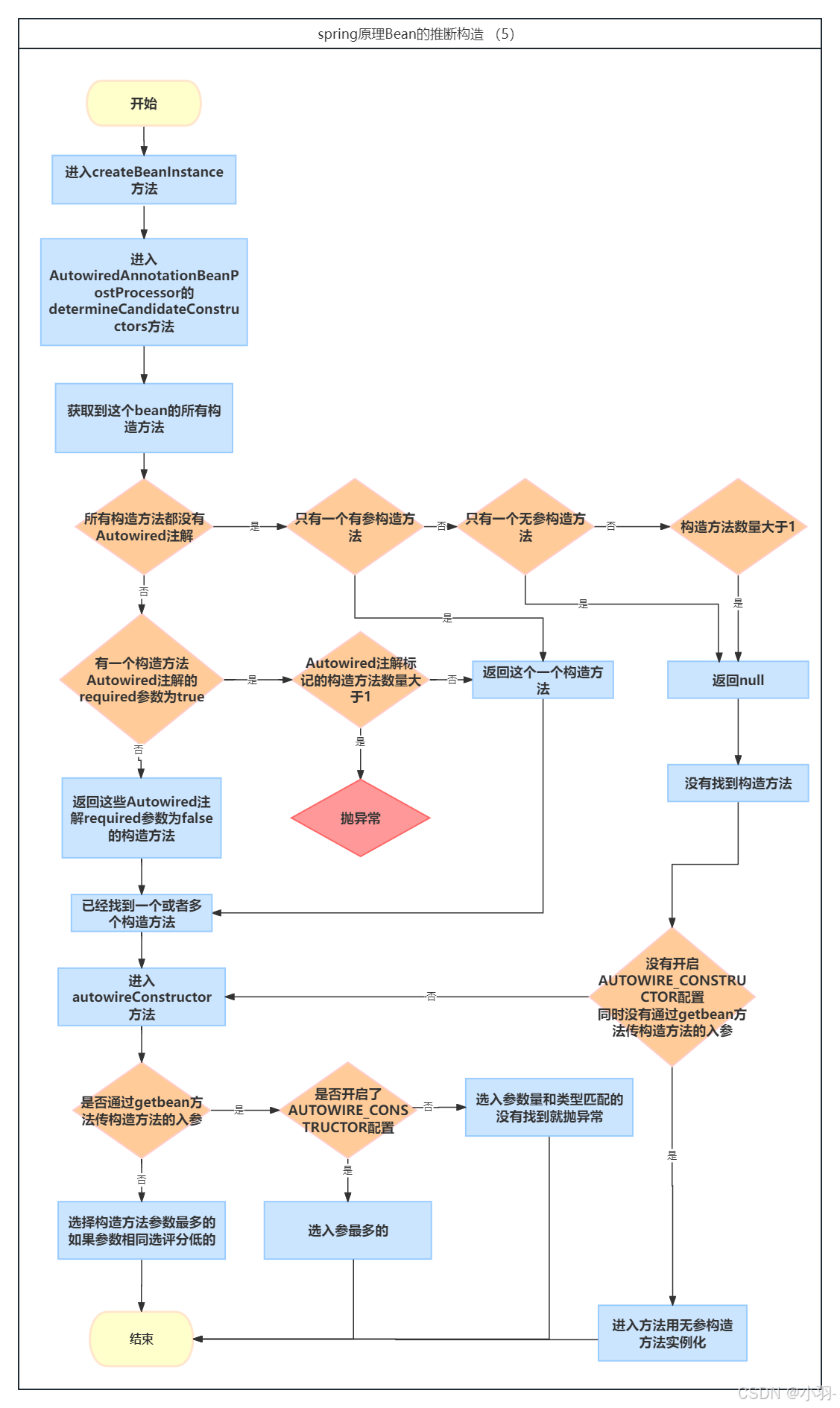
3.2.5.3 总结
先拿到这个bean所有的构造方法,如果所有构造方法都没有加autwire注解的情况下,有多个构造方法或者只有一个无参构造方法返回null,只有一个有参构造方法就过滤出这个构造方法,如果有加注解的情况,autwire注解required参数为true,那么不能有一个以上的构造方法被autwire注解标记,否则抛异常,如果参数为false,那么没有次数限制,然后筛选出这些被autwire注解标记的构造方法
如果你筛选出来的构造方法为null,同时没有开启spring自动找构造方法的配置,getbean也没有传构造方法的入参,那么直接用无参构造去实例化,
如果你筛选出了一个或者多个,首先会看getbean有没有传入参,有的话根据这个入参的数量和类型找到构造方法,没有的话抛异常,如果开启了自动找构造方法的配置,那么就选入参最多的,如果都没有,spring会从你筛选出的构造方法选参数最多的,如果参数最多的构造方法有多个,spring会对其进行评分,选评分最低的