/**
* 判断2个时间段是否有重叠(交集)
* @param startDate1 时间段1开始时间戳
* @param endDate1 时间段1结束时间戳
* @param startDate2 时间段2开始时间戳
* @param endDate2 时间段2结束时间戳
* @param isStrict 是否严格重叠,true 严格,没有任何相交或相等;false 不严格,可以首尾相等,比如2021/5/29-2021/5/31和2021/5/31-2021/6/1,不重叠。
* @return HashMap<String,DateTime> key startDate endDate
* 思路:将有交集的情况列出,若不符合有交集的情况,则无交集
* 有交集的情况
* 1.第一个时间段的开始时间在第二个时间段的开始时间和结束时间当中 第一个时间段的结束时间大于第二个时间段的结束时间
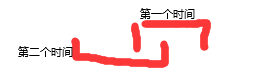
* 2.第一个时间段的结束时间在第二个时间段的开始时间和结束时间当中 第一个时间段的开始时间小于第二个时间段的开始时间
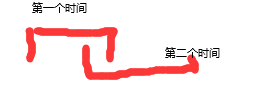
* 3.第一个时间段的开始结束时间在第二个时间段的开始时间和结束时间当中
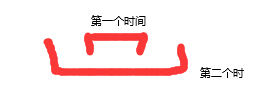
* 3.第二个时间段的开始结束时间在第一个时间段的开始时间和结束时间当中
* 判断两个时间段是否有交集
*/
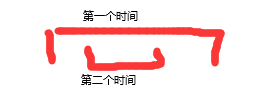
public static HashMap<String,DateTime> setOverlap(DateTime startDate1,
DateTime endDate1, DateTime startDate2, DateTime endDate2, boolean isStrict){
HashMap<String,DateTime> intersection = new HashMap<>();
if(endDate1.compareTo(startDate1) < 0){
throw new XbootException("endDate1不能小于startDate1");
}
if(endDate2.compareTo(startDate2) < 0){
throw new XbootException("endDate2不能小于startDate2");
}
if(isStrict){
if(startDate1.compareTo(startDate2) >= 0 && startDate1.compareTo(endDate2) <= 0
&& endDate1.compareTo(endDate2) > 0)
{
intersection.put("startDate",startDate1);
intersection.put("endDate",endDate2);
}else if(endDate1.compareTo(startDate2) >= 0
&& startDate1.compareTo(endDate2) <= 0
&& startDate1.compareTo(startDate2) < 0)
{
intersection.put("startDate",endDate1);
intersection.put("endDate",endDate2);
}else if(startDate1.compareTo(startDate2) >= 0
&& endDate1.compareTo(endDate2) <= 0)
{
intersection.put("startDate",startDate1);
intersection.put("endDate",endDate1);
}else if((startDate1.compareTo(startDate2) <= 0
&& endDate1.compareTo(endDate2) >= 0) ){
intersection.put("startDate",startDate2);
intersection.put("endDate",endDate2);
}
}else{
if(startDate1.compareTo(startDate2) > 0
&& startDate1.compareTo(endDate2) < 0
&& endDate1.compareTo(endDate2) > 0)
{
intersection.put("startDate",startDate1);
intersection.put("endDate",endDate2);
}else if(endDate1.compareTo(startDate2) > 0
&& startDate1.compareTo(endDate2) < 0
&& startDate1.compareTo(startDate2) < 0)
{
intersection.put("startDate",endDate1);
intersection.put("endDate",endDate2);
}else if(startDate1.compareTo(startDate2) > 0
&& endDate1.compareTo(endDate2) < 0){
intersection.put("startDate",startDate1);
intersection.put("endDate",endDate1);
}else if((startDate1.compareTo(startDate2) < 0
&& endDate1.compareTo(endDate2) > 0) ){
intersection.put("startDate",startDate2);
intersection.put("endDate",endDate2);
}
}
return intersection;
}
/**
* 判断2个时间段是否有重叠(交集)
* @param startDate1 时间段1开始时间
* @param endDate1 时间段1结束时间
* @param startDate2 时间段2开始时间
* @param endDate2 时间段2结束时间
* @param isStrict 是否严格重叠,true 严格,没有任何相交或相等;false 不严格,可以首尾相等,比如2021 *05-29到2021-05-31和2021-05-31到2021-06-01,不重叠。
* @return ashMap<String,DateTime> key startDate endDate
*/
public static HashMap<String,DateTime> getOverlap(DateTime startDate1,
DateTime endDate 1, DateTime startDate2, DateTime endDate2, boolean isStrict){
Objects.requireNonNull(startDate1, "startDate1");
Objects.requireNonNull(endDate1, "endDate1");
Objects.requireNonNull(startDate2, "startDate2");
Objects.requireNonNull(endDate2, "endDate2");
return setOverlap(startDate1, endDate1, startDate2, endDate2, isStrict);
}
根据网友建议判断部分可做修改
if(isStrict){
if(! (endDate1.getTime() <= startDate2.getTime() || startDate1.getTime() >= endDate2.getTime() )){
//存在交集 取 两个时间中最大的开始时间和最小的结束时间
intersection.put("startDate",startDate1.getTime() < startDate2.getTime() ? startDate2 : startDate1);
intersection.put("endDate",endDate1.getTime() < endDate2.getTime() ? endDate1 : endDate2);
}
}else{
if(! (endDate1.getTime() < startDate2.getTime() || startDate1.getTime() > endDate2.getTime() )){
//存在交集 取 两个时间中最大的开始时间和最小的结束时间
intersection.put("startDate",startDate1.getTime() < startDate2.getTime() ? startDate2 : startDate1);
intersection.put("endDate",endDate1.getTime() < endDate2.getTime() ? endDate1 : endDate2);
}
}