import jieba
import jieba.posseg as posseg
import re
from datetime import datetime,timedelta
from dateutil.parser import parser
# 中文词性标注和命名实体识别
sent = "中文分词是文本处理不可或缺的一步!"
seglist = posseg.cut(sent)
# print(seglist)
print(''.join(['{0}/{1}'.format(w, t) for w, t in seglist]))
# 中文/nz分词/n是/v文本处理/n不可或缺/l的/uj一步/m!/x
# 日期识别
UTIL_CN_NUM = {'零': 0, '一': 1, '二': 2, '两': 2, '三': 3, '四': 4,
'五': 5, '六': 6, '七': 7, '八': 8, '九': 9,
'0': 0, '1': 1, '2': 2, '3': 3, '4': 4,
'5': 5, '6': 6, '7': 7, '8': 8, '9': 9}
UTIL_CN_UNIT = {'十': 10, '百': 100, '千': 1000, '万': 10000}
def cn2dig(src):
if src == "":
return None
m = re.match("\\d+", src)
if m:
return int(m.group(0))
rsl = 0
unit = 1
for item in src[::-1]:
if item in UTIL_CN_UNIT.keys():
unit = UTIL_CN_UNIT[item]
elif item in UTIL_CN_NUM.keys():
num = UTIL_CN_NUM[item]
rsl += num * unit
else:
return None
if rsl < unit:
rsl += unit
return rsl
def year2dig(year):
res = ''
for item in year:
if item in UTIL_CN_NUM.keys():
res = res + str(UTIL_CN_NUM[item])
else:
res = res + item
m = re.match("\\d+"
jieba中文词性表注和CRF命名实体识别代码示例
最新推荐文章于 2024-05-23 09:51:11 发布
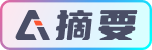