An inorder binary tree traversal can be implemented in a non-recursive way with a stack. For example, suppose that when a 6-node binary tree (with the keys numbered from 1 to 6) is traversed, the stack operations are: push(1); push(2); push(3); pop(); pop(); push(4); pop(); pop(); push(5); push(6); pop(); pop(). Then a unique binary tree (shown in Figure 1) can be generated from this sequence of operations. Your task is to give the postorder traversal sequence of this tree.
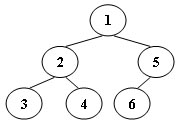
Figure 1
Input Specification:
Each input file contains one test case. For each case, the first line contains a positive integer N (≤30) which is the total number of nodes in a tree (and hence the nodes are numbered from 1 to N). Then 2N lines follow, each describes a stack operation in the format: "Push X" where X is the index of the node being pushed onto the stack; or "Pop" meaning to pop one node from the stack.
Output Specification:
For each test case, print the postorder traversal sequence of the corresponding tree in one line. A solution is guaranteed to exist. All the numbers must be separated by exactly one space, and there must be no extra space at the end of the line.
Sample Input:
6
Push 1
Push 2
Push 3
Pop
Pop
Push 4
Pop
Pop
Push 5
Push 6
Pop
Pop
Sample Output:
3 4 2 6 5 1
#include<stdio.h>
#include<stdlib.h>
#define MAXSIZE 35
typedef struct line {
int a[MAXSIZE];
int f;
int r;
}Line,*qLine;
typedef struct SNode {
int a[MAXSIZE];
int top;
}Stack,*qStack;
void Push(qStack *q, int X);
int Pop(qStack *q);
int Get(qStack q);
void pirnt(qLine q,int count);
int PopLine(qLine *q);
void PushLine(qLine *q, int X);
int main()
{
qLine line = (Line*)malloc(sizeof(Line));
line->f = 0;
line->r = 0;
qStack stack = (Stack*)malloc(sizeof(Stack));
stack->top = -1;
int count = 0,number=0,temp=0;
char a[5];
scanf("%d", &count);
int nn = count;
if (count == 1) {
scanf("%s ", a);
scanf("%d", &number);
printf("%d", number);
}
else {
while (count)
{
scanf("%s ", a);
if (a[0] == 'P'&&a[1] == 'u'&&a[2] == 's'&&a[3] == 'h') {
Pop(&stack);
scanf("%d", &number);
Push(&stack, number);
Push(&stack, -2);
Push(&stack, -2);
count--;
}
else {
do {
temp = Pop(&stack);
if (temp >=0) {
PushLine(&line, temp);
}
} while (Get(stack) != -2);
}
}
while (Get(stack) != -1) {
temp = Pop(&stack);
if (temp >= 0) {
PushLine(&line, temp);
}
}
pirnt(line,nn);
}
system("pasue");
return 0;
}
void Push(qStack *q, int X) {
if ((*q)->top < MAXSIZE - 1) {
(*q)->top++;
(*q)->a[(*q)->top] = X;
}
}
int Pop(qStack *q) {
int n = -1;
if ((*q)->top != -1) {
n = (*q)->a[(*q)->top];
(*q)->top--;
}
return n;
}
int Get(qStack q) {
int n = -1;
if (q->top != -1) {
n = q->a[q->top];
}
return n;
}
void PushLine(qLine *q,int X) {
if (((*q)->r- (*q)->f+1+MAXSIZE)%MAXSIZE!=0) {
(*q)->r++;
(*q)->a[(*q)->r] =X;
}
}
int PopLine(qLine *q) {
if ((*q)->r != (*q)->f) {
(*q)->f++;
return (*q)->a[(*q)->f];
}
return -1;
}
void pirnt(qLine q ,int count) {
if (q->r != q->f) {
while ((count-1))
{
q->f++;
printf("%d ", q->a[q->f]);
count--;
}
q->f++;
printf("%d", q->a[q->f]);
}
}