真的,看完这个就去看navigationController,哎,还有picker view啊。
UIViewController
An object that manages a view hierarchy for your UIKit app.
一个管理为你的app管理视图层次的对象。
要去看的文档:
View Controller Programming Guide for iOS(link)
Declaration
class UIViewController : UIResponder
UIViewController是继承于UIResponder的
Overview
--The UIViewController
class defines the shared behavior that is common to all view controllers. You rarely create instances of the UIViewController
class directly.
UIViewController定义了所有view controller 的公共行为,你一般很少去直接实例化UIViewController,而是子类化,然后在实例化子类。
--A view controller’s main responsibilities include the following:
一个VC 的主要有以下几个功能:
-
Updating the contents of the views, usually in response to changes to the underlying data.
负责更新视图内容,通常是为了响应底层数据的变更。
-
Responding to user interactions with views.
负责响应客户与视图的交互
-
Resizing views and managing the layout of the overall interface.
裁剪视图和管理整个用户界面的布局
-
Coordinating with other objects—including other view controllers—in your app.
协调与其他vc的行为
--A view controller is tightly bound to the views it manages and takes part in handling events in its view hierarchy.
一个vc是紧紧捆绑着它管理的view的,而且vc参与处理view 层次的事件的。
--View controllers are UIResponder
objects and are inserted into the responder chain between the view controller’s root view and that view’s superview
因为vc也是UIResponder对象,所以vc在响应链中的位置是插在自己的根视图与根视图的父视图的中间,是view的中间,不是vc的中间,vc和view要注意区分。
--If none of the view controller’s views handle an event, the view controller has the option of handling the event or passing it along to the superview.
如果没有别的vc来处理事件,那么该vc可以处理该事件,或者把事件传递给父视图,交给父视图的vc处理。
--View controllers are rarely used in isolation. Instead, you often use multiple view controllers, each of which owns a portion of your app’s user interface.
vc很少单独使用,你一般都会使用多个vc来搭建你的用户界面,每个vc管理用户界面的一部分。不一定是一个界面的一部分,也可以是所用的用户界面中的一个界面,这也只是用户界面的一部分。
--Usually, only the views from one view controller are visible at a time
通常,一个时刻只有一个vc的的视图集是可见。
--A view controller may present a different view controller to display a new set of views, or it may act as a container for other view controllers’ content and animate views however it wants.
一个vc可以展示另外一个vc的视图集,注意,是一个展示一个;或者一个vc作为其他vc的容器来展示其他vc的内容和动画。
Subclassing Notes -- 子类化注意事项
--Every app contains at least one custom subclass of UIViewController
.
一个app至少包含一个自定义的UIViewController 的子类
--For detailed information about using and implementing view controllers, see View Controller Programming Guide for iOS.
更多关于如何实现并使用一个vc,请参考超链接。这个要看。
下面的几个小节都是简要的介绍了自定义vc子类时的任务。
View Management -- 管理视图
--Each view controller manages a view hierarchy, the root view of which is stored in the view
property of this class.
每一个vc都管理了一个视图层次,而根视图就是vc类的view属性。
--The size and position of the root view is determined by the object that owns it, which is either a parent view controller or the app’s window.
根视图的大小和位置是由它的拥有者决定的,这个拥有者要么是vc,要么就是window
--The view controller that is owned by the window is the app’s root view controller and its view is sized to fill the window.
直接被window拥有的vc被称作根vc,根vc的view大小填充整个window
--View controllers load their views lazily.
vc是延迟加载它的视图的,也就是说只有首次访问该视图的属性时,vc才会创建和加载该视图出来。
--There are several ways to specify the views for a view controller:
这里有几种方式来指定vc的view
-
Specify the view controller and its views in your app’s Storyboard. To load a view controller from a storyboard, call the
instantiateViewController(withIdentifier:)
method of the appropriateUIStoryboard
object. The storyboard object creates the view controller and returns it to your code.
通过storyboard指派view的vc。你可以调用UIStoryboard的instantiateViewController方法来生成一个vc,这个vc就与storyboard中的view绑定了
-
Specify the views for a view controller using a Nib file. A nib file lets you specify the views of a single view controller but does not let you define segues or relationships between view controllers. The nib file also stores only minimal information about the view controller itself.
To initialize a view controller object using a nib file, create your view controller class programmatically and initialize it using the
init(nibName:bundle:)
method. When its views are requested, the view controller loads them from the nib file.
通过 nib文件来创建vc的view。与storyboard不同的是nib文件并不提供segue功能来绑定vc与view,nib文件只存储了vc的最小化的信息,所以你需要通过编程的方式来实例化一个vc,然后调用这个vc的init方法来加载nib文件。
-
Specify the views for a view controller using the
loadView()
method. In that method, create your view hierarchy programmatically and assign the root view of that hierarchy to the view controller’sview
property.
通过vc的loadView方法来加载view,在这个方法中以编程的方式给你的vc的view属性赋值绑定view就可以了。
--All of these techniques have the same end result, which is to create the appropriate set of views and expose them through the view
property.
上述的几种方式产生的结果都是一样的,就是给vc的view属性赋值,赋予了vc一个视图集。
--A view controller is the sole owner of its view and any subviews it creates.
一个vc只能是一个视图集的唯一拥有者。所以当你的vc释放的时候,该vc需要让出它对该视图集的拥有权。storyboard是通过复制视图集来给另外一个vc赋值的,当有需要的时候。
--A view controller’s root view is always sized to fit its assigned space. For other views in your view hierarchy, use Interface Builder to specify the Auto Layout constraints that govern how each view is positioned and sized within its superview’s bounds.For more information about how to create constraints, see Auto Layout Guide.
一个vc的根视图需要根据它被分配的空间来调整自己的大小。对于那些在你的视图层次中的视图,你可以使用“自动布局约束”来管理每一个view如何在它的父视图的边界内布局。更多关于自动布局的约束,参考超链接。
Handling View-Related Notifications -- 操纵与视图相关的通知
--When the visibility of its views changes, a view controller automatically calls its own methods so that subclasses can respond to the change.
当视图的可见性发生改变时,vc就会自动调用自身的一些方法,UIKit这样做是为了让你在使用vc子类的时候可以很好地响应这些改变。
--Figure 1 shows the possible visible states for a view controller’s views and the state transitions that can occur.Not all ‘will’ callback methods are paired with only a ‘did’ callback method.
下图展示了view的所有可能的状态,以及这些状态之间的转换。注意,并不是所有的will回调方法都只与唯一一个did的回调方法对应。所以你要确保,在一个过程开始时调用了will方法之后,在过程结束的时候必须调用相应的did方法来结束过程。
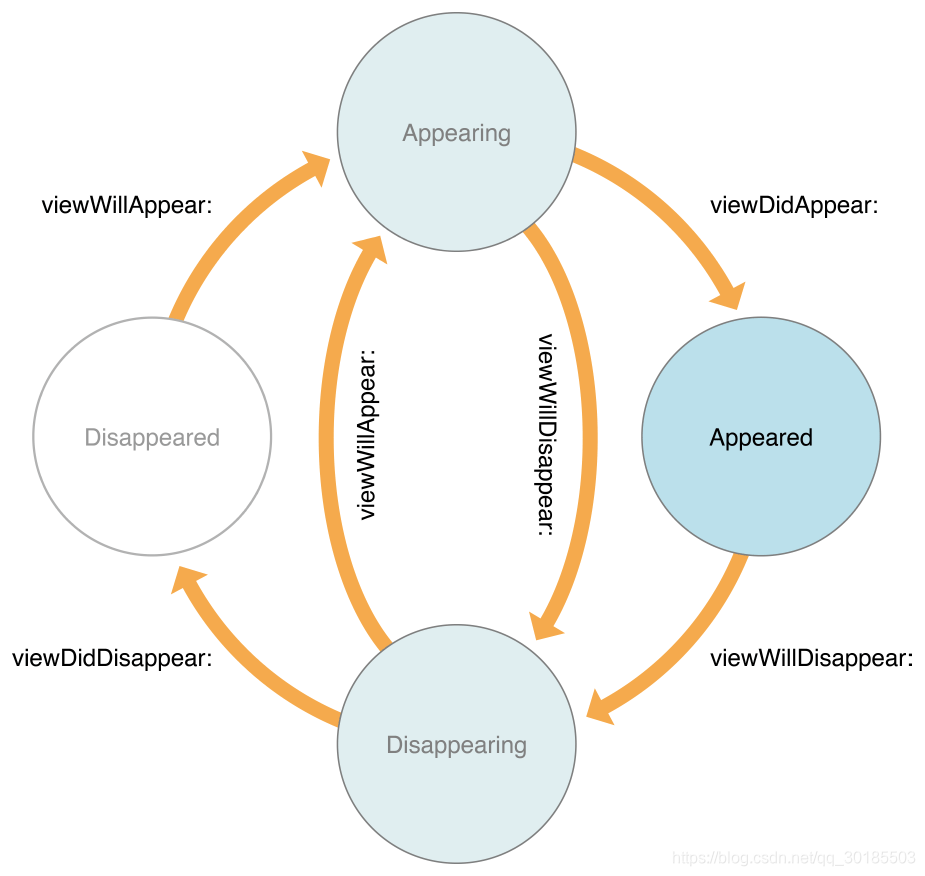
Handling View Rotations -- 操作视图的旋转
--As of iOS 8, all rotation-related methods are deprecated.Instead, rotations are treated as a change in the size of the view controller’s view and are therefore reported using the viewWillTransition(to:with:)
method.
从iOS 8 开始,所有与rotation名字相关的方法都被弃用了。苹果公司开始将旋转视为view的大小发生改变,所以与旋转相关的方法都以viewWillTransition的形式来命名了。
--When the interface orientation changes, UIKit calls this method on the window’s root view controller. That view controller then notifies its child view controllers, propagating the message throughout the view controller hierarchy.
当用户界面的方向发生改变时,UIKit会调用vc的viewWillTransition方法来通知vc的子vc,将方向的消息扩播到整个vc层次中。
--In iOS 6 and iOS 7, your app supports the interface orientations defined in your app’s Info.plist
file.
在iOS 6 和 iOS 7 中,你的app将有关方位的信息定义在Info.plist文件中。那么久远的事,知道一下就行了。
--A view controller can override the supportedInterfaceOrientations
method to limit the list of supported orientations.
vc可以通过修改supportedInterfaceOrientations
计算属性来限制方位列表中给出的方位。这个计算属性一般被根vc或者覆盖整个屏幕的vc调用
--child view controllers use the portion of the window provided for them by their parent view controller and no longer participate directly in decisions about what rotations are supported.
只使用了window部分空间的子vc不再支持直接参与视图的旋转,而是通过父vc控制子vc的view的旋转。
--The intersection of the app's orientation mask and the view controller's orientation mask is used to determine which orientations a view controller can be rotated into.
app的方位掩模与vc的方位掩模的交集,决定了vc的view可以旋转到哪些方位。掩模在图形处理上指的就是用一个模版盖住该图形的部分,被盖住的部分不能被修改。如果是用图形矩阵编码的方式修改图形的话,那么掩模也称为掩码。
--You can override the preferredInterfaceOrientationForPresentation
for a view controller that is intended to be presented full screen in a specific orientation.
你可以重写vc的 preferredInterfaceOrientationForPresentation
计算属性,来指定全屏显示时view旋转到指定的方向。
--When a rotation occurs for a visible view controller, the willRotate(to:duration:)
, willAnimateRotation(to:duration:)
, and didRotate(from:)
methods are called during the rotation.
当可见的vc的view发生旋转时,旋转期间,UIKit会自动调用vc的he willRotate(to:duration:)
, willAnimateRotation(to:duration:)
, 和 didRotate(from:)
方法。所以你又可以复写这些方法来操作旋转了。哦,这是旧版的叫法,新版的方法名叫viewWillTransiton了。
--The viewWillLayoutSubviews()
method is also called after the view is resized and positioned by its parent.
当子视图被父视图调整大小方向的时候,vc的viewWillLayoutSubviews方法也会被vc自动调用
--If a view controller is not visible when an orientation change occurs, then the rotation methods are never called.
如果当前vc不可见,当是它的视图方位发生改变时,那些旋转相关的方法不会被自动调用
--However, the viewWillLayoutSubviews()
method is called when the view becomes visible.
除了方位发生改变的情况外,当视图变成可见的时候,vc也会自动调用viewWillLayoutSubviews方法。
--Your implementation of this method can call the statusBarOrientation
method to determine the device orientation.
你可以修改vc的statusBarOrientation计算属性,来确定在调用viewWillLayoutSubviews方法时此时设备的方向。
--At launch time, apps should always set up their interface in a portrait orientation. After the application(_:didFinishLaunchingWithOptions:)
method returns, the app uses the view controller rotation mechanism described above to rotate the views to the appropriate orientation prior to showing the window.
在app启动期间,app总是把用户界面设置为竖直方向。只有在application(_:didFinishLaunchingWithOptions:)
方法返回之后,app才会在window展示之前使用vc的方向机制。
Implementing a Container View Controller -- 实现一个vc容器
--A container view controller manages the presentation of content of other view controllers it owns, also known as its child view controllers.A child's view can be presented as-is or in conjunction with views owned by the container view controller.
vc自身也可以作为一个vc容器,vc容器中的vc也称为子vc。一个子vc的视图可以原样展示,也可以与容器vc中的其他vc的视图一起展示。
--Your container view controller subclass should declare a public interface to associate its children. The nature of these methods is up to you and depends on the semantics of the container you are creating.
你定义的容器vc应该要提供一个公共的接口来联系它的子vc。接口里的那些方法性质取决于你,也取决于容器vc的语义
--You need to decide how many children can be displayed by your view controller at once, when those children are displayed, and where they appear in your view controller's view hierarchy.
你需要确定一次可以展示多少个子vc,这些子vc什么时候展示,以及在你的vc层次中的哪一层展示。
--Your view controller class defines what relationships, if any, are shared by the children.
有的话,你的vc需要定义子vc间的共享关系。
--By establishing a clean public interface for your container, you ensure that children use its capabilities logically, without accessing too many private details about how your container implements the behavior.
为了创建一个干净的容器接口,你需要确保子vc只是使用了容器逻辑上的功能,而不能访问容器实现这些功能行为的细节。
--Your container view controller must associate a child view controller with itself before adding the child's root view to the view hierarchy.
在将子vc的根视图添加到视图层次中之前,你的子vc必须先与容器关联。
--Likewise, after it removes a child's root view from its view hierarchy, it should disconnect that child view controller from itself.
同样,当从视图层次中移除子vc的根视图时,也必须把子vc与容器vc解除关联
--To make or break these associations, your container calls specific methods defined by the base class.they are to be used only by your container's implementation to provide the expected containment behavior.
vc的基类定义了解除和建立容器vc与子vc关联的方法,这些方法只能由宿主容器vc调用,不能被客户端容器vc调用。这些方法仅仅用来提供 在容器vc中的 你预期的 包含关系。方法如下:
Here are the essential methods you might need to call:
--You are not required to override any methods when creating a container view controller.
在创建一个容器vc时,你没有权利来复写上述这些方法。
--By default, rotation and appearance callbacks are automatically forwarded to children. You may optionally override the shouldAutomaticallyForwardRotationMethods()
and shouldAutomaticallyForwardAppearanceMethods
methods to take control of this behavior yourself.
默认的,旋转和外观回调会自动地发送给子vc,所以你可以在子vc中复写shouldAutomaticallyForwardRotationMethods()
和 shouldAutomaticallyForwardAppearanceMethods
方法来控制视图的旋转和回调行为。
Memory Management -- 内存管理
--Memory is a critical resource in iOS, and view controllers provide built-in support for reducing their memory footprint at critical times. The UIViewController
class provides some automatic handling of low-memory conditions through its didReceiveMemoryWarning()
method, which releases unneeded memory.
内存是iOS中的关键资源,释放内存是iOS内置支持的,你不能操作。你只能通过调用vc的didReceiveMemoryWarning()
方法来通知vc释放内存,然后就没有然后了,你只能做这么多。
State Preservation and Restoration -- 状态保存与恢复
--If you assign a value to the view controller's restorationIdentifier
property, the system may ask the view controller to encode itself when the app transitions to the background.
如果你个vc的restorationIdentifier
属性赋值的话,当app进入后台时,系统就会请求vc编码自身。肯定是为了保存和恢复app 的状态啊。
--Each child you encode must have a unique restoration identifier.
状态的储存和恢复都是需要每一个vc都有自身的唯一恢复标识符的。
--To see an example of state preservation and restoration, see Restoring Your App’s State.
关于如何储存和恢复vc的状态,参考超链接的例子
Topics --专题
Creating a View Controller --创建一个vc
init(nibName: String?, bundle: Bundle?)
//通过指定的bundle中的nib文件创建vc
--Returns a newly initialized view controller with the nib file in the specified bundle.
init?(coder: NSCoder)
//显式继承必要初始化器
Getting the Storyboard and Nib Information --获取storyboard和nib文件的信息
var storyboard: UIStoryboard?
//get属性:获取该vc来源的storyboard
--The storyboard from which the view controller originated.
var nibName: String?
//get属性:获取该vc来源的nib文件的名字
--The name of the view controller's nib file, if one was specified.
var nibBundle: Bundle?
//get属性:获取该vc来源的nib文件的包(句柄)
--The view controller's nib bundle if it exists.
Managing the View --管理vc中的view
var view: UIView!
//属性:vc的根view,访问即加载
--The view that the controller manages.
var viewIfLoaded: UIView?
//get属性:返回已加载进内存的根view或者nil
--The view controller’s view, or nil
if the view is not yet loaded.
var isViewLoaded: Bool
//get属性:判断根view是否已经加载进内存
--A Boolean value indicating whether the view is currently loaded into memory.
func loadView()
//方法:当vc发现缺少根view时,自动调用该方法创建,你可复写
--Creates the view that the controller manages.
func viewDidLoad()
//方法:当view已经完成加载进内存时,vc自动调用该方法
Called after the controller's view is loaded into memory.
func loadViewIfNeeded()
//方法:你调用该方法来促使vc加载还没在内存中的view
Loads the view controller’s view if it has not yet been loaded.
var title: String?
//属性:设置根view的名字,优先级高于导航栏的名字
A localized string that represents the view this controller manages.
var preferredContentSize: CGSize
The preferred size for the view controller’s view.
//属性:根view在弹窗中时的首选size
Responding to View-Related Events --响应与根view相关的事件
func viewWillAppear(Bool)
//方法:当根view将要被添加到视图层次中时,自动调用,复写时参数可设置动画
Notifies the view controller that its view is about to be added to a view hierarchy.
For more information about the how views are added to view hierarchies by a view controller, and the sequence of messages that occur, see Supporting Accessibility.
--更多关于添加进视图层次的次序知识,参考超链接
func viewDidAppear(Bool)
//方法:当根vc已经添加到视图层次中时,自动调用该方法,可复写
Notifies the view controller that its view was added to a view hierarchy.
func viewWillDisappear(Bool)
//方法:当根view将要从视图层次中移除时,自动调用该方法,可复写
Notifies the view controller that its view is about to be removed from a view hierarchy.
func viewDidDisappear(Bool)
//方法:当根view已经从视图层次中移除时,自动调用该方法,可复写
Notifies the view controller that its view was removed from a view hierarchy.
var isBeingDismissed: Bool
//get属性:获知vc是否将要被解除
A Boolean value indicating whether the view controller is being dismissed.
var isBeingPresented: Bool
//get属性:获知vc是否将要被呈现
A Boolean value indicating whether the view controller is being presented.
var isMovingFromParent: Bool
//get属性:获知vc是否将要从父vc中移除
A Boolean value indicating whether the view controller is being removed from a parent view controller.
var isMovingToParent: Bool
//get属性:获知vc是否将要移进父vc中
A Boolean value indicating whether the view controller is being moved to a parent view controller.
Extending the View's Safe Area --拓展根view的安全区域
Positioning Content Relative to the Safe Area
//★文章:定位相对于安全区域的视图内容,里面有关于安全区域的介绍
Position views so that they are not obstructed by other content.
--定位view,以至于他们不会被其他的视图内容遮住。
var additionalSafeAreaInsets: UIEdgeInsets
//属性:你可以自定义安全区域的内边距
Custom insets that you specify to modify the view controller's safe area.
func viewSafeAreaInsetsDidChange()
//方法:自动调用,当根view的安全区域的内边距发生改变时
Called to notify the view controller that the safe area insets of its root view changed.
Managing the View's Margins --管理根view的页边距
Positioning Content Within Layout Margins
//★文章:定位视图内容的布局的页边距,里面有关于页边距的介绍
Position views so that they are not crowded by other content.
var viewRespectsSystemMinimumLayoutMargins: Bool
//属性:设置是否遵循系统的最小页边距的阈值
A Boolean value indicating whether the view controller's view uses the system-defined minimum layout margins.
var systemMinimumLayoutMargins: NSDirectionalEdgeInsets
//属性:设置根view页边距的最小阈值
The minimum layout margins for the view controller's root view.
func viewLayoutMarginsDidChange()
//方法:自动调用,当根view的页边距已经发生改变时
Called to notify the view controller that the layout margins of its root view changed.
Configuring the View’s Layout Behavior --配置根view的布局行为
var edgesForExtendedLayout: UIRectEdge
//属性:iOS 10之前使用,现在用安全区域来定义视图边界的遮掩问题
The edges that you extend for your view controller.
struct UIRectEdge
//常量结构体:view矩形edgesForExtendedLayout
的边界值的类型
Constants that specify the edges of a rectangle.
var extendedLayoutIncludesOpaqueBars: Bool
//属性:断定扩展布局是否包含不透明条
A Boolean value indicating whether or not the extended layout includes opaque bars.
func viewWillLayoutSubviews()
//方法:自动调用,当子 view将要布局时(即view的bounds值将发生改变),可复写
Called to notify the view controller that its view is about to layout its subviews.
func viewDidLayoutSubviews()
//方法:自动调用,当子 view刚完成布局时,可复写
Called to notify the view controller that its view has just laid out its subviews.
func updateViewConstraints()
//方法:自动调用,当根view的布局约束需要发生变化时。
Called when the view controller's view needs to update its constraints.
Configuring the View Rotation Settings --配置view的旋转设置
var shouldAutorotate: Bool
//get属性:获知vc的视图内容是否自动旋转
Returns a Boolean value indicating whether the view controller's contents should auto rotate.
var supportedInterfaceOrientations: UIInterfaceOrientationMask
//get属性:获知vc支持的界面方位
Returns all of the interface orientations that the view controller supports.
var preferredInterfaceOrientationForPresentation: UIInterfaceOrientation
//get属性:view全屏时,偏好的界面方位,可复写
Returns the interface orientation to use when presenting the view controller.
class func attemptRotationToDeviceOrientation()
//方法:调用该方法改变界面方位
Attempts to rotate all windows to the orientation of the device.
Performing Segues --执行Segues (切入)
func shouldPerformSegue(withIdentifier: String, sender: Any?) -> Bool
//方法:供子类复写,决定是否执行segues,默认是所有都执行
Determines whether the segue with the specified identifier should be performed.
func prepare(for: UIStoryboardSegue, sender: Any?)
//方法:segues将要执行时自动调用,可复写
Notifies the view controller that a segue is about to be performed.
func performSegue(withIdentifier: String, sender: Any?)
//方法:手工初始化segue,虽然默认自动初始化了
Initiates the segue with the specified identifier from the current view controller's storyboard file.
func allowedChildrenForUnwinding(from: UIStoryboardUnwindSegueSource) -> [UIViewController]
//方法:UIKit在搜索unwind segue的目的地时,自动调用该方法,可复写来重置UIKit搜索segue目的地的次序。
Returns an array of child view controllers to search for an unwind segue destination.
func childContaining(UIStoryboardUnwindSegueSource) -> UIViewController?
//方法:返回指定unwind segue的源vc,一般时vc容器调用该方法
Returns the child view controller that contains the source of the unwind segue.
func canPerformUnwindSegueAction(Selector, from: UIViewController, sender: Any?) -> Bool
//方法:断定当前vc是否可以响应指定vc中的segue的action方法
Called on a view controller to determine whether it responds to an unwind action.
func unwind(for: UIStoryboardSegue, towards: UIViewController)
//方法:自动调用,当unwind segue执行从源vc切入到目标vc时
Called when an unwind segue transitions to a new view controller.
Presenting a View Controller --显示一个vc
func show(UIViewController, sender: Any?)
//方法:在主上下文环境中显示vc,一般是vc容器复写该方法,可自定义
Presents a view controller in a primary context.
func showDetailViewController(UIViewController, sender: Any?)
//方法:在第二上下文环境中显示vc
Presents a view controller in a secondary (or detail) context.
func present(UIViewController, animated: Bool, completion: (() -> Void)?)
//方法:以模态的方式显示vc
Presents a view controller modally.
func dismiss(animated: Bool, completion: (() -> Void)?)
//方法:解除模态显示的vc,所有该vc以上的vc都被解除
Dismisses the view controller that was presented modally by the view controller.
var modalPresentationStyle: UIModalPresentationStyle
//属性:设置vc模态显示时的种类
The presentation style for modally presented view controllers.
enum UIModalPresentationStyle
//枚举类型:模态显示的种类的值
Modal presentation styles available when presenting view controllers.
var modalTransitionStyle: UIModalTransitionStyle
//属性:设置过渡模态显示时的种类
The transition style to use when presenting the view controller.
enum UIModalTransitionStyle
//枚举类型:模态过渡的种类的值
Transition styles available when presenting view controllers.
var isModalInPresentation: Bool
//属性:设置vc是否模态显示
A Boolean value indicating whether the view controller enforces a modal behavior.
var definesPresentationContext: Bool
//属性:设置view是否被其他vc的view覆盖
A Boolean value that indicates whether this view controller's view is covered when the view controller or one of its descendants presents a view controller.
var providesPresentationContextTransitionStyle: Bool
//属性:设置vc是否为其他vc指定过渡显示的样式
A Boolean value that indicates whether the view controller specifies the transition style for view controllers it presents.
var disablesAutomaticKeyboardDismissal: Bool
//get属性:获知控件改变时是否可以取消输入视图(键盘),子类可复写,常用于模态显示时,取消键盘的显示。
Returns a Boolean indicating whether the current input view is dismissed automatically when changing controls.
class let showDetailTargetDidChangeNotification: NSNotification.Name
//静态属性:通知,当折叠vc发生折叠展开时,发布该通知。
Posted when a split view controller is expanded or collapsed.
Adding a Custom Transition or Presentation --添加自定义动画过渡或者显示
var transitioningDelegate: UIViewControllerTransitioningDelegate?
//属性:代理对象,用于代理动画制作器,交互控制器,自定义显示控制器,用于模态的过渡。
The delegate object that provides transition animator, interactive controller, and custom presentation controller objects.
var transitionCoordinator: UIViewControllerTransitionCoordinator?
//get属性:返回vc被显示或者被取消时的过渡调节器对象
Returns the active transition coordinator object.
func targetViewController(forAction: Selector, sender: Any?) -> UIViewController?
//方法:返回参数中 动作的拥有者 vc,会在vc层次中寻找。
Returns the view controller that responds to the action.
var presentationController: UIPresentationController?
//get属性:如果当前vc是被呈现vc所管理,返回呈现vc
The presentation controller that’s managing the current view controller.
var popoverPresentationController: UIPopoverPresentationController?
//get属性:如果当前vc是被弹窗呈现vc所管理,返回弹窗呈现vc
The nearest popover presentation controller that is managing the current view controller.
var restoresFocusAfterTransition: Bool
//属性:断定vc的某item是否保留vc再现时的焦点
A Boolean value that indicates whether an item that previously was focused should again become focused when the item's view controller becomes visible and focusable.
Adapting to Environment Changes --适应手机环境的改变
func collapseSecondaryViewController(UIViewController, for: UISplitViewController)
//方法:当spilt vc过渡为紧凑型宽度时,自动调用该方法
Called when a split view controller transitions to a compact-width size class.
func separateSecondaryViewController(for: UISplitViewController) -> UIViewController?
//方法:当spilt vc过渡为常规型宽度时,自动调用该方法
Called when a split view controller transitions to a regular-width size class.
Adjusting the Interface Style --调整界面的风格
var overrideUserInterfaceStyle: UIUserInterfaceStyle
//属性:设置用户自定义的界面风格
The user interface style adopted by the view controller and all of its children.
var preferredUserInterfaceStyle: UIUserInterfaceStyle
//get属性:tvOS的偏好风格设置
The preferred interface style for this view controller.
var childViewControllerForUserInterfaceStyle: UIViewController?
//get属性:tvOS的风格设置
The child view controller that supports the preferred user interface style.
func setNeedsUserInterfaceAppearanceUpdate()
//方法:tvOS的
Notifies the view controller that a change occurred that might affect the preferred interface style.
enum UIUserInterfaceStyle
//枚举类型:界面风格的选值
Constants indicating the interface style for the app.
Managing Child View Controllers in a Custom Container --在自定义vc容器中管理子vc
var children: [UIViewController]
//get属性:获取当前vc的子vc数组
An array of view controllers that are children of the current view controller.
func addChild(UIViewController)
//方法:往当前vc中添加子vc
Adds the specified view controller as a child of the current view controller.
func removeFromParent()
//方法:将自己从父vc中移除
Removes the view controller from its parent.
//方法:在自己的子vc中进行过渡显示,前一个vc会从view层中被移除
Transitions between two of the view controller's child view controllers.
var shouldAutomaticallyForwardAppearanceMethods: Bool
//get属性:外观相关方法的回调是否自动转发给子vc,默认true
Returns a Boolean value indicating whether appearance methods are forwarded to child view controllers.
func beginAppearanceTransition(Bool, animated: Bool)
//方法:你可调用该方法告知子vc,它的外观将要发生改变
Tells a child controller its appearance is about to change.
func endAppearanceTransition()
//方法:你可调用该方法告知子vc,它的外观已经完成改变
Tells a child controller its appearance has changed.
func setOverrideTraitCollection(UITraitCollection?, forChild: UIViewController)
//方法:你可调用该方法修改指定子vc的特征
Changes the traits assigned to the specified child view controller.
func overrideTraitCollection(forChild: UIViewController) -> UITraitCollection?
//方法:获取指定子vc的特征的集合
Retrieves the trait collection for a child view controller.
class let hierarchyInconsistencyException: NSExceptionName
//静态常量:如果vc的层次结构和view的层次结构不一致,则抛出该异常
Raised if the view controller hierarchy is inconsistent with the view hierarchy.
Responding to Containment Events --响应包含事件
func willMove(toParent: UIViewController?)
//方法:当自己将要从容器vc中添加或移除时,自动调用
Called just before the view controller is added or removed from a container view controller.
func didMove(toParent: UIViewController?)
//方法:当自己将要完成从容器vc中添加或移除时,自动调用
Called after the view controller is added or removed from a container view controller.
Getting Other Related View Controllers --获取其他相关的vc
var presentingViewController: UIViewController?
//get属性:展示当前vc的vc
The view controller that presented this view controller.
var presentedViewController: UIViewController?
//get属性:被当前vc展示的vc(或其父vc)
The view controller that is presented by this view controller, or one of its ancestors in the view controller hierarchy.
var parent: UIViewController?
//get属性:返回父vc(容器vc)
The parent view controller of the recipient.
var splitViewController: UISplitViewController?
//get属性:返回最接近的spilt vc
The nearest ancestor in the view controller hierarchy that is a split view controller.
var navigationController: UINavigationController?
//get属性:返回最接近的导航vc
The nearest ancestor in the view controller hierarchy that is a navigation controller.
var tabBarController: UITabBarController?
//get属性:返回最接近的标签栏vc
The nearest ancestor in the view controller hierarchy that is a tab bar controller.
Configuring a Navigation Interface --配置导航界面
var navigationItem: UINavigationItem
//get属性:获取父vc的导航栏的导航item
The navigation item used to represent the view controller in a parent's navigation bar.
var hidesBottomBarWhenPushed: Bool
//属性:设置屏幕底部的工具栏是否显示
A Boolean value indicating whether the toolbar at the bottom of the screen is hidden when the view controller is pushed on to a navigation controller.
func setToolbarItems([UIBarButtonItem]?, animated: Bool)
//方法:调用该方法设置底部工具栏的item
Sets the toolbar items to be displayed along with the view controller.
var toolbarItems: [UIBarButtonItem]?
//数组属性:设置屏幕底部工具栏的item(选项卡栏)
The toolbar items associated with the view controller.
Configuring Tab Bar Content --配置选项卡栏vc的内容(标签栏)
var tabBarItem: UITabBarItem!
//属性:设置选项卡栏中的item,该也是一个vc
The tab bar item that represents the view controller when added to a tab bar controller.
var tabBarObservedScrollView: UIScrollView?
//属性:设置标签栏为滚动视图(内建)
The full-screen scroll view to synchronize with a scrolling tab bar.
Supporting App Extensions --app外设支持
var extensionContext: NSExtensionContext?
//get属性:获取外设的上下文
Returns the extension context of the view controller.
Coordinating with System Gestures --配合系统手势
var preferredScreenEdgesDeferringSystemGestures: UIRectEdge
//get属性:获取屏幕边缘手势,子类可复写
The screen edges for which you want your gestures to take precedence over the system gestures
var childForScreenEdgesDeferringSystemGestures: UIViewController?
//get属性:查询子vc的手势是否优先于系统的默认手势,可复写
Returns the child view controller that should be queried to see if its gestures should take precedence.
func setNeedsUpdateOfScreenEdgesDeferringSystemGestures()
//方法:调用该方法,则UIKit会请求你定义的屏幕边缘手势,而非系统默认的屏幕边缘手势
Call this method when you change the screen edges that you use for deferring system gestures.
var prefersHomeIndicatorAutoHidden: Bool
//get属性:是否隐藏home指示器,iphone10之后的home键。子类可复写
Returns a Boolean indicating whether the system is allowed to hide the visual indicator for returning to the Home screen.
var childForHomeIndicatorAutoHidden: UIViewController?
//get属性:隐藏home指示器后的首选子vc,配合上一个属性使用
Returns the child view controller that is consulted about its preference for displaying a visual indicator for returning to the Home screen.
func setNeedsUpdateOfHomeIndicatorAutoHidden()
//方法:调用该方法告知UIkit你隐藏home指示器后的首选项
Notifies UIKit that your view controller updated its preference regarding the visual indicator for returning to the Home screen.
Managing the Status Bar --管理状态栏
var childForStatusBarHidden: UIViewController?
//get属性:自动调用,当系统需要隐藏/显示状态栏时,配合下面的方法一起使用(跟随子vc的设定),返回nil则不跟随,可复写
Called when the system needs the view controller to use for determining status bar hidden/unhidden state.
var childForStatusBarStyle: UIViewController?
//get属性:返回子vc,用于跟随该子vc的状态栏风格,返回nil则不跟随,可复写
Called when the system needs the view controller to use for determining status bar style.
var preferredStatusBarStyle: UIStatusBarStyle
//get属性:自己偏好的状态栏风格,可复写
The preferred status bar style for the view controller.
var prefersStatusBarHidden: Bool
//get属性:获知是否显示偏好的状态栏风格
Specifies whether the view controller prefers the status bar to be hidden or shown.
var modalPresentationCapturesStatusBarAppearance: Bool
//属性:设置非全屏vc是否夺取显示vc的状态栏控制权
Specifies whether a view controller, presented non-fullscreen, takes over control of status bar appearance from the presenting view controller.
var preferredStatusBarUpdateAnimation: UIStatusBarAnimation
//get属性:获取状态栏显示的动画效果,可复写
Specifies the animation style to use for hiding and showing the status bar for the view controller.
func setNeedsStatusBarAppearanceUpdate()
//方法:调用该方法告知UIKit,状态栏的属性发生了改变,可以在该方法的尾随闭包中设置动画效果
Indicates to the system that the view controller status bar attributes have changed.
Accessing the Available Key Commands --访问可用的键盘快捷键命令
var performsActionsWhilePresentingModally: Bool
//get属性:获知当前vc是否处理Info.plist里的动作方法,可复写
A Boolean value indicating whether the view controller performs menu-related actions.
func addKeyCommand(UIKeyCommand)
//方法:为当前vc添加键盘快捷键命令
Associates the specified keyboard shortcut with the view controller.
func removeKeyCommand(UIKeyCommand)
//方法:为当前vc移除键盘快捷键命令
Removes the key command from the view controller.
Adding Editing Behaviors to Your View Controller --为vc添加可编辑行为
var isEditing: Bool
//属性:设置用户是否可以编辑当前vc的视图内容
A Boolean value indicating whether the view controller currently allows the user to edit the view contents.
func setEditing(Bool, animated: Bool)
//方法:设置vc是否显示可编辑视图,供子类复写
Sets whether the view controller shows an editable view.
var editButtonItem: UIBarButtonItem
//get属性:获取可编辑按钮,可复写
Returns a bar button item that toggles its title and associated state between Edit and Done.
Handling Memory Warnings --处理内存警告
func didReceiveMemoryWarning()
//方法:自动调用,用于告知内存过低
Sent to the view controller when the app receives a memory warning.
Managing State Restoration --管理vc的状态恢复机制
Restoring Your App’s State //★文章和示例:保存客户的当前活动信息
Preserve and restore information related to the user’s current activities.
var restorationIdentifier: String?
//属性:设置vc的状态恢复标识符
The identifier that determines whether the view controller supports state restoration.
var restorationClass: UIViewControllerRestoration.Type?
//属性:设置重建该vc的类
The class responsible for recreating this view controller when restoring the app's state.
func encodeRestorableState(with: NSCoder)
//方法:供子类复写,编码vc的状态信息
Encodes state-related information for the view controller.
func decodeRestorableState(with: NSCoder)
//方法:供子类复写,解码vc的状态信息时,自动调用
Decodes and restores state-related information for the view controller.
func applicationFinishedRestoringState()
//方法:当其他对象的状态信息已经解码完成时(vc其后),自动调用
Called on restored view controllers after other object decoding is complete.
Supporting Swift Playgrounds --对swift playground的支持
var playgroundLiveViewRepresentation: PlaygroundLiveViewRepresentation
//get属性
Deprecated --过时了的一些特性
Deprecated Symbols //文章:过时了的特性
Instance Properties --实例属性
var childViewControllerForTouchBar: UIViewController?
Instance Methods --实例方法
Relationships --关系
Inherits From --继承关系
Conforms To --遵循的协议
See Also --参考文档
Content View Controllers --内容视图控制器
Displaying and Managing Views with a View Controller //★文章:通过vc管理view
Build a view controller in storyboards, configure it with custom views, and fill those views with your app’s data.
Showing and Hiding View Controllers //★文章:展示或隐藏vc的技术,vc之间传递数据
Display view controllers using different techniques, and pass data between them during transitions.
class UITableViewController
//参考的类:列表vc类
A view controller that specializes in managing a table view.
class UICollectionViewController
//参考的类:集合vc类
A view controller that specializes in managing a collection view.
protocol UIContentContainer
//参考的协议:视图内容容器协议
A set of methods for adapting the contents of your view controllers to size and trait changes.
UIViewController使用总结
好多好多属性和好多好多方法,这里只介绍我用到的和参考链接里面的属性和方法
参考链接:https://www.jianshu.com/p/d19c4e5b6bee
视图控制器的生命周期:
alloc
创建一个视图控制器对象,并分配内存空间。
init()
对视图控制器对象进行初始化。
loadView
如果从storyboard创建视图,则从storyboard中加载视图。
viewDidLoad
视图加入完成,可以进行一些自定义操作
viewWillAppear
视图即将要展示在屏幕上。
viewDidAppear
视图已经站在屏幕上显示并完成渲染。
viewWillLayoutSubviews
视图即将布局其子视图
viewDidLayoutSubviews
视图已经完成子视图的布局
viewWillDisappear
视图即将从屏幕中消失
viewDidDisappear
视图已经从屏幕上消失
dealloc
视图被销毁
好吧,文章里好像也没什么说的。用着再说吧。