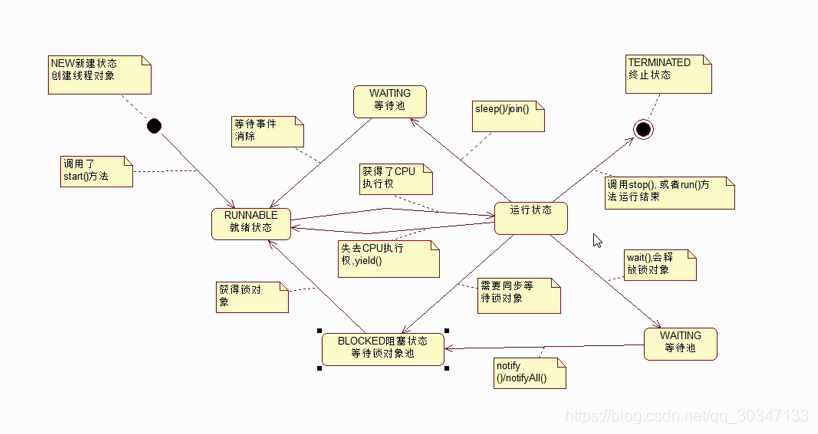
package com.bjpowernode.demo01;
import java.util.LinkedList;
public class MyStorage {
LinkedList<Object> list = new LinkedList<>();
private static final int MAX_CAPACITY = 100;
public synchronized void store() {
while ( list.size() >= MAX_CAPACITY) {
try {
this.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println( Thread.currentThread().getName() + "存储产品 前, 仓库容量:" + list.size());
for( int i =1; i <= 10;i++){
list.offer(new Object());
}
System.out.println( Thread.currentThread().getName() + "存了10个产品后, 仓库容量:" + list.size());
this.notify();
}
public synchronized void get() {
while ( list.size() <= 0) {
try {
this.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println( Thread.currentThread().getName() + "消费 前, 仓库容量:" + list.size());
for( int i =1; i <= 10;i++){
list.poll();
}
System.out.println( Thread.currentThread().getName() + "消费了10个产品 后, 仓库容量:" + list.size());
this.notifyAll();
}
}
package com.bjpowernode.demo01;
public class Producer extends Thread {
MyStorage storage;
public Producer(MyStorage storage) {
super();
this.storage = storage;
}
@Override
public void run() {
for(int d = 1; d <= 200; d++){
storage.store();
}
}
}
package com.bjpowernode.demo01;
public class Consumer extends Thread {
MyStorage storage;
public Consumer(MyStorage storage) {
super();
this.storage = storage;
}
@Override
public void run() {
for(int d = 1; d <= 200; d++){
storage.get();
}
}
}
package com.bjpowernode.demo01;
public class Test01 {
public static void main(String[] args) {
MyStorage storage = new MyStorage();
Producer producer = new Producer(storage);
Consumer consumer = new Consumer(storage);
producer.setName("生产者");
consumer.setName("销售人员");
producer.start();
consumer.start();
}
}