thymeleaf完整代码案例
1.導入依賴
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>ognl</groupId>
<artifactId>ognl</artifactId>
<version>3.1.12</version>
</dependency>
2.添加配置
thymeleaf:
servlet:
content-type: text/html;
suffix: .html
prefix: classpath:/templates/
encoding: UTF-8
mode: HTML
cache: false
3.工具類
package com.github.util;
import org.thymeleaf.TemplateEngine;
import org.thymeleaf.context.Context;
import org.thymeleaf.templateresolver.ClassLoaderTemplateResolver;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
public class TemplateUtil {
public static void template(String templateName,Context context, File file) throws IOException {
//创建模版加载器
ClassLoaderTemplateResolver resolver = new ClassLoaderTemplateResolver();
//模板文件的所在目录
resolver.setPrefix("templates/");
//模板文件后缀
resolver.setSuffix(".html");
//创建模板引擎
TemplateEngine templateEngine = new TemplateEngine();
//将加载器放入模板引擎
templateEngine.setTemplateResolver(resolver);
//创建字符输出流并且自定义输出文件的位置和文件名
FileWriter writer = new FileWriter(file);
//创建静态文件,"text"是模板html名字
templateEngine.process(templateName, context, writer);
}
}
4.測試類
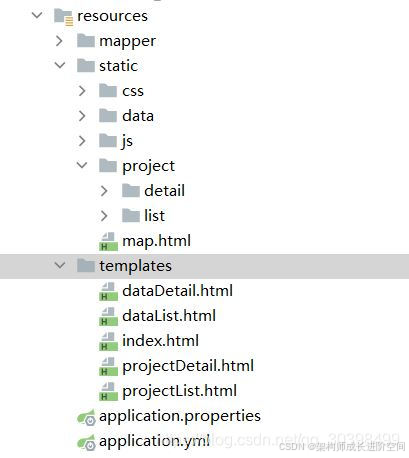
package com.github.controller;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.geidco.dcp.pojo.BaseProjectView;
import com.geidco.dcp.service.BaseProjectViewService;
import com.geidco.dcp.util.TemplateUtil;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import org.thymeleaf.context.Context;
import java.io.File;
import java.io.IOException;
import java.util.List;
import java.util.Map;
@RequestMapping("/project")
@Controller
public class ProjectController {
@Autowired
private BaseProjectViewService baseProjectViewService;
@Value("${filepath}")
String filepath;
private void generateTemplateDetail2() {
int current = 1;
int pageSize = 20;
int totalCount = baseProjectViewService.count();
int totalPage = totalCount / pageSize;
if (totalPage * pageSize < totalCount) {
totalPage += 1;
}
for (int i = 1; i <= totalPage; i++) {
List<BaseProjectView> list = baseProjectViewService.getProjectInfo((current - 1) * pageSize, current * pageSize);
for (BaseProjectView baseProjectView : list) {
//创建Context对象(存放Model)
Context context = new Context();
//放入数据
context.setVariable("name", baseProjectView.getProjectName());
context.setVariable("date", baseProjectView.getSetUpDate());
context.setVariable("desc", baseProjectView.getProjectDescription());
context.setVariable("continent", baseProjectView.getContinent());
context.setVariable("banner", baseProjectView.getBannerOne());
context.setVariable("engType", baseProjectView.getEngType());
context.setVariable("engScope", baseProjectView.getEngScope());
context.setVariable("country", baseProjectView.getCountry());
context.setVariable("currently", baseProjectView.getCurrently());
try {
TemplateUtil.template("projectDetail", context,
new File(filepath + "/project/detail/" + baseProjectView.getId() + ".html"));
} catch (IOException e) {
e.printStackTrace();
}
}
current++;
}
}
private void generateTemplateDetail() {
QueryWrapper<BaseProjectView> wrapper = new QueryWrapper<>();
wrapper.eq("language", "ZH");
List<BaseProjectView> list = baseProjectViewService.list(wrapper);
for (BaseProjectView baseProjectView : list) {
//创建Context对象(存放Model)
Context context = new Context();
//放入数据
context.setVariable("name", baseProjectView.getProjectName());
context.setVariable("date", baseProjectView.getSetUpDate());
context.setVariable("desc", baseProjectView.getProjectDescription());
try {
TemplateUtil.template("projectDetail", context,
new File(filepath + "/project/detail/" + baseProjectView.getId() + ".html"));
} catch (IOException e) {
e.printStackTrace();
}
}
}
private void generateTemplateList() {
int current = 1;
int size = 20;
while (true) {
Page<BaseProjectView> page = new Page<>(current, size);
QueryWrapper<BaseProjectView> wrapper = new QueryWrapper<>();
wrapper.eq("LANGUAGE", "ZH");
Page<BaseProjectView> page1 = baseProjectViewService.page(page, wrapper);
int total = (int) page1.getTotal();
int totalPage = total / size;
if (totalPage * size < total) {
totalPage += 1;
}
List<BaseProjectView> list = page1.getRecords();
//创建Context对象(存放Model)
Context context = new Context();
//放入数据
context.setVariable("list", list);
context.setVariable("current", current);
context.setVariable("total", total);
context.setVariable("totalPage", totalPage);
context.setVariable("page", page1);
context.setVariable("jumpUrl", "list");
try {
if (list.size() == 0) {
break;
}
TemplateUtil.template("projectList", context,
new File(filepath + "/project/list/list" + current + ".html"));
current++;
} catch (IOException e) {
e.printStackTrace();
}
}
}
@GetMapping("generate")
@ResponseBody
public String generate() {
// 生成静态页面
generateTemplateList();
generateTemplateDetail2();
return "成功静态页面生成";
}
}
5.測試模板頁面
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="../../css/global.css">
<link rel="stylesheet" href="../../css/list.css">
<link rel="stylesheet" type="text/css" href="https://www.layuicdn.com/layui/css/layui.css" />
<script src="../../js/global.js"></script>
<script src="../../js/list.js"></script>
<script src="https://www.layuicdn.com/layui/layui.js"></script>
<title>站点列表</title>
</head>
<body>
<div class="list">
<div class="navbar">
<div class="container">
<span class="layui-breadcrumb" lay-separator=">">
<a href="../../map.html">首页</a>
</span>
</div>
</div>
<div class="container">
<div class="listitems">
<div class="itemlist">
<div class="itemhead">
<div class="index">序号</div>
<div class="title">标题</div>
<div class="date">日期</div>
</div>
<div class="itemcontent" th:each="item,serviceStat: ${list}">
<a class="item" th:href="'../detail/'+${item.getId()}+'.html'">
<div class="index" th:text="${serviceStat.index+1}"></div>
<div class="title" th:text="${item.getProjectName()}"></div>
<div class="date" th:text="${item.getSetUpDate()}"></div>
</a>
</div>
</div>
</div>
</div>
<!-- 分頁 -->
<div style="float: left" th:if="${page.hasNext()}">
当前第<span th:text="${page.current}"></span>页,共<span
th:text="${totalPage}"></span>页,总记录数<span th:text="${page.total}"></span>
</div>
<div style="float: right">
<a th:href="@{${jumpUrl}+'1.html'}" th:text="首页" th:if="${page.current>1}" th:class="page-item"></a>
<a th:href="@{${jumpUrl}+${page.current-1}+'.html'}" th:text="上一页" th:class="page-item"
th:if="${page.current>1}"></a>
<a th:href="@{${jumpUrl}+ ${i}+'.html'}"
th:each="i :${#numbers.sequence(1, totalPage)}" th:text="${i}"
th:class="${page.current == i}? 'page-item active' :'page-item' "></a>
<a th:href="@{${jumpUrl}+${page.current+1}+'.html'}" th:text="下一页" th:class="page-item"
th:if="${page.current<totalPage}"></a> <a
th:href="@{${jumpUrl}+${totalPage}+'.html'}" th:text="尾页" th:class="page-item"
th:if="${page.current<totalPage}"></a>
</div>
</div>
</body>
</html>
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="../../css/global.css">
<link rel="stylesheet" href="../../css/detail.css">
<link rel="stylesheet" type="text/css" href="https://www.layuicdn.com/layui/css/layui.css" />
<script src="https://www.layuicdn.com/layui/layui.js"></script>
<script src="../../js/global.js"></script>
<script src="../../js/detail.js"></script>
<title>站点详情</title>
</head>
<body>
<div class="detail">
<div class="navbar">
<div class="container">
<span class="layui-breadcrumb" lay-separator=">">
<a href="../../map.html">首页</a>
</span>
</div>
</div>
<div class="container content">
<h3 class="detailTile" th:text="${name}"></h3>
<div class="info">
<div class="releaseDate">发布时间:<span th:text="${date}"></span></div>
<div class="releaseDate">大洲:<span th:text="${continent}"></span></div>
<!-- <div class="releaseDate">图片地址:<span th:text="${banner}"></span></div>-->
<div class="releaseDate">技术门类:<span th:text="${engType}"></span></div>
<div class="releaseDate">技术范围:<span th:text="${engScope}"></span></div>
<div class="releaseDate">国家:<span th:text="${country}"></span></div>
<div class="releaseDate">当前阶段:<span th:text="${currently}"></span></div>
<div class="source">来源:<span>https://www.2021cn.com</span></div>
</div>
<div class="article">
<img src="" alt="">
<span th:utext="${desc}"></span>
</div>
</div>
</div>
<script>
//初始化样式
function initSize(){
let el_detail = document.querySelector('.detail');
let el_content = document.querySelector('.content');
el_detail.style.minHeight = getWindowSize().wh + 'px';
el_content.style.minHeight = getWindowSize().wh -160 + 'px';
}
initSize()
</script>
</body>
</html>