1.前端代码
<template>
<div>
<el-upload
style="height: 100%"
ref="upload"
action="action"
:limit="limitNum"
:auto-upload="true"
:drag="true"
:accept="accept"
:show-file-list="false"
:list-type="listType"
:http-request="httpRequest"
:before-upload="beforeUpload"
:on-change="fileChange"
:on-exceed="exceedFile"
:on-success="handleSuccess"
:on-progress="handleProgress"
:on-error="handleError"
:on-remove="removeFile"
:file-list="fileList"
:class="status == 3? 'uploadRedBorder':'uploadBorder'"
>
<div style="width:100%">
<div class="upload-content">
<i :class="icon" :style="{'color':color}"></i>
<el-tooltip
class="item"
effect="dark"
content="请上传符合模板格式的文件哦!"
placement="top"
>
<span v-if="status === 1" :style="{'color':color}">{{text}}</span>
</el-tooltip>
<span v-if="status !== 1" :style="{'color':color}">
<div class= "box" v-if="status == 4">
<span class= "clip" :style= "clipStyle"></span>
<span class="clipNum">{{Math.round(progress)+"%"}}</span>
</div>
{{text}}
</span>
</div>
<!--点击上传 1-->
<div class="el-upload__text" v-if="status == 1">
您也可以将文件拖动到这里
<el-tooltip
class="item"
effect="dark"
:content="tip"
placement="top"
>
<i class="el-icon-warning"></i>
</el-tooltip>
</div>
<!--上传成功 2-->
<div class="el-upload__text" v-else-if="status == 2" style="display:flex">
<div style="width: 70%" class="file-name"><div class="ellipsis"><i class="el-icon-document"></i>{{fileName}}</div></div>
<div style="flex: 0 0 auto" class="del"><el-link type="danger" @click.stop="handleDelFile(file)">删除</el-link></div>
</div>
<!--上传中 4-->
<div class="el-upload__text" v-else-if="status == 4" style="display:flex">
<div style="width: 70%" class="file-name"><div class="ellipsis"><i class="el-icon-document"></i>{{fileName}}</div></div>
<div style="flex: 0 0 auto" class="del"><el-link type="primary" @click.stop="handleDelFile(file)">取消</el-link></div>
</div>
<!--上传失败 3-->
<div class="el-upload__text" v-else-if="status == 3" style="display:flex">
<div style="width: 70%" class="file-name"><div class="ellipsis"><i class="el-icon-document"></i>{{fileName}}</div></div>
<div style="flex: 0 0 auto" class="del"><el-link type="primary" @click.stop="handleDelFile(file)">重新上传</el-link></div>
</div>
</div>
</el-upload>
<div class="errTipText" v-show="status == 3"><i class="el-icon-warning-outline"></i>{{msg}} <div v-if="link != ''">您可以<div class="downModel" @click="downloadError">下载文件</div>查看原因</div></div>
</div>
</template>
<script>
export default {
name: "test",
data () {
return {
limitNum: 2,
listType: '',
isPictureImg: false,
fileList: [],
menu: false,
progress: '0',
status: 1,
icon: 'el-icon-upload',
color: '#1E78FF',
text: '点击上传',
button: '',
msg: '',
formLabelWidth: '80px',
file: null,
fileName: '',
link: '',
action: '/upload/upload',
maxSize: 10,
drag: true,
accept: '.xlsx',
tip: '支持xlsx文件类型,限制10M'
};
},
methods: {
httpRequest(param){
var fd = new FormData()
fd.append('file', param.file)
fd.append('name', param.file.name)
this.$axios({
url: this.action,
method: 'post',
data: fd,
headers: { 'Content-Type': 'multipart/form-data', 'Authorization': sessionStorage.getItem("token") },
onUploadProgress: progressEvent => {
param.file.percent = (progressEvent.loaded / progressEvent.total * 100)
param.onProgress(param.file)
}
}).then(res => {
if (res.data.success) {
param.onSuccess(res)
} else {
param.onError(res)
}
}).catch(error => {
param.onError(error)
console.log(error)
})
},
handleProgress(event, file, fileList){
if(file){
this.status = 4
this.text = '上传中'
this.icon = ''
this.fileName = file.name
this.color = '#BBC1D9'
this.button = '取消'
this.progress = event.percent
}else{
this.$refs.upload.abort(file)
this.status = 3
this.progress = 0
}
},
removeFile(file, fileList){
if(fileList.length > 0){
this.file = fileList[fileList.length-1]
}else{
this.file = null
}
},
handleDelFile(file){
this.text = '点击上传'
this.icon = 'el-icon-upload',
this.color = '#1E78FF',
this.msg = ''
this.link = ''
this.fileName = ''
this.status = 1
this.$refs.upload.handleRemove(file);
this.$emit("handleDisable",true)
},
exceedFile(file, fileList) {
},
fileChange(file, fileList) {
if (fileList.length > 1) {
fileList.splice(0, 1)
this.fileName = ''
}
if(fileList.length > 0){
this.file = fileList[fileList.length-1]
this.fileName = file.name
}else{
this.showFileList = false
}
},
beforeUpload(file) {
this.msg = '';
var extension = file.name.substring(file.name.lastIndexOf('.') + 1)
if (this.accept.indexOf(extension)<0) {
this.fileName = file.name
this.msg = '文件类型错误'
this.status = 3
return false
}
var size = file.size / 1024 / 1024
if (size > 10) {
this.fileName = file.name
this.msg = '文件大小不能超过10MB!'
this.status = 3;
this.button = '重新上传'
this.text = '上传失败'
this.color = '#F43C3C'
this.icon = 'el-icon-error'
this.$emit("handleDisable",true)
return false;
}
},
handleSuccess(res, file, fileList) {
if(res.data.msg != '操作成功'){
this.status = 3;
this.fileName = file.name
this.icon = 'el-icon-error'
this.button = '重新上传'
this.text = '上传失败'
this.color = '#F43C3C'
this.msg = res.data.msg
if(res.data.data != ''){
this.link = res.data.data
}
if(!res.data.msg){
this.msg = '上传失败,请再试一次'
}
}else{
this.status = 2;
this.icon = 'el-icon-success'
this.text = '上传成功'
this.button = '删除'
this.color = '#67C23A'
}
this.$emit("handle",res)
},
handleError(err, file, fileList) {
this.status = 3;
this.fileName = file.name
this.icon = 'el-icon-error'
this.button = '重新上传'
this.text = '上传失败'
this.color = '#F43C3C'
this.msg = err.data.msg
if(!err.data.msg){
this.msg = '上传失败,请再试一次'
}
this.$emit("handleDisable",true)
},
clearFileData(){
this.text = '点击上传'
this.icon = 'el-icon-upload',
this.color = '#1E78FF',
this.msg = ''
this.fileName = ''
this.status = 1
this.fileList = []
this.file = null
}
}
};
</script>
<style lang="scss" scope>
.ellipsis{
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
}
.upload-content {
font-size: 14px;
line-height: 18px;
color: #1E78FF;
margin-top: 28px !important;
margin-bottom: 16px !important;
cursor: pointer;
}
.upload-content i {
font-size: 22px;
line-height: 24px;
color: #1E78FF;
}
.upload-content span {
vertical-align: text-top;
}
.el-upload-dragger {
width: 248px !important;
height: 110px !important;
background:transparent !important;
}
.el-upload .el-upload-dragger .upload-content i {
margin: 0;
line-height: 20px;
font-size: 20px;
}
</style>
2.后端代码
import com.github.xiaoymin.knife4j.annotations.ApiOperationSupport;
import io.swagger.annotations.*;
import lombok.AllArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import springfox.documentation.annotations.ApiIgnore;
import java.io.File;
import java.util.Map;
@Slf4j
@RestController
@AllArgsConstructor
@RequestMapping("upload")
@Api(value = "文件上传", tags = "文件上传")
public class UploadFacade2 {
@RequestMapping(value = "/upload", method = RequestMethod.POST)
@ApiOperationSupport(order = 1)
@ApiOperation(value = "导入文件", notes = "导入文件")
public Object importKnowledgeExcel(@ApiParam(value = "上传文件", required = true) @RequestParam("file") MultipartFile mf, @ApiIgnore @RequestParam Map<String, Object> params) {
if (mf.isEmpty()) {
return "导入文件为空";
}
String tmpDir = "D:\\";
File tmpDirFile = new File(tmpDir);
if (!tmpDirFile.exists() && !tmpDirFile.isDirectory()) {
tmpDirFile.mkdir();
}
Long time = System.currentTimeMillis();
File file = new File(tmpDir + time);
try {
mf.transferTo(file);
return time;
} catch (Exception e1) {
log.error(e1.getMessage());
}
return false;
}
}
3.示例图片
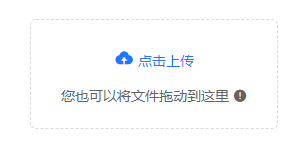