1.图解
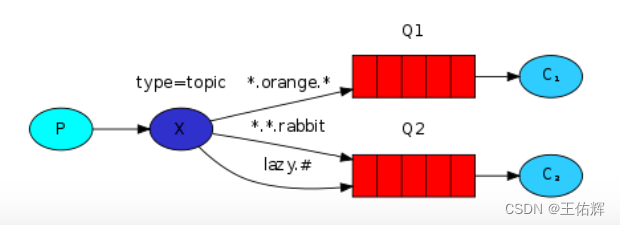
2.生产者
package com.producer;
import com.rabbitmq.client.BuiltinExchangeType;
import com.rabbitmq.client.Channel;
import com.rabbitmq.client.Connection;
import com.rabbitmq.client.ConnectionFactory;
import java.io.IOException;
import java.util.concurrent.TimeoutException;
public class Producer_Topics {
public static void main(String[] args) throws IOException, TimeoutException {
ConnectionFactory connectionFactory = new ConnectionFactory();
connectionFactory.setHost("127.0.0.1");
connectionFactory.setPort(5672);
connectionFactory.setVirtualHost("/learning");
connectionFactory.setUsername("admin");
connectionFactory.setPassword("admin");
Connection connection = connectionFactory.newConnection();
Channel channel = connection.createChannel();
String exchangeName = "test_topic";
channel.exchangeDeclare(exchangeName, BuiltinExchangeType.TOPIC, true, false, false, null);
String queue1 = "test_topic_queue_1";
String queue2 = "test_topic_queue_2";
channel.queueDeclare(queue1, true, false, false, null);
channel.queueDeclare(queue2, true, false, false, null);
channel.queueBind(queue1, exchangeName, "#.error");
channel.queueBind(queue1, exchangeName, "order.*");
channel.queueBind(queue2, exchangeName, "*.*");
String body = "发布订阅-扇形类型";
channel.basicPublish(exchangeName,"order.error",null,body.getBytes());
channel.close();
connection.close();
}
}
3.消费者
a.消费者1
package com.consumer;
import com.rabbitmq.client.*;
import java.io.IOException;
public class Consumer_Topic_1 {
public static void main(String[] args) throws Exception {
ConnectionFactory connectionFactory = new ConnectionFactory();
connectionFactory.setHost("127.0.0.1");
connectionFactory.setPort(5672);
connectionFactory.setVirtualHost("/learning");
connectionFactory.setUsername("admin");
connectionFactory.setPassword("admin");
Connection connection = connectionFactory.newConnection();
Channel channel = connection.createChannel();
String queue1 = "test_topic_queue_1";
Consumer consumer = new DefaultConsumer(channel){
@Override
public void handleDelivery(String consumerTag, Envelope envelope, AMQP.BasicProperties properties, byte[] body) throws IOException {
System.out.println("消费者1打印");
System.out.println("body:" + new String(body));
}
};
channel.basicConsume(queue1, true, consumer);
}
}
b.消费者2
package com.consumer;
import com.rabbitmq.client.*;
import java.io.IOException;
public class Consumer_Topic_2 {
public static void main(String[] args) throws Exception {
ConnectionFactory connectionFactory = new ConnectionFactory();
connectionFactory.setHost("127.0.0.1");
connectionFactory.setPort(5672);
connectionFactory.setVirtualHost("/learning");
connectionFactory.setUsername("admin");
connectionFactory.setPassword("admin");
Connection connection = connectionFactory.newConnection();
Channel channel = connection.createChannel();
String queue2 = "test_topic_queue_2";
Consumer consumer = new DefaultConsumer(channel){
@Override
public void handleDelivery(String consumerTag, Envelope envelope, AMQP.BasicProperties properties, byte[] body) throws IOException {
System.out.println("消费者1打印");
System.out.println("body:" + new String(body));
}
};
channel.basicConsume(queue2, true, consumer);
}
}