SwipeView
- 用户可以通过侧向滑动来浏览界面
- 属性
horizontal : 保存滑动视图是否为水平
interactive : 描述用户是否可以与SwipeView进行交互,设置为true则用户无法滑动
orientation : 保留方向
vertical : 保存滑动视图是否垂直 - 附加属性
index : 保存SwipeView中每个子项的索引
isCurrentItem : 若子项为当前项,则此属性为true
isNextItem : 若子项是下一个项目,则此属性为true
isPreviousItem : 若子项是上一个项目,则此属性为true
view : 包含管理此子项的视图 - 例1 填充一组矩形
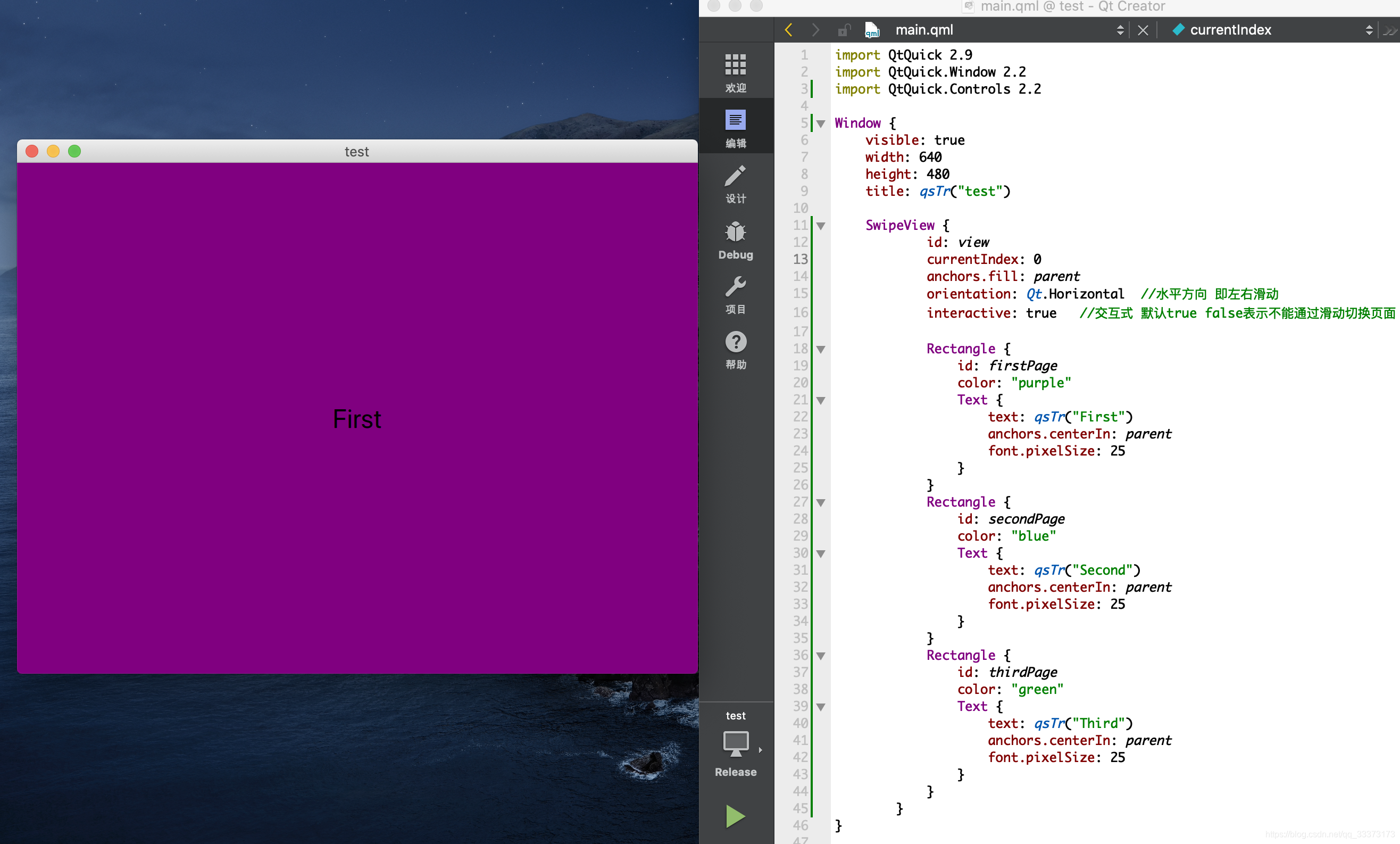
import QtQuick 2.9
import QtQuick.Window 2.2
import QtQuick.Controls 2.2
Window {
visible: true
width: 640
height: 480
title: qsTr("test")
SwipeView {
id: view
currentIndex: 0
anchors.fill: parent
orientation: Qt.Horizontal //水平方向 即左右滑动
interactive: true //交互式 默认true false表示不能通过滑动切换页面
Rectangle {
id: firstPage
color: "purple"
Text {
text: qsTr("First")
anchors.centerIn: parent
font.pixelSize: 25
}
}
Rectangle {
id: secondPage
color: "blue"
Text {
text: qsTr("Second")
anchors.centerIn: parent
font.pixelSize: 25
}
}
Rectangle {
id: thirdPage
color: "green"
Text {
text: qsTr("Third")
anchors.centerIn: parent
font.pixelSize: 25
}
}
}
}
- 例2 填充Page组件
Page是一个容器控件,可以方便地将页眉和页脚项添加到页面。三个重要属性footer、header、title分别对应着页脚、页眉和标题栏。因为我们的SwipeView本身是不可见的,需要为其设置指示才可以知道当前是第几页。通过page的属性我们可以设置指示栏
一般将ToolBar,TabBar或DialogButtonBox分配为页眉页脚
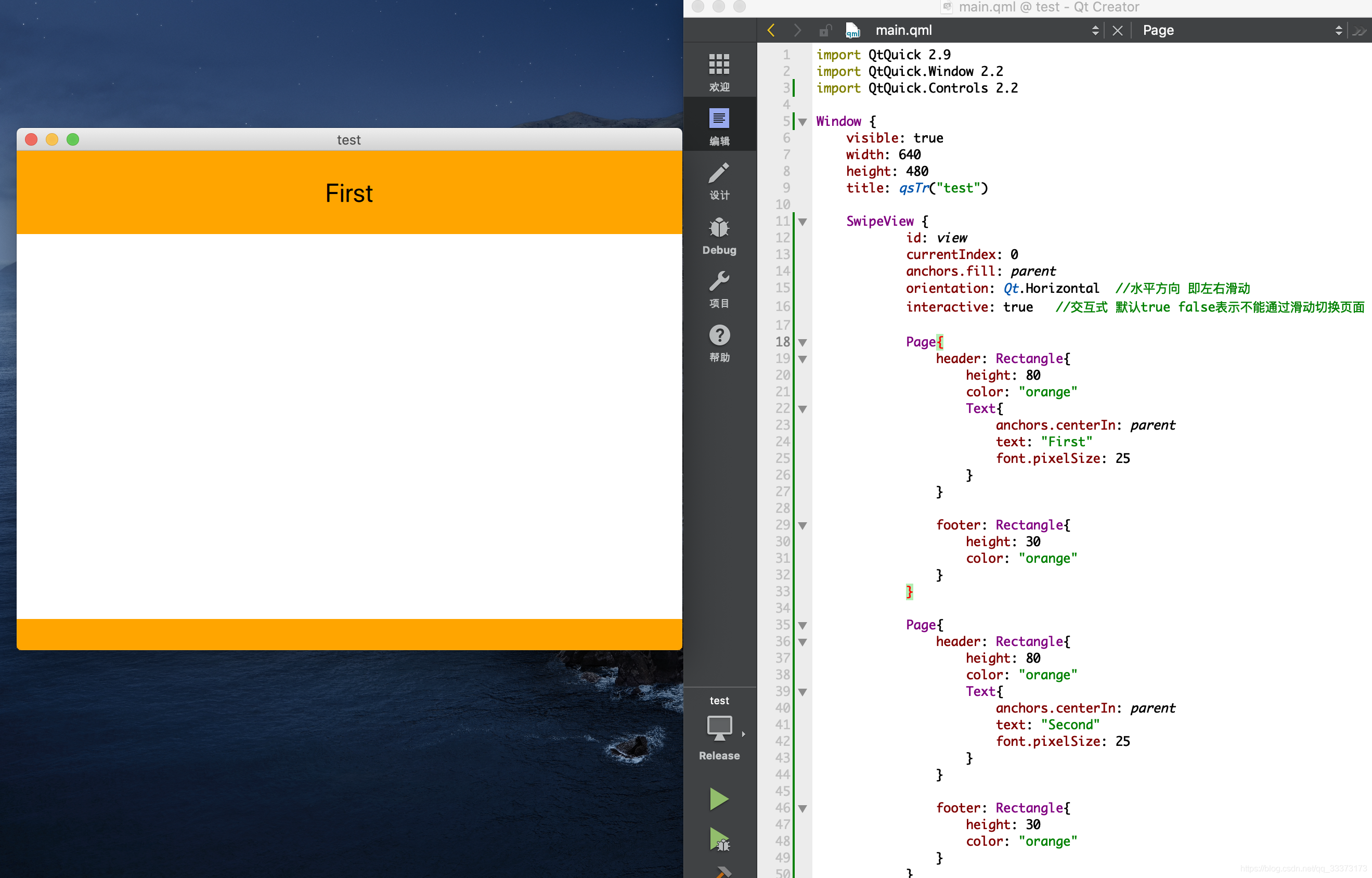
import QtQuick 2.9
import QtQuick.Window 2.2
import QtQuick.Controls 2.2
Window {
visible: true
width: 640
height: 480
title: qsTr("test")
SwipeView {
id: view
currentIndex: 0
anchors.fill: parent
orientation: Qt.Horizontal //水平方向 即左右滑动
interactive: true //交互式 默认true false表示不能通过滑动切换页面
Page{
header: Rectangle{
height: 80
color: "orange"
Text{
anchors.centerIn: parent
text: "First"
font.pixelSize: 25
}
}
footer: Rectangle{
height: 30
color: "orange"
}
}
Page{
header: Rectangle{
height: 80
color: "orange"
Text{
anchors.centerIn: parent
text: "Second"
font.pixelSize: 25
}
}
footer: Rectangle{
height: 30
color: "orange"
}
}
Page{
header: Rectangle{
height: 80
color: "orange"
Text{
anchors.centerIn: parent
text: "Third"
font.pixelSize: 25
}
}
footer: Rectangle{
height: 30
color: "orange"
}
}
}
}
页面指示符PageIndicator
- 与SwipeView的区别是:页面下方多了几个【小圆点】,这些小圆点就是指示符
- 通常很小(为了避免使用户从用户界面的实际内容中分散注意力)。可能难以点击,可能不容易被用户识别为交互式。所以最好用于补充主要的导航方法(例如SwipeView),而不是单独使用
- 属性
count : 保存页数
currentIndex : 保存当前页面的索引
delegate : 保存当前页面的索引
interactive : 保存控件是否为交互式 - 例子
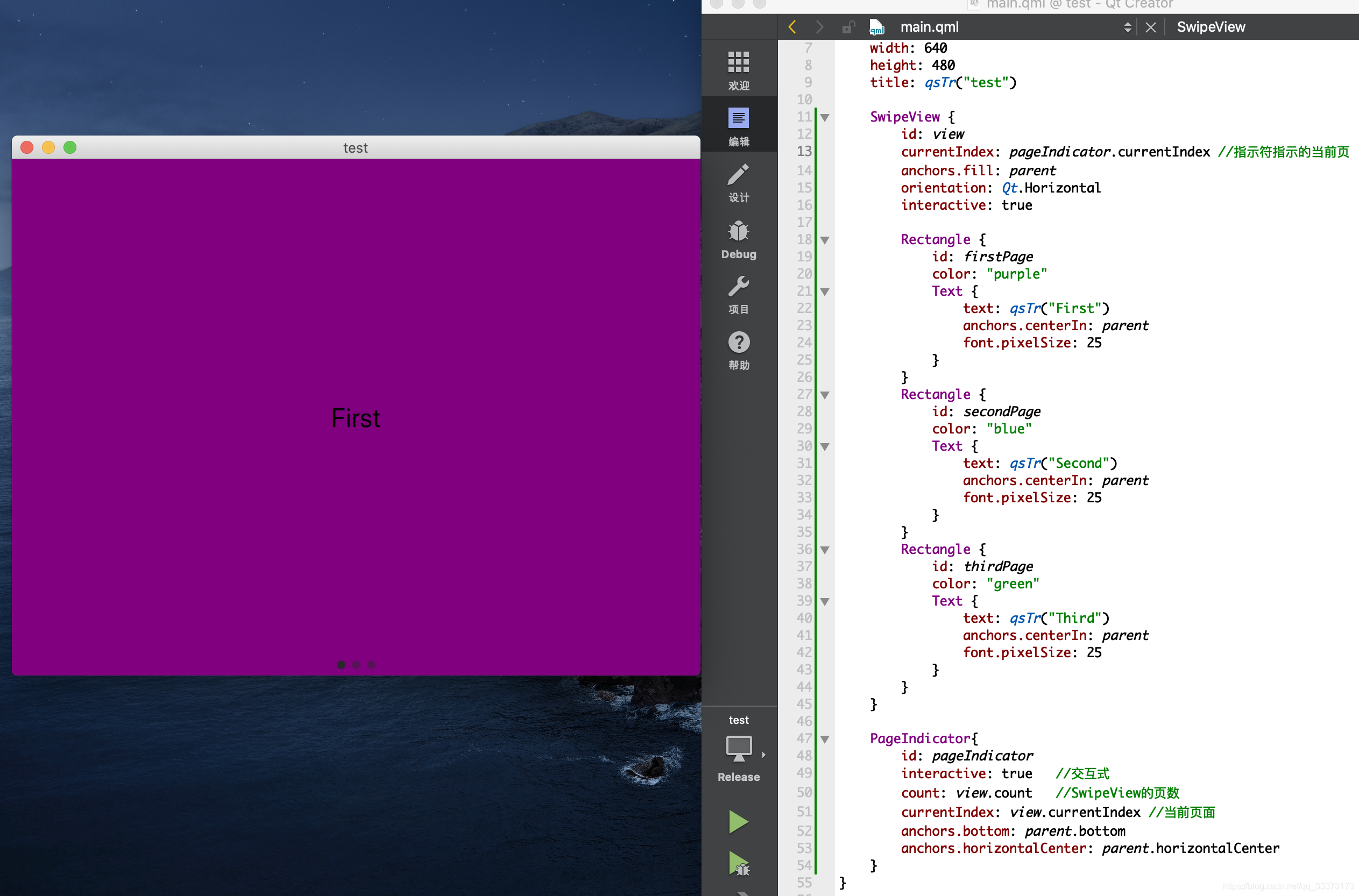
import QtQuick 2.9
import QtQuick.Window 2.2
import QtQuick.Controls 2.2
Window {
visible: true
width: 640
height: 480
title: qsTr("test")
SwipeView {
id: view
currentIndex: pageIndicator.currentIndex //指示符指示的当前页
anchors.fill: parent
orientation: Qt.Horizontal
interactive: true
Rectangle {
id: firstPage
color: "purple"
Text {
text: qsTr("First")
anchors.centerIn: parent
font.pixelSize: 25
}
}
Rectangle {
id: secondPage
color: "blue"
Text {
text: qsTr("Second")
anchors.centerIn: parent
font.pixelSize: 25
}
}
Rectangle {
id: thirdPage
color: "green"
Text {
text: qsTr("Third")
anchors.centerIn: parent
font.pixelSize: 25
}
}
}
PageIndicator{
id: pageIndicator
interactive: true //交互式
count: view.count //SwipeView的页数
currentIndex: view.currentIndex //当前页面
anchors.bottom: parent.bottom
anchors.horizontalCenter: parent.horizontalCenter
}
}
import QtQuick 2.9
import QtQuick.Window 2.2
import QtQuick.Controls 2.2
Window {
visible: true
width: 640
height: 480
title: qsTr("test")
SwipeView {
id: view
currentIndex: pageIndicator.currentIndex //指示符指示的当前页
anchors.fill: parent
orientation: Qt.Horizontal
interactive: true
}
PageIndicator {
id: control
anchors.centerIn: parent
count: 5 //页数有5页
currentIndex: 2 //当前是第3页
delegate: Rectangle { //委托 即一个个的小圆点
implicitWidth: 8
implicitHeight: 8
radius: width / 2
color: "#21be2b"
opacity: index === control.currentIndex ? 0.95 : 0.45 //透明度
}
}
}