1.1
导入预约管理模块实体类
将资料中提供的
POJO
实体类复制到
health_common
工程中。
1.2入项目所需公共资源
项目开发过程中一般会提供一些公共资源,供多个模块或者系统来使用。
本章节我们导入的公共资源有:
(
1
)返回消息常量类
MessageConstant
,放到
health_common
工程中
(
2
)返回结果
Result
和
PageResult
类,放到
health_common
工程中
(
3
)封装查询条件的
QueryPageBean
类,放到
health_common
工程中
(
4
)
html
、
js
、
css
、图片等静态资源,放到
health_backend
工程中
注意:后续随着项目开发还会陆续导入其他一些公共资源。
install 项目:
运行项目: -------- debug方式运行,方便调试
访问url:
http://localhost:82/pages/main.html ,页面显示如下:
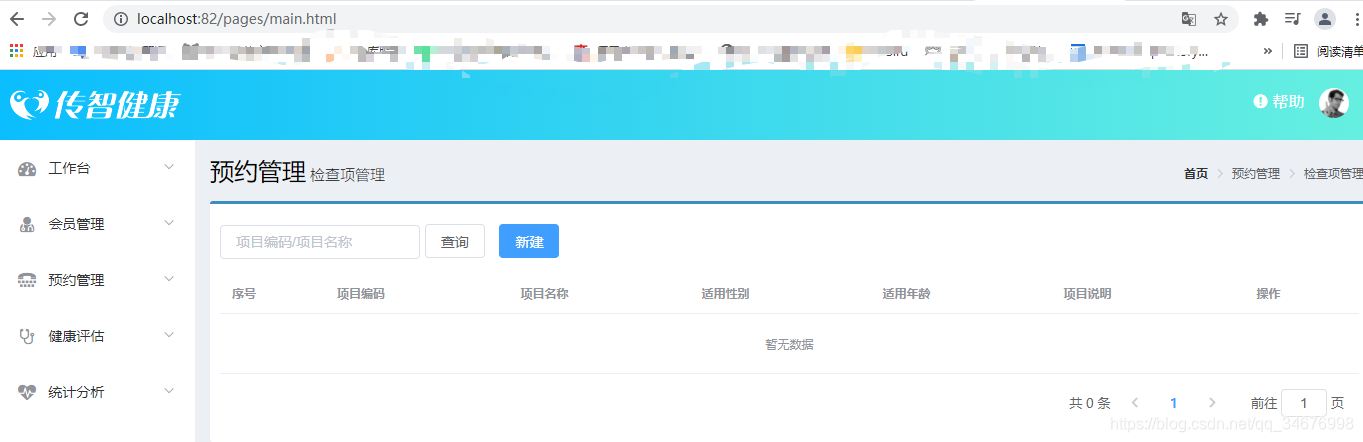
至此,搭建项目已告一段落。
2 新增检查项 完善页面
检查项管理页面对应的是
checkitem.html
页面,根据产品设计的原型已经完成了页面基本结构的编写, 现在需要完善页面动态效果。
使用mybatisX自动生成 mapper和mapper.xml文件(注意其中可能会存在一些错误,手动修正)
2.1 后台代码
Controller
在
health_backend
工程中创建
CheckItemController
@RestController
@RequestMapping("/checkitem")
public class CheckItemController {
@Reference//查找服务
private CheckItemService checkItemService;
//新增检查项
@RequestMapping("/add")
public Result add(@RequestBody CheckItem checkItem){
try{
checkItemService.add(checkItem);
}catch (Exception e){
e.printStackTrace();
//服务调用失败
return new Result(false, MessageConstant.ADD_CHECKITEM_FAIL);
}
return new Result(true, MessageConstant.ADD_CHECKITEM_SUCCESS);
}
}
service实现类 -- health_service_provider
@Service(interfaceClass = CheckItemService.class)
@Transactional
public class CheckItemServiceImpl implements CheckItemService {
//注入DAO对象
@Autowired
private CheckItemDao checkItemDao;
public void add(CheckItem checkItem) {
checkItemDao.add(checkItem);
}
}
dao 层
public interface TCheckItemMapper {
public void add( CheckItem checkItem);
}
================================================
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.itheima.dao.TCheckItemMapper">
<insert id="add" parameterType="com.itheima.pojo.CheckItem">
insert into t_checkitem(code,name,sex,age,price,type,remark,attention)
values
(#{code},#{name},#{sex},#{age},#{price},#{type},#{remark},#{attention})
</insert>
</mapper>
运行项目 ,测试代码
install parent
debugger 运行 health_backend ===(dubbo中的消费者)
运行health_service_provider == dubbo中的提供者
访问url:http://localhost:82/pages/main.html ,点击新建 按钮, 并测试功能。
至此,测试新增检查项目的功能已实现
=========================================================================
1、在启动项目之前需要先启动zookeeper,
2、可以搭建一个dubbo管控台,方便查看生产者和消费者
=========================================================================
4、检查项分页
本项目所有分页功能都是基于
ajax
的异步请求来完成的,请求参数和后台响应数据格式都使用
json
数据 格式。
请求参数包括页码、每页显示记录数、查询条件。
请求参数的
json
格式为:
{currentPage:1,pageSize:10,queryString:''itcast''}
后台响应数据包括总记录数、当前页需要展示的数据集合。
响应数据的
json
格式为:
{total:1000,rows:[]}
定义分页相关模型数据
pagination: {//分页相关模型数据
currentPage: 1,//当前页码
pageSize:10,//每页显示的记录数
total:0,//总记录数
queryString:null//查询条件
},
dataList: [],//当前页要展示的分页列表数据
4.1.2
定义分页方法
在页面中提供了
findPage
方法用于分页查询,为了能够在
checkitem.html
页面加载后直接可以展示分页数据,可以在VUE
提供的钩子函数
created
中调用
findPage
方法
//钩子函数,VUE对象初始化完成后自动执行
created() {
this.findPage();
}
//分页查询
findPage() {
//分页参数
var param = {
currentPage:this.pagination.currentPage,//页码
pageSize:this.pagination.pageSize,//每页显示的记录数
queryString:this.pagination.queryString//查询条件
};
//请求后台
axios.post("/checkitem/findPage.do",param).then((response)=> {
//为模型数据赋值,基于VUE的双向绑定展示到页面
this.dataList = response.data.data.rows;
this.pagination.total = response.data.data.total;
});
}
4.1.3
完善分页方法执行时机
除了在
created
钩子函数中调用
fifindPage
方法查询分页数据之外,当用户点击查询按钮或者点击分页条中的页码时也需要调用findPage
方法重新发起查询请求。
<el-button @click="findPage()" class="dalfBut">查询</el-button>
为查询按钮绑定单击事件,调用findPage方法 为分页条组件绑定current-change事件,此事件是分页条组件自己定义的事件,当页码改变时触发,对应的处理函数为handleCurrentChange
<el-pagination
class="pagiantion"
@current-change="handleCurrentChange"
:current-page="pagination.currentPage"
:page-size="pagination.pageSize"
layout="total, prev, pager, next, jumper"
:total="pagination.total">
</el-pagination>
定义
handleCurrentChange
方法
//切换页码
handleCurrentChange(currentPage) {
//设置最新的页码
this.pagination.currentPage = currentPage;
//重新调用findPage方法进行分页查询
this.findPage();
},
4.2
后台代码
4.2.1 Controller
在
CheckItemController
中增加分页查询方法
@PostMapping("findPage")
public Result findPage(QueryPageBean queryPageBean){
PageResult pageResult = checkItemService.findPage(queryPageBean);
return new Result(true, MessageConstant.QUERY_CHECKITEM_SUCCESS, pageResult);
}
4.2.2
服务接口
在
CheckItemService
服务接口中扩展分页查询方法
@Override
public PageResult findPage(QueryPageBean queryPageBean) {
PageHelper.startPage(queryPageBean.getCurrentPage(),queryPageBean.getPageSize());
Page<CheckItem> page = checkItemDao.selectByCondition(queryPageBean.getQueryString());
long total = page.getTotal();
List<CheckItem> rows = page.getResult();
return new PageResult(total,rows);
}
页面效果: