Hey Friend, it's Anthony from Fullstack React. When we first start writing React code, we got our apps running with minimal setup using <script> tags. While this was easy to get started, for some apps it isn't a good long-term solution (for reasons we'll look at below). Thankfully, the create-react-app was launched. create-react-app generates the foundation for a Webpack-powered React app with no configuration required. Since its inception, we've loved using create-react-app to run our apps. Webpack is a powerful tool for developing and deploying React applications and create-react-app makes it easy to use. So why might you want to use create-react-app? Support for ES6 modules In software, a module is a self-contained component of a software system that is responsible for some discrete functionality. The module exposes a limited interface to the rest of the system, ideally the minimum viable interface the rest of the system needs to effectively use the module. Until ES6, modules were not natively supported in JavaScript. If developers wanted to split modules across many different files and have each module "hide" functionality intended to be private, they had to use one of many odd patterns to do so. Browsers don't yet support ES6 modules. But ES6 modules are the future. The syntax is intuitive, we avoid bizarre tactics employed in ES5, and they work both in and outside of the browser. Because of this, the React community has quickly adopted ES6 modules. To get an idea of how ES6 modules work, consider this example module:
// greetings.js
export const sayHi = ()
=> (console.log('Hi!'));
export const sayBye = ()
=> (console.log('Bye!'));
const saySomething = ()
=> (console.log('Something!'));
|
|
Now, anywhere we wanted to use these functions we could use import . We need to specify which functions we want to import. A common way of doing this is using ES6's destructuring assignment syntax to list them out like this:
// app.js
import { sayHi, sayBye } from './greetings';
sayHi(); // -> Hi!
sayBye(); // => Bye!
|
|
Importantly, the function that was not exported (saySomething ) is unavailable outside of the module. React components as ES6 modules In React, we can compose each of our individual components as their own modules. Each component is responsible for some discrete part of our interface. React components might contain their own state or perform complex operations, but the interface for all of them is the same: they accept inputs (props) and output their DOM representation (render ). Users of a React component need not know any of the internal details. React components are inherently modular, but we can take this to the fullest extent using ES6. Consider this PlaylistTable component, composed inside its own file playlist-table.js :
import React from 'react';
import SongRow from './SongRow';
const headerStyle = {
color: "#333",
fontFamily: "monospace",
fontSize: "32",
};
const PlaylistTable = (props)
=> (
<table class="ui celled
table">
<thead style={headerStyle}>
<tr>
<th>Title</th>
<th>Artist</th>
</tr>
</thead>
<tbody>
{
props.songs.map((song)
=> (
<SongRow
song={song}
onSongClick={props.onSongClick}
/>
))
}
</tbody>
</table>
);
export default PlaylistTable;
|
|
The PlaylistTable component is an ES6 module. It lives in its own dedicated file. At the top, it declares its dependencies, like React and its child component SongRow . The module also has a styles object (headerStyle ) that only it can access. You can imagine how this modular component model is intended to scale well as the number of components grows to the hundreds or thousands. We don't have to worry about polluting the global namespace. Developers on the codebase can quickly reason what this component's dependencies are. And this setup paves the way for only sending the user the React components necessary to render the page he or she is on at any given moment. Managing external dependencies npm is loaded with open-source libraries that you can use in your applications. Wouldn't it be nice to be able to use a package.json to install and manage these dependencies for our React apps like we do our Node apps? Enter Webpack Webpack is a JavaScript bundler. You may have used Webpack or another JavaScript bundler before, like Browserify. In essence, JavaScript bundlers take all of your app's JavaScript code - both your code and the code of any external libraries - and "bundle" them up into a single file. This enables you to write ES6 JavaScript modules and manage external dependencies with npm. Furthermore, bundling your JavaScript improves performance in production. It's faster for a browser to download a few larger files than it is to download many smaller files. Webpack builds on this paradigm and offers developers much, much more. As we explore in the chapter, Webpack comes with a variety of features for both development and deployment. In development, Webpack can hot-reload certain assets (no refreshing the browser needed) or automatically lint your JavaScript code whenever you save. For production, Webpack can prepare an optimized bundle, ready to deploy to any asset host. A powerful tool like Webpack comes at a cost, however. Webpack is complex and often hard to setup. That's where create-react-app comes in. What's been your biggest challenge in learning React? There's been a lot of discussion about how JavaScript development can be overwhelming. Could you do me a favor? Could you reply to this email and tell me specifically what your biggest challenges have been learning React this year? I'd love to hear your struggles and help out any way I can. With love, Anthony 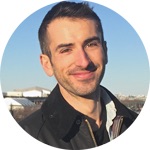 P.S. In Fullstack React we have a bonus chapter: Using Webpack with create-react-app! In this chapter, we dive deep into Webpack, demystifying how it works. And while create-react-app provides a "black box" setup, we also demystify it too. We expose create-react-app's internals and get an idea of how all the pieces fit together. Needless to say, when you're finished with this chapter you'll be comfortable and confident using Webpack with create-react-app in your own applications! |