功能:主要获取自己的文件数据存储,然后展示链接等;
例如:通过可以了解treewidget
的数据绑定,信号改变链接等只是,是个很好的例子;
转载请注明https://mp.csdn.net/postedit/8077449
8点击打开链接
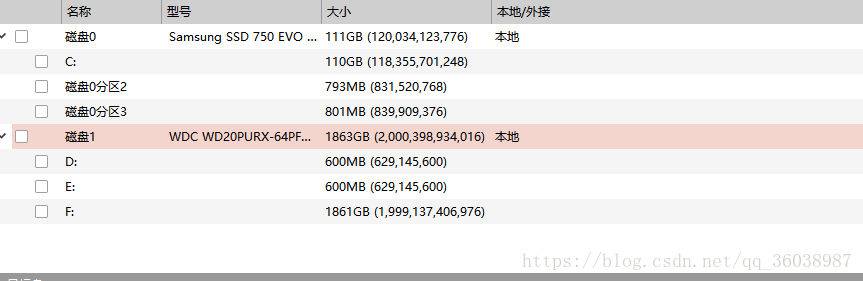
#include
"stdafx.h"
#include "uicommon/device/PDeviceTreeWidget.h"
#include "common/PUtils.h"
namespace puic
{
CPDeviceTreeWidget::CPDeviceTreeWidget(bool withUsbFlag, QWidget* parent/* = nullptr*/,
bool isRadioMode/* = false*/)
: QTreeWidget(parent),
_inited(false),
_withUsbFlag(withUsbFlag),
_isRadioMode(isRadioMode)
{
if (isRadioMode) {
setObjectName("common_radio");
}
else {
setObjectName("common");
}
setAlternatingRowColors(true); //交替背景色
//设置最小列宽
header()->setMinimumSectionSize(60);
connect(this, SIGNAL(itemChanged(QTreeWidgetItem*, int)), this, SLOT(slotItemChanged(QTreeWidgetItem*, int)));//内容发生变化
}
void CPDeviceTreeWidget::slotItemChanged(QTreeWidgetItem *item, int num)
{
bool isChecked = item->checkState(0) == Qt::Checked;
if (_isRadioMode) {
//单选模式时,选中一个条目时,把其他条目不选中
if (isChecked) {
int topItemCount = topLevelItemCount();
for (int i = 0; i < topItemCount; ++i) {
//把其他顶层item Uncheck
QTreeWidgetItem* topItem = topLevelItem(i);
if (topItem != item && topItem->checkState(0) != Qt::Unchecked) {
topItem->setCheckState(0, Qt::Unchecked);
}
//把其他子item Uncheck
int childCount = topItem->childCount();
for (int i = 0; i < childCount; ++i) {
QTreeWidgetItem* childItem = topItem->child(i);
if (childItem != item && childItem->checkState(0) != Qt::Unchecked) {
childItem->setCheckState(0, Qt::Unchecked);
}
}
}
}
}
else {
//选中磁盘时,分区不能选中
int childCount = item->childCount();
for (int i = 0; i < childCount; ++i) {
QTreeWidgetItem* childItem = item->child(i);
if (isChecked) {
childItem->setCheckState(0, Qt::Unchecked);
childItem->setDisabled(true);
}
else {
childItem->setDisabled(false);
}
}
}
}
void CPDeviceTreeWidget::init()
{
if (_inited) {
return;
}
QStringList headerLabels;
getHeaderLabels(headerLabels);
setHeaderLabels(headerLabels);
setColumnWidths();
_inited = true;
}
void CPDeviceTreeWidget::getHeaderLabels(QStringList& list)
{
list << "" << tr("name") << tr("model") << tr("size");
if (_withUsbFlag) {
list << tr("local/usb");
}
}
void CPDeviceTreeWidget::setColumnWidths()
{
if (_isRadioMode) {
setColumnWidth(0, 50);
}
else {
setColumnWidth(0, 70);
}
setColumnWidth(1, 100);
setColumnWidth(2, 160);
setColumnWidth(3, 170);
if (_withUsbFlag) {
setColumnWidth(4, 100);
}
}
void CPDeviceTreeWidget::getDiskStrlist(const pdevice::DeviceDisk& disk,
QStringList& list)
{
list << ""
<< QString::fromStdWString(disk.displayName)
<< QString::fromStdWString(disk.vendor + L" " + disk.model)
<< QString::fromStdWString(CPUtils::formatSizeUnitComma(disk.size));
if (_withUsbFlag) {
list << (disk.removable ? tr("usb") : tr("local"));
}
}
void CPDeviceTreeWidget::getPartitionStrlist(const pdevice::DevicePartition& partition,
QStringList& list)
{
list << ""
<< QString::fromStdWString(partition.displayName)
<< ""
<< QString::fromStdWString(CPUtils::formatSizeUnitComma(partition.size));
if (_withUsbFlag) {
list << "";
}
}
void CPDeviceTreeWidget::setDevices(const pdevice::DeviceDisks& disks,
bool withPartition)
{
//判断磁盘之前是否是选中的
libdt::OneDevices selectedDevices;
getSelectedDevices(selectedDevices);
auto isDeviceSelectedFunc = [&selectedDevices](const std::wstring& devName){
for (auto& oneDev : selectedDevices) {
if (oneDev.deviceName == devName) {
return true;
}
}
return false;
};
init();
clear();
for (auto& disk : disks) {
QStringList strs;
getDiskStrlist(disk, strs);
if (strs.empty()) {
continue;
}
QTreeWidgetItem* topItem = new QTreeWidgetItem(this, strs);
topItem->setData(0, DevNameRole, QString::fromStdWString(disk.name));
topItem->setData(0, DevTypeRole, disk.deviceType);
addTopLevelItem(topItem);
Qt::CheckState checkState = isDeviceSelectedFunc(disk.name) ?
Qt::Checked : Qt::Unchecked;
topItem->setCheckState(0, checkState);
if (withPartition) {
for (auto& partition : disk.partitions) {
QStringList pstrs;
getPartitionStrlist(partition, pstrs);
QTreeWidgetItem* subItem = new QTreeWidgetItem(topItem, pstrs);
subItem->setCheckState(0, Qt::Unchecked);
subItem->setData(0, DevNameRole, QString::fromStdWString(partition.name));
subItem->setData(0, DevTypeRole, partition.deviceType);
}
}
}
//不默认展开了
//expandAll();
}
void CPDeviceTreeWidget::addLogicalPartitions(const pdevice::DevicePartitions& partitions)
{
for (auto& partition : partitions) {
QStringList strs;
getPartitionStrlist(partition, strs);
if (strs.empty()) {
continue;
}
QTreeWidgetItem* topItem = new QTreeWidgetItem(this, strs);
topItem->setData(0, DevNameRole, QString::fromStdWString(partition.name));
topItem->setData(0, DevTypeRole, partition.deviceType);
addTopLevelItem(topItem);
topItem->setCheckState(0, Qt::Unchecked);
}
}
void CPDeviceTreeWidget::getSelectedDevices(libdt::OneDevices& devices)
{ /*当选择数据的列表的时候*可以通过data获取相应的存储数据-》直线通过setdata存储的*/
auto putDevNameFunc = [&devices](QTreeWidgetItem* item, bool isPartition) { //心语法? c++11
QString qdevName = item->data(0, DevNameRole).toString();
libdt::OneDevice oneDevice;
oneDevice.deviceName = qdevName.toStdWString();
oneDevice.isPartition = isPartition;
devices.push_back(oneDevice);
};
int topItemCount = topLevelItemCount();
//遍历
for (int i = 0; i < topItemCount; ++i) {
QTreeWidgetItem* topItem = topLevelItem(i);
if (topItem->checkState(0) == Qt::Checked) {
int devType = topItem->data(0, DevTypeRole).toInt();
bool isPartition = devType == pdevice::PDEVICE_PARTITION;
putDevNameFunc(topItem, isPartition);
}
else {
int childCount = topItem->childCount();
for (int j = 0; j < childCount; ++j) {
QTreeWidgetItem* childItem = topItem->child(j);
if (childItem->checkState(0) == Qt::Checked) {
putDevNameFunc(childItem, true);
}
}
}
}
}
void CPDeviceTreeWidget::selectAll(bool select)
{
Qt::CheckState state = select ? Qt::Checked : Qt::Unchecked;
int count = topLevelItemCount();
for (int i = 0; i < count; ++i) {
QTreeWidgetItem* topItem = topLevelItem(i);
topItem->setCheckState(0, state);
int childCount = topItem->childCount();
for (int j = 0; j < childCount; ++j) {
QTreeWidgetItem* childItem = topItem->child(j);
childItem->setCheckState(0, state);
}
}
}
bool CPDeviceTreeWidget::setDeviceSelected(const std::wstring& deviceName, bool select)
{
if (deviceName.empty()) {
return false;
}
Qt::CheckState state = select ? Qt::Checked : Qt::Unchecked;
//查找磁盘
int topItemCount = topLevelItemCount();
for (int i = 0; i < topItemCount; ++i) {
QTreeWidgetItem* topItem = topLevelItem(i);
QString devName = topItem->data(0, DevNameRole).toString();
if (devName.toStdWString() == deviceName) {
topItem->setCheckState(0, state);
return true;
}
//查找分区
int childCount = topItem->childCount();
for (int j = 0; j < childCount; ++j) {
QTreeWidgetItem* childItem = topItem->child(j);
QString childName = childItem->data(0, DevNameRole).toString();
if (childName.toStdWString() == deviceName) {
childItem->setCheckState(0, state);
return true;
}
}
}
return false;
}的
}