public class SingleLinkedList {
private Node headNode;
private Node lastNode;
private int size;
public void add(Object element) {
Node node = new Node(element);
if (headNode == null) {
headNode = node;
lastNode = node;
} else {
lastNode.next = node;
lastNode = node;
}
size++;
}
public Object get(int index) {
if (index < 0 || index >= size) {
throw new IndexOutOfBoundsException("数组越界");
}
return node(index).object;
}
/**
* 根据序号删除元素
*
* @param index 序号
*/
public void remove(int index) {
// 1.判断序号是否合法,合法取值范围:[0, size - 1]
if (index < 0 || index >= size) {
throw new IndexOutOfBoundsException("序号不合法,index:" + index);
}
// 2.处理删除节点在开头的情况
if (index == 0) {
// 2.1 获得删除节点的后一个节点
Node nextNode = headNode.next;
// 2.2 设置 headNode 的 next 值为 null
headNode.next = null;
// 2.3 设置 nextNode 为单链表的首节点
headNode = nextNode;
}
// 3.处理删除节点在末尾的情况
else if (index == size - 1) {
// 3.1 获得删除节点的前一个节点
Node preNode = node(index - 1);
// 3.2 设置 preNode 的 next 值为 null
preNode.next = null;
// 3.3 设置 preNode 为单链表的尾节点
lastNode = preNode;
}
// 4.处理删除节点在中间的情况
else {
// 4.1 获得 index-1 所对应的节点对象
Node preNode = node(index - 1);
// 4.2 获得 index+1 所对应的节点对象
Node nextNode = preNode.next.next;
// 4.3 获得删除节点并设置 next 值为 null
preNode.next.next = null;
// 4.4 设置 preNode 的 next 值为 nextNode
preNode.next = nextNode;
}
// 5.更新 size 的值
size--;
}
private Node node(int index) {
Node temp = headNode;
for (int i = 0; i < index; i++) {
temp = temp.next;
}
return temp;
}
public static class Node {
private Object object;
private Node next;
public Node(Object object) {
this.object = object;
}
}
java 模拟 单链表
最新推荐文章于 2024-08-07 11:15:21 发布
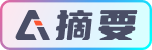