接口文档
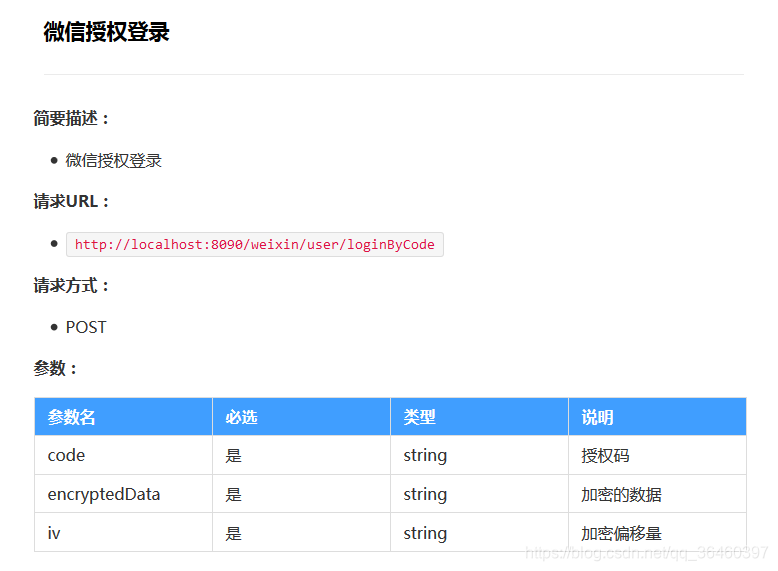
pom文件
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
<version>3.10.0</version>
</dependency>
controller
@PostMapping("loginByCode")
public ResultData loginByCode(@RequestBody WxLoginVo wxLoginVo){
return ResultData.ok200().setData(wxUserService.loginByCode(wxLoginVo));
}
service
WxUser loginByCode(WxLoginVo wxLoginVo);
serviceimpl
package com.youruan.examine.service.impl;
import com.alibaba.fastjson.JSONObject;
import com.youruan.examine.config.enums.AuthorizeEnum;
import com.youruan.examine.config.enums.CommonEnum;
import com.youruan.examine.config.excetion.ServiceException;
import com.youruan.examine.config.global.Mark;
import com.youruan.examine.entity.WxUser;
import com.youruan.examine.entity.vo.WxLoginVo;
import com.youruan.examine.entity.vo.WxUserVo;
import com.youruan.examine.mapper.WxUserMapper;
import com.youruan.examine.service.WxUserService;
import com.youruan.examine.util.HttpUtil;
import com.youruan.examine.util.WxUtil;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import tk.mybatis.mapper.entity.Example;
import java.io.IOException;
import java.util.Date;
import java.util.List;
import java.util.Map;
import java.util.UUID;
@Transactional
@Service
public class WxUserServiceImpl implements WxUserService {
private Logger logger = LoggerFactory.getLogger(WxUserServiceImpl.class);
@Autowired
WxUserMapper userMapper;
@Value("${examine.wxchat.appId}")
private String appId;
@Value("${examine.wxchat.secret}")
private String secret;
@Value("${examine.wxchat.grantType}")
private String grantType;
@Override
public WxUser loginByCode(WxLoginVo loginVo) {
logger.info("loginByCode进入============="+loginVo.toString());
WxUser resVo = new WxUser();
if (StringUtils.isBlank(loginVo.getCode())) {
throw new ServiceException("授权码不能为空");
}
WxUserVo wxUserVo = new WxUserVo();
try {
Map<String, Object> wxResult = getOpenIdByHttpClient(loginVo.getCode());
logger.info(wxResult.toString());
String sessionkey = "";
if (wxResult.containsKey("session_key")) {
sessionkey = wxResult.get("session_key").toString();
}
String openid = "";
if (wxResult.containsKey("openid")) {
openid = wxResult.get("openid").toString();
logger.info(openid);
List<WxUser> userList = listByOppenId(openid);
if (userList.size()>0) {
BeanUtils.copyProperties(userList.get(0),resVo);
return resVo;
}
}
if (StringUtils.isBlank(loginVo.getEncryptedData()) || StringUtils.isBlank(loginVo.getIv())) {
throw new ServiceException("加密的数据不能为空");
}
if (StringUtils.isBlank(sessionkey)) {
throw new ServiceException("授权信息有误");
}
JSONObject userInfo = WxUtil.getUserInfo(loginVo.getEncryptedData(), sessionkey, loginVo.getIv());
logger.info(userInfo.toJSONString());
wxUserVo = JSONObject.toJavaObject(userInfo, WxUserVo.class);
} catch (IOException e) {
e.printStackTrace();
}
loginVo.setSourceType(AuthorizeEnum.WX.getCode());
changeUserInfo(loginVo, wxUserVo);
WxUser user = registerUserByLoginVo(loginVo);
BeanUtils.copyProperties(user,resVo);
return resVo;
}
public Map<String, Object> getOpenIdByHttpClient(String code) throws IOException {
HttpUtil httpUtil = new HttpUtil();
String url = Mark.WX_OPENID_URL;
String result = httpUtil.header("X-Requested-With", "XMLHttpRequest")
.data("appid", appId)
.data("secret", secret)
.data("js_code", code)
.data("grant_type", grantType)
.url(url)