从开始接触Angular至今已经近半年时间,已经基本上熟悉了angular的语法规范和用法。我认为angular最重要的部分还是用自定义指令来封装自己想用的组件。这也正所谓得自定义指令者得angular,可见自定义指令在angular中的地位是何等的高。
效果图
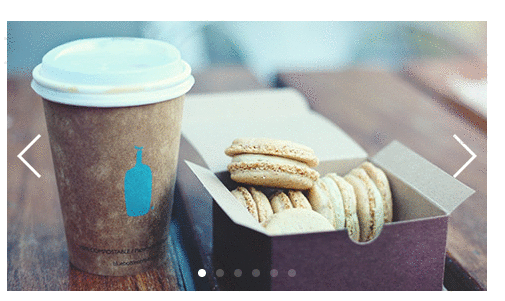
1.swiper插件的使用在上一篇博客中已经阐述,这里就不再多说;
2.使用angular必须首先引入angular.js
3.实现的难点还是在于需要在自定义的指令中的属性中获取数据。实现动态切换的效果
3.1 首先在controller中封装图片数组
var myCtr = ["$scope", function($scope) {
$scope.value = "";
$scope.slides = [];
var slides = $scope.slides =[];
$scope.addSlide = function(){
slides.push({
image: '../img/c' + slides.length + '.jpg',
});
}
for (var i=0; i< 6 ; i++) {
$scope.addSlide();
}
}];
3.2 由于大段代码加载费时,我们使用了缓存加载的机制来加载模板
// 1.开启缓存
app.run(function($templateCache){
// 2 .将片段存入模板
$templateCache.put("temp.html",
"<div class='swiper-container'> \
<div class='swiper-wrapper' > \
<div class='swiper-slide' ng-repeat='slide in slides track by $index'> \
<img src='{{slide.image}}'/> \
</div> \
</div> \
<div class='swiper-pagination swiper-pagination-white'></div> \
<div class='swiper-button-prev swiper-button-white'></div> \
<div class='swiper-button-next swiper-button-white'></div> \
</div>"
)});
3.3 最为华丽的地方最是通过指令系统将控制器中的数据设置到模板片段中,由于一开始没有考虑到切换的时间间隔,没有使用$timeout指令,效果一直出不来,真是急死个人呀。
app.directive("directiveOne", ['$timeout', '$templateCache', function($timeout,$templateCache) {
return {
restrict: "ECMA",
// 3.调用模板
template:$templateCache.get("temp.html"),
replace:false,
scope: {
sValue: "@",
},
controller: function($scope,$attrs) {
//$scope.slides = $attrs.sValue;
//console.log($scope.sValue);
},
link:function(scope,element,attrs){
scope.slides = JSON.parse(attrs.sValue);
//初始化 默认自适应
console.log()
$timeout(function(){
var myswiper = new Swiper('.swiper-container',{
autoplay:2000,//逗号结尾
/*自动切换的时间间隔(单位ms),不写不自动切换
类型:数字 默认是0
*/
initialSlide:0,
/*
设定初始化时的slide索引(默认显示第几张)
类型:数字
索引从0开始
*/
direction:'horizontal',
/*
滑动的方向 可以是水平,垂直
可选值horizontal,vertical
*/
speed:1000,
/*
滑动速度,自动滑动开始到结束的时间(ms)
类型:数字 默认:300
配合autoplay
*/
autoplayDisabledOninteraction:true,
/*
用户操作swiper,是否禁止autoplay
操作:触碰 拖动 点击 (分页)
默认是true 可选的值false
*/
autoplayStopOnLast:true,
/*
切换到最后一个slide停止自动切换
ps:loop时无效
默认:false不停
*/
grabCursor:true,
/*
鼠标指针形状(鼠标覆盖的时候是手,拖动的时候是抓手)
可选值:true false
默认:flase没有抓手
*/
// freeMode:true,
effect:'slider',
pagination:'.swiper-pagination',
prevButton:'.swiper-button-prev',
nextButton:'.swiper-button-next',
loop:true,
});
},100);
}
}
}]);
4.附上完整代码
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>自定义指令</title>
<script type="text/javascript" src="../js/angular-1.5.8.js"></script>
<link rel="stylesheet" type="text/css" href="../css/swiper.min.css"/>
<script src="../css/swiper.min-3.js" type="text/javascript" charset="utf-8"></script>
<style type="text/css">
*{
padding:0;
margin:0;
}
.swiper-container{
width:480px;
height:270px;
margin:50px auto;
}
.swiper-slide{
line-height:300px;
text-align:center;
font-size:40px;
background:aqua;
}
/*.swiper-slide:nth-child(2){
background:pink;
}
.swiper-slide:nth-child(3){
background:yellow;
}
.swiper-slide:nth-child(4){
background:lime;
}
.swiper-slide:nth-child(5){
background:silver;
}
.swiper-slide:nth-child(6){
background:purple;
}*/
</style>
</head>
<body>
<div ng-app="myApp" ng-controller="myCtr">
<!--<input type="" name="" ng-model="value">-->
<!-- 注意此处的svalue要写成s-Value,不然没效果-->
<directive-One s-Value ={{slides}}></directive-One>
</div>
<!-- -->
</body>
<script type="text/javascript">
var myCtr = ["$scope", function($scope) {
$scope.value = "";
$scope.slides = [];
var slides = $scope.slides =[];
$scope.addSlide = function(){
slides.push({
image: '../img/c' + slides.length + '.jpg',
});
}
for (var i=0; i< 6 ; i++) {
$scope.addSlide();
}
}];
var app = angular.module("myApp", []);
app.controller("myCtr", myCtr);
// 1.开启缓存
app.run(function($templateCache){
// 2 .将片段存入模板
$templateCache.put("temp.html",
"<div class='swiper-container'> \
<div class='swiper-wrapper' > \
<div class='swiper-slide' ng-repeat='slide in slides track by $index'> \
<img src='{{slide.image}}'/> \
</div> \
</div> \
<div class='swiper-pagination swiper-pagination-white'></div> \
<div class='swiper-button-prev swiper-button-white'></div> \
<div class='swiper-button-next swiper-button-white'></div> \
</div>"
)});
app.directive("directiveOne", ['$timeout', '$templateCache', function($timeout,$templateCache) {
return {
restrict: "ECMA",
// 3.调用模板
template:$templateCache.get("temp.html"),
replace:false,
scope: {
sValue: "@",
},
controller: function($scope,$attrs) {
//$scope.slides = $attrs.sValue;
//console.log($scope.sValue);
},
link:function(scope,element,attrs){
scope.slides = JSON.parse(attrs.sValue);
//初始化 默认自适应
console.log()
$timeout(function(){
var myswiper = new Swiper('.swiper-container',{
autoplay:2000,//逗号结尾
/*自动切换的时间间隔(单位ms),不写不自动切换
类型:数字 默认是0
*/
initialSlide:0,
/*
设定初始化时的slide索引(默认显示第几张)
类型:数字
索引从0开始
*/
direction:'horizontal',
/*
滑动的方向 可以是水平,垂直
可选值horizontal,vertical
*/
speed:1000,
/*
滑动速度,自动滑动开始到结束的时间(ms)
类型:数字 默认:300
配合autoplay
*/
autoplayDisabledOninteraction:true,
/*
用户操作swiper,是否禁止autoplay
操作:触碰 拖动 点击 (分页)
默认是true 可选的值false
*/
autoplayStopOnLast:true,
/*
切换到最后一个slide停止自动切换
ps:loop时无效
默认:false不停
*/
grabCursor:true,
/*
鼠标指针形状(鼠标覆盖的时候是手,拖动的时候是抓手)
可选值:true false
默认:flase没有抓手
*/
// freeMode:true,
effect:'slider',
pagination:'.swiper-pagination',
prevButton:'.swiper-button-prev',
nextButton:'.swiper-button-next',
loop:true,
});
},100);
}
}
}]);
</script>
</html>
回头想想,实现一个轮播平时我们需要长篇大论的写一通,自从有了自定义指令,只需一行代码就可以轻松搞定。嫲嫲再也不用担心我的工作,心里是不是美滋滋呢。