实现最简单的文件上传
-
1演示图 点击头像打开上传模态框,选择图片上传成功
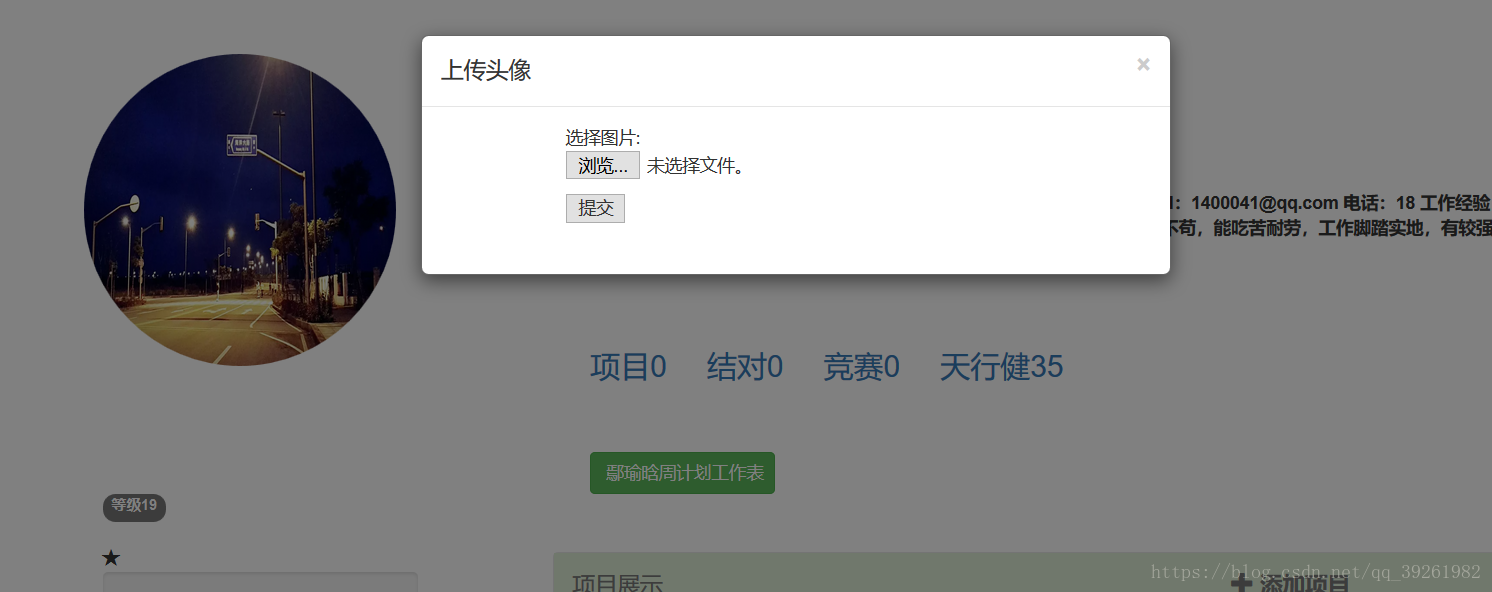 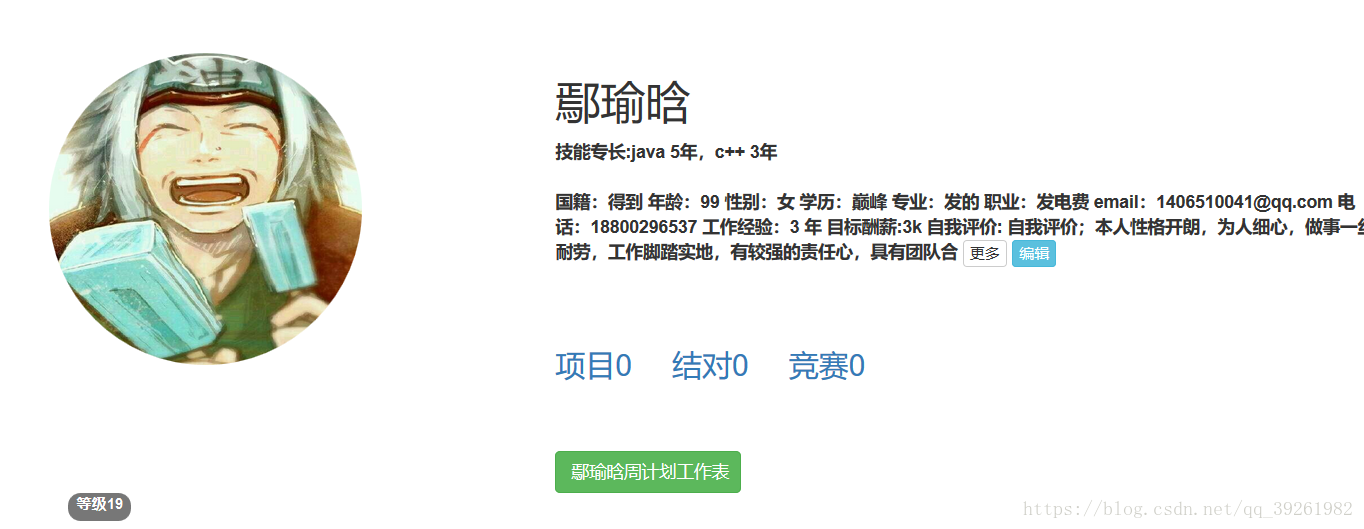
-
2 前端代码
头像图片代码,这段写在js文件动态添加到html。src是拼接的图片名,数据库存的图片字段为img,<img src="img/'+img+'" data-toggle="modal" data-target="#yupdatetx" class="img-circle" height="250" width="250">
通过点击图片打开的模态框代码:
通过form表单提交请求
<div class="modal fade" id="yupdatetx" tabindex="-1" role="dialog"
aria-labelledby="myModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal"
aria-hidden="true">×</button>
<h4 class="modal-title" id="myModalLabel">上传头像</h4>
</div>
<div class="modal-body">
<form action="txUpload" method="post" enctype="multipart/form-data" class="form-horizontal" role="form">
<div class="form-group">
<div class="col-sm-2"></div>
<div class="col-sm-8">
<p>选择图片: <input type="file" name="txName"/></p>
<p><input type="submit" value="提交"/></p>
</div>
<div class="col-sm-2"></div>
</div>
</form>
</div>
</div>
<!-- /.modal-content -->
</div>
<!-- /.modal -->
</div>
- 3后台controller层
package com.zz.bsea.controller;
import javax.servlet.http.HttpServletRequest;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.core.io.ResourceLoader;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.MultipartFile;
import com.zz.bsea.model.Project2;
import com.zz.bsea.model.User;
import com.zz.bsea.service.YyhProject2Service;
import com.zz.bsea.service.YyhUserService;
import com.zz.bsea.util.FileUtils;
@Controller
public class ImgUplodController {
private final ResourceLoader resourceLoader;
@Autowired
public ImgUplodController(ResourceLoader resourceLoader) {
this.resourceLoader = resourceLoader;
}
@Autowired
YyhUserService yyhuser;
@Value("${upload-path}")
private String path2; //文件路径在配置文件
/**
* 跳转到文件上传页面
* @return
*/
/**
*
* @param file 要上传头像
* @return
*/
@RequestMapping("txUpload")
public String upload2(@RequestParam("txName") MultipartFile file,HttpServletRequest req){
// 要上传的目标文件存放路径
FileUtils.upload(file, path2, file.getOriginalFilename());//这个方法调用文件就已经上传到目标文件夹了,
User u=new User(); 这里是更改我用户表我的账号的头像字段的图片,file.getOriginalFilename()这个方法是获取你上传的文件的名字,前端然后显示
u.setImg(file.getOriginalFilename());
yyhuser.updateOwnTx(file.getOriginalFilename(), req);
return "redirect:demo.html"; //这里要改为自己的显示页面html
}
}
文件上传类;
package com.zz.bsea.util;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.io.IOException;
/**
* 文件上传工具包
*/
public class FileUtils {
/**
*
* @param file 文件
* @param path 文件存放路径
* @param fileName 源文件名
* @return
*/
public static boolean upload(MultipartFile file, String path, String fileName){
// 生成新的文件名
//String realPath = path + "/" + FileNameUtils.getFileName(fileName);
//使用原文件名
String realPath = path + "/" + fileName;
File dest = new File(realPath);
//判断文件父目录是否存在
if(!dest.getParentFile().exists()){
dest.getParentFile().mkdir();
}
try {
//保存文件
file.transferTo(dest);
return true;
} catch (IllegalStateException e) {
// TODO Auto-generated catch block
e.printStackTrace();
return false;
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
return false;
}
}
}
配置文件:
server.port=80
server.context-path=/tz
spring.datasource.url=jdbc:mysql://xxx:3306/codebuilder8?useUnicode=true&characterEncoding=utf8
spring.datasource.username=xx
spring.datasource.password=xx
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
spring.profiles.active=dev
## Mybatis
mybatis.typeAliasesPackage=org.spring.springboot.domain
mybatis.mapperLocations=classpath:com/zz/bsea/mapping/*.xml
#分页的配置
pagehelper:
offset-as-page-num: true
row-bounds-with-count: true
reasonable: true
# 设置上传的文件大小
multipart.maxFileSize=10Mb
# All files max size
multipart.maxRequestSize=1000Mb
spring.mvc.static-path-pattern=/**
spring.resources.static-locations=classpath:/META-INF/resources/,classpath:/resources/,classpath:/static/,classpath:/public/,file:${uploadpic.path}
### FreeMarker 配置
spring.freemarker.allow-request-override=false
#Enable template caching.启用模板缓存。
spring.freemarker.cache=false
spring.freemarker.check-template-location=true
spring.freemarker.charset=UTF-8
spring.freemarker.content-type=text/html
spring.freemarker.expose-request-attributes=false
spring.freemarker.expose-session-attributes=false
spring.freemarker.expose-spring-macro-helpers=false
#设置面板后缀
spring.freemarker.suffix=.ftl
upload-path=C:/bsea/wp/20181/201807223555781916/zzjava8/tz/src/main/resources/static/img
#改为自己上传的文件路径
pom.xml配置加入
<!--FreeMarker模板视图依赖-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-freemarker</artifactId>
</dependency>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
<!--以下两项需要如果不配置,解析themleaft 会有问题-->
<thymeleaf.version>3.0.2.RELEASE</thymeleaf.version>
<thymeleaf-layout-dialect.version>2.0.5</thymeleaf-layout-dialect.version>
</properties>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>