一、引入Jar包
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-jexl3</artifactId>
<version>3.1</version>
</dependency>
二、实例
import org.apache.commons.jexl3.JexlBuilder;
import org.apache.commons.jexl3.JexlContext;
import org.apache.commons.jexl3.JexlEngine;
import org.apache.commons.jexl3.MapContext;
import javax.script.Invocable;
import javax.script.ScriptEngine;
import javax.script.ScriptEngineManager;
import javax.script.ScriptException;
import java.util.HashMap;
import java.util.Map;
public class JexlUtil {
private static JexlEngine engine = new JexlBuilder().create();
private static JexlContext context = new MapContext();
private static String VALUE = "value";
private static String VALUES = "values";
public static boolean evaluate(Object value, String operator, Object values) {
String expressionStr = VALUE + " " + operator + " " + VALUES;
context.set(VALUE, value);
context.set(VALUES, values);
return (Boolean) engine.createExpression(expressionStr).evaluate(context);
}
private static ScriptEngineManager scriptEngineManager = new ScriptEngineManager();
private static ScriptEngine javaScriptEngine = scriptEngineManager.getEngineByName("JavaScript");
public static Object executeFunction() {
String script = "function add(x, y) { return x + y;}";
try {
javaScriptEngine.eval(script);
} catch (ScriptException e) {
e.printStackTrace();
}
Invocable invocable = (Invocable) javaScriptEngine;
Object result = null;
try {
result = invocable.invokeFunction("add", 10, 20);
} catch (ScriptException | NoSuchMethodException e) {
e.printStackTrace();
}
return result;
}
public static void execute() {
JexlContext mapContext = new MapContext();
String script = "if (company.type == 1) {\n" +
" company.name = 'a';\n" +
" company.age = 12;\n" +
"} else if (company.type == 3 && company.num > 100) {\n" +
" company.name = 'b';\n" +
" company.age = 13;\n" +
"} else if (company.type == 3 && company.num > 200) {\n" +
" company.name = 'c';\n" +
" company.age = 14;\n" +
"} else {\n" +
" company.name = 'd';\n" +
" company.age = 15;\n" +
"}\n";
Map<String, Object> map = new HashMap<>();
map.put("type", "3");
map.put("num", 200);
mapContext.set("company", map);
engine.createScript(script).execute(mapContext);
System.out.println(((Map)mapContext.get("company")).get("name"));
System.out.println(((Map)mapContext.get("company")).get("age"));
}
public static void main(String[] args) {
boolean result = evaluate(1, "!=", 2);
System.out.println(result);
System.out.println(executeFunction());
execute();
}
}
三、结果
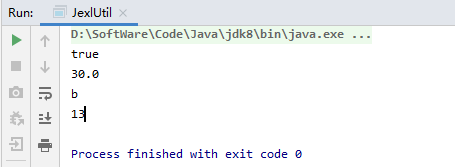