appbar属性
属性 | 释义 |
---|
leading | 导航按钮显示的图标 |
title | 标题 |
actions | 相当于menu |
bottom | 通常用来放置tabBar |
backgroundColor | 导航背景颜色 |
iconTheme | 图表样式 |
textTheme | 文字样式 |
centerTitle | 标题是否居中显示 |
自定义AppBar使用
import 'package:flutter/material.dart';
class AppBarDemoPage extends StatelessWidget {
const AppBarDemoPage({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title:Text("AppBarDemoPage"),
backgroundColor: Colors.red,
centerTitle:true,
leading: IconButton(
icon: Icon(Icons.menu),
onPressed: (){
print('menu');
},
),
actions: <Widget>[
IconButton(
icon: Icon(Icons.search),
onPressed: (){
print('search');
},
),
IconButton(
icon: Icon(Icons.settings),
onPressed: (){
print('settings');
},
)
],
),
body: Text('appbardemo'),
);
}
}
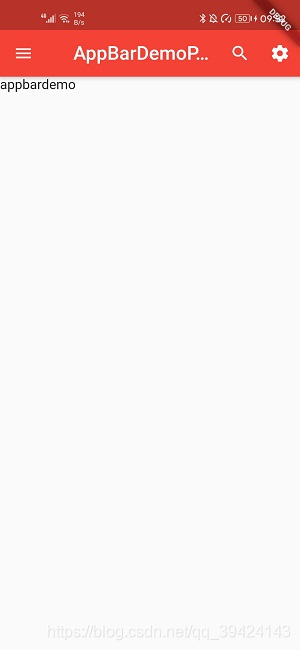
TabBar(TabLayout)
属性
属性 | 释义 |
---|
tabs | 显示标签内容 |
controller | TabController对象 |
isScrollable | 是否可滚动,即是否在一个屏幕放下所有Tab |
indicatorColor | 指示器颜色 |
indicatorWeight | 指示器高度 |
indicatorPadding | 底部指示器的padding |
indicator | 指示器decoration |
indicatorSize | 指示器大小计算方式,TabBarIndicatorSize.label 跟文字等宽,TabBarIndicatorSize.tab 跟每个tab等宽 |
labelColor | 选中label颜色 |
labelStyle | 选中label的style |
labelPadding | 每个label的padding值 |
unselectedLabelColor | 未选中 label 颜色 |
unselectedLabelStyle | 未选中label的style |
import 'package:flutter/material.dart';
class AppBarDemoPage extends StatelessWidget {
const AppBarDemoPage({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return DefaultTabController(
length: 4, // tab个数
child: Scaffold(
// Tab组件必须放到Scaffold中
appBar: AppBar(
title: Text("TabBarDemo"),
bottom: TabBar(
tabs: <Widget>[
Tab(
text: "热点",
),
Tab(
text: "新闻",
),
Tab(
text: "推荐",
),
Tab(
text: "同城",
)
],
)),
body: TabBarView(
// 类似ViewPage
children: <Widget>[
ListView(
children: <Widget>[
ListTile(title: Text("这是第1个 tab")),
ListTile(title: Text("这是第1个 tab")),
ListTile(title: Text("这是第1个 tab"))
],
),
ListView(
children: <Widget>[
ListTile(title: Text("这是第2个 tab")),
ListTile(title: Text("这是第2个 tab")),
ListTile(title: Text("这是第2个 tab"))
],
),
ListView(
children: <Widget>[
ListTile(title: Text("这是第3个 tab")),
ListTile(title: Text("这是第3个 tab")),
ListTile(title: Text("这是第3个 tab"))
],
),
ListView(
children: <Widget>[
ListTile(title: Text("这是第4个 tab")),
ListTile(title: Text("这是第4个 tab")),
ListTile(title: Text("这是第4个 tab"))
],
),
],
),
),
);
}
}
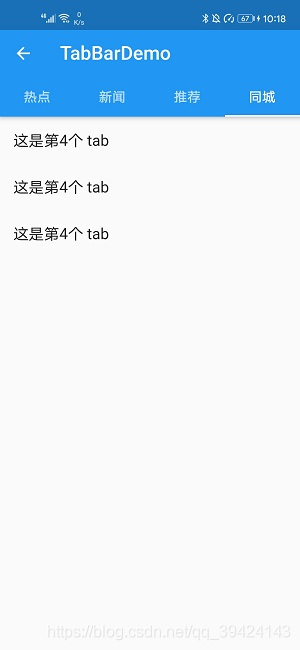
存在Bottomnavigation的页面创建TabLayout
import 'package:flutter/material.dart';
class SystemPage extends StatefulWidget {
SystemPage({Key key}) : super(key: key);
_SystemPageState createState() => _SystemPageState();
}
class _SystemPageState extends State<SystemPage> {
@override
Widget build(BuildContext context) {
return DefaultTabController(
length: 4,
child: Scaffold( // 外部布局已经存在Scaffold,此时内部还可以再嵌套一个Scaffold
appBar: AppBar( // 此时我们在同一个页面创建了两个appbar,所以直接在title中创建tab即可
title: TabBar(
tabs: <Widget>[
Tab(
text: "热点",
),
Tab(
text: "新闻",
),
Tab(
text: "推荐",
),
Tab(
text: "同城",
)
],
)),
body: TabBarView(
// 类似ViewPage
children: <Widget>[
ListView(
children: <Widget>[
ListTile(title: Text("这是第1个 tab")),
ListTile(title: Text("这是第1个 tab")),
ListTile(title: Text("这是第1个 tab"))
],
),
ListView(
children: <Widget>[
ListTile(title: Text("这是第2个 tab")),
ListTile(title: Text("这是第2个 tab")),
ListTile(title: Text("这是第2个 tab"))
],
),
ListView(
children: <Widget>[
ListTile(title: Text("这是第3个 tab")),
ListTile(title: Text("这是第3个 tab")),
ListTile(title: Text("这是第3个 tab"))
],
),
ListView(
children: <Widget>[
ListTile(title: Text("这是第4个 tab")),
ListTile(title: Text("这是第4个 tab")),
ListTile(title: Text("这是第4个 tab"))
],
),
],
),
),
);
}
}
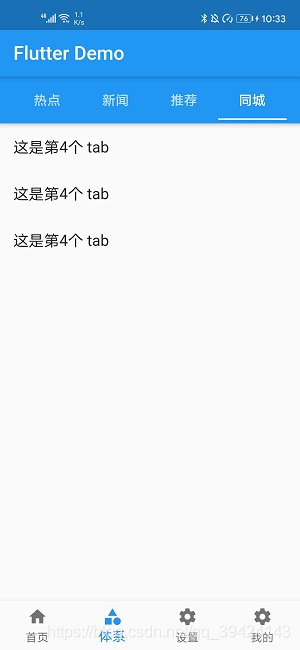
通过TabController实现TabLayout
import 'package:flutter/material.dart';
class TabbarControllerPage extends StatefulWidget {
TabbarControllerPage({Key key}) : super(key: key);
_TabbarControllerPageState createState() => _TabbarControllerPageState();
}
class _TabbarControllerPageState extends State<TabbarControllerPage>
with SingleTickerProviderStateMixin {
TabController _tabController;
// 生命周期函数,销毁时取消订阅,类似Rx
@override
void dispose() {
_tabController.dispose();
super.dispose();
}
// 生命周期函数, 加载即触发
@override
void initState() {
super.initState();
_tabController = new TabController(
vsync: this,
length: 3);
// 接受监听
_tabController.addListener((){
print(_tabController.index);
});
}
@override
Widget build(BuildContext context) {
return new Scaffold(
appBar: new AppBar(
title: new Text('顶部 tab 切换'),
bottom: new TabBar(
controller: _tabController, // 使用TabbarController必须加,相当于设置监听
tabs: <Widget>[
Tab(
text: "热点",
),
Tab(
text: "新闻",
),
Tab(
text: "推荐",
),
],
),
),
body: new TabBarView(
controller: _tabController,
children: <Widget>[
new Center(child: new Text('热点')),
new Center(child: new Text('新闻')),
new Center(child: new Text('推荐')),
],
),
);
}
}
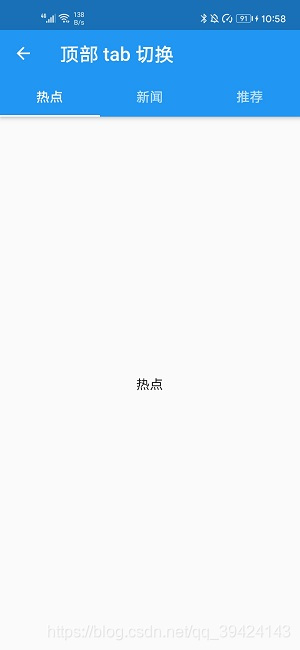