#include<iostream>
using namespace std;
typedef struct Node {
int data;
struct Node* pNext;
}Node,*linkList;
Node* creatList(int length);
bool showList(linkList);
bool emptyList(linkList);
int length(linkList);
bool insertList(linkList head, int position, int data);
bool deleteList(linkList head, int& value, int position);
Node* findList(linkList, int);
bool tranves(linkList);
int main()
{
Node* head = nullptr;
int length;
int value;
cout << "***************************************************\n";
printf("请输入所需要构造链表的长度:");
scanf_s("%d", &length);
cout << endl;
head = creatList(length);
printf("输出单链表的数据:\n");
showList(head);
cout << "***************************************************\n";
printf("对单链表进行插入操作:\n");
insertList(head, 2, 3);
printf("输出单链表的数据:\n");
showList(head);
cout << "***************************************************\n";
Node* node = findList(head, 2);
if (node != nullptr) {
printf("打印链表结点值%d", node->data);
}
else {
printf("链表元素查找失败");
}
printf("删除结点之后单链表的数据:\n");
deleteList(head, value, 2);
showList(head);
cout << "***************************************************\n";
tranves(head);
printf("翻转链表之后单链表的数据:\n");
showList(head);
cout << "***************************************************\n";
return 0;
}
bool tranves(linkList head) {
if (head == nullptr)
return false;
Node* p = head->pNext;
Node* temp = p->pNext;
Node* a = temp;
p->pNext = nullptr;
while (a != nullptr) {
a = a->pNext;
temp->pNext = p;
head->pNext = temp;
p = temp;
temp = a;
}
return true;
}
Node* findList(linkList head, int position) {
if (head == nullptr) {
printf("链表为空!!!\n");
return nullptr;
}
Node* node = head->pNext;
int i = 0;
if (position < 0) {
printf("删除位置有误!!!\n");
return nullptr;
}
while (i < position - 1 && node != nullptr) {
i++;
node = node->pNext;
}
return node;
}
bool deleteList(linkList head, int& value, int position) {
if (head == nullptr) {
printf("链表为空!!!\n");
return false;
}
Node* node = head->pNext;
int i = 0;
if (position < 0) {
printf("删除位置有误!!!\n");
return false;
}
while (i < position -1 && node != nullptr) {
i++;
node = node->pNext;
}
Node* q = node->pNext;
value = q->data;
node->pNext = q->pNext;
delete q;
return true;
}
bool insertList(linkList head, int position, int data) {
if (head == nullptr){
printf("链表为空!!!\n");
return false;
}
Node* node = head->pNext;
int i = 0;
int len = length(head);
if (position < 0 || position>len) {
printf("插入位置有误!!!\n");
return false;
}
while (i < position - 1 && node != nullptr) {
i++;
node = node->pNext;
}
Node* newNode = new Node;
newNode->data = data;
newNode->pNext = node->pNext;
node->pNext = newNode;
return true;
}
int length(linkList head) {
Node* node = head->pNext;
int length = 0;
while (node != nullptr) {
length++;
node = node->pNext;
}
return length;
}
bool emptyList(linkList head) {
return head == nullptr;
}
bool showList(linkList head) {
if (head == nullptr)
return false;
Node* node = head->pNext;
int i = 0;
while (node != nullptr) {
printf("第%d结点的数据为%d\n", i++, node->data);
node = node->pNext;
}
return true;
}
Node* creatList(int length) {
Node* head = new Node;
head->pNext = nullptr;
if (head == nullptr) {
printf("结点创建失败!!!");
exit(-1);
}
int data;
Node* temp = head;
for (int i = 0; i < length; i++) {
Node* node = new Node;
if (head == nullptr) {
printf("结点创建失败!!!");
exit(-1);
}
printf("请输入结点的数据:");
scanf_s("%d", &data);
node->data = data;
temp->pNext = node;
temp = node;
temp->pNext = nullptr;
}
return head;
}
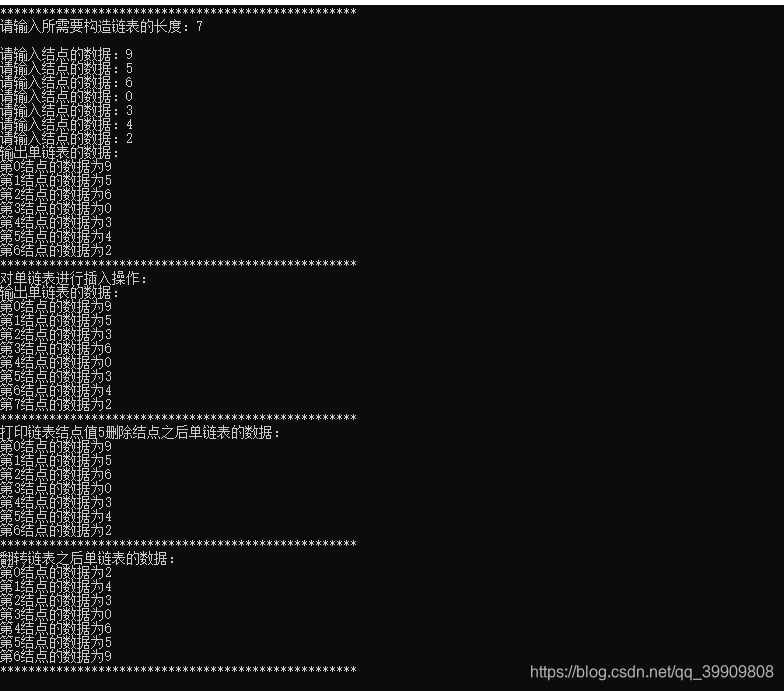