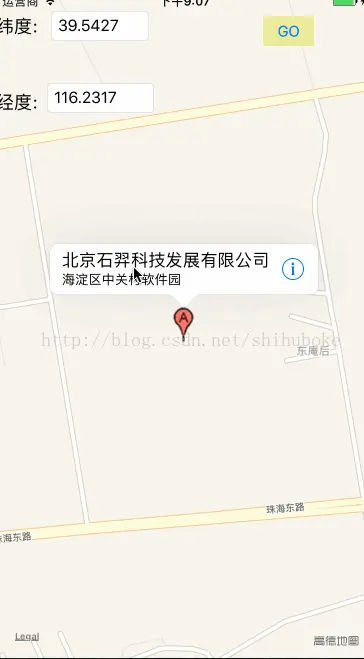
#import "ViewController.h"
#import <MapKit/MapKit.h>//地图
#import <CoreLocation/CoreLocation.h>//定位
@interface ViewController () <MKMapViewDelegate>
- (IBAction)goClicked:(id)sender;
@property (strong,nonatomic) IBOutletUITextField *latitudeField;
@property (strong,nonatomic) IBOutletUITextField *longitudeField;
@property (strong,nonatomic) IBOutletMKMapView *mapView;
@property (strong,nonatomic) CLGeocoder *geocoder;
@end
@implementation ViewController
- (void)viewDidLoad{
[superviewDidLoad];
_geocoder = [[CLGeocoder alloc] init];
self.mapView.mapType = MKMapTypeStandard;
self.mapView.zoomEnabled =YES;
self.mapView.scrollEnabled =YES;
self.mapView.rotateEnabled =YES;
self.mapView.showsUserLocation = YES;
[self locateToLatitude:37.23longitude:122.1234];
UILongPressGestureRecognizer *gesture = [[UILongPressGestureRecognizer
alloc]initWithTarget:self action:@selector(longPress:)];
[self.view addGestureRecognizer:gesture];
self.mapView.delegate =self;
}
#pragma mark --手势回调
- (void) longPress:(UILongPressGestureRecognizer*)gesture{
CGPoint pos = [gesture locationInView:self.mapView];
CLLocationCoordinate2D coord = [self.mapViewconvertPoint:pos
toCoordinateFromView:self.mapView];
CLLocation *location = [[CLLocation alloc] initWithLatitude:coord.latitude
longitude:coord.longitude];
[_geocoder reverseGeocodeLocation:location completionHandler:
^(NSArray *placemarks,NSError *error)
{
if (placemarks.count >0 && error == nil)
{
CLPlacemark *placemark = [placemarks objectAtIndex:0];
NSArray *addrArray = placemark
.addressDictionary[@"FormattedAddressLines"];
NSMutableString *address = [[NSMutableString alloc] init];
for(int i =0 ; i < addrArray.count ; i ++)
{
[address appendString:addrArray[i]];
}
MKPointAnnotation *annotation = [[MKPointAnnotation alloc] init];
annotation.title = placemark.name;
annotation.subtitle = address;
annotation.coordinate = coord;
[self.mapView addAnnotation:annotation];
}
}];
}
#pragma mark -点击回到输入的的经纬度位置
- (IBAction)goClicked:(id)sender{
[self.latitudeField resignFirstResponder];
[self.longitudeField resignFirstResponder];
NSString *latitudeStr =self.latitudeField.text;
NSString *longtitudeStr =self.longitudeField.text;
if (latitudeStr !=nil && latitudeStr.length >0
&& longtitudeStr != nil && longtitudeStr.length >0)
{
[self locateToLatitude:latitudeStr.floatValue
longitude:longtitudeStr.floatValue];
}
}
#pragma mark --自定义封装定位方法
- (void)locateToLatitude:(CGFloat)latitude longitude:(CGFloat)longitude{
CLLocationCoordinate2D center = {latitude , longitude};
MKCoordinateSpan span;
span.latitudeDelta =0.01;
span.longitudeDelta =0.01;
MKCoordinateRegion region = {center,span};
[self.mapViewsetRegion:region animated:YES];
MKPointAnnotation *annotation = [[MKPointAnnotation alloc] init];
annotation.title =@"北京石羿科技发展有限公司";
annotation.subtitle =@"海淀区中关村软件园";
CLLocationCoordinate2D coordinate = {latitude , longitude};
annotation.coordinate = coordinate;
[self.mapViewaddAnnotation:annotation];
}
#pragma mark -自定义锚点
- (MKAnnotationView *) mapView:(MKMapView *)mapView
viewForAnnotation:(id <MKAnnotation>) annotation{
staticNSString *annoId = @"fkAnno";
MKAnnotationView *annoView = [mapView
dequeueReusableAnnotationViewWithIdentifier:annoId];
if (!annoView)
{
annoView= [[MKAnnotationView alloc] initWithAnnotation:annotation reuseIdentifier:annoId];
}
annoView.image = [UIImageimageNamed:@"pos.gif"];
annoView.canShowCallout =YES;
UIButton *button = [UIButton buttonWithType:UIButtonTypeDetailDisclosure];
[button addTarget:selfaction:@selector(buttonTapped:)
forControlEvents:UIControlEventTouchUpInside];
annoView.rightCalloutAccessoryView = button;
return annoView;
}
#pragma mark -自定义锚点 --里面的详情按钮
- (void) buttonTapped:(id)sender
{
NSLog(@"您点击了锚点信息!");
}
@end