依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.78</version>
</dependency>
自定义注解
import java.lang.annotation.*;
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface OperLog {
String title();
String operation();
}
切面
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONArray;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Pointcut;
import org.aspectj.lang.reflect.MethodSignature;
import org.springframework.stereotype.Component;
import org.springframework.web.context.request.RequestContextHolder;
import org.springframework.web.context.request.ServletRequestAttributes;
import javax.servlet.http.HttpServletRequest;
import java.lang.reflect.Method;
@Aspect
@Component
public class SysLogAspect {
@Pointcut("@annotation(com.dxy.demo_05_08.annotation.OperLog)")
public void logPointCut() {
}
@Around("logPointCut()")
public Object saveSysLog(ProceedingJoinPoint proceedingJoinPoint) {
try {
MethodSignature signature = (MethodSignature) proceedingJoinPoint.getSignature();
Method method = signature.getMethod();
OperLog myLog = method.getAnnotation(OperLog.class);
if (myLog != null) {
System.out.println(myLog.title()+","+myLog.operation());
}
String className = proceedingJoinPoint.getTarget().getClass().getName();
String methodName = method.getName();
System.out.println(className + "." + methodName+"()");
Object[] args = proceedingJoinPoint.getArgs();
System.out.println(args[0]);
String params = JSON.toJSONString(args);
JSONArray jsonArray = JSON.parseArray(params);
ServletRequestAttributes attributes = (ServletRequestAttributes) RequestContextHolder.getRequestAttributes();
HttpServletRequest request = attributes.getRequest();
Object user = request.getAttribute("user");
Object result = proceedingJoinPoint.proceed();
System.out.println(result);
return result;
} catch (Throwable e) {
}
return "";
}
}
controller
@OperLog(title = "账号密码登陆",operation="查询")
@RequestMapping(path = "/hello", method = {RequestMethod.GET, RequestMethod.POST})
public @ResponseBody
Object adduser(@RequestParam(defaultValue ="username") String t) {
return "hello";
}
截图
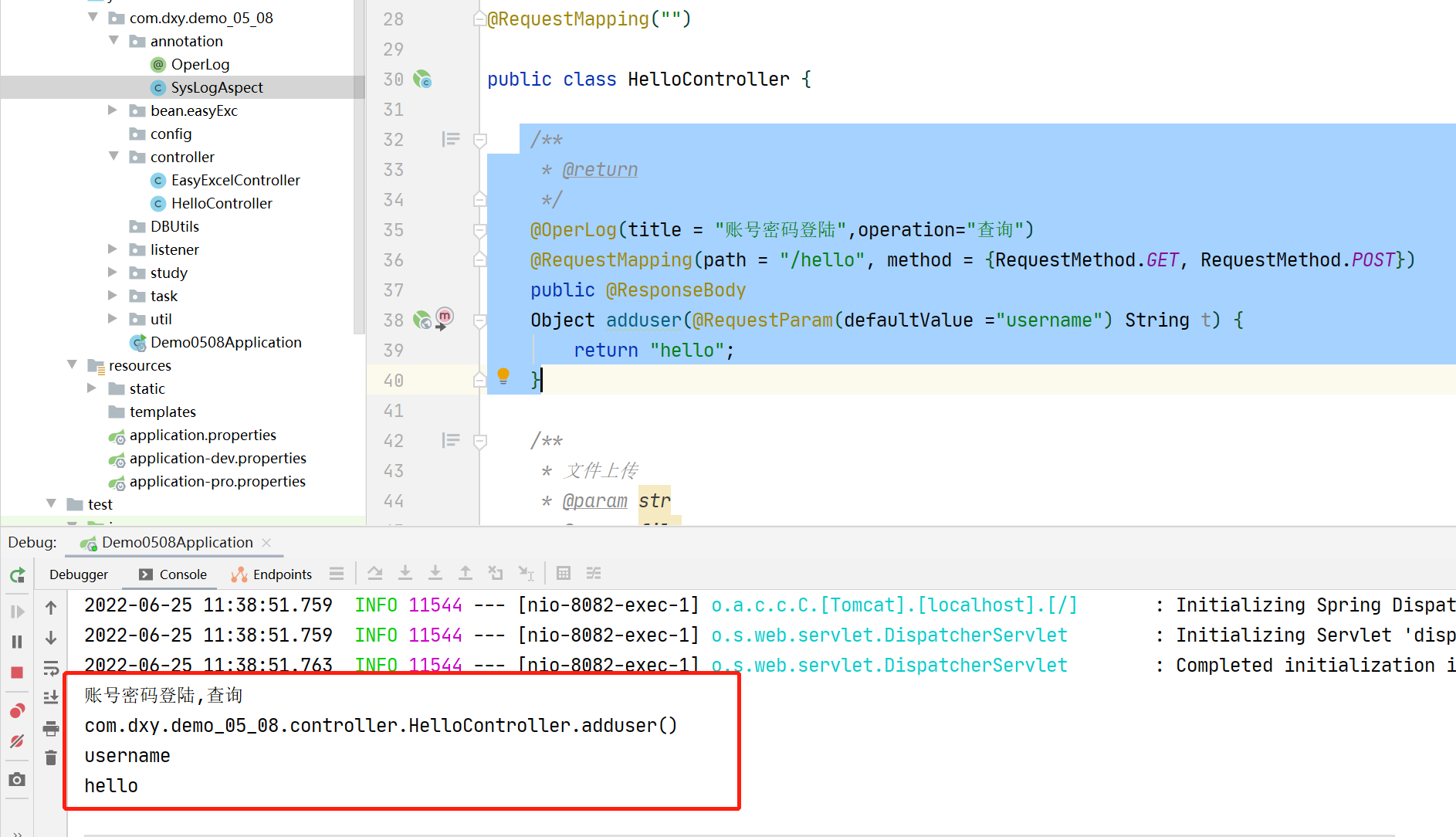
参考文章
https://www.jianshu.com/p/c2ae8a23f937