1. Description
-
Given head, the head of a linked list, determine if the linked list has a cycle in it.
There is a cycle in a linked list if there is some node in the list that can be reached again by continuously following the next pointer. Internally, pos is used to denote the index of the node that tail’s next pointer is connected to. Note that pos is not passed as a parameter.
Return true if there is a cycle in the linked list. Otherwise, return false. -
给定一个链表,判断链表中是否有环。
如果链表中有某个节点,可以通过连续跟踪 next 指针再次到达,则链表中存在环。 为了表示给定链表中的环,我们使用整数 pos 来表示链表尾连接到链表中的位置(索引从 0 开始)。 如果 pos 是 -1,则在该链表中没有环。注意:pos 不作为参数进行传递,仅仅是为了标识链表的实际情况。
如果链表中存在环,则返回 true 。 否则,返回 false 。
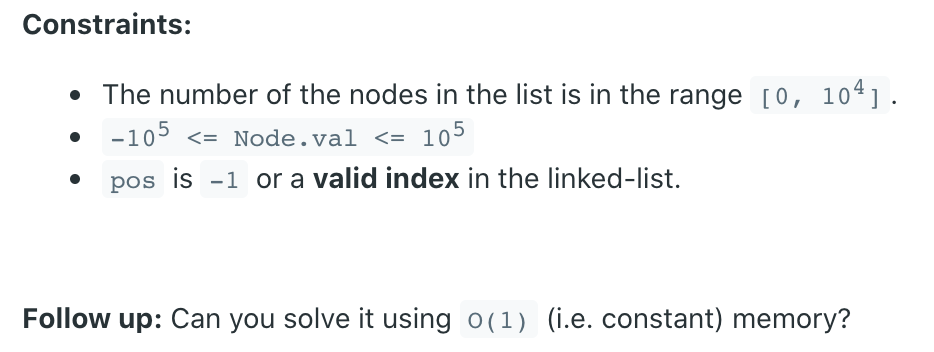
2. Analysis
采用双指针——快慢指针法,快指针每次移动两步,慢指针每次移动一步。如果存在环,则快指针最终将追上满指针,即fast == null
;若不存在环,则fast
指针会指向尾结点或null
。
3. Code
class Solution {
public boolean hasCycle(ListNode head) {
ListNode fast, slow;
fast = slow = head;
// while判断同时解决了corner case(单节点或空链表)
while(fast != null && fast.next != null){
slow = slow.next;
fast = fast.next.next;
if(slow == fast) return true;
}
return false;
}
}
进阶:如果已知链表中有环,要判断环开头的位置怎么办?
本题类似一道脑筋急转弯,可以先让快慢指针在环内相遇,再让快指针指向头节点后二者以相同速度移动至第二次相遇,此时二者所在位置一定是环的开头。
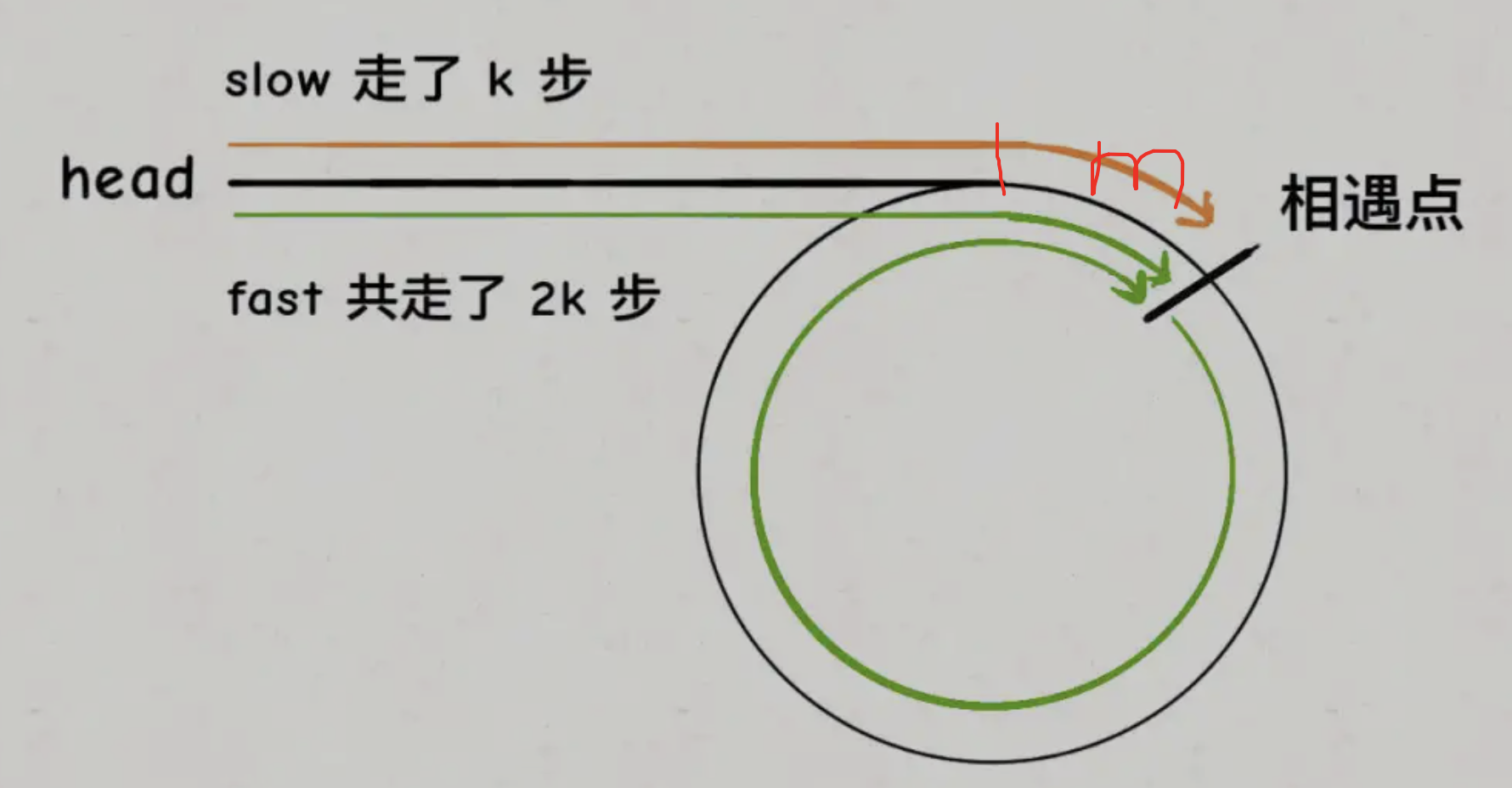
从上图可以看出,第一次相遇时,fast
比slow
多走了k
步,则k
一定是圈中节点数的整数倍。故第一次相遇后当fast
从head出发走k-m
步到环头时,slow
一定从相遇点走到了环头与fast
相遇。
ListNode detectCycle(ListNode head) {
ListNode fast, slow;
fast = slow = head;
while(fast != null && fast.next != null){
slow = slow.next;
fast = fast.next.next;
if(slow == fast) break;
}
fast = head;
while(fast != slow){
slow = slow.next;
fast = fast.next;
}
return fast;
}
4. Summary
快慢指针是双指针法的一种,是经常用到的一种操作技巧。
本文总结了快慢指针的常用场景——判断链表成环及判断环起点
本文参考:labuladong 双指针技巧