从b站学习springcloud,现在进行总结,该总结除去了视频中出现的小错误,对有些易错的地方进行了提醒
b站链接:https://www.bilibili.com/video/av55993157
资料链接:
https://pan.baidu.com/s/1o0Aju3IydKA15Vo1pP4z5w
提取码: 21ru
上一节链接:https://blog.csdn.net/qq_40893824/article/details/106872203
下面的内容总结:
不用自己写过滤器、拦截
类似上1节,新建工程 springSecurity
安全性
1 在 pom 文件中,加入代码:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.southwind</groupId>
<artifactId>springSecurity</artifactId>
<version>1.0-SNAPSHOT</version>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-parent</artifactId>
<version>2.1.6.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
</dependencies>
</project>
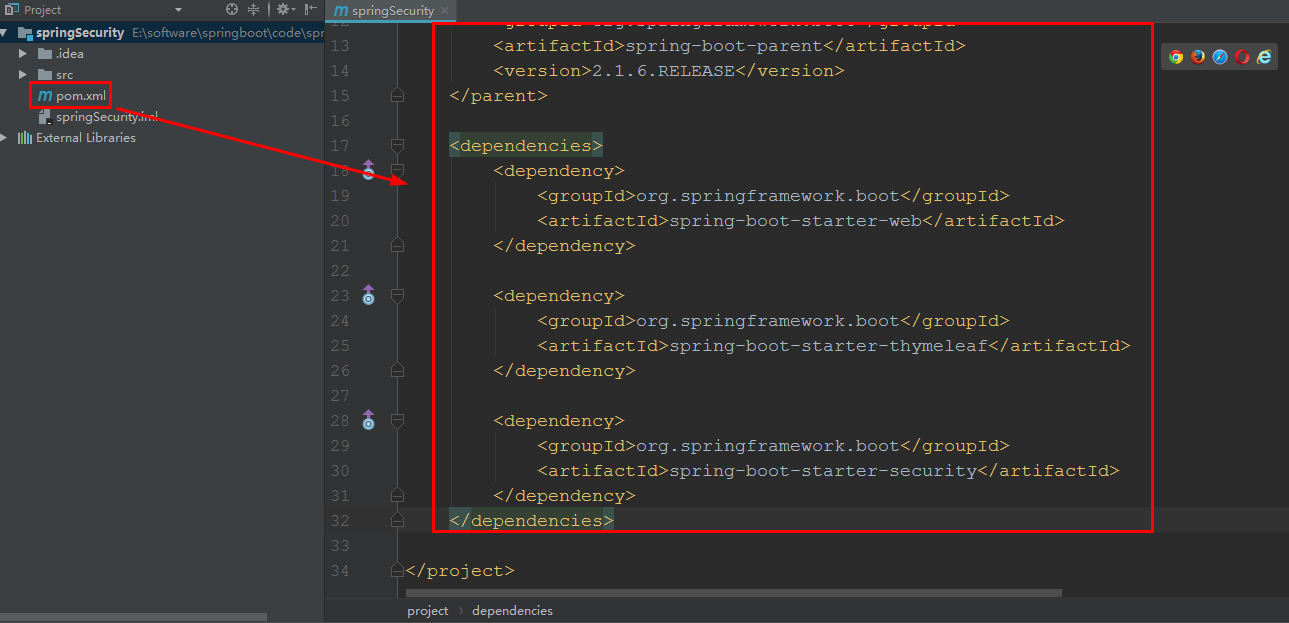
2 src\main\java 中创包 com.southwind.controller,其内创建 控制类 HelloHandler
package controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class HelloHandler {
@GetMapping("/index")
public String index(){
return "index";
}
}
@Controller:视图解析器能解析 return 的 jsp, html 页面
3 在 resources 中创建文件夹 templates
其内,创建 index.html,加入代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>hello world</h1>
</body>
</html>
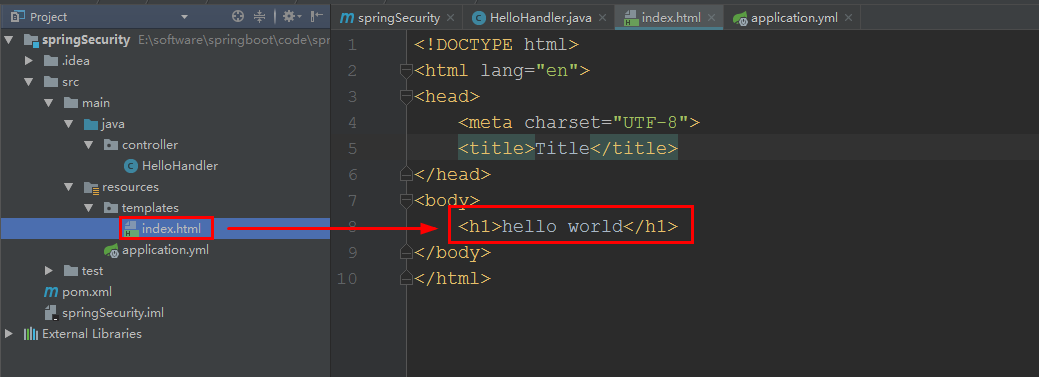
4 在 resources 中创建配置文件 application.yml,加入代码:
spring:
thymeleaf:
prefix: classpath:/templates/
suffix: .html
属于映射文件,classpath = 文件夹 resources
在控制类 handler 中,返回页面的名称,如 “index”,IOC 就会去 resources\templates 中寻找 index.html,找到就会返回!
5 在 southwind 中创建启动类 Application,加入代码:
package com.southwind;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class,args);
}
}
6 开启启动类 Application,进入 http://localhost:8080 或 http://localhost:8080/index 均是下面的情况:
这就是安全性了
它对应 pom 中的:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
默认用户名:user
密码随机生成,在控制台看:
7 在 application 中加入代码:(设置自定义密码)
security:
user:
name: admin
password: 123456
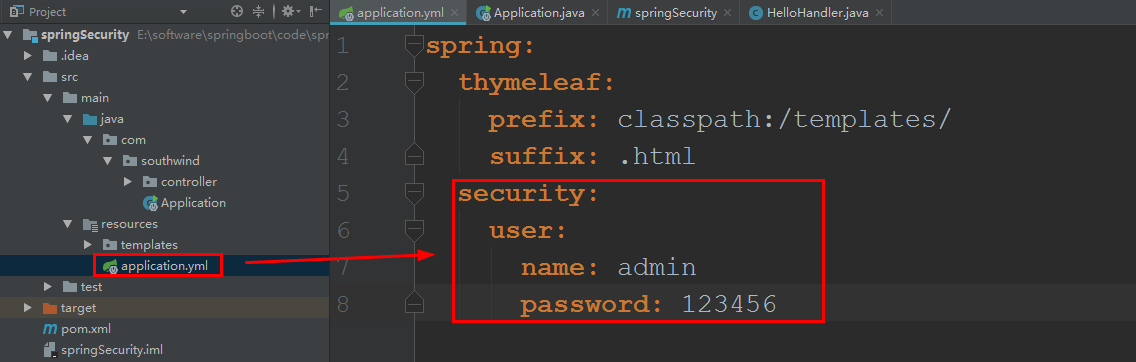
8 进入 http://localhost:8080/login 或 http://localhost:8080/index
填入 自定义的 用户名 和 密码
权限管理
定义 2 个 html 资源:index.html、admin.html
定义 2 个用户:ADMIN 和 USER
ADMIN 能访问 index.html 和 admin.html
USER 只能访问 index.html
9 在 southwind 中创包 config,其内创建类 MyPasswordEncoder,加入代码:
package com.southwind.config;
import org.springframework.security.crypto.password.PasswordEncoder;
public class MyPasswordEncoder implements PasswordEncoder {
public String encode(CharSequence rawPassword) {
return rawPassword.toString();
}
public boolean matches(CharSequence rawPassword, String encodedPassword) {
return encodedPassword.equals(rawPassword);
}
}
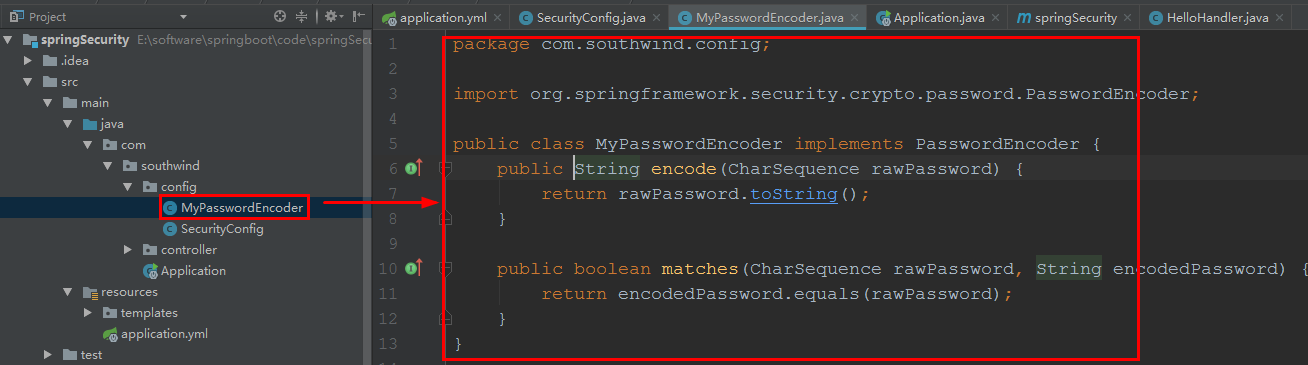
10 config 中创建类 SecurityConfig,加入代码:
2个均要实现
package com.southwind.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication().passwordEncoder(new MyPasswordEncoder())
.withUser("user")
.password(new MyPasswordEncoder().encode("000")).roles("USER")
.and()
.withUser("admin")
.password(new MyPasswordEncoder().encode("123")).roles("ADMIN","USER");
}
// 下面,权限验证
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin").hasRole("ADMIN")
.antMatchers("/index").access("hasRole('ADMIN') or hasRole('USER')")
.anyRequest().authenticated()
.and()
.formLogin().loginPage("/login")
.permitAll()//登录时,不要验证
.and()
.logout().permitAll()//退出时,不验证
.and()
.csrf().disable();//禁用一些东西
}
}
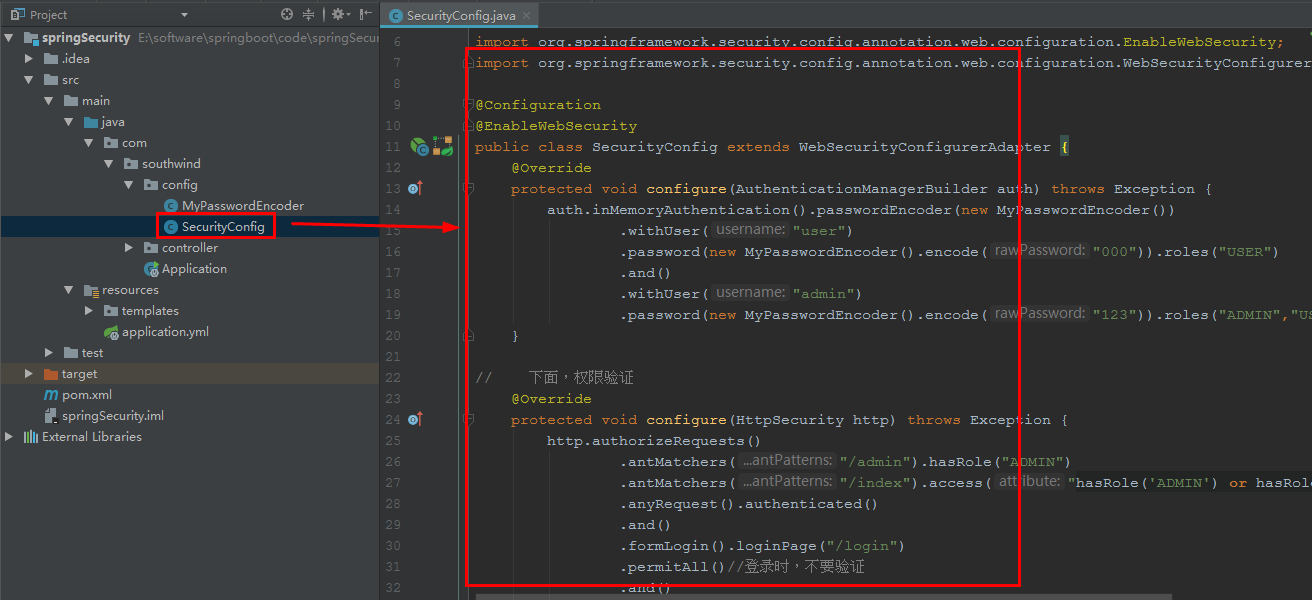
11 在 controller/ HelloHandler 中添加代码:
package com.southwind.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class HelloHandler {
@GetMapping("/index")
public String index(){
return "index";
}
@GetMapping("/admin")
public String admin(){
return "admin";
}
@GetMapping("/login")
public String login(){
return "login";
}
}
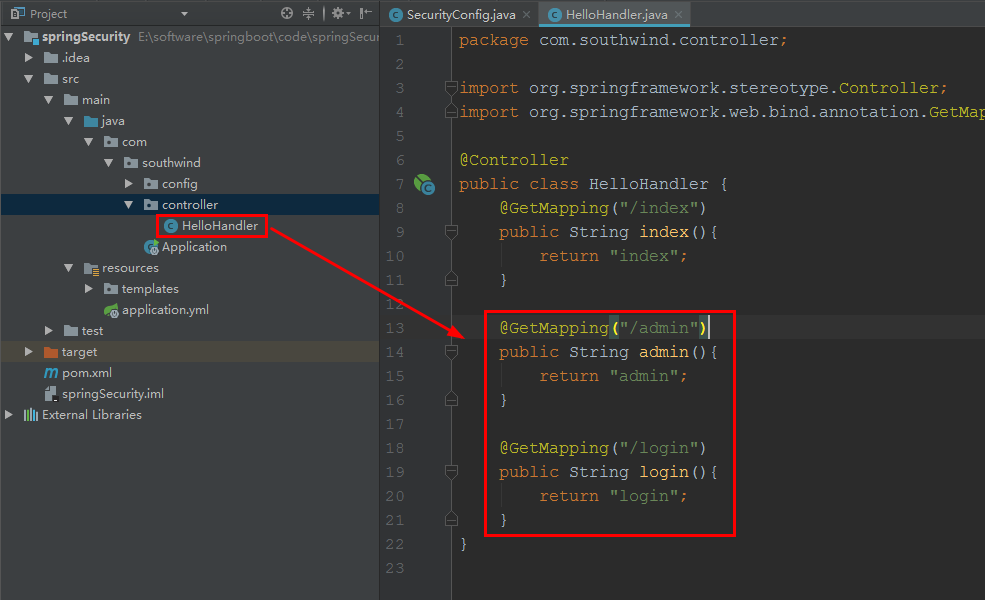
12 在 templates 中新建 login.html,加入代码:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org"></html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<form th:action="@{/login}" method="post">
用户名:<input type="text" name="username" /><br/>
密码:<input type="text" name="password" /><br/>
<input type="submit" value="登录"/>
</form>
</body>
</html>
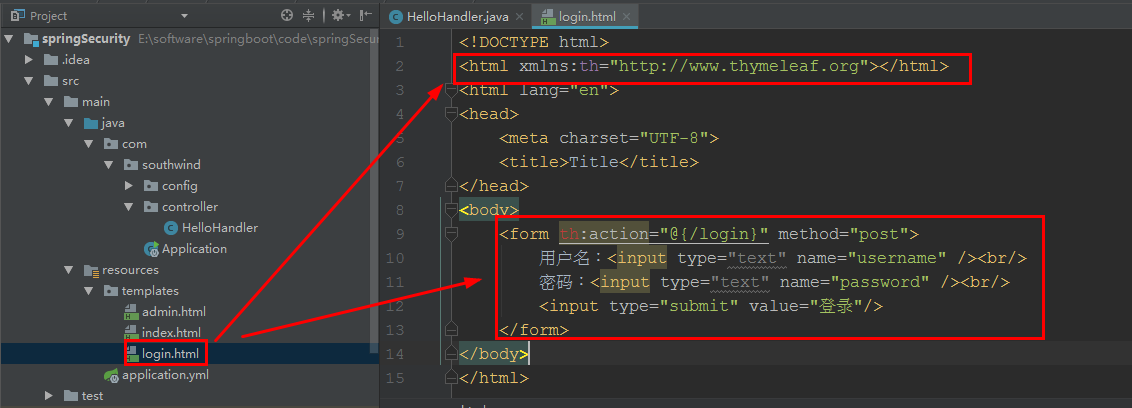
13 在 index.html 中加入代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>hello world</h1>
<form action="/logout" method="post">
<input type="submit" value="退出">
</form>
</body>
</html>
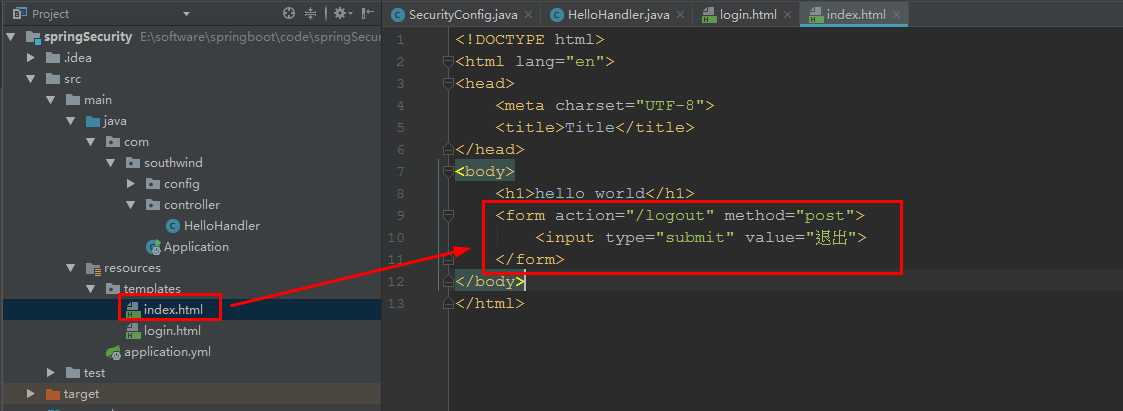
14 在 templates 中新建 admin.html,加入代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>后台管理</h1>
<form action="logout" method="post">
<input type="submit" value="退出">
</form>
</body>
</html>
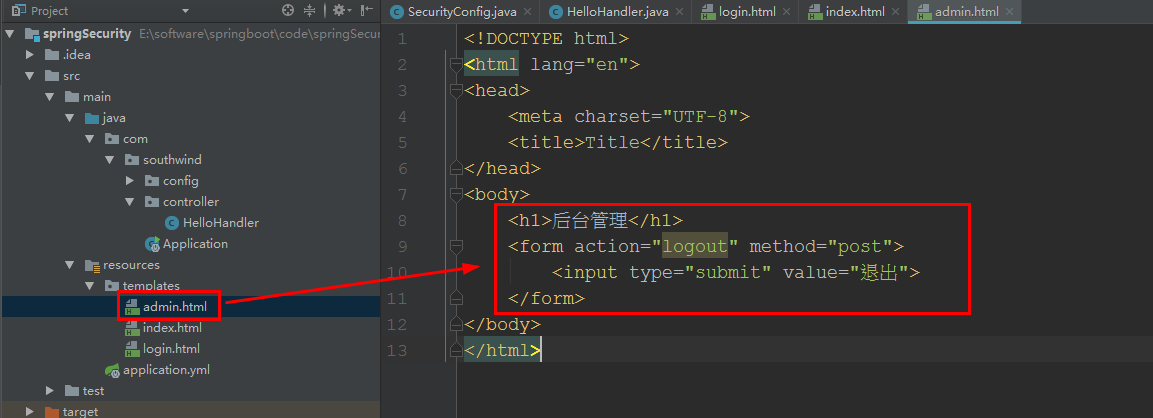
15 开启 启动类 Application
15.1 user:
进入 http://localhost:8080/index
user 可以进入 index.html,退出
进入 http://localhost:8080/admin
说明 user 仅能访问 index.html,不能访问 admin.html
15.2 admin:
进入 http://localhost:8080/index
admin 可以访问 index.html
进入 http://localhost:8080/admin
admin 可以访问 index.html、admin.html
上一节链接:https://blog.csdn.net/qq_40893824/article/details/106872203