来源:https://www.bilibili.com/video/BV1GE411d7KE?p=2
上一节:https://blog.csdn.net/qq_40893824/article/details/107041897
下一节:https://blog.csdn.net/qq_40893824/article/details/107154193
相关参数
@Controller | 类 交给 IoC 容器来管理 |
---|
@RequestMapping | 将 URL 网址请求 与 业务方法 映射 用在 handler 控制类里 |
---|---|
value | @RequestMapping("/index") = @RequestMapping(value = "/index") |
method | GET, POST, PUT, DELETE HEAD, OPTIONS, PATCH, TRACE @RequestMapping(value = "/index",method = RequestMethod.GET) 表示 该方法只能接收 GET 请求 ![]() ![]() |
params | 指定 网址请求中 必须包含某些参数@RequestMapping(value = "/index",method = RequestMethod.GET,params = {"name","id"}) @RequestMapping(value = "/index",method = RequestMethod.GET,params = {"name","id=10"}) "id=1" 不能有空格 请求中必须包含 name 和 id 两个参数,id 的值必须是 10 |
@RequestMapping(value = "/index",method = RequestMethod.GET, params = {"name","id=1"})
public String index(String name, int id){
System.out.println(name);
System.out.println(id);
System.out.println("执行了index...");
return "index";
}
重启 tmcat services
进入 http://localhost:8080/hello/index?name=zhangsan&id=1
@RequestParam 可以使方法参数 的名字修改:
@RequestMapping(value = "/index",method = RequestMethod.GET, params = {"name","id=1"})
public String index(@RequestParam("name") String str, @RequestParam("id") int age){
System.out.println(str);
System.out.println(age);
System.out.println("执行了index...");
return "index";
}
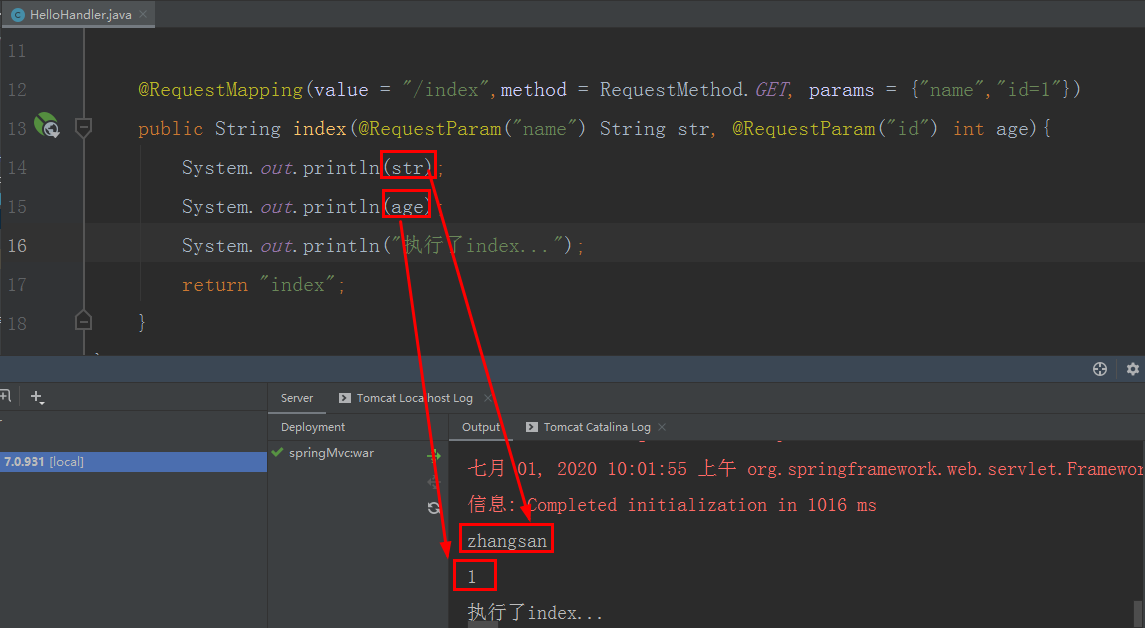
Spring MVC 支持 RESTful 风格的 URL
传统类型:http://localhost:8080/hello/index?name=zhangsan&id=10
REST:http://localhost:8080/hello/index/zhangsan/10
REST 用到 @PathVariable 映射网址参数
@RequestMapping("/rest/{name}/{id}")
public String rest(@PathVariable("name") String str, @PathVariable("id") int age){
System.out.println(str);
System.out.println(age);
return "index";
}
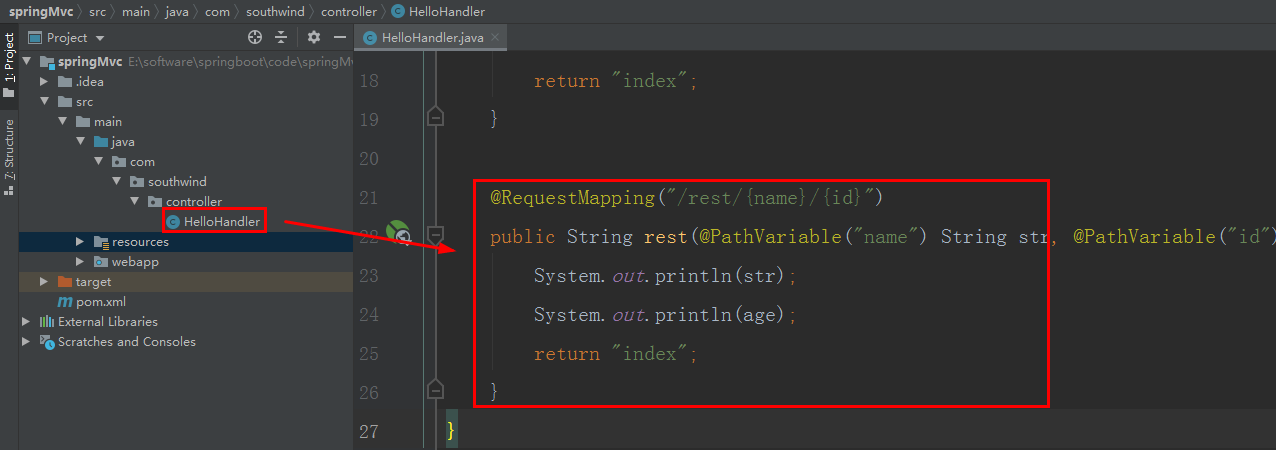
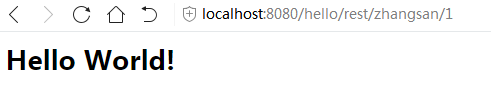
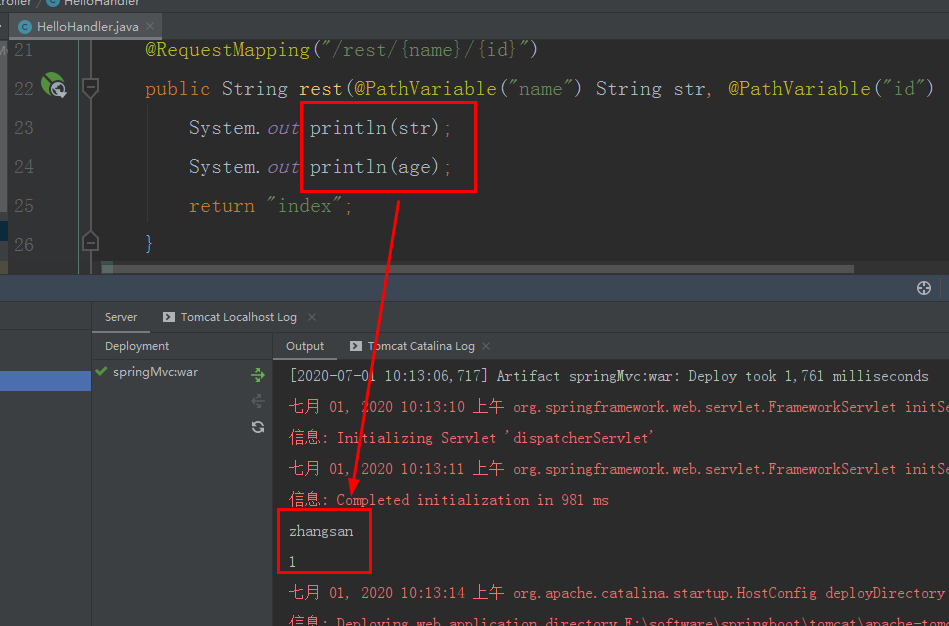
映射 Cookie
HelloHandler 中,加入代码:
@RequestMapping("/cookie")
public String cookie(@CookieValue(value = "JSESSIONID") String sessionId){
System.out.println(sessionId);
return "index";
}
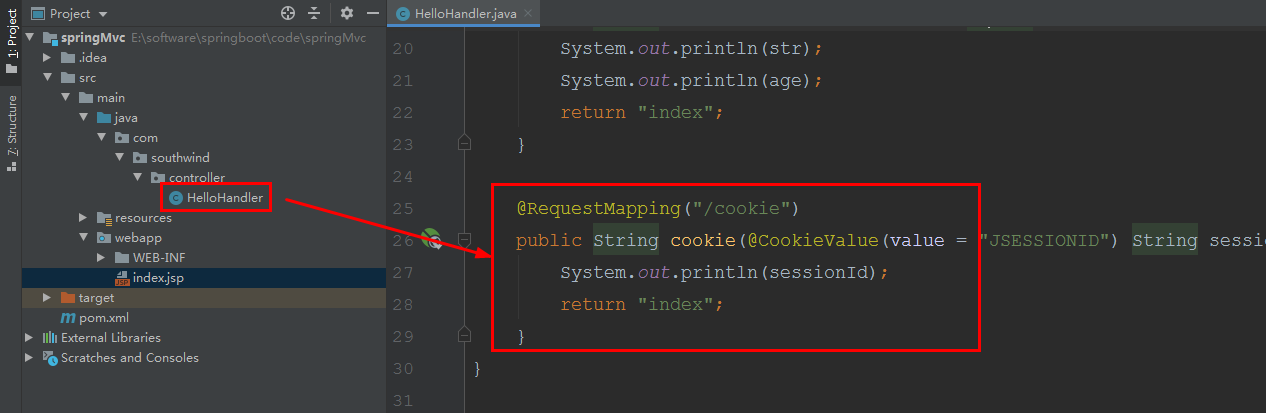
进入 http://localhost:8080/hello/cookie
前提是 网页之前要进入过 http://localhost:8080/hello/rest/zhangs/23
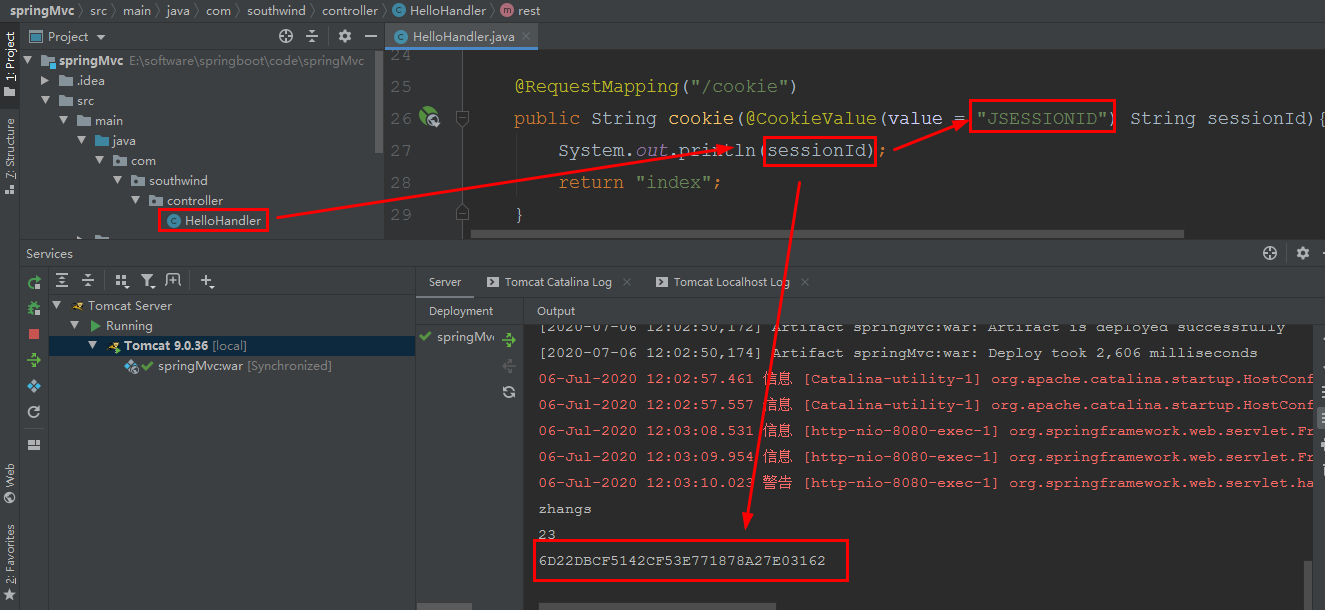
JavaBean 绑定参数
1 pom 文件中,添加代码:
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.12</version>
<scope>provided</scope>
</dependency>
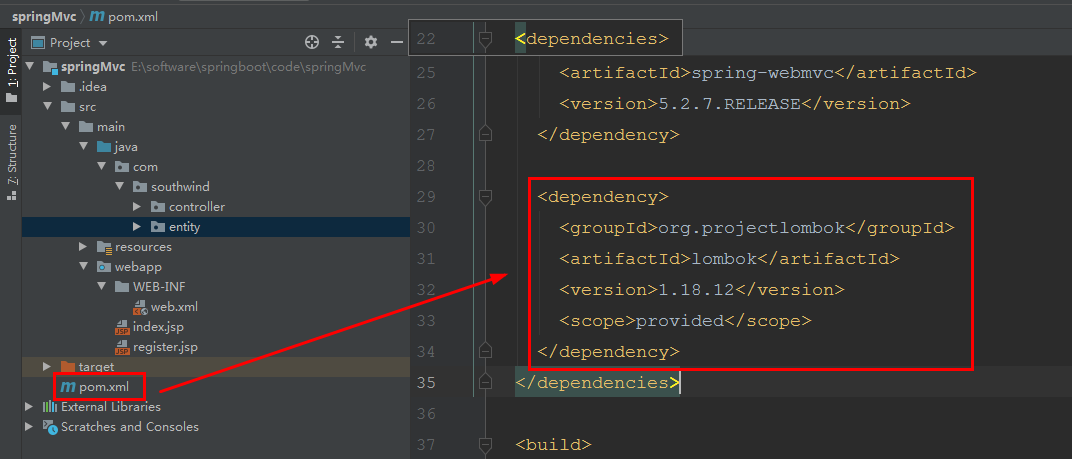
2 southwind 中,创包 entity,其内 创建实体类 User,加入代码:
package com.southwind.entity;
import lombok.Data;
@Data
public class User {
private long id;
private String name;
}
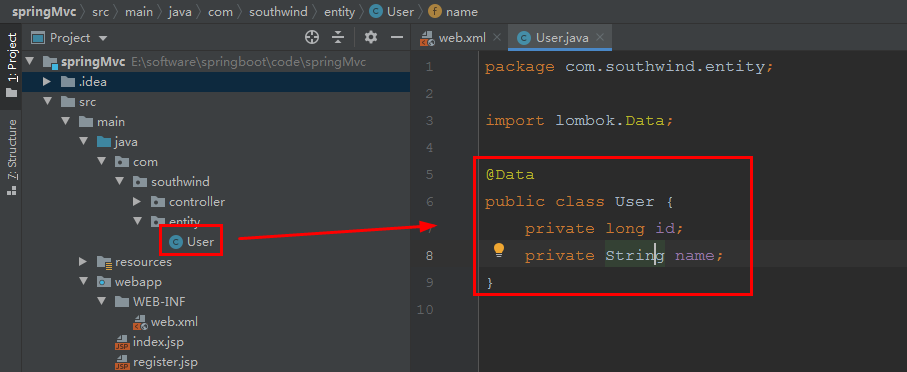
3 HelloHandler 中,添加代码:
@RequestMapping(value = "/save" ,method = RequestMethod.POST)
public String save(User user){
System.out.println(user);
return "index";
}
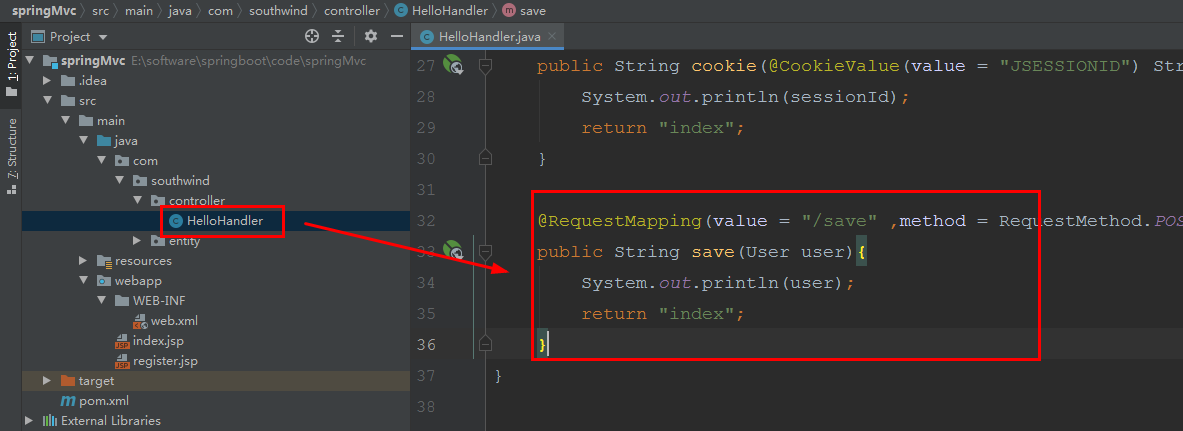
4 webapp 中,创建 register.jsp,加入代码:
<form action="/hello/save" method="post">
用户id:<input type="text" name="id"/><br/>
用户名:<input type="text" name="name"/><br/>
<input type="submit" value="注册">
</form>
name 映射 对应属性
5 进入 http://localhost:8080/register.jsp
中文乱码,解决:
WEB-INF/ web.xml 中,添加代码:
<filter>
<filter-name>encodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
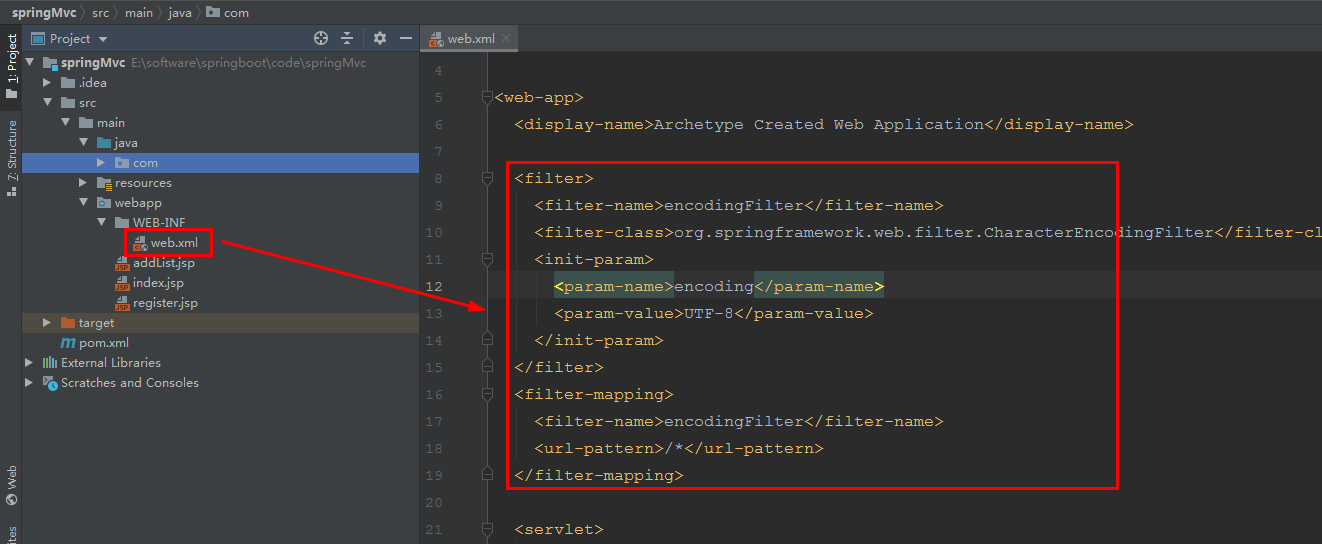
重启 tomcat
进入 http://localhost:8080/register.jsp
依然中文乱码的,看:
https://blog.csdn.net/qq_40893824/article/details/107080857
级联
1 entity 中,新建实体类,加入代码:
package com.southwind.entity;
import lombok.Data;
@Data
public class Address {
private String value;
}
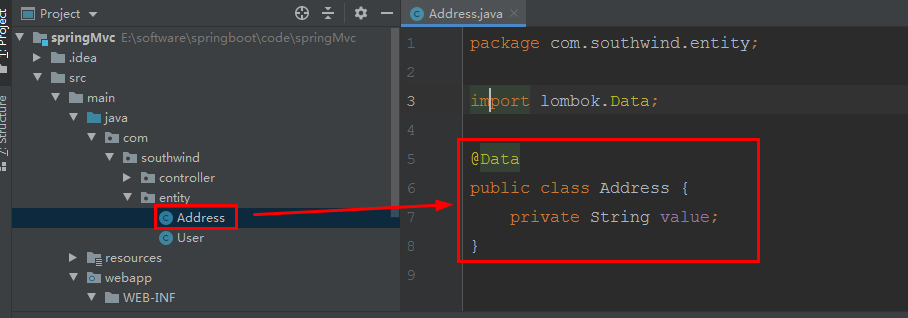
2 User 中,添加代码:private Address address;
3 register.jsp 中,添加代码:用户地址:<input type="text" name="address.value"/><br/>
4 重启 tomcat
进入 http://localhost:8080/register.jsp
转发、重定向
转发:默认,服务端行为,浏览器地址不变,转发路径必须是同一个 web 容器下的 url
重定向:客户端行为,浏览器地址会变
转发:
1 HelloHandler 中,添加代码:
@RequestMapping("/forward")
public String forward(){
return "forward:/index.jsp";
// return "index"; //两者效果一样
}
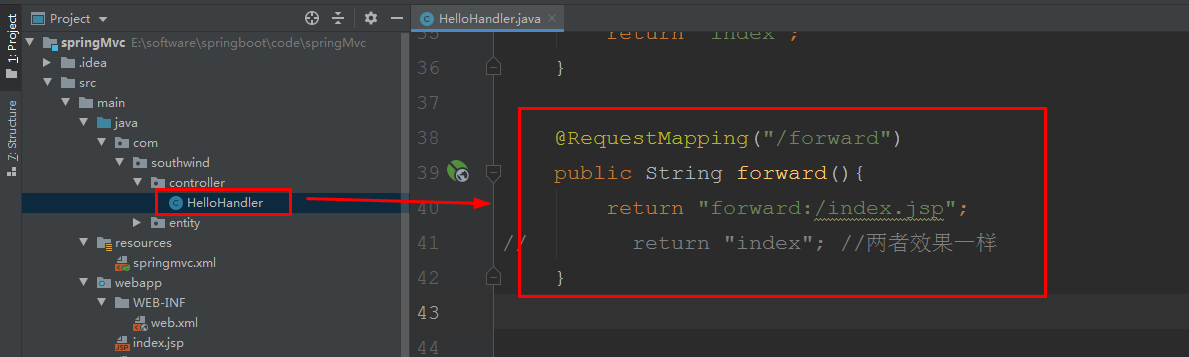
2 重启 tomcat,进入 http://localhost:8080/hello/forward
这是 index.jsp 的内容,但网页地址没变,这是转发
重定向:
1 HelloHandler 中,添加代码:
@RequestMapping("/redirect")
public String redirect(){
return "redirect:/index.jsp";
}
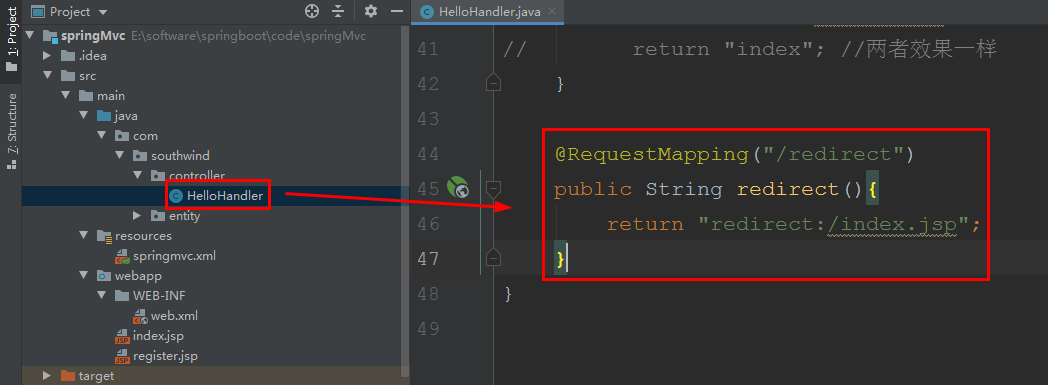
2 重启 tomcat,进入 http://localhost:8080/hello/redirect
这是 index.jsp 的内容,但网页地址变了,这是重定向
上一节:https://blog.csdn.net/qq_40893824/article/details/107041897
下一节:https://blog.csdn.net/qq_40893824/article/details/107154193