https://www.nowcoder.com/tutorial/94/ae05554a3ad84e42b6f9fc4d52859dc4
https://how2j.cn/k/class-object/class-object-class-attribute/296.html#nowhere
目录
Object类
Object类是所有类的父类
其常用方法:
对象名.toString
返回类的字符串形式,需要自己重写,否则返回的是对象的地址
boolean i = 对象名1.equals(对象名2)
两对象是否一样,而不是单值是否相等
Class 对象名.getClass()
返回对象所属类
对象名.hashCode()
:返回其所在对象的物理地址(哈希码值)
1 引用
变量类型是 类,该变量叫 “引用”
java 默认会有无参构造
package 第1个程序.引用;
public class Hero {
String name;
float hp; //血量
float armor; //护甲
int moveSprrd; //速度
public static void main(String[] args) {
// 创建1个对象
new Hero();
// 引用h 指向这个对象
Hero h = new Hero();
}
}
1.1 多引用,1个对象
package 第1个程序.引用;
//多引用,1个对象
public class Hero1 {
String name;
float hp; //血量
float armor; //护甲
int moveSprrd; //速度
public static void main(String[] args) {
Hero1 h1 = new Hero1();
Hero1 h2 = h1;
Hero1 h3 = h1;
Hero1 h4 = h1;
Hero1 h5 = h1;
}
}
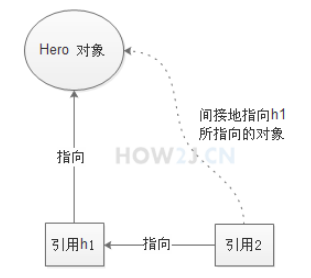
1.2 单引用,多对象
package 第1个程序.引用;
//单引用,多对象
public class Hero2 {
String name;
float hp; //血量
float armor; //护甲
int moveSprrd; //速度
public static void main(String[] args) {
Hero2 h1 = new Hero2();
h1 = new Hero2();
}
}
此时,h1 仅指向1个对象,就没指向第1个对象了!
2 继承
不能从别的包中继承类。。。
可以用 import 解决
import 第1个程序.a2_引用.Hero;
package 第1个程序.a3_继承;
// 物品
public class Item {
String name;
int price;
}
package 第1个程序.a3_继承;
public class Weapon extends Item{
int damage; // 攻击力
public static void main(String[] args) {
Weapon w1 = new Weapon();
w1.name = "无尽之刃";
w1.price = 3600;
w1.damage = 65;
}
}
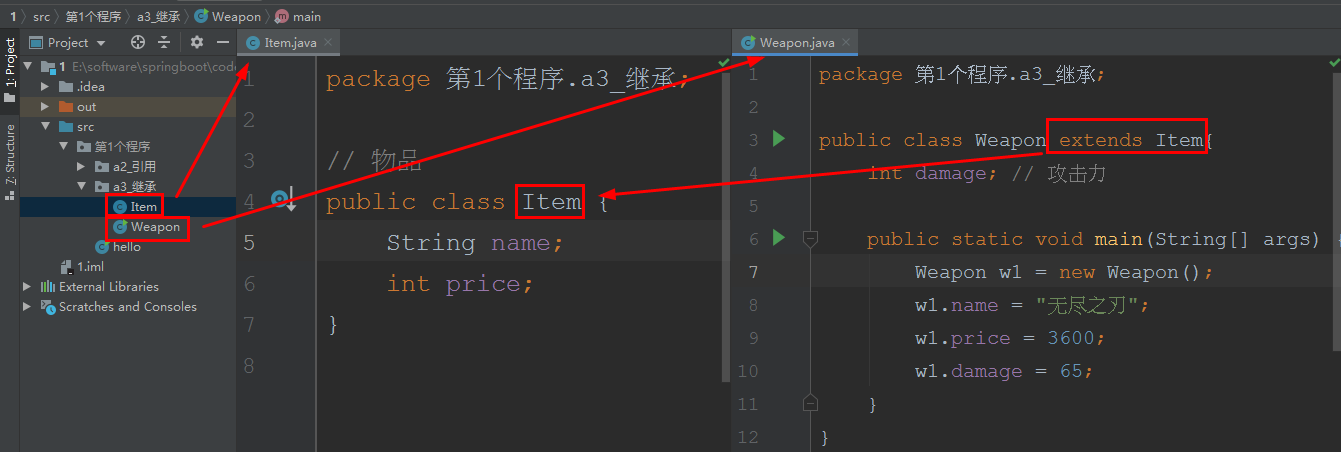
设计1个类 Armor (护甲类)
继承 Item,提供属性 ac (int): 护甲值
实例化 2 件护甲
名称 价格 护甲等级
布甲 200 15
锁子甲 500 40
package 第1个程序.a3_继承;
public class Armor extends Item{
int ac; //护甲值
public static void main(String[] args) {
Armor a1 = new Armor();
a1.name = "布甲";
a1.price = 200;
a1.ac = 15;
Armor a2 = new Armor();
a2.name = "锁子甲";
a2.price = 800;
a2.ac = 40;
}
}
3 重载
重载:方法名一样,参数类型不同
package 第1个程序.a3_重载;
public class ADHero extends Hero {
public void attack(){
System.out.println(name + "进行了1次攻击,但不确定打中了谁");
}
public void attack(Hero h1){
System.out.println(name + "对 " + h1.name + "进行了1次攻击");
}
public void attack(Hero h1, Hero h2){
System.out.println(name + "对 " + h1.name + "和" + h2.name + "进行了1次攻击");
}
public static void main(String[] args) {
ADHero h = new ADHero();
h.name = "赏金猎人";
Hero h1 = new Hero();
h1.name = "盖伦";
Hero h2 = new Hero();
h2.name = "提莫";
h.attack(h1);
h.attack(h1,h2);
}
}
3.1 参数可变
public void attack(Hero... heroes){
for(int i = 0; i < heroes.length ; i++){
System.out.println(name + "对 " + heroes[i].name + "进行了1次攻击");
}
}
3.2 练习
设计类Support (辅助英雄)继承Hero
提供 heal(治疗)方法
heal方法可重载:
heal()
heal(Hero h) //为指定的英雄加血
heal(Hero h, int hp) //为指定的英雄加了hp的血
package 第1个程序.a3_重载;
public class Support extends Hero{
public void heal(){
System.out.println(name + "不知对谁奶了一口");
}
public void heal(Hero h1){
System.out.println(name + "奶了一口" + h1.name);
}
public void heal(Hero h1, float hp){
System.out.println(name + "奶了一口" + h1.name + ",加血" + hp);
}
public static void main(String[] args) {
Support support = new Support();
support.name = "辅助";
Hero h1 = new Hero();
h1.name = "盖伦";
Hero h2 = new Hero();
h2.name = "提莫";
support.heal();
support.heal(h1);
support.heal(h1,100);
}
}
4 this super
小明说:“我吃了” ,“我” 是小明
小红说:“我吃了” ,“我” 是小红
"我"代表当前人物
this = “我”
this 代表当前对象
super 其父类对象
this 仅在构造函数中能用
package 第1个程序.a4_this;
public class Hero {
String name;
float hp; //血量
float armor; //护甲
int moveSprrd; //速度
public void show(){
System.out.println("该 this虚拟地址 = " + this);
}
public static void main(String[] args) {
Hero h1 = new Hero();
h1.name = "盖伦";
System.out.println("打印看到的对象虚拟地址 = " + h1);
h1.show();
System.out.println();
Hero h2 = new Hero();
h2.name = "提莫";
System.out.println("打印看到的对象虚拟地址 = " + h2);
h2.show();
}
}
4.1 赋值
package 第1个程序.a4_this;
public class Hero2 {
String name;
float hp; //血量
float armor; //护甲
int moveSprrd; //速度
public void setName(String heroname){
name = heroname;
}
public void setName2(String name){
this.name = name;
}
public static void main(String[] args) {
Hero2 h1 = new Hero2();
h1.setName("盖伦");
System.out.println(h1.name);
Hero2 h2 = new Hero2();
h2.setName("提莫");
System.out.println(h2.name);
}
}
4.2 调用方法
package 第1个程序.a4_this;
// this调用方法
public class Hero3 {
String name;
float hp; //血量
float armor; //护甲
int moveSprrd; //速度
public Hero3(String name){
System.out.println("1个参数的构造方法");
this.name = name;
}
public Hero3(String name, float hp){
this(name);
System.out.println("2参数的构造方法");
this.hp = hp;
}
public static void main(String[] args) {
Hero3 h1 = new Hero3("盖伦",72);
System.out.println(h1.name + " " + h1.hp);
Hero3 h2 = new Hero3("提莫",35);
System.out.println(h2.name + " " + h2.hp);
}
}
4.3 练习
设计构造方法,参数名称分别是
String name
float hp
float armor
int moveSpeed
package 第1个程序.a4_this;
// this调用方法
public class Hero4 {
String name;
float hp; //血量
float armor; //护甲
int moveSprrd; //速度
public Hero4(String name, float hp){
this.name = name;
this.hp = hp;
}
public Hero4(String name, float hp, float armor, int moveSprrd){
this(name,hp);
this.armor = armor;
this.moveSprrd = moveSprrd;
}
public static void main(String[] args) {
Hero4 h1 = new Hero4("盖伦",900);
System.out.println(h1.name + " " + h1.hp);
Hero4 h2 = new Hero4("提莫",800,35,130);
System.out.println(h2.name + " " + h2.hp + " " + h2.armor + " " + h2.moveSprrd);
}
}
5 传参
基本类型(不写了)
引用:Hero h = new Hero();
类类型
package 第1个程序.传参;
// 类作为形参
public class Hero {
String name;
float hp; //血量
float armor; //护甲
int moveSprrd; //速度
public Hero(String name, float hp){
this.name = name;
this.hp = hp;
}
public void attack(Hero hero, float damage){
hero.hp -= damage;
System.out.println(hero.name + "被砍" + damage + ",还剩" + hero.hp);
}
public static void main(String[] args) {
Hero h1 = new Hero("盖伦", 900);
Hero h2 = new Hero("提莫",700);
h1.attack(h2,300);
}
}
package 第1个程序.传参;
// 类作为形参
public class Hero1 {
String name;
float hp; //血量
float armor; //护甲
int moveSprrd; //速度
public Hero1(String name, float hp){
this.name = name;
this.hp = hp;
}
public void attack(Hero1 hero, float damage){
hero.hp -= damage;
System.out.println(hero.name + "被砍" + damage + ",还剩" + hero.hp);
}
public void revive(Hero1 hero){
hero.hp = 700;
System.out.println(hero.name + "已复活!");
}
public static void main(String[] args) {
Hero1 h1 = new Hero1("盖伦", 900);
Hero1 h2 = new Hero1("提莫",700);
h1.attack(h2,10000);
if(h2.hp<0){
h2.revive(h2);
System.out.println("血量:" + h2.hp);
}
}
}
6 访问修饰
红字表示不可行
7 类属性 static
public class Hero {
public String name; //实例属性,对象属性,非静态属性
protected float hp;
public static String copyright;//类属性,静态属性
}
public String name; // 实例属性 = 对象属性 = 非静态属性
static String copyright; // 类属性 = 静态属性
1个属性,所有对象都共享,是一样的,就该是类属性
package 第1个程序.类属性;
public class Hero {
public String name; //姓名
protected float hp; //血量
static String copyright; //版权
public Hero(String name, float hp){
this.name = name;
this.hp = hp;
}
public void print(Hero hero){
System.out.println(hero.name + ", 血量:" + hero.hp + "版权:" + hero.copyright);
}
public static void main(String[] args) {
Hero h1 = new Hero("盖伦",800);
Hero.copyright = "腾讯专有";
h1.print(h1);
Hero h2 = new Hero("提莫", 500);
h2.print(h2);
}
}
7.1 修改
package 第1个程序.类属性;
public class Hero {
public String name; //姓名
protected float hp; //血量
static String copyright; //版权
public Hero(String name, float hp){
this.name = name;
this.hp = hp;
}
public void print(Hero hero){
System.out.println(hero.name + ", 血量:" + hero.hp + "版权:" + hero.copyright);
}
public static void main(String[] args) {
Hero h1 = new Hero("盖伦",800);
Hero.copyright = "腾讯专有";
h1.print(h1);
Hero h2 = new Hero("提莫", 500);
h2.print(h2);
h2.copyright = "123";
System.out.println(h2.copyright);
}
}
加上 final 就不会变化了!
8 类方法
类方法 = 静态方法
对象方法 = 实例方法 = 非静态方法
方法里访问了对象属性,这个方法,就必须是对象方法
否则可以是类方法
package 第1个程序.类方法;
public class Hero {
public void AAA(){
System.out.println("AAA");
}
public static void BBB(){
System.out.println("BBB");
}
public static void main(String[] args) {
Hero hero = new Hero();
hero.AAA();
hero.BBB();
// Hero.AAA();//错误
Hero.BBB();
}
}
9 属性初始化
9.1 对象属性初始化
1 声明该属性时,初始化
2 构造方法中,初始化
3 初始化块
package 第1个程序.a8_属性初始化;
public class Hero {
public String name = "some hero"; //声明时初始化
protected float hp;
float maxHP;
{
maxHP = 10000;
}
public Hero(){
hp = 70;
}
}
9.2 类属性初始化
1 声明该属性时,初始化
2 静态初始化块
package 第1个程序.a8_属性初始化;
// 类属性初始化
public class Hero2 {
public String name = "some hero"; //声明时初始化
protected float hp;
float maxHP;
public static int Capacity = 8;
public Hero2(){}
static {
Capacity = 10;
}
public static void main(String[] args) {
System.out.println(Hero2.Capacity);
}
}
9.3 练习
package 第1个程序.a8_属性初始化;
// 练习
public class Hero3 {
public String name = "some hero"; //声明时初始化
{
System.out.println("声明");
}
public Hero3(){
name = "1";
System.out.println("构造方法");
}
{
name = "2";
System.out.println("块");
}
public static void main(String[] args) {
Hero3 h1 = new Hero3();
System.out.println(h1.name);
}
}
输出:
属性赋值顺序(优先级):
1 属性默认初始化
2 属性显式初始化、初始化块
3 构造器中,初始化
4 对象.属性、对象.方法