import WKT from "ol/format/WKT"
import Point from 'ol/geom/Point';
import Feature from 'ol/Feature';
import { transform } from "ol/proj";
import { Fill, Stroke, Circle, Style, Text } from "ol/style";
export function createPoint(coordinate) {
return new Point(coordinate)
}
export function createTextStyle(text, attribute) {
let fillColor = attribute.color;
let font = attribute.font || "10px sans-serif"
let textBaseline = attribute.textBaseline || 'middle'
let padding = attribute.padding || [0, 0, 0, 0]
let offsetX = attribute.offsetX || 0
let offsetY = attribute.offsetY || 0
return new Text({
text: text,
fill: new Fill({ color: fillColor }),
font,
textAlign: attribute.textAlign,
textBaseline,
padding,
offsetX,
offsetY
});
}
export function createPointFeature(coordinate) {
let feature = new Feature({
geometry: new Point(transformTo3857(coordinate)),
});
return feature
}
export function transformTo3857(coordinate) {
return transform(coordinate, 'EPSG:4326', 'EPSG:3857')
}
export function transformTo4326(coordinate) {
return transform(coordinate, 'EPSG:3857', 'EPSG:4326')
}
export function toWKT(object) {
let wkt
if ('function' == typeof object.getArray) {
//传入features
wkt = wktFormat.writeFeatures(object)
} else if ('function' == typeof object.getGeometry) {
//传入feature
wkt = wktFormat.writeFeature(object)
} else if ('function' == typeof object.getExtent) {
//传入geometry
wkt = wktFormat.writeGeometry(object)
}
return wkt
}
export function convertToWKT(object) {
let wkt
wkt = wktFormat.writeGeometry(object)
return wkt
}
//多边形上距离指定点的最短距离的坐标
export function pointToPolygonShortCoord(lng, lat, wkt) {
let reader = new jsts.io.WKTReader()
let geom = reader.read(wkt)
let coords = geom.getCoordinates()
if (coords.length <= 1) {
//点坐标
return [lng, lat]
}
let p = { x: lng, y: lat }
let minDistance = Number.MAX_VALUE
let shortCoord = {}
for (let idx = 0; idx < coords.length - 1; idx++) {
let p1 = coords[idx]
let p2 = coords[idx + 1]
let result = pointToSegDist(p, p1, p2)
if (result.distance < minDistance) {
shortCoord.x = result.x
shortCoord.y = result.y
minDistance = result.distance
}
}
return [shortCoord.x, shortCoord.y]
}
//计算点到线段之间的距离和最短距离的交点
export function pointToSegDist(p, p1, p2) {
let cross = (p2.x - p1.x) * (p.x - p1.x) + (p2.y - p1.y) * (p.y - p1.y); //|AB*AP|:矢量乘
if (cross <= 0) {
return { distance: Math.sqrt((p.x - p1.x) * (p.x - p1.x) + (p.y - p1.y) * (p.y - p1.y)), x: p1.x, y: p1.y }//是|AP|:矢量的大小
}
let d2 = (p2.x - p1.x) * (p2.x - p1.x) + (p2.y - p1.y) * (p2.y - p1.y); //|AB|^2:矢量AB的大小的平方
if (cross >= d2) {
return { distance: Math.sqrt((p.x - p2.x) * (p.x - p2.x) + (p.y - p2.y) * (p.y - p2.y)), x: p2.x, y: p2.y }//是|BP|:矢量的大小
}
let r = cross / d2; //相似三角形原理求出c点的坐标
let px = p1.x + (p2.x - p1.x) * r;
let py = p1.y + (p2.y - p1.y) * r;
let distance = Math.sqrt((p.x - px) * (p.x - px) + (py - p.y) * (py - p.y));
return { distance: distance, x: px, y: py }
}
export function checkPointWithIn(x, y, wkt) {
//判断点是否在多边形内
var reader = new jsts.io.WKTReader()
var point = reader.read('POINT (' + x + ' ' + y + ')')
var geom = reader.read(wkt)
return point.within(geom)
}
export function createLineStyle(color, weight) {
weight = weight || 5
let style = new Style({
fill: new Fill({
color: "rgba(255, 255, 255, 0.4)"
}),
stroke: new Stroke({
color: color,
width: weight
}),
})
return style
}
export function createOtherPolygonStyle(color, width) {
width = width || 1
let polygonStyle = new Style({
fill: new Fill({
color: "rgba(255, 255, 255, 0)"
}),
stroke: new Stroke({
color: color,
width: width
}),
})
return polygonStyle
}
//虚线
export function createOtherDashPolygonStyle(color, width) {
width = width || 1
let polygonStyle = new Style({
fill: new Fill({
color: "rgba(255, 255, 255, 0)"
}),
stroke: new Stroke({
color: color,
width: width,
lineDash: [5, 5]
}),
})
return polygonStyle
}
//创建多边形style
export function createPolygonStyle(color, iconColor, width) {
width = width || 1
let polygonStyle = new Style({
fill: new Fill({
color: "rgba(255, 255, 255, 0)"
}),
stroke: new Stroke({
color: color,
width: width
}),
})
if (!iconColor) {
return polygonStyle
}
let iconStyle = iconColor
if (typeof (iconStyle) === "string") {
iconStyle = createCircelStyle(iconStyle)
}
let imgStyle = new Style({
image: iconStyle,
geometry: function (feature) {
// return the coordinates of the first ring of the polygon
let taskCollect = feature.getProperties().taskCollect;
let data = feature.getProperties().data;
if (!data) {
//任务坐标
let lng = taskCollect.longitude || taskCollect.srcLongitude || taskCollect.taskLng
let lat = taskCollect.latitude || taskCollect.srcLatitude || taskCollect.taskLat
return createPoint(transformTo3857([lng, lat]))
} else {
return createPoint(transformTo3857([data.dotLng, data.dotLat]))
}
}
})
return [polygonStyle, imgStyle]
}
export function createCircelStyle(color, radius) {
radius = radius || 3
return new Circle({
radius: radius,
fill: new Fill({
color
})
})
}
//创建点坐标style
export function createPointStyle(param, radius) {
radius = radius || 1
let iconStyle = param
if (typeof (param) === "string") {
iconStyle = createCircelStyle(param, radius)
}
return new Style({
image: iconStyle
});
}
openlayers 常用方法
于 2022-07-07 15:46:14 首次发布
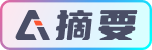