效果图
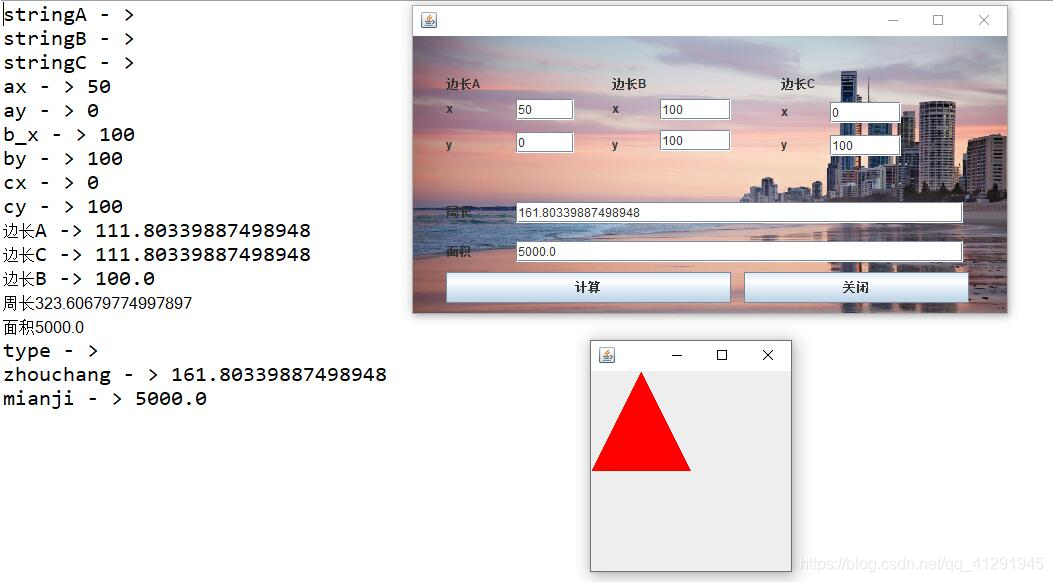
主要流程
- Main 展示出主界面
- SanJiao 用来接受主界面的坐标值
- Work 用来检查输入的坐标是否能构成三角形
- Ans 用来存放计算出的周长和面积
- Drwg 从SanJiao中获得坐标值,并且绘制出图像
- BackgroundPanel.java 设置背景图
代码展示
绘制图像
public class Drwg {
public static void write(SanJiao sanJiao) {
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new BorderLayout());
frame.add(new TestPane(sanJiao));
frame.pack();
frame.setVisible(true);
}
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new BorderLayout());
frame.add(new TestPane());
frame.pack();
frame.setVisible(true);
}
}
class TestPane extends JPanel {
Polygon poly;
public TestPane(SanJiao sanJiao) {
poly = new Polygon(new int[] { sanJiao.getAx(), sanJiao.getBx(), sanJiao.getCx() },
new int[] { sanJiao.getAy(), sanJiao.getBy(), sanJiao.getCy() }, 3);
}
public TestPane() {
poly = new Polygon(new int[] { 50, 100, 0 }, new int[] { 0, 100, 100 }, 3);
}
@Override
public Dimension getPreferredSize() {
return new Dimension(200, 200);
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g.create();
g2d.setColor(Color.RED);
g2d.fill(poly);
g2d.dispose();
}
}
计算按钮
JButton btnNewButton = new JButton("\u8BA1\u7B97");
btnNewButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String stringA = tfa.getText();
System.out.println("stringA - > " + stringA);
String stringB = tfb_1.getText();
System.out.println("stringB - > " + stringB);
String stringC = tfc.getText();
System.out.println("stringC - > " + stringC);
int ax = Integer.valueOf(a_x.getText());
System.out.println("ax - > " + ax);
int ay = Integer.valueOf(a_y.getText());
System.out.println("ay - > " + ay);
int bx = Integer.valueOf(b_x.getText());
System.out.println("b_x - > " + bx);
int by = Integer.valueOf(b_y.getText());
System.out.println("by - > " + by);
int cx = Integer.valueOf(c_x.getText());
System.out.println("cx - > " + cx);
int cy = Integer.valueOf(c_y.getText());
System.out.println("cy - > " + cy);
Work work = new Work();
Double lineA = Math.sqrt((ax - bx) * (ax - bx) + (ay - by) * (ay - by));
Double lineC = Math.sqrt((ax - cx) * (ax - cx) + (ay - cy) * (ay - cy));
Double lineB = Math.sqrt((bx - cx) * (bx - cx) + (by - cy) * (by - cy));
System.out.println("边长A -> " + lineA);
System.out.println("边长C -> " + lineC);
System.out.println("边长B -> " + lineB);
Ans ans = work.Check(lineA, lineB, lineC);
if (ans != null) {
textField_2.setText(String.valueOf(ans.getP()));
textField_3.setText(String.valueOf(ans.getS()));
SanJiao sanJiao = new SanJiao(lineA, lineB, lineC, ax, ay, bx, by, cx, cy);
drwg(sanJiao);
} else {
JOptionPane.showMessageDialog(null, "数据错误!");
tfa.setText("");
tfb_1.setText("");
tfc.setText("");
}
}
});
检查构成三角形
public static Ans Check(double a, double b, double c) {
double s, perimeter, p;
if (((a + b) > c) && ((b + c) > a) && ((a + c) > b)) {
perimeter = a + b + c;
p = perimeter / 2.0;
s = Math.sqrt((p * (p - a) * (p - b) * (p - c)));
System.out.println("周长" + perimeter);
System.out.println("面积" + s);
return new Ans(p, s);
} else {
System.out.println("error");
return null;
}
}
BackgroundPanel
public class BackgroundPanel extends JPanel {
private Image image;
public BackgroundPanel(Image image) {
super();
this.image = image;
}
protected void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2 = (Graphics2D) g;
if (image != null) {
int width = getWidth();
int height = getHeight();
g2.drawImage(image, 0, 0, width, height, this);
}
}
}