子类的继承性
public class People {
int age,leg=2,hand=2;
protected void showPeoleMess(){
System.out.printf("%d岁,%d只脚,%d只脚\t",age,leg,hand);
}
}
public class Student extends People {
int number;
void tellNumber(){
System.out.printf("学号:%d\t",number);
}
int add(int x,int y){
return x+y;
}
}
public class UniverStudent extends Student {
int multi(int x,int y){
return x*y;
}
}
public class Exam {
public static void main(String[] args) {
Student zhang = new Student();
zhang.age=17; //访问继承的成员变量
zhang.number=100101;
zhang.showPeoleMess(); //调用继承的成员变量
zhang.tellNumber();
int x=9,y=29;
System.out.println("会做加法");
int result=zhang.add(x, y);
System.out.printf("%d+%d=%d\n",x,y,result);
UniverStudent geng = new UniverStudent();
geng.age=21;//访问继承的成语变量
geng.number=6609;//访问继承的成语变量
geng.showPeoleMess();//调用继承的成员变量
geng.tellNumber();//调用继承的成员变量
System.out.print("会做加法");
result=geng.add(x, y); //调用继承的成员变量
System.out.printf("%d+%d=%d\t",x,y,result);
System.out.print("会做乘法");
result=geng.multi(x, y); //调用继承的成员变量
System.out.printf("%d*%d=%d\t",x,y,result);
}
}
子类与对象
class People{
private int averHeight=166;
public int getAverHeight() {
return averHeight;
}
}
class ChinaPeople extends People{
int height;
public void setHeight(int h) {
//height=h+averHeight; 非法,子类没有继承averHeight
height=h;
}
public int getHeight(){
return height;
}
}
public class exam {
public static void main(String[] args) {
ChinaPeople zhangsan=new ChinaPeople();
System.out.println("子类对象未继承的averageHeight的值是:"+zhangsan.getAverHeight());
zhangsan.setHeight(178);
System.out.println("子类对象的实例变量height的值是:"+zhangsan.getHeight());
}
}
成员变量的隐藏
public class Goods {
public double weight;
public void oldSetWeight(double w){
weight=w;
System.out.println("double型的weight="+weight);
}
public double oldGetPrice(){
double price=weight*10;
return price;
}
}
public class CheapGoods extends Goods{
public int weight;
public void newSetWeight(int w){
weight=w;
System.out.println("int型的weight="+weight);
}
public double newGetPrice(){
double price=weight*10;
return price;
}
}
public class Exam {
public static void main(String[] args) {
CheapGoods cheapGoods=new CheapGoods();
//cheapGoods.weight=198.98; 是非法的,因为子类对象的weight变量已经是int型
cheapGoods.newSetWeight(198);
System.out.println("对象cheapGoods的weight的值是:"+cheapGoods.weight);
System.out.println("cheapGoods用子类新增的优惠方法计算价格:"+cheapGoods.newGetPrice());
cheapGoods.oldSetWeight(198.987);//子类对象调用继承的方法操作隐藏的double型变量weight;
System.out.println("cheapGoods使用继承的方法(无优惠)价格计算:"+cheapGoods.oldGetPrice());
}
}
方法重写
public class University {
void enterRule(double math,double english,double chinese){
double total=math+english+chinese;
if(total>=80)
System.out.println(total+"分数线达到大学录取线");
else
System.out.println(total+"分数线未达到大学录取线");
}
}
public class ImportantUniversity {
void enterRule(double math,double english,double chinese){
double total=math+english+chinese;
if(total>=220)
System.out.println(total+"分数线达到重点大学录取线");
else
System.out.println(total+"分数线未达到重点大学录取线");
}
}
public class exam {
public static void main(String[] args) {
double math=62,english=76.5,chinese=67;
ImportantUniversity univer=new ImportantUniversity();
univer.enterRule(math, english, chinese); //调用重写的方法
math=91;
english=82;
chinese=86;
univer.enterRule(math, english, chinese);//调用重写的方法
}
}
package hah;
class A{
float computer(float x,float y){
return x+y;
}
public int g(int x,int y){
return x+y;
}
}
class B extends A{
float computer(float x,float y){
return x*y;
}
}
public class exam {
public static void main(String[] args) {
B b=new B();
double result = b.computer(8, 9);//b调用重写的方法
System.out.println(result);
int m=b.g(12, 8); //b调用继承的方法
System.out.println(m);
}
}
super关键字
class Sum{
int n;
float f(){
float sum=0;
for(int i=1;i<=n;i++)
sum=sum+i;
return sum;
}
}
class Average extends Sum{
int n;
float f(){
float c;
super.n=n;
c=super.f();
return c/n;
}
float g(){
float c;
c=super.f();
return c/2;
}
}
public class exam {
public static void main(String[] args) {
Average aver=new Average();
aver.n=100;
float resultOne=aver.f();
float resultTwo=aver.g();
System.out.println("resultOne="+resultOne);
System.out.println("resultTwo="+resultTwo);
}
}
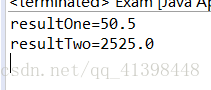