顺序队列的实现
#include<iostream.h>
const int maxSize=20;
template<typename T>
class Queue
{
private:
int front,rear;
T data[maxSize];
public:
Queue()
{front=rear=maxSize-1;}
~Queue(){}
void In(T x);
void Out();
T Getfront();
bool Empty();
};
template<typename T>
void Queue<T>::In(T x)
{
if((rear+1)%maxSize==front)throw"上溢";
rear=(rear+1)%maxSize;
data[rear]=x;
}
template<typename T>
void Queue<T>::Out()
{
if(rear==front)throw"下溢";
front=(front+1)%maxSize;
}
template<typename T>
T Queue<T>::Getfront()
{
int i;
if(rear==front)throw"下溢";
i=(front+1)%maxSize;
return data[i];
}
template<typename T>
bool Queue<T>::Empty()
{
if(front==rear)return 1;
else return 0;
}
int main()
{
Queue<int>S;
cout<<"对1、2、3执行入队操作"<<endl;
S.In(1);
S.In(2);
S.In(3);
cout<<"队头元素为:"<<S.Getfront()<<endl;
cout<<"执行一次出队操作"<<endl;
S.Out();
cout<<"队头元素为:"<<S.Getfront()<<endl;
return 0;
}
链队列的实现
#include<iostream.h>
template<class D>
struct Node
{
D data;
Node<D> *next;
};
template<class D>
class LinkQueue
{
public:
LinkQueue();
~LinkQueue();
void In(D x);
D Out();
D Getfront();
int Empty();
private:
Node<D> *front,*rear;
};
template<class D>
LinkQueue<D>::LinkQueue()
{
Node<D>*s=NULL;
s=new Node<D>;
s->next=NULL;
front=rear=s;
}
template<class D>
LinkQueue<D>::~LinkQueue()
{
Node<D> *p=NULL;
while (front!=NULL)
{
p=front->next;
delete front;
front=p;
}
}
template<class D>
void LinkQueue<D>::In(D x)
{
Node<D> *s=NULL;
s=new Node<D>;
s->data=x;
s->next=NULL;
rear->next=s;rear=s;
}
template<class D>
D LinkQueue<D>::Out()
{
Node<D> *p=NULL;
int x;
if (rear==front)throw"下溢";
p=front->next;
x=p->data;
front->next=p->next;
if(p->next=NULL)rear=front;
delete p;
return x;
}
template<class D>
D LinkQueue<D>::Getfront()
{
if(front!=rear)
return front->next->data;
}
template<class D>
int LinkQueue<D>::Empty()
{
if(front==rear)return 1;
else return 0;
}
void main()
{
LinkQueue<int>Q;
if(Q.Empty())
cout<<"队列为空"<<endl;
else
cout<<"队列非空"<<endl;
cout<<"对元素10和15执行入队操作"<<endl;
try
{
Q.In(10);
Q.In(15);
}
catch(char *wrong)
{
cout<<wrong<<endl;
}
cout<<"查看队头元素:"<<Q.Getfront()<<endl;
cout<<"执行出队操作"<<endl;
try
{
Q.Out();
}
catch(char * wrong)
{
cout<<wrong<<endl;
}
cout<<"查看队头元素:"<<Q.Getfront()<<endl;
}
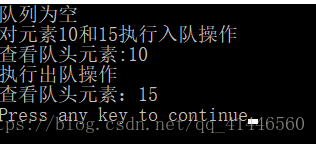