如何使用Spring框架调用构造方法
1. 创建用例
1 新建test,vo两个包,包内分别创建Java文件Teat.java和Student.java。
-
建立名为application.xml的Spring Bean Configuration File
通过File->New->Other->搜索spring->Spring Bean Configuration File,打开如下窗口,输入文件名后Finish
2 新建文件夹lib并拷贝jar包,然后选中所拷jar包->右键->Build Path->点击Add to Build Path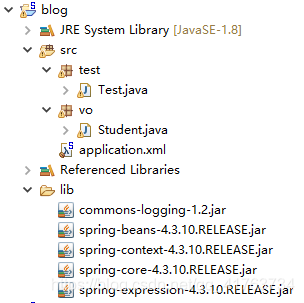
2.各种参数类型如何调用构造方法
1. 测试类
测试类代码如下
package test;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Test {
public static void main(String[] args) {
ClassPathXmlApplicationContext applicationContext = new ClassPathXmlApplicationContext("application.xml");
Student student = applicationContext.getBean(Student.class);
System.out.println(student);
}
}
2 不同参数类型的赋值
- 基本数据类型和String类型
当数据类型为基本数据类型和String时,通过value标签属性直接赋值
Student类:
package vo;
public class Student {
private String name;
private int age;
public Student(int age) {
this.age = age;
System.out.println("单个参数构造方法1");
}
public Student(int age,String name) {
this.age = age;
this.name = name;
System.out.println("多个参数构造方法1");
}
@Override
public String toString() {
return "age=" + age + ", name=" + name + "";
}
}
在application中添加一个< bean>< /bean>标签
constructor-arg标签内的name值对应Student类中定义的变量
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean class = "vo.Student">
<constructor-arg name = "age" value = "12"></constructor-arg>
<constructor-arg name = "name" value = "Tom"></constructor-arg>
</bean>
</beans>
测试类运行结果:
用constructor定义了两个参数,执行了多个参数的构造方法
- 引用数据类型
如果参数类型是引用数据类型,则用ref属性指定对象
Student类:
package vo;
import java.util.Date;
public class Student {
private Date birthday;
public Student(Date birthday) {
this.birthday = birthday;
System.out.println("引用类型数据构造方法");
}
@Override
public String toString() {
return "birthday=" + birthday;
}
}
application.xml中配置,用ref标签属性指定对象
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id = "date" class = "java.util.Date"/>
<bean class = "vo.Student">
<constructor-arg name ="birthday" ref="date"></constructor-arg>
</bean>
</beans>
测试类运行结果:
- 数组类型
使用array子标签设置数组,通过array子标签的value子标签设置数组每个元素的值
Student类:
package vo;
public class Student {
int [] scores;
public Student(int [] scores) {
this.scores = scores;
for (int score : scores) {
System.out.println(score);
}
System.out.println("数组参数构造方法");
}
}
application.xml 中配置
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean class = "vo.Student">
<constructor-arg name ="scores">
<array>
<value>99</value>
<value>77</value>
<value>66</value>
</array>
</constructor-arg>
</bean>
</beans>
测试类运行结果:
下面是集合类型参数的构造方法,修改Test类中的代码为
package test;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Test {
public static void main(String[] args) {
new ClassPathXmlApplicationContext("application.xml");
}
}
加载容器后,xml里面的class=“com.jd.vo.Students”,使加载类,并调用构造方法来创建对象
- List集合
Student类:
package vo;
import java.util.List;
public class Student {
private List<String> names;
public Student(List<String> names) {
this.names = names;
for (String name : names) {
System.out.println(name);
}
System.out.println("list集合");
}
}
application.xml 中配置
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean class = "vo.Student">
<constructor-arg name ="names">
<list>
<value>Tom</value>
<value>Candy</value>
<value>Lucy</value>
</list>
</constructor-arg>
</bean>
</beans>
测试类运行结果:
- Set集合:
Student类:
package vo;
import java.util.Set;
public class Student {
private Set<String> ids;
public Student(Set<String> ids) {
this.ids = ids;
for (String id : ids) {
System.out.println(id);
}
System.out.println("set集合");
}
}
application.xml 中配置
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean class = "vo.Student">
<constructor-arg name ="ids">
<set>
<value>AAA</value>
<value>BBB</value>
<value>CCC</value>
</set>
</constructor-arg>
</bean>
</beans>
测试类运行结果:
数组,list集合,set集合调用构造方法的方式类似,如果数组、List、Set集合中的参数类型是基本数据类型或String类型,则使用value标签赋值,如果是引用类型,使用bean子标签或ref子标签。
举例:
Student类:
package vo;
import java.util.Date;
import java.util.List;
public class Student {
private List<Date> birthdays;
public Student(List<Date> birthdays) {
this.birthdays = birthdays;
for (Date birthday : birthdays) {
System.out.println(birthday);
}
}
}
application配置:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="date" class="java.util.Date"/>
<bean class = "vo.Student">
<constructor-arg name ="birthdays">
<list>
<bean class = "java.util.Date"></bean>
<ref bean="date"/>
</list>
</constructor-arg>
</bean>
</beans>
运行测试类:
- Map集合:
如果参数类型是Map集合,则用< map>标签赋值,元素使用< entry>标签
Student类:
package vo;
import java.util.Date;
import java.util.Map;
import java.util.Set;
public class Student {
private Map<String,Date> birthdays;
public Student(Map<String,Date> birthdays) {
this.birthdays = birthdays;
Set<String> keys = birthdays.keySet();
for (String key : keys) {
Date birthday = birthdays.get(key);
System.out.println(birthday);
}
System.out.println("map集合");
}
}
application.xml 中配置
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="date" class="java.util.Date"/>
<bean class = "vo.Student">
<constructor-arg name ="birthdays">
<map>
<entry key="father" value-ref="date"></entry>
<entry key="mother" value-ref="date"></entry>
</map>
</constructor-arg>
</bean>
</beans>
测试类运行结果: