JAVA实现发送信息到邮箱
一、使用场景
1、使用场景:注册、登录时使用邮箱获取验证码,获取异常日志、推送广告等等
二、JAVA使用示例(框架使用RUOYI框架,此鸣谢)
1、引入相关依赖
<!-- 发送邮箱依赖 -->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-email</artifactId>
<version>1.4</version>
</dependency>
<!-- 接口请求依赖 -->
<dependency>
<groupId>commons-httpclient</groupId>
<artifactId>commons-httpclient</artifactId>
<version>3.1</version>
</dependency>
2、配置QQ邮箱获取邮箱授权码
2.1、登录QQ后进入设置
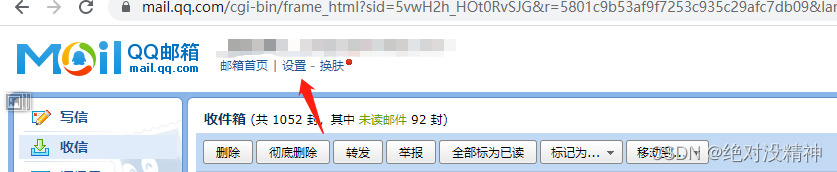
2.2、进入账号设置

2.3、找到账号安全,开启POP3/IMAP/SMTP/Exchange/CardDAV/CalDAV服务,我这已开启。开启后获取密钥,要保存起来,不记得需要重新生成密钥
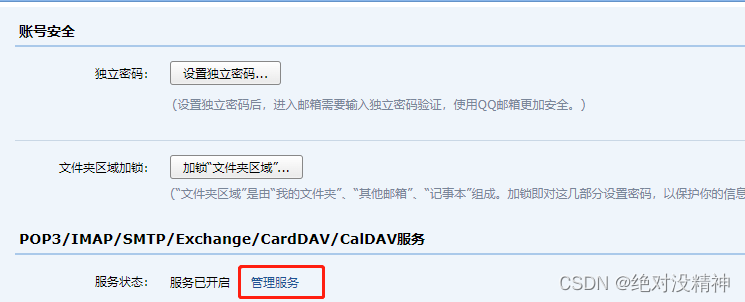
3、邮箱配置(在yml里配置)
spring:
# 邮箱配置
mail:
# 邮箱地址 默认为 smtp.qq.com
host: smtp.qq.com
# 发送人 邮箱
username: xxxxx@qq.com
# 邮箱授权码(这个填密钥)
password: xxxxxxx
4、封装http工具类
package com.ruoyi.common.utils.http;
import org.apache.commons.httpclient.Header;
import org.apache.commons.httpclient.HttpClient;
import org.apache.commons.httpclient.HttpException;
import org.apache.commons.httpclient.NameValuePair;
import org.apache.commons.httpclient.methods.GetMethod;
import org.apache.commons.httpclient.methods.PostMethod;
import org.apache.commons.httpclient.methods.RequestEntity;
import org.apache.commons.httpclient.methods.StringRequestEntity;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.*;
import java.nio.charset.StandardCharsets;
import java.util.Map;
/**
* 封装http方法
* @date 2023-08-02 15:00:00
* @author lizan
*
*/
public class HttpUtil {
/**
* 本地异常日志记录对象
*/
protected static Logger logger = LoggerFactory.getLogger(HttpUtil.class);
/**
* 执行post方法
*
* @param url 调用地址 调用地址
* @param params 参数 参数
* @return 响应数据
*/
public static String doPost(String url, Map<String, Object> params) {
try {
HttpClient client = new HttpClient();
PostMethod post = new PostMethod(url);
int count = 0;
NameValuePair[] nameValuePairs = new NameValuePair[params.size()];
for (String key : params.keySet()) {
String value = params.get(key) == null ? "" : params.get(key).toString();
NameValuePair param = new NameValuePair(key, value);
nameValuePairs[count] = param;
count++;
}
post.setRequestBody(nameValuePairs);
client.executeMethod(post);
String respStr = post.getResponseBodyAsString();
post.releaseConnection();
return respStr;
} catch (Exception e1) {
e1.printStackTrace();
throw new RuntimeException("远程调用" + url + "出错");
}
}
/**
* 执行post方法
*
* @param url 调用地址
* @param params 参数
* @return 响应数据
*/
public static String doPost1(String url, Map<String, Object> params) {
try {
HttpClient client = new HttpClient();
PostMethod post = new PostMethod(url);
post.setRequestHeader("Content-Type", "application/x-www-form-urlencoded; charset=utf-8");
int count = 0;
NameValuePair[] nameValuePairs = new NameValuePair[params.size()];
for (String key : params.keySet()) {
String value = params.get(key) == null ? "" : params.get(key).toString();
NameValuePair param = new NameValuePair(key, value);
nameValuePairs[count] = param;
count++;
}
post.setRequestBody(nameValuePairs);
client.executeMethod(post);
String respStr = new String(post.getResponseBody(), StandardCharsets.UTF_8);
post.releaseConnection();
return respStr;
} catch (Exception e1) {
e1.printStackTrace();
throw new RuntimeException("远程调用" + url + "出错");
}
}
/**
* 执行post方法
*
* @param url 调用地址
* @param params 参数
* @return 响应数据
*/
public static void doResponsePost(String url, Map<String, Object> params, HttpServletResponse response) {
InputStream is = null;
OutputStream os = null;
HttpClient client = new HttpClient();
try {
PostMethod post = new PostMethod(url);
int count = 0;
NameValuePair[] nameValuePairs = new NameValuePair[params.size()];
for (String key : params.keySet()) {
String value = params.get(key) == null ? "" : params.get(key).toString();
NameValuePair param = new NameValuePair(key, value);
nameValuePairs[count] = param;
count++;
}
post.setRequestBody(nameValuePairs);
client.executeMethod(post);
byte[] respStr = post.getResponseBody();
is = new ByteArrayInputStream(respStr);
os = response.getOutputStream();
byte[] buffer = new byte[1024 * 1024];
while ((count = is.read(buffer)) != -1) {
os.write(buffer, 0, count);
os.flush();
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (is != null) {
is.close();
}
if (os != null) {
os.close();
}
response.flushBuffer();
} catch (Exception ex) {
ex.printStackTrace();
}
}
}
/**
* 执行下载附件方法
*
* @param url 调用地址
* @param params 参数
* @return 响应数据
*/
public static void doAppDownLoadPost(String url, Map<String, Object> params, HttpServletResponse response) {
InputStream is = null;
OutputStream os = null;
HttpClient client = new HttpClient();
try {
PostMethod post = new PostMethod(url);
int count = 0;
NameValuePair[] nameValuePairs = new NameValuePair[params.size()];
for (String key : params.keySet()) {
String value = params.get(key) == null ? "" : params.get(key).toString();
NameValuePair param = new NameValuePair(key, value);
nameValuePairs[count] = param;
count++;
}
post.setRequestBody(nameValuePairs);
client.executeMethod(post);
byte[] respStr = post.getResponseBody();
// 文件名称
Header fileName = post.getResponseHeader("fileName");
is = new ByteArrayInputStream(respStr);
response.addHeader("Content-Disposition", "attachment;filename=" + fileName.getValue());
os = response.getOutputStream();
byte[] buffer = new byte[1024 * 1024];
while ((count = is.read(buffer)) != -1) {
os.write(buffer, 0, count);
os.flush();
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (is != null) {
is.close();
}
if (os != null) {
os.close();
}
response.flushBuffer();
} catch (Exception ex) {
ex.printStackTrace();
}
}
}
/**
* 执行post方法
*
* @param url 调用地址
* @param params 参数
* @return 响应数据
*/
public static String doPost3(String url, Map<String, Object> params, HttpServletRequest request) {
try {
HttpClient client = new HttpClient();
PostMethod post = new PostMethod(url);
post.setRequestHeader("Content-Type", "application/x-www-form-urlencoded; charset=utf-8");
post.addRequestHeader("Cookie",request.getHeader("Cookie"));
int count = 0;
NameValuePair[] nameValuePairs = new NameValuePair[params.size()];
for (String key : params.keySet()) {
String value = params.get(key) == null ? "" : params.get(key).toString();
NameValuePair param = new NameValuePair(key, value);
nameValuePairs[count] = param;
count++;
}
post.setRequestBody(nameValuePairs);
client.executeMethod(post);
String respStr = new String(post.getResponseBody(), StandardCharsets.UTF_8);
post.releaseConnection();
return respStr;
} catch (Exception e1) {
e1.printStackTrace();
throw new RuntimeException("远程调用" + url + "出错");
}
}
/**
* 执行get方法
*
* @param url 调用地址
* @param params 参数
* @return 响应数据
*/
public String doGet(String url, Map<String, Object> params) {
try {
HttpClient client = new HttpClient();
GetMethod get = new GetMethod(url);
int count = 0;
NameValuePair[] nameValuePairs = new NameValuePair[params == null ? 0 : params.size()];
if (params != null) {
for (String key : params.keySet()) {
String value = params.get(key) == null ? "" : params.get(key).toString();
NameValuePair param = new NameValuePair(key, value);
nameValuePairs[count] = param;
count++;
}
}
get.setQueryString(nameValuePairs);
client.executeMethod(get);
String respStr = new String(get.getResponseBody(), StandardCharsets.UTF_8);
get.releaseConnection();
return respStr;
} catch (Exception e) {
logger.error(e.getMessage());
e.printStackTrace();
throw new RuntimeException("远程调用" + url + "出错");
}
}
/** *
* POST请求 JSON格式传参
* @param url 调用地址 url地址
* @param json json格式字符串
* @param headerParam 头部信息
* */
public static String doPostJson(String url, String json , Map<String, Object> headerParam) {
StringBuilder sb = new StringBuilder();
// 创建连接对象
HttpClient httpClient = new HttpClient();
// 创建请求
PostMethod method = new PostMethod(url);
// 设置请求头格式为json格式
RequestEntity entity = null;
try {
entity = new StringRequestEntity(json, "application/json", "UTF-8");
// 设置请求体信息
if (entity == null){
return "";
}
method.setRequestEntity(entity);
// 设置请求头信息
for (String key : headerParam.keySet()) {
method.setRequestHeader(key, headerParam.get(key).toString());
}
// 创建连接
httpClient.executeMethod(method);
// 获取返回信息
InputStream in = method.getResponseBodyAsStream();
InputStreamReader isr = new InputStreamReader(in, "UTF-8");
char[] b = new char[4096];
for (int n; (n = isr.read(b)) != -1;) {
sb.append(new String(b, 0, n));
}
} catch (UnsupportedEncodingException e) {
logger.error("=====JSON数据转换有误。参数json数据:" + json + "END=====");
e.printStackTrace();
} catch (HttpException e) {
logger.error("=====URL连接失败。url:" + url + "END=====");
e.printStackTrace();
} catch (IOException e) {
logger.error("=====参数传递失败。END=====");
e.printStackTrace();
}
return sb.toString();
}
}
5、封装发送邮箱工具类
package com.ruoyi.common.utils.mail;
import org.apache.commons.mail.EmailAttachment;
import org.apache.commons.mail.MultiPartEmail;
import org.apache.commons.mail.SimpleEmail;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
import javax.mail.internet.MimeUtility;
/**
* @author lizan
* @date 2023-08-03 11:14
*/
@Component
public class MailUtil {
/** 发送方的邮件服务器 */
@Value("${spring.mail.host}")
private String hostName;
/** 收件人邮箱 */
@Value("${spring.mail.username}")
private String sendEmail;
/** 发件人密码 */
@Value("${spring.mail.password}")
private String password;
/**
* 发送文本到指定邮箱
* @param receiverEmail 接收人邮箱
* @param subject 主题
* @param context 内容
* @throws Exception
*/
public void sendCommonTextMail(String receiverEmail, String subject, String context) throws Exception {
System.out.println("hostName" + hostName + "--" + receiverEmail + subject + context + hostName + sendEmail + password);
//发送普通邮件
SimpleEmail email = new SimpleEmail();
//发送方的邮件服务器
email.setHostName(hostName);
//设置登录的账号密码
email.setAuthentication(sendEmail, password);
// 设置发送方,给发送方指定名字
email.setFrom(sendEmail, sendEmail, "utf-8");
//设置接收方
email.addTo(receiverEmail);
//设置邮件主题
email.setSubject(subject);
//设置邮件内容
email.setContent(context, "text/html;charset=utf-8");
email.send();
}
public void sendCommonFileMail(String receiverEmail, String subject, String context) throws Exception {
// TODO Auto-generated method stub
MultiPartEmail email = new MultiPartEmail();
//发送方的邮件服务器
email.setHostName(hostName);
//设置登录的账号密码
email.setAuthentication(sendEmail, password);
// 设置发送方,给发送方指定名字
email.setFrom(sendEmail, sendEmail, "utf-8");
//设置接收方
email.addTo(receiverEmail);
//设置邮件主题
email.setSubject(subject);
email.setCharset("utf-8");
// 发附件的时候不能用setContent方法,否则不显示附件
email.setMsg(context);
EmailAttachment attachment = new EmailAttachment();
attachment.setPath("D:\\test.xlsx");
attachment.setName(MimeUtility.encodeText("test.xlsx"));
// 把附件添加到email对象上
email.attach(attachment);
email.send();
}
}
6、调用结果(进入QQ邮箱可查看)
protected Logger logger = LoggerFactory.getLogger(this.getClass());
@Autowired
private MailUtil mailUtil;
public void interfaceMultipleParams(String mail ,String subject , String dataReturn)
{
logger.info("接受者邮箱: {} 主题: {} 信息: {}" ,mail ,subject , dataReturn);
try {
mailUtil.sendCommonTextMail(mail, subject , dataReturn);
} catch (Exception e){
e.printStackTrace();
logger.error("调用发送邮箱接口报错", e.fillInStackTrace());
}
}
