java生成图片验证码
使用场景:
- 获取手机、邮件验证码之前做一层检测是否是人为验证
- 登录、注册之前做一层检测是否是人工验证
- 频繁点击登录、注册接口做一层检测是否是人为验证
一、引入相关依赖
<!-- 添加图形验证码依赖 -->
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-captcha</artifactId>
<version>5.8.6</version>
</dependency>
- createCode 创建验证码,实现类需同时生成随机验证码字符串和验证码图片
- getCode 获取验证码的文字内容
- verify 验证验证码是否正确,建议忽略大小写
- write 将验证码写出到目标流中
- 而AbstractCaptcha为一个ICaptcha抽象实现类,此类实现了验证码文本生成、非大小写敏感的验证、写出到流和文件等方法,通过继承此抽象类只需实现createImage方法定义图形生成规则即可。
二、生成图形验证码(模拟场景发送手机验证码)
@GetMapping("/createCaptcha")
public ApiResult createCaptcha(HttpServletResponse response, @NotNull @RequestParam("captchaCode") String captchaCode) {
String phoneCode = CODE_PHONE_CAPTCHA + captchaCode;
LineCaptcha lineCaptcha = CaptchaUtil.createLineCaptcha(200, 100);
logger.info("lineCaptcha.getCode(){}",lineCaptcha.getCode());
String code = lineCaptcha.getCode();
String imageBase64 = lineCaptcha.getImageBase64();
Map<String, Object> data = Maps.newConcurrentMap();
data.put("imageBase64" , imageBase64);
data.put("captchaCode", captchaCode);
redisUtils.set(phoneCode , code, 30);
return ApiResult.success("获取成功", data);
}
@ApiOperation(value = "发送短信", notes = "根据手机号发送通用短信")
@ApiImplicitParam(name = "phone", value = "手机号码", required = true, dataType = "String", paramType = "query", example = "15243686469")
@PostMapping("/verificationCodeToPhone")
public ApiResult sendPhoneMsg(HttpServletRequest request,@NotNull @RequestParam("phone") String phone, @NotNull @RequestParam("captchaValue") String captchaValue,
@NotNull @RequestParam("captchaCode") String captchaCode) {
String ipAddress = IpAdrressUtil.getIpAddress(request);
if (redisUtils.hasKey(CODE_IPADDRESS + ipAddress)) {
Integer ipAddressCount = Integer.valueOf(redisUtils.get(CODE_IPADDRESS + ipAddress).toString());
if (ipAddressCount > 200) {
return ApiResult.error("当前IP已被限制发送短信,请求过多,请第二天重试!", 500);
}
redisUtils.incr(CODE_IPADDRESS + ipAddress, 1);
} else {
redisUtils.set(CODE_IPADDRESS + ipAddress, 1, 60 * 60 * 24);
}
if (isMobile(phone)) {
if (!redisUtils.hasKey(CODE_PHONE_CAPTCHA + captchaCode)) {
throw new RuntimeException("图形码验证失败,请先刷新图片验证码重新输入!");
}
String codePhone = redisUtils.get(CODE_PHONE_CAPTCHA + captchaCode).toString();
if (!StringUtils.equals(codePhone,captchaValue)) {
throw new RuntimeException("图形码验证失败,请先刷新图片验证码重新输入!");
}
if (redisUtils.hasKey(CODE_PHONE + phone)) {
throw new RuntimeException("获取验证码失败,请求频繁,稍后再试!!!");
}
String code = roundNum();
SmsUtils.getInstance().sendSms(phone, code);
redisUtils.set(CODE_PHONE + phone, code, 60);
return success(BaseErrorEnum.SUCCESS_SEND_OK.getErrorMessage(), phone);
}
return error(BaseErrorEnum.ERR_PHONE_FORMAT.getErrorMessage());
}
private boolean isMobile(String mobiles) {
String telRegex = "[1][3456789]\\d{9}";
if (TextUtils.isEmpty(mobiles)) {
return false;
} else {
return mobiles.matches(telRegex);
}
}
三、前端转为图片验证码
- JS代码,调用接口
createCaptchaCode(){
var captchaCode = this.uuid();
var params = {
captchaCode: captchaCode
}
this.captchaCode = captchaCode;
getCreateCaptcha(params, data => {
if (data && data.success){
var datas = data.attributes;
this.imgCodeObj.codeImg = datas.imageBase64;
this.imgCodeObj.codeId = datas.captchaCode;
console.log("data",data)
}
});
console.log("this.captchaCode",this.captchaCode)
},
uuid() {
return 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function(c) {
var r = Math.random()*16|0, v = c == 'x' ? r : (r&0x3|0x8);
return v.toString(16);
});
},
- vue代码(最主要这段::src=“‘data:image/png;base64,’ + imgCodeObj.codeImg”)
<div class="input-group mar-btm" v-show="isPhone">
<input :focus="inputFocus.codeFocus" v-model="captchaValue" id="captchaValue"
@keyup.enter="submit" autocomplete="off" class="form-control "
placeholder="图形验证码" style=" width: 250px; height: 50px;"/>
<span class="input-group-btn" >
<img
@click="createCaptchaCode"
style="margin-top: -30px; width: 140px; height: 50px;"
:src="'data:image/png;base64,' + imgCodeObj.codeImg"
/>
</span>
</div>
四、结果展示
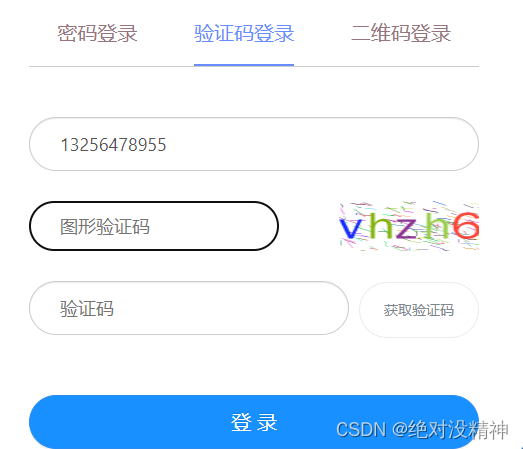