前言
Scatter的开发文档写的就…很多细节它都没说,导致我踩了很多坑。你敢信我是从报错->google报错->git上面的一个issue->vue版本的连接代码这样子搞出来的吗,我人傻了.
前置准备
1. eos链 比如下面的
let jungle2 = {
protocol: "http",
blockchain: "eos",
host: "jungle2.cryptolions.io",
port: 80,
chainId: "e70aaab8997e1dfce58fbfac80cbbb8fecec7b99cf982a9444273cbc64c41473"
};
2. 在该链上有账户
Scatter中的一些设置地方
- 添加自定义网络链(chainid点击画圈处会自动生成)
- 通过私钥添加或创建账户
下载依赖
npm i -S scatterjs-core //核心
npm i -S eosjs //eos开发所依赖的eosjs,
npm i -S scatterjs-plugin-eosjs //eosjs版本小于等于16.0.9时候下载这个
npm i -S scatterjs-plugin-eosjs2 // eosjs最新版本的下载这个(npm i -S eosjs这下载的必定是最新版本,所以肯定下载这个啦)
头部引入
下面所有代码全在一个index,js的文件中
import ScatterJS,{Network} from 'scatterjs-core';
import {Api, JsonRpc} from "eosjs";
import ScatterEOS from 'scatterjs-plugin-eosjs2'
// Don't forget to tell ScatterJS which plugins you are using.
ScatterJS.plugins( new ScatterEOS() );
相关配置(就是个对象和常量啦)
//网络配置
const network = Network.fromJson({
blockchain:'eos',
host:'写你自己的',
port:8888,
protocol:'http',
chainId:'写你自己的'
});
//rpc是eosjs中的东西,有很多方法可以查询余额之类的
const rpc = new JsonRpc(network.fullhost());
组件的基本样子
export default function ScatterTest () {
const [scatter,setScatter]=useState(null)//等会会获取的scatter
const [state,setState]=useState({//等会会获取数据,先占个茅坑
identity:null,
account:null,
eos:null,
})
//简单的打印下state的值
useEffect(()=>{
console.log('state',state);
},[state])
//连接Scatter
function connectToScatter() {...}
//获取用户身份,用户登录
function getIdentity(scatter){...}
//断开连接,用户登出
function forgetIdentity(scatter){...}
//获取余额信息
function getCurrencyBalance(account){}
return(
<>
<button onClick={connectToScatter}>连接scatter</button>
<button onClick={()=>{forgetIdentity(scatter)}}>断开连接scatter</button>
<button onClick={()=>{getCurrencyBalance(state.account)}}>获取余额信息</button>
</>
)
}
连接scatter和登录
//连接scatter
function connectToScatter() {
ScatterJS.scatter.connect("这里是随便起的名字").then(connected => {
// 用户没有下载Scatter或者下载了但是没有打开运行
if(!connected) {
alert('User does not have Scatter Desktop, Mobile or Classic installed.')
return false;
}
//获取到了scatter
const scatter = ScatterJS.scatter;
setScatter(scatter)
//用户登录
getIdentity(scatter)
/*
* Always remember to null out the window.ScatterJS reference, if you don't extensions
* will be able to catch that reference and make requests to the user's Scatter on behalf of
* your domain, and you will have to take responsibility.
* (我概况下:必写,不然会被截取伪造操作,你要负责的哦)
* */
window.ScatterJS = null;
});
}
//获取用户身份
function getIdentity(scatter) {
scatter.getIdentity({
personal:['firstname', 'lastname','email','birthdate'],
location:['country','address','city','state','country'],
accounts:[network]
}).then(identity => {
// Always use the accounts you got back from Scatter. Never hardcode them even if you are prompting
// the user for their account name beforehand. They could still give you a different account.
//概况:就这么写对了。
const account = scatter.identity.accounts.find(x => x.blockchain === 'eos');//EOSIO account
// Get a proxy reference to eosjs which you can use to sign transactions with a user's Scatter.
//在这拿到eos
//beta3:true 如果你调用api交易 sign(签名)后的时候返回什么iterate undefined
//就要加这个。具体的去看Scatter 上github的issue 74 这个是两年前为了
//支持最新版本eosjs beta3 时候加的,但是现在两年后Scatter
//最新代码上还是有这个判断就emmm
//具体看scatterjs-plugin-eosjs2/scr/index.js 里面的sign这个方法
//看里面有没有 if(!beta3)这东西
const eos = scatter.eos(network, Api, {rpc,beta3:true});//a reference to an eosjs object
//把值保存下来
setState({
...state,
identity,
account,
eos
})
}).catch(error => {
console.log('error',error);
})
}
如果没下载或运行Scatter情况
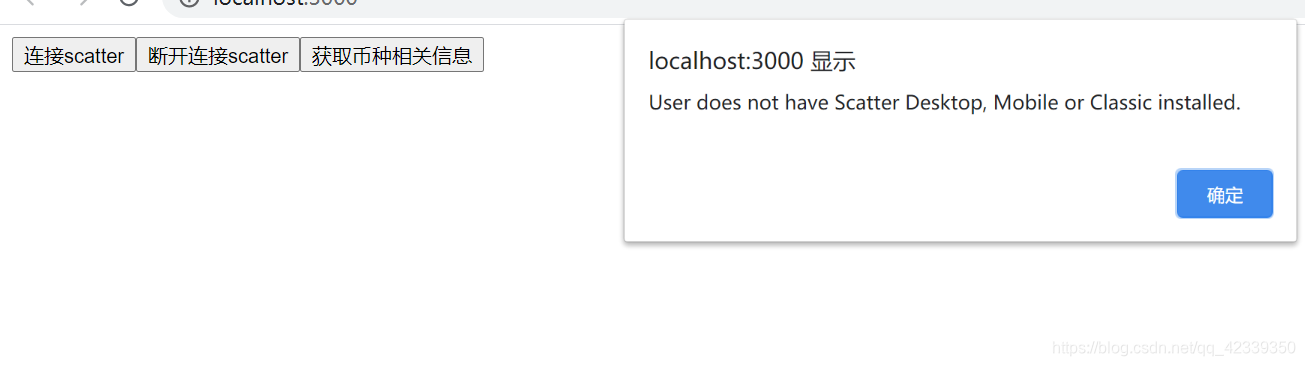
如果下载且Scatter在运行的情况
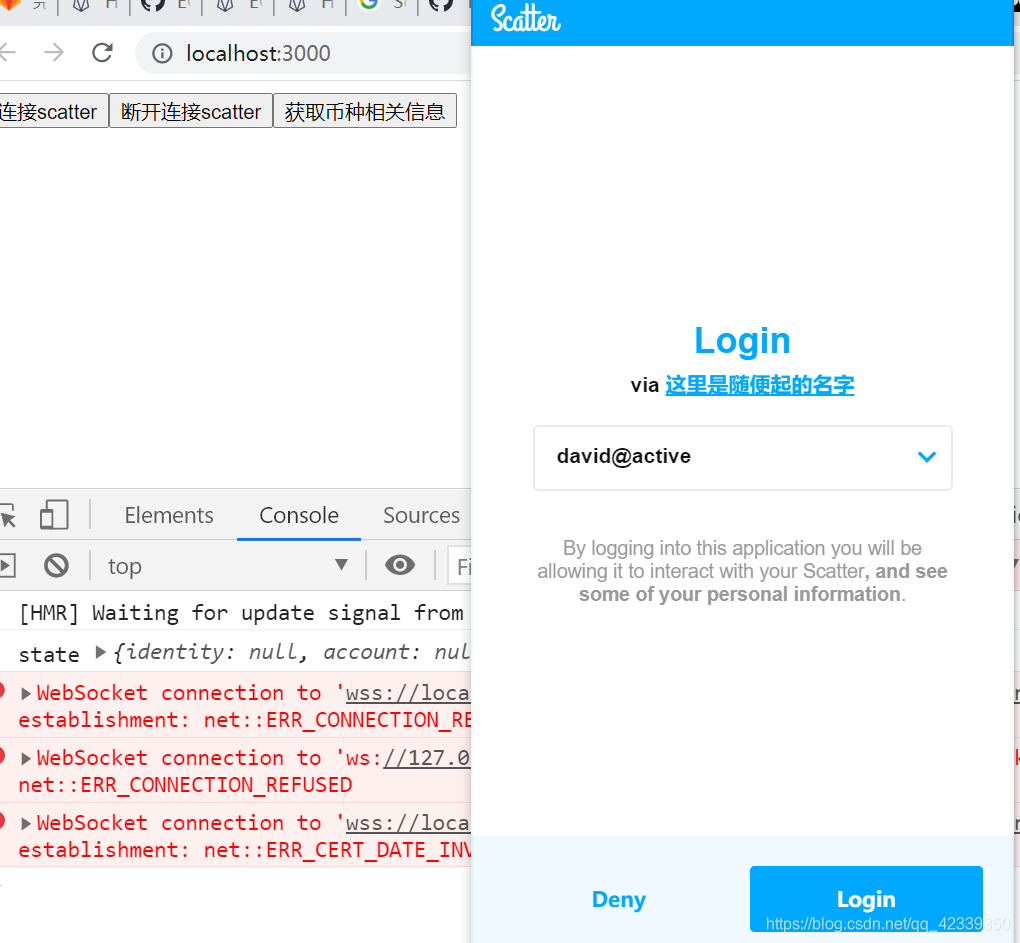
获取到的数据
退出登录
//断开连接,用户登出
function forgetIdentity(scatter) {
//主要就是调用scatter的这个方法
scatter.forgetIdentity()
//都清空掉
setScatter(null)
setState({
identity:null,
account:null,
eos:null,
})
}
查询余额
//查询账户余额
function getCurrencyBalance(account) {
(async () => {
console.log('EOS',await rpc.get_currency_balance('eosio.token', account.name, 'EOS'));
})();
(async () => {
console.log('HODLC',await rpc.get_currency_balance('eosio.token', account.name, 'HODLC'));
})();
(async () => {
console.log('HODLT',await rpc.get_currency_balance('eosio.token', account.name, 'HODLT'));
})();
}
获取到的数据
Faq
1. 怎么没用到eos这个值呢?,查询你用的是rpc啊
const eos = scatter.eos(network, Api);//a reference to an eosjs object
const rpc = new JsonRpc(network.fullhost());
这就涉及到这两个东西的介绍了.
- JsonRpc 主要是用来查询数据,与交易无关,就比如余额,块信息,交易信息啥的,你甚至都不用登录的,只要知道账户名字就能查到相关信息
- Api 主要是和交易相关的操作,肯定要用户授权的就是登录。
上面我就是简单的查了下余额所以也用不到eos呀
相关地址
Scatter开发文档(大概看看,写的是真的一言难尽)
eosjs开发文档(提供了各种api和rpc方法)