1、html部分
<template>
<div id="playArea" class="container">
<div id="mapContainer"></div>
<div class="mapBtn">
<el-button
v-if="isEditStatus"
class="custom green button map-btn"
@click="editPoint"
>编辑范围</el-button>
<el-button
v-else
class="custom green button map-btn"
@click="addPoint"
>添加范围</el-button>
<el-button
class="custom green button map-btn"
@click="savePoint"
>保存范围</el-button>
</div>
</div>
</template>
2、js部分:实现对某一个街道进行手动勾画区域并保存,保存结束后并支持修改区域。
export default {
data() {
return {
polygonPointsEntities: [], // 区域的点位集合
polygonPointsEntitiesLine: [], // 连线的多点
polygonPointsEntitiesEntity: [], // 区域查询所画线实体
pointPositionList: [], // 画点的经纬度集合
editPoints: [], // 编辑时编辑点集合,删除点用
isAddStatus:false, // 是否是添加区域
isEditStatus:false, // 是否是编辑区域
spaceArea:"", // 区域范围坐标
polygonEntity:null, // 区域面实体
isSaved:false, // 保存状态
viewer: null, // 地图实例化
curBillboard: null, // 当前高亮的广告牌
entityMarkerList: [], // 地图上点的资源实体对象集合
companyPoint:[], // 企业上点数据
// 标注图层
tdtImgAnnoLayerProvider: new Cesium.WebMapTileServiceImageryProvider(
{
url:
"http://t6.tianditu.com/cia_w/wmts?service=wmts&request=GetTile&version=1.0.0&LAYER=cia&tileMatrixSet=w&TileMatrix={TileMatrix}&TileRow={TileRow}&TileCol={TileCol}&style=default&format=tiles&tk=b6b320a7ccfabfdc30536330efc07f3e",
layer: "tdtImgAnnoLayer",
style: "default",
format: "image/jpeg",
tileMatrixSetID: "GoogleMapsCompatible",
}
),
}
},
props: ["parentRow"],
mounted() {
this.initMap(119.666612,34.249119,40000);
if(this.parentRow.id){
this.getGridDetail();
}
},
methods: {
// 获取网格信息
getGridDetail(){
this.axios.get(`${gridSettingManagement.detail}/${this.parentRow.id}`).then(res =>{
if(res.data.returnCode === "0000000" && res.data.data.coordinate){
let path = [];
this.pointPositionList = res.data.data.coordinate.split(',');
this.pointPositionList.map((item) => {
path.push(Number(item));
});
this.polygonEntity = this.viewer.entities.add({
name: 'polygon',
polygon: {
hierarchy: Cesium.Cartesian3.fromDegreesArray(path),
material: new Cesium.Color.fromCssColorString(
"#4296FF"
).withAlpha(0.4),
},
range: path,
});
this.isEditStatus = true;
}
});
},
// 保存范围
savePoint(){
let poinstArrString = this.pointPositionList.join(",");
let query = {
streetId:this.parentRow.id,
coordinate:poinstArrString
};
this.axios.post(gridSettingManagement.saveSpace,query).then(res =>{
if(res.data.returnCode === "0000000"){
this.$message({ type: "success", message: "保存成功!" });
this.$emit("hideDialog");
}
});
},
// 编辑范围
editPoint(){
this.polygonEntity.polygon.hierarchy._value.positions.forEach(
(item) => {
let cartesian = new Cesium.Cartesian3(
item.x,
item.y,
item.z,
item.z
);
let point = this.viewer.entities.add({
name: 'gon_point',
position: cartesian,
point: {
color: Cesium.Color.WHITE,
pixelSize: 10,
outlineColor: Cesium.Color.BLACK,
outlineWidth: 1,
},
});
this.editPoints.push(point);
this.polygonPointsEntities.push(cartesian);
this.viewer.scene.screenSpaceCameraController.enableRotate = false;
this.viewer.scene.screenSpaceCameraController.enableZoom = false;
}
);
},
// 添加范围
addPoint(){
if (this.polygonEntity && !this.isSaved) {
this.$message({
type: 'warning',
message: '请先保存勾画的范围!',
});
return;
}
this.isSaved = false;
this.isAddStatus = true;
},
// 初始化地图(根据当前企业所在位置初始化中心位置)
initMap(longitude,latitude,height){
Cesium.Ion.defaultAccessToken =
"eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJqdGkiOiJmNjJkN2ZjNC04OTYxLTQyZmItYmM5Mi0yOTgyMzQxMjEzZGQiLCJpZCI6MTg5NTIsInNjb3BlcyI6WyJhc2wiLCJhc3IiLCJhc3ciLCJnYyIsInByIl0sImlhdCI6MTU4OTYyODkwMn0.ibuCu13_8ksLkyimUHR4e1LrWRb-_sfZrPMhSWSzJBg";
let viewer = new Cesium.Viewer("mapContainer", {
baseLayerPicker: false,
geocoder: false, // 是否显示地名查找控件
homeButton: false,
sceneModePicker: false, // 是否显示投影方式控件
selectionIndicator: false,
baseLayerPicker: false, // 是否显示图层选择控件
navigationHelpButton: false, // 是否显示帮助信息控件
animation: false, // 是否显示动画控件
// creditContainer: "credit",
timeline: false, // 是否显示时间线控件
fullscreenButton: false,
vrButton: false,
infoBox: false, // 是否显示点击要素之后显示的信息
// requestRenderMode: true, // 启用请求渲染模式
scene3DOnly: true, // 每个几何实例将只能以3D渲染以节省GPU内存
sceneMode: 3, // 初始场景模式 1 2D模式 2 2D循环模式 3 3D模式 Cesium.SceneMode
imageryProvider: new Cesium.WebMapTileServiceImageryProvider(
{
url:
"http://t0.tianditu.gov.cn/img_w/wmts?tk=b6b320a7ccfabfdc30536330efc07f3e",
layer: "img",
style: "default",
tileMatrixSetID: "w",
format: "tiles",
maximumLevel: 18,
}
),
});
this.viewer = viewer;
this.viewer._cesiumWidget._creditContainer.style.display =
"none";
this.viewer.scene.globe.depthTestAgainstTerrain = false; // 图标被遮挡
this.viewer.scene.fxaa = false;
// 设置后当相机高度达到设置的最大和最小高度时将不再放大和缩小
this.viewer.scene.screenSpaceCameraController.minimumZoomDistance = 0; // 相机的高度的最小值
this.viewer.scene.screenSpaceCameraController.maximumZoomDistance = 20000000; // 相机高度的最大值
// 天地图矢量标注图层
this.tdtImgAnnoLayer = this.viewer.imageryLayers.addImageryProvider(
this.tdtImgAnnoLayerProvider
);
this.viewer.imageryLayers.raiseToTop(this.tdtImgAnnoLayer);
// 定位到中心点
// this.flyTo([Number(longitude), Number(latitude)], height,0);
this.setQiantang();
// 关闭双击事件
this.viewer.cesiumWidget.screenSpaceEventHandler.removeInputAction(
Cesium.ScreenSpaceEventType.LEFT_DOUBLE_CLICK
);
this.addMouseListener(); // 地图鼠标移动事件
this.addMouseClick(); // 地图鼠标左键单击事件
this.addMouseRightClick(); // 地图鼠标右键点击事件
this.addMouseLeftDown(); // 地图鼠标左键压下事件
},
// 设置区域范围
setQiantang(){
let xiangshuiJson = require('../../../../assets/geoJson/xiangshui.json');
let pointArr = xiangshuiJson.features[0].geometry.coordinates[0][0]
let polygonArr = [];
for (let i = 0;i < pointArr.length;i++) {
polygonArr.push(pointArr[i][0]);
polygonArr.push(pointArr[i][1]);
}
let materialPoline = colorRgba('#6495ED', 0.6);
// 创建线实例
let polyline = {
type: 'quyuPolyline',
polyline: {
positions: Cesium.Cartesian3.fromDegreesArray(polygonArr),
width: 6,
material: new Cesium.Color(
Number(materialPoline[0] / 255),
Number(materialPoline[1] / 255),
Number(materialPoline[2] / 255),
Number(materialPoline[3])
),
},
};
this.viewer.entities.add(polyline);
this.flyTo([Number(119.666612), Number(34.249119)], 100000,0);
},
// 类数组转化
listToArray(likeArray) {
var ary = [];
try {
ary = Array.prototype.slice.call(likeArray);
} catch (e) {
for (var i = 0; i < likeArray.length; i++) {
ary[ary.length] = likeArray[i];
}
}
return ary;
},
// 鼠标左键压下事件
addMouseLeftDown(){
let that = this;
let currentPoint = null;
const handler = new Cesium.ScreenSpaceEventHandler(
that.viewer.scene.canvas
);
handler.setInputAction((event) => {
let windowPosition = event.position;
let pickedObject = that.viewer.scene.pick(windowPosition);
if (Cesium.defined(pickedObject)) {
let entity = pickedObject.id;
if (entity.name === 'gon_point') {
currentPoint = entity;
}
}
}, Cesium.ScreenSpaceEventType.LEFT_DOWN);
// 对鼠标移动事件的监听
handler.setInputAction((event) => {
if (currentPoint) {
let cartesian = that.viewer.camera.pickEllipsoid(
event.startPosition,
that.viewer.scene.globe.ellipsoid
);
let points = [],
gon = null;
if (!cartesian) {
return;
}
currentPoint.position = cartesian;
for (let point of that.editPoints) {
points.push(point.position._value);
}
that.polygonPointsEntities = points;
that.polygonEntity.polygon.hierarchy = new Cesium.CallbackProperty(
() => {
return new Cesium.PolygonHierarchy(points);
},
false
);
that.pointPositionList = that.getLolat(points);
}
}, Cesium.ScreenSpaceEventType.MOUSE_MOVE);
// 对鼠标抬起事件的监听
handler.setInputAction((event) => {
currentPoint = null;
}, Cesium.ScreenSpaceEventType.LEFT_UP);
},
// cesium 笛卡尔坐标系转WGS84坐标系
getLolat(position) {
if (position) {
let gonPoints = [];
position.forEach((point, index) => {
var cartesian = new Cesium.Cartesian3(
point.x,
point.y,
point.z
);
var cartographic = Cesium.Cartographic.fromCartesian(
cartesian
);
var lat = Cesium.Math.toDegrees(
cartographic.latitude
).toFixed(6);
var lng = Cesium.Math.toDegrees(
cartographic.longitude
).toFixed(6);
var alt = cartographic.height;
gonPoints.push(lng);
gonPoints.push(lat);
});
return gonPoints;
} else {
return [];
}
},
// 鼠标右键点击事件
addMouseRightClick() {
let that = this;
that.viewer.screenSpaceEventHandler.setInputAction(function (
movement
) {
// 结束区域查询
that.isAddStatus = false;
for (let point of that.editPoints) {
// 去除白点
that.viewer.entities.remove(point);
}
let newpolygonPointsEntitiesLine = [];
// 闭合
newpolygonPointsEntitiesLine = that.polygonPointsEntitiesLine.push(
that.polygonPointsEntitiesLine[0]
);
newpolygonPointsEntitiesLine = that.listToArray(
that.polygonPointsEntitiesLine
);
let polylineLast = that.viewer.entities.add({
name: "线几何对象",
polyline: {
positions: newpolygonPointsEntitiesLine,
width: 3,
material: new Cesium.PolylineGlowMaterialProperty({
color: Cesium.Color.GOLD,
}),
depthFailMaterial: new Cesium.PolylineGlowMaterialProperty(
{
color: Cesium.Color.GOLD,
}
),
},
});
that.polygonPointsEntitiesEntity.push(polylineLast);
},
Cesium.ScreenSpaceEventType.RIGHT_CLICK);
},
// 鼠标左键点击事件
addMouseClick(){
let that = this;
that.viewer.screenSpaceEventHandler.setInputAction(function (movement) {
// 添加区域情况
if(that.isAddStatus){
// 平面坐标(x,y)
let windowPosition = movement.position;
// 三维坐标(x,y,z)
let ellipsoid = that.viewer.scene.globe.ellipsoid;
let cartesian = that.viewer.camera.pickEllipsoid(
windowPosition,
that.viewer.scene.globe.ellipsoid
);
// 坐标转换:世界坐标转换为经纬度
let cartographic = Cesium.Cartographic.fromCartesian(
cartesian
);
let lng = Cesium.Math.toDegrees(cartographic.longitude); // 经度
let lat = Cesium.Math.toDegrees(cartographic.latitude); // 纬度
// 经度在前纬度在后
that.pointPositionList.push(lng.toFixed(6));
that.pointPositionList.push(lat.toFixed(6));
if (!cartesian) {
return;
}
// 实例化点实体
let point = that.viewer.entities.add({
name: "gon_point",
position: cartesian,
point: {
color: Cesium.Color.WHITE,
pixelSize: 10,
outlineColor: Cesium.Color.BLACK,
outlineWidth: 1,
},
});
// 存储点的实体,用于下图
that.editPoints.push(point);
that.polygonPointsEntities.push(cartesian);
that.polygonPointsEntitiesLine = that.listToArray(
that.polygonPointsEntities
);
if (that.editPoints.length > 0) {
if (that.polygonPointsEntitiesLine.length < 1) return;
let polyline = that.viewer.entities.add({
name: "线几何对象",
polyline: {
positions: that.polygonPointsEntitiesLine,
width: 3,
material: new Cesium.PolylineGlowMaterialProperty(
{
color: Cesium.Color.GOLD,
}
),
depthFailMaterial: new Cesium.PolylineGlowMaterialProperty(
{
color: Cesium.Color.GOLD,
}
),
},
});
// 存储线的实体,用于下图
that.polygonPointsEntitiesEntity.push(polyline);
}
// 大于三个点开始画面
if (that.polygonPointsEntities.length >= 3) {
if (!that.polygonEntity) {
that.polygonEntity = that.viewer.entities.add({
name: "polygon",
polygon: {
hierarchy: new Cesium.CallbackProperty(
() => {
return new Cesium.PolygonHierarchy(
that.polygonPointsEntities
);
},
false
),
material: new Cesium.Color.fromCssColorString(
"#4296FF"
).withAlpha(0.4),
},
});
} else {
that.polygonEntity.polygon.hierarchy = new Cesium.CallbackProperty(
() => {
return new Cesium.PolygonHierarchy(
that.polygonPointsEntities
);
},
false
);
}
}
}
},Cesium.ScreenSpaceEventType.LEFT_CLICK);
},
// 鼠标移入事件
addMouseListener(){
let that = this;
const handler = new Cesium.ScreenSpaceEventHandler(
that.viewer.scene.canvas
);
handler.setInputAction(function (movement) {
if (that.isEditStatus || that.isAddStatus) {
document.getElementsByTagName("body").item(0).style.cursor =
"pointer";
} else {
document.getElementsByTagName("body").item(0).style.cursor =
"default";
}
}, Cesium.ScreenSpaceEventType.MOUSE_MOVE);
},
// 地图移动
flyTo(coord = [116.405285, 40.123456], height = 450000,time = 0) {
this.viewer.camera.flyTo({
destination: Cesium.Cartesian3.fromDegrees(
coord[0],
coord[1],
height
),
orientation: {
heading: Cesium.Math.toRadians(0.0),
roll: 0.0,
},
duration: time,
});
},
}
};
3、实现效果图:
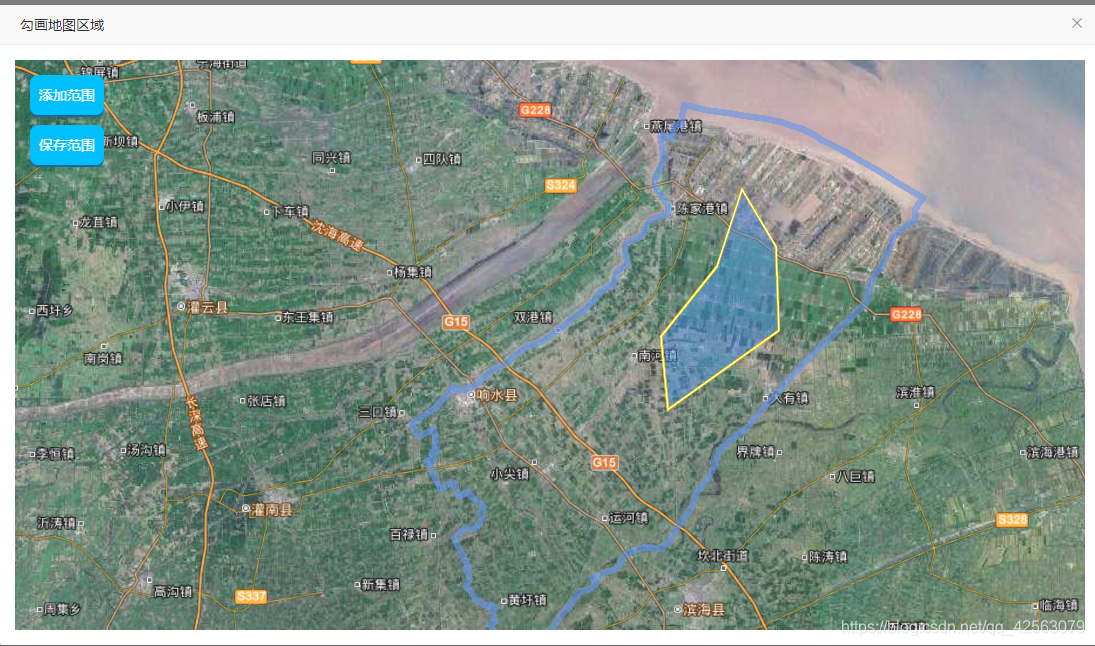
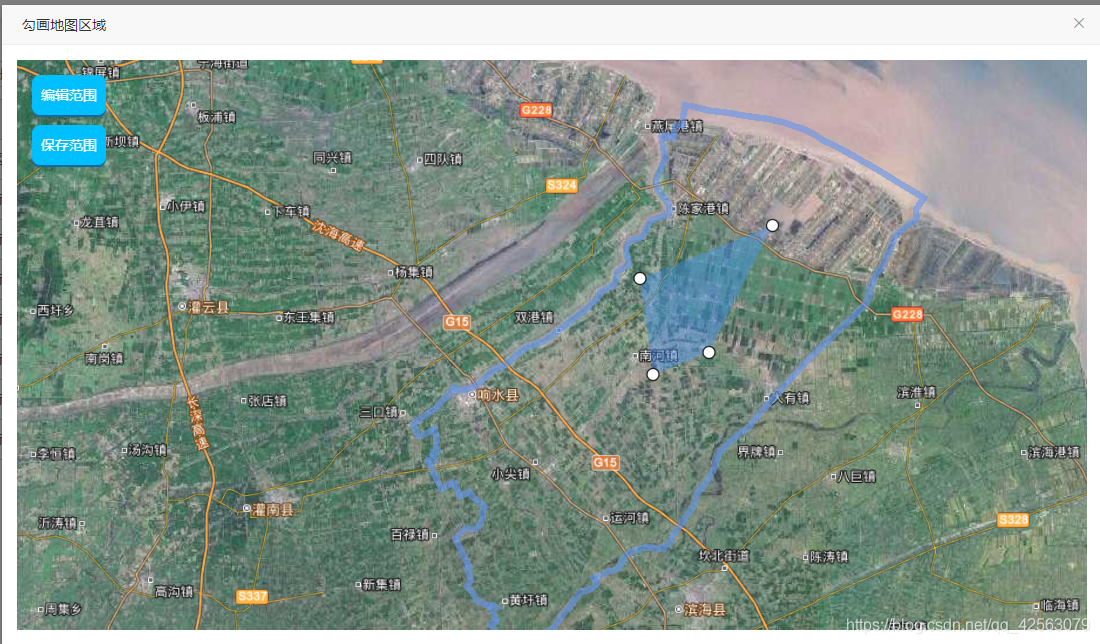