欢迎进入xx汽车租赁公司
请输入用户名
请输入密码
(用户名默认是名字缩写,密码是123,将登陆模块封装到方法中去调用方法)
请输入您的操作
1)查看现在车库中的所有车辆信息
2)租赁汽车
3)往车库中添加汽车
4)修改汽车租赁价格信息
用switch去判断操作
类分析
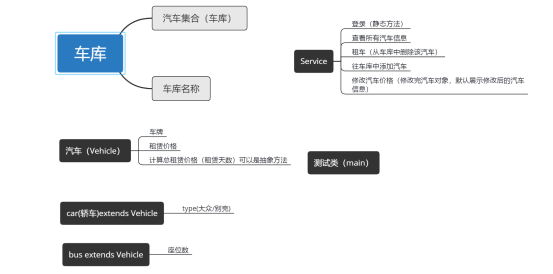 {
Wellcome();
}
public static void Wellcome(){
System.out.println("***********************************");
System.out.println("\t欢迎来到何老板图书馆 ");
System.out.println("***********************************");
// 获取用户信息
Tool.inputInfo();
}
}
package com.youjiuye.bms;
/*
* 汽车租赁系统的功能模块类
* 1、管理员添加车库中的车辆信息
* 2、用户租赁车辆
* 3、用户查看车库中的车辆
* 4、用户查看自己租赁的车辆
* 5、管理员修改车辆的价格
* 6、用户结算租金
*/
public class CRMSService {
// 1、管理员添加车库中的车辆信息
public boolean addVehicel(MotoVehicel mo){
boolean bo = false;
MotoVehicel[] ms = MotoVehicel.getMs();
if(ms.length > 0){
for (int i = 0; i < ms.length; i++) {
if(ms[i] == null){
ms[i] = mo;
bo = true;
System.out.println("添加成功!");
break;
}
}
}
return bo;
}
// 2、用户租赁车辆
public void rent(Users u,MotoVehicel mo){
MotoVehicel[] ms = u.getUms();
for (int i = 0; i < ms.length; i++) {
if(ms[i] == null){
ms[i] = mo;
break;
}
}
}
// 4、用户查看自己租赁的车辆
public boolean browse(Users u){
boolean bo = false;
MotoVehicel[] mo = u.getUms();
if(mo.length > 0){
for (int i = 0; i < mo.length; i++) {
if(mo[i] != null){
System.out.println(mo[i].toString());
bo = true;
}
}
}
return bo;
}
// 5、管理员修改车辆的价格
public boolean update(String no,double price){
boolean bo = false;
if(MotoVehicel.arrayExit()){
MotoVehicel[] ms = MotoVehicel.getMs();
for (int i = 0; i < ms.length; i++) {
if(ms[i] != null && ms[i].getNo().equals(no)){
ms[i].setRentPrice(price);
System.out.println("修改成功!");
System.out.println(ms[i]);
bo = true;
}
}
}else{
System.out.println("当前车库中还没有车辆");
}
return bo;
}
// 6、用户结算租金
public double settleAccount(Users u,int days){
double price = 0;
MotoVehicel[] mo = u.getUms();
if(mo.length > 0){
for (int i = 0; i < mo.length; i++) {
if(mo[i] != null){
price += mo[i].getRentPrice() * days;
}
}
}
return price;
}
// 删除车库中的车辆
public void delete(MotoVehicel moo){
MotoVehicel[] mo = MotoVehicel.getMs();
if(mo.length > 0){
for (int i = 0; i < mo.length; i++) {
if(mo[i] != null && mo[i].equals(moo)){
mo[i] = null;
}
}
}
}
// 根据车牌号来判断车库中是否含有该车辆
public boolean judgeExitMotoVehicel(String no){
boolean bo = false;
MotoVehicel[] ms = MotoVehicel.getMs();
if(ms.length >0){
for (int i = 0; i < ms.length; i++) {
if(ms[i].getNo().equals(no)){
bo = true;
break;
}
}
}
return bo;
}
}
package com.youjiuye.bms;
/*
* 汽车租赁系统的功能模块类
* 1、管理员添加车库中的车辆信息
* 2、用户租赁车辆
* 3、用户查看车库中的车辆
* 4、用户查看自己租赁的车辆
* 5、管理员修改车辆的价格
* 6、用户结算租金
*/
public class CRMSService {
// 1、管理员添加车库中的车辆信息
public boolean addVehicel(MotoVehicel mo){
boolean bo = false;
MotoVehicel[] ms = MotoVehicel.getMs();
if(ms.length > 0){
for (int i = 0; i < ms.length; i++) {
if(ms[i] == null){
ms[i] = mo;
bo = true;
System.out.println("添加成功!");
break;
}
}
}
return bo;
}
// 2、用户租赁车辆
public void rent(Users u,MotoVehicel mo){
MotoVehicel[] ms = u.getUms();
for (int i = 0; i < ms.length; i++) {
if(ms[i] == null){
ms[i] = mo;
break;
}
}
}
// 4、用户查看自己租赁的车辆
public boolean browse(Users u){
boolean bo = false;
MotoVehicel[] mo = u.getUms();
if(mo.length > 0){
for (int i = 0; i < mo.length; i++) {
if(mo[i] != null){
System.out.println(mo[i].toString());
bo = true;
}
}
}
return bo;
}
// 5、管理员修改车辆的价格
public boolean update(String no,double price){
boolean bo = false;
if(MotoVehicel.arrayExit()){
MotoVehicel[] ms = MotoVehicel.getMs();
for (int i = 0; i < ms.length; i++) {
if(ms[i] != null && ms[i].getNo().equals(no)){
ms[i].setRentPrice(price);
System.out.println("修改成功!");
System.out.println(ms[i]);
bo = true;
}
}
}else{
System.out.println("当前车库中还没有车辆");
}
return bo;
}
// 6、用户结算租金
public double settleAccount(Users u,int days){
double price = 0;
MotoVehicel[] mo = u.getUms();
if(mo.length > 0){
for (int i = 0; i < mo.length; i++) {
if(mo[i] != null){
price += mo[i].getRentPrice() * days;
}
}
}
return price;
}
// 删除车库中的车辆
public void delete(MotoVehicel moo){
MotoVehicel[] mo = MotoVehicel.getMs();
if(mo.length > 0){
for (int i = 0; i < mo.length; i++) {
if(mo[i] != null && mo[i].equals(moo)){
mo[i] = null;
}
}
}
}
// 根据车牌号来判断车库中是否含有该车辆
public boolean judgeExitMotoVehicel(String no){
boolean bo = false;
MotoVehicel[] ms = MotoVehicel.getMs();
if(ms.length >0){
for (int i = 0; i < ms.length; i++) {
if(ms[i].getNo().equals(no)){
bo = true;
break;
}
}
}
return bo;
}
}
package com.youjiuye.bms;
public class Users {
private String identity;
private String password;
// 存放租赁的车辆信息
private MotoVehicel[] ums = new MotoVehicel[10];
public MotoVehicel[] getUms() {
return ums;
}
public void setUms(MotoVehicel[] ums) {
this.ums = ums;
}
public Users(){}
public Users(String identity, String password) {
super();
this.identity = identity;
this.password = password;
}
public String getIdentity() {
return identity;
}
public void setIdentity(String identity) {
this.identity = identity;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
@Override
public String toString() {
return "Users [identity=" + identity + ", password=" + password + "]";
}
}
package com.youjiuye.bms;
/*
* 所有车的父类
*
*/
public abstract class MotoVehicel {
private String no;
private String brand;
private String Color;
private int mileage;
private double rentPrice;
private static MotoVehicel[] ms= new MotoVehicel[10];
public MotoVehicel(){}
public MotoVehicel(String no, String brand, String color, int mileage, double rentPrice) {
super();
this.no = no;
this.brand = brand;
Color = color;
this.mileage = mileage;
this.rentPrice = rentPrice;
}
public String getNo() {
return no;
}
public void setNo(String no) {
this.no = no;
}
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
public String getColor() {
return Color;
}
public void setColor(String color) {
Color = color;
}
public int getMileage() {
return mileage;
}
public void setMileage(int mileage) {
this.mileage = mileage;
}
public double getRentPrice() {
return rentPrice;
}
public void setRentPrice(double rentPrice) {
this.rentPrice = rentPrice;
}
// 获取车库数组
public static MotoVehicel[] getMs() {
return ms;
}
// 租赁功能
public abstract double rent(int days);
// 初始化车库数组
public static final void init(){
Car c1 = new Car("001", "bwm","蓝色",10000, 500,"x5");
ms[0] = c1;
Bus b1 = new Bus("8567", "景龙", "绿色",2000, 800,16);
ms[1] = b1;
}
// 判断当前车库是否有车存在
public static boolean arrayExit(){
boolean bo = false;
if(ms.length > 0){
for (int i = 0; i < ms.length; i++) {
if(ms[i] != null){
bo = true;
}
}
}else{
bo = false;
}
return bo;
}
// 显示车库中现有的车辆
public static void show(){
System.out.println("当前车库的车:");
if(arrayExit()){
for (int i = 0; i < ms.length; i++) {
if(ms[i] != null){
System.out.println(ms[i]);
}
}
}else{
System.out.println("当前车库中没有车辆");
}
}
}
```java
package com.youjiuye.bms;
/*
* 公交车
*/
public class Bus extends MotoVehicel{
private int seatCount;
public Bus(){}
public Bus(String no, String brand, String color, int mileage, double rentPrice,int seatCount) {
super(no, brand, color, mileage, rentPrice);
this.seatCount = seatCount;
}
public int getSeatCount() {
return seatCount;
}
public void setSeatCount(int seatCount) {
this.seatCount = seatCount;
}
@Override
public String toString() {
return "Bus [ 车牌号:"+ getNo()+"\t品牌:"+getBrand()+"\t座位数:"+getSeatCount()+"\t颜色:"+ getColor()+"\t里程:"+getMileage()+"\t日租价:"+getRentPrice()+ "]";
}
@Override
public double rent(int days) {
return days * getRentPrice();
}
}
package com.youjiuye.bms;
/*
* 小轿车
*/
public class Car extends MotoVehicel{
private String type;
public Car(){}
public Car(String no, String brand, String color, int mileage, double rentPrice,String type) {
super(no, brand, color, mileage, rentPrice);
this.type = type;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
@Override
public String toString() {
return "Car [ 车牌号: "+ getNo()+"\t品牌:"+getBrand()+"\t型号:"+getType()+"\t颜色:"+ getColor()+"\t里程:"+getMileage()+"\t日租价:"+getRentPrice()+ "]";
}
@Override
public double rent(int days) {
return days * getRentPrice();
}
}