1:引入pom
<!--阿里云对象存储 start-->
<dependency>
<groupId>com.aliyun.oss</groupId>
<artifactId>aliyun-sdk-oss</artifactId>
<version>2.8.3</version>
</dependency>
<!--OSS end-->
2:在yml文件里配置阿里云OSS信息
#OSS
oss:
endpoint: 阿里云控制台获取
keyid: 阿里云控制台获取
keysecret: 阿里云控制台获取
bucketname: 阿里云控制台获取
filehost: test #bucket下文件夹的路径
3:创建ConstantProperties来获取yml文件的信息
@Data
@Component
@ConfigurationProperties(prefix = "oss")
public class ConstantProperties {
private String endpoint;
private String keyid;
private String keysecret;
private String bucketname;
private String filehost;
省略set get 。。。。
}
4:创建文件上传Util
@Service
public class AliyunOSSUtil {
@Autowired
private ConstantProperties constantProperties;
private static SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd");
/**
* 上传
* @param file
* @return
*/
public String upload(File file){
System.out.println("=========>OSS文件上传开始:"+file.getName());
String endpoint= constantProperties.getEndpoint();
String accessKeyId= constantProperties.getKeyid();
String accessKeySecret=constantProperties.getKeysecret();
String bucketName=constantProperties.getBucketname();
String fileHost=constantProperties.getFilehost();
System.out.println(endpoint+"endpoint");
SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd");
String dateStr = format.format(new Date());
if(null == file){
return null;
}
OSSClient ossClient = new OSSClient(endpoint,accessKeyId,accessKeySecret);
try {
//容器不存在,就创建
if(! ossClient.doesBucketExist(bucketName)){
ossClient.createBucket(bucketName);
CreateBucketRequest createBucketRequest = new CreateBucketRequest(bucketName);
createBucketRequest.setCannedACL(CannedAccessControlList.PublicRead);
ossClient.createBucket(createBucketRequest);
}
//创建文件路径
String fileUrl = (dateStr + "/" + UUID.randomUUID().toString().replace("-","")+"-"+file.getName());
//上传文件
PutObjectResult result = ossClient.putObject(new PutObjectRequest(bucketName, fileUrl, file));
//设置权限 这里是公开读
ossClient.setBucketAcl(bucketName,CannedAccessControlList.PublicRead);
if(null != result){
String url=bucketName+"."+endpoint+"/"+fileUrl;
//System.out.println("==========>OSS文件上传成功,OSS地址:"+ url);
return url;
}
}catch (OSSException oe){
oe.getMessage();
}catch (ClientException ce){
ce.getMessage();
}finally {
//关闭
ossClient.shutdown();
}
return null;
}
/**
* 删除
* @param fileKey
* @return
*/
public String deleteBlog(String fileKey){
System.out.println("=========>OSS文件删除开始");
String endpoint= constantProperties.getEndpoint();
String accessKeyId= constantProperties.getKeyid();
String accessKeySecret=constantProperties.getKeysecret();
String bucketName=constantProperties.getBucketname();
String fileHost=constantProperties.getFilehost();
try {
OSSClient ossClient = new OSSClient(endpoint,accessKeyId,accessKeySecret);
if(!ossClient.doesBucketExist(bucketName)){
System.out.println("==============>您的Bucket不存在");
return "您的Bucket不存在";
}else {
System.out.println("==============>开始删除Object");
ossClient.deleteObject(bucketName,fileKey);
System.out.println("==============>Object删除成功:"+fileKey);
return "==============>Object删除成功:"+fileKey;
}
}catch (Exception ex){
System.out.println("删除Object失败"+ex);
return "删除Object失败";
}
}
/**
* 查询文件名列表
* @param bucketName
* @return
*/
public List<String> getObjectList(String bucketName){
List<String> listRe = new ArrayList<>();
String endpoint= constantProperties.getEndpoint();
String accessKeyId= constantProperties.getKeyid();
String accessKeySecret=constantProperties.getKeysecret();
try {
System.out.println("===========>查询文件名列表");
OSSClient ossClient = new OSSClient(endpoint,accessKeyId,accessKeySecret);
ListObjectsRequest listObjectsRequest = new ListObjectsRequest(bucketName);
//列出11111目录下今天所有文件
listObjectsRequest.setPrefix("11111/"+format.format(new Date())+"/");
ObjectListing list = ossClient.listObjects(listObjectsRequest);
for(OSSObjectSummary objectSummary : list.getObjectSummaries()){
System.out.println(objectSummary.getKey());
listRe.add(objectSummary.getKey());
}
return listRe;
}catch (Exception ex){
System.out.println("==========>查询列表失败"+ex);
return new ArrayList<>();
}
}
}
5:上传到OSS返回URL
/**
* 文件上传
* @param file
* @return
*/
public String updateImg(List<MultipartFile> file){
List list=new ArrayList();
String str ="";
try {
if (null != file) {
for (int i=0;i<file.size();i++){
MultipartFile f=file.get(i);
String filename = f.getOriginalFilename();
if (!"".equals(filename.trim())) {
File newFile = new File(filename);
FileOutputStream os = new FileOutputStream(newFile);
os.write(f.getBytes());
os.close();
f.transferTo(newFile);
//上传到OSS
String uploadUrl = aliyunOSSUtil.upload(newFile);
list.add(uploadUrl);
}
}
str = StringUtils.join(list, ",");
/* String[] strs=str.split(",");
for (int i=0;i<strs.length;i++){
list1.add(strs[i]);
}
for (int i=0;i<list1.size();i++){
System.out.println(list1.get(i)+">>>>>>>>>>>>>>>>>>>>>>>>>>>>");
}*/
}
} catch (Exception ex) {
ex.printStackTrace();
}
return str;
}
自己开发的出门必备小程序可以扫码体验交流交流
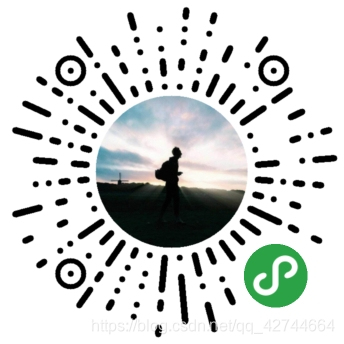